Function purpose:
remove duplicate code and increase code maintainability
Defining a function: def function name (): [It will not be executed when defining a function]
Call function: function name () [execute when calling function]
Documentation description:
The first line is described with a string
Formal parameters:
parameters when defining a function
Actual parameters:
parameters when calling a function
The role of parameters:
the same function can perform different effects
1 def text(formal parameter 1, formal parameter 2): 2 """ document description """ 3 pass 4 5 text(actual parameter 1, actual parameter 2) 6 help(text) #Product see document description
PS: The position and the number must be in one-to-one correspondence
Type of parameter:
1. Default parameters (assignment to formal parameters):
Parameters with default values. When calling a function, if no value is assigned to the default parameter, it is the default
Default parameters must be at the end of the formal parameter list
1 def printinfo(name, age=35): # age=35 : default parameter 2 # print any string passed in 3 print ( " name: %s " % name) 4 print ( " age %d " % age ) 5 6 #Call the printinfo function 7 printinfo(name= " miki " ) #age goes to the default value 35 during the execution of the function 8 printinfo(age=9 ,name= " miki " )
2. Positional parameters:
formal parameters and actual parameters need to be passed in one-to-one correspondence
3. Keyword parameters (named parameters):
specify the name of the formal parameter to be passed (can be unordered, used in the actual parameter)
4. Variable length parameters:
use an asterisk, which is received by a tuple (*args); use two asterisks, which are received by a dictionary (**kwargs)
If many values are variable-length parameters, in this case, the default parameters can be placed after *args, but if there are **kwargs, **kwargs must be the last
1 def functionname([formal_args,] *args, **kwargs): #Variable length parameter 2 """ function_docstring """ 3 function_suite 4 return [expression]
Notice:
The variable args with an asterisk (*) will store all unnamed variable arguments, and args is a tuple
The variable kwargs with ** will store named parameters, that is, parameters in the form of key=value, and kwargs is a dictionary.
The role of the return value: the
caller needs to use the function result (know its result)
return value:
1. return: will terminate the function immediately and return to the place where the function was called. Multiple returns only execute the first one
2, None: no data, the function has no return value
3. Multiple returns are allowed on different branches.
ps:
nothing is written in return in the function, it is specially used to terminate the function
Return can be followed by tuples, lists, dictionaries, etc. As long as it is a type that can store multiple data, multiple data can be returned at one time, then the default is a tuple
There are four types of functions:
-
No parameters, no return value
-
No parameters, return value
-
With parameters, no return value
-
parameter, return value
Nesting of functions:
A function calls another function
def testB( ): print ( ' ---- testB start---- ' ) print ( ' here is the code executed by the testB function... (omitted)... ' ) print ( ' ---- testB end ---- ' ) def testA (): print ( ' ---- testA start ---- ' ) testB( ) print('---- testA end----') testA ( ) #Execute
{If another function B is called in function A, then the tasks in function B will be executed before returning to the position where function A was executed last time}
local variable:
Definition: A variable defined inside a function, its scope is limited to the inside of the function, it can only be used inside the function, and cannot be used outside the function
Global variable:
Definition: A variable defined outside a function can be accessed in all functions
{is a global variable in the function, which actually creates a line local variable}
global:
declare in a function, modify global variables
# In a function, global variables and global variables cannot have the same name
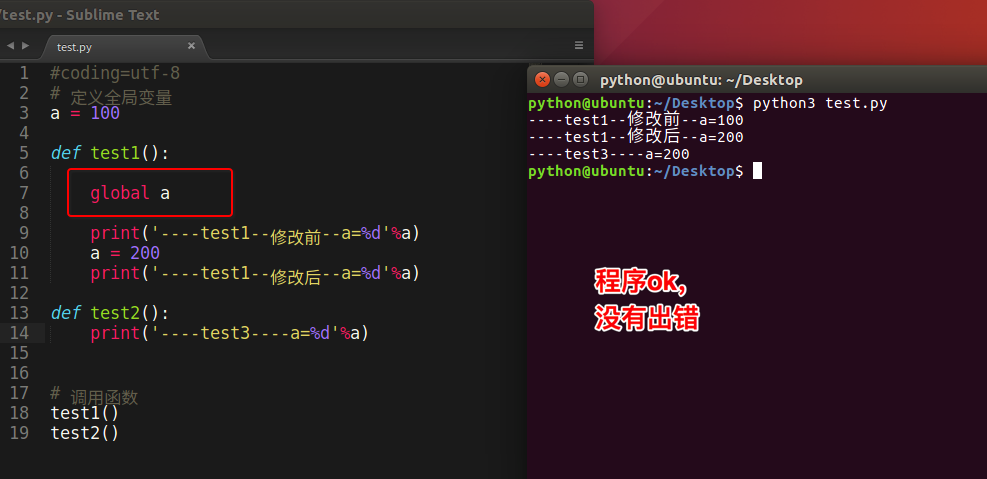
Packing and unpacking:
Packing: put multiple values in one data
Unpacking: splitting one into multiple values
In addition to unpacking tuples, you can also unpack lists, dictionaries, etc.
When unpacking, pay attention that the number of data to be unpacked should be the same as the number of variables, otherwise the program will be abnormal
>>a, b = (11, 22)
>> a = 11, b = 22
Swap the values of two variables: a,b = b,a
Attachment: 1. When passing actual parameters, write an asterisk in front of the list or tuple, you can split the list or tuple into separate
positional
parameters to pass
2. When passing actual parameters, write two asterisks before the dictionary, and the dictionary will be split into separate
keyword
parameters to pass