(1) LEGB of python:
LEGB means: variable search is carried out according to the order priority of L>E>G>B.
L: The internal scope of the local function, which is the bottom-level single function;
E: Between the inside of the enclosing function and the nested function, it is inside the function with the inner function;
G: global global scope, is a .py file;
B: build-in built-in scope, such as: tuple, list, tuple. is in all .py files.
(2) Closure
A closure means that a function is embedded with another function, and the embedded function will use the parameter variables of the external function as a reference for judging the different operating modes of the embedded function. Finally, the external function will return its embedded function, that is, the address of the function object, and this embedded function is what we call a closure. Use the following example to illustrate:
def set_passline(passline): def cmp(val): if val >= passerine: print('Pass') else: print('failed') return cmp f_100 = set_passline(60) f_100(89) f_150 = set_passline(96) f_150(89)
What set_passline returns is the function object of the built-in function cmp. Different arguments passline determine different cmp function objects. The built-in function cmp is also a closure.
In short, a closure refers to an embedded function that refers to an external function. Its main functions are: (1) encapsulation; (2) code reuse
In addition, the external function parameter referenced in the closure can also be a function. In this case, the function is to abstract the same processing part in multiple different functions, put it into the closure for encapsulation, and the closure passes in the function object, so that , after running the same processing part in the closure, you can run specific code for different functions. Examples are as follows:
def my_sum(*arg): print('my_sum') return sum(arg) def my_average(*arg): print('my_average') return sum(arg) / len(arg) def dec(func): #closure def in_dec (* arg): print ( ' in_dec()= ' , arg) # encapsulate the same processing department if len(arg) == 0: return 0 for val in arg : if not isinstance(val, int): return 0 #After processing the same department, return the specific processing of different functions return func(* arg) return in_dec my_sum = dec(my_sum) print(my_sum(1, 2, 3, 4, 5, 6)) print(my_sum(1, 2, 3, 4, 5, '6')) my_average = dec(my_average) print(my_average(1, 2, 3, 4, 5, 6)) print(my_average(1, 2, 3, 4, 5, '6'))
The running result is:
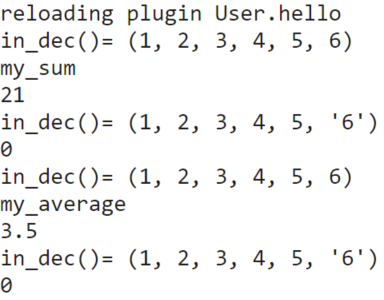
(3) Decorator
Decorators are essentially the use of closures to reuse code by encapsulating the same part of code or some decorative code (somewhat similar to the "decoration pattern" in design patterns). The closure is used explicitly through the decorator syntax sugar of the python interpreter (@dec, dec is the name of the external function of the closure), the function is passed into the closure as an argument, and finally the decorator returns the function decorated by the closure. To the decorated function, ie: bar = dec(bar). The usage example is as follows:
def deco(func): print("this is deco") def in_deco(x,y): print('this is closure') func(x,y) return in_deco @deco #The syntactic sugar of the decorator, the function below it will be passed into deco as a function object argument func, which is equivalent to calling bar = dec(bar) def bar(x,y): print ( " this is bar " ) print ( " %d+%d=%d " % (x,y,x+ y)) bar(2,4)
The results are as follows:
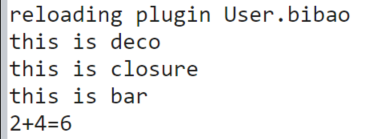