目录
本次作业的内容为:根据课程实操实现APP门户界面框架设计,至少包含4个tab页,能实现tab页之间的点击切换;
技术:使用布局(layouts)和分段(fragment),对控件进行点击监听。
APP界面效果展示:
实现过程:
注:所有定义以及相关赋值可在文章最后查看项目源码或点此链接查看(包括文中提到的onCreate函数)。
一、基本框架实现:
观察APP实现后的效果图,可见基本框架可分为三个部分:顶部title,中部的FrameLayout,底部的四个点击切换动作。
可知需要七个layout文件才能将完整的界面显示,分别为:顶部,底部,中部四个tag,以及一个总框架。中部四个tag会在后面点击切换部分展示,这里先看其他三个部分,可按以下顺序依次实现。
1.顶部top.xml:
顶部较为简单,只需一个TextView即可,最外层为一个水平的LinearLayout布局。
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:background="@color/black"
android:layout_height="wrap_content">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="80dp"
android:layout_weight="1"
android:gravity="center"
android:text="Wangkai Wechat"
android:textColor="@color/white"
android:textSize="30sp" />
</LinearLayout>
2.底部bottom.xml:
底部四个点击切换动作每个都需要一个ImageView和一个TextView,并且两个控件要被包含在同一个LinearLayout之中,最后四个LinearLayout的外层使用水平的LinearLayout布局。以下为其中一个LinearLayout的代码(其余三个替换图片和文字即可):
<LinearLayout
android:id="@+id/LinearLayout_wechat"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:gravity="center"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:src="@drawable/wechat2" />
<TextView
android:id="@+id/textView1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:text="微信"
android:textColor="@color/white"
android:textSize="25sp" />
</LinearLayout>
3.总框架activity_main.xml:
再创建一个Layout文件,使用垂直布局的LinearLayout,添加一个FrameLayout,设置其height=“0dp”、weight=“1”;将top和bottom的布局include进来,并放在FrameLayout的上下两边。这样基本的界面框架就做了出来。
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity" >
<include layout="@layout/top" />
<FrameLayout
android:id="@+id/id_content"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1">
</FrameLayout>
<include layout="@layout/bottom" />
</LinearLayout>
基本框架效果图:
二、点击切换动作的实现:
要想实现点击切换的效果,需要设置一个FragmentManager来对四个不同的tab进行控制,让他们叠加在一起的同时,实现在不同的点击动作发生时进行一个tab的显示及其余三个的隐藏。
1.fragmentManager标准化
首先定义一个FragmentManager的变量并写一个函数进行fragmentManager的标准化:
private FragmentManager fragmentManager;
private void initFragment(){
fragmentManager=getFragmentManager();
FragmentTransaction transaction=fragmentManager.beginTransaction(); //开始交互控制
transaction.add(R.id.id_content,wechatFragment); //把fragment加入到id_content
transaction.add(R.id.id_content,friendFragment);
transaction.add(R.id.id_content,contactFragment);
transaction.add(R.id.id_content,configFragment);
transaction.commit();
}
这里的transaction.add()函数的参数需要一个int类型和一个fragment类型,意为将把fragment加入到一个FrameLayout里,int值为FrameLayout的id。
根据本界面的框架,可知需要4个这样的fragment,那么就需要创建四个这样的Fragment类,比如第一个微信界面的类可以这样写:
package com.example.mywork;
import android.os.Bundle;
import android.app.Fragment;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
public class wechatFragment extends Fragment {
public wechatFragment() {
// Required empty public constructor
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
// 压缩器,把xml文件压缩到容器中
return inflater.inflate(R.layout.fragment_wechat, container, false);
}
}
这里用到了Inflater,可以将xml文件压缩到容器中,第一个参数就是一个Layout参数。前面讲了,我们需要四个tag页面,也就是四个Layout来实现中间部分,我们需要将四个fragment做出对应的Layout页面,这是上面微信界面fragment的Layout:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".wechatFragment">
<!-- TODO: Update blank fragment layout -->
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="这是微信聊天界面"
android:textSize="30dp"
android:gravity="center"/>
</LinearLayout>
在写完这些后,由于Java自导包的问题会导致报错,只需将import的包中与Fragment、FragmentManager、FragmentTransaction相关的包改为以下内容即可修复:
import android.app.Fragment; import android.app.FragmentManager; import android.app.FragmentTransaction;
将四个fragment和Layout写好之后,fragmentManager标准化也完成了,接下来就需要实现四个tag页面的显示、隐藏,以及点击动作的实现。
2.tag页面的显示、隐藏、点击切换
tag的显示与隐藏较好实现,使用函数即可(在show之前隐藏其他tag页面,这样就可做到点哪一个只显示哪一个,其余隐藏):
//tag页面的隐藏
private void hideFragment(FragmentTransaction transaction){
transaction.hide(wechatFragment);
transaction.hide(friendFragment);
transaction.hide(contactFragment);
transaction.hide(configFragment);
}
//tag页面的显示
private void showfragment(int i) {
FragmentTransaction transaction=fragmentManager.beginTransaction();
hideFragment(transaction);
switch (i){
case 0:
transaction.show(wechatFragment);
break;
case 1:
transaction.show(friendFragment);
break;
case 2:
transaction.show(contactFragment);
break;
case 3:
transaction.show(configFragment);
break;
default:
break;
}
transaction.commit();
}
现在只差对控件的点击监听了,各个LinearLayout的监听放在了onCreate函数中。这里用到了Onclick(),使用switch语句即可实现通过点击获得不同Layout的id来显示不同tag页面,如下:
@Override
public void onClick(View v) {
switch (v.getId()){
case R.id.LinearLayout_wechat:
showfragment(0);
break;
case R.id.LinearLayout_friend:
showfragment(1);
break;
case R.id.LinearLayout_contact:
showfragment(2);
break;
case R.id.LinearLayout_config:
showfragment(3);
break;
default:
break;
}
}
要注意的是,使用了Onclick()会有报错的情况,需要将主函数的函数头更改:
public class MainActivity extends AppCompatActivity{} 改为: public class MainActivity extends AppCompatActivity implements View.OnClickListener{}
做完这些,已经基本实现了此APP的功能,接下来做的就是对界面外观的一些调整了。
三、对外观结构的调整
1.点击切换图标和文本颜色
运行程序会发现,没有细节的处理会让使用起来很不舒服,在我们使用的微信中,点击切换时会发现底部的图标和字体颜色是会改变的,我这里使用的方法是找到下面四个图标的另一组不同颜色的图,如下图所示:
写一个函数,使用imageView.setImageResource和textView.setTextColor先把图片设置为灰色的并把文本设置为白色,然后再通过点击动作传入一个Layout的id选择相应的Layout进行图片的改变以及文本颜色设置(imageView1/2/3/4,textView1/2/3/4定义在onCreate中):
private void changeColor(int i){
imageView1.setImageResource(R.drawable.wechat2);
imageView2.setImageResource(R.drawable.friend2);
imageView3.setImageResource(R.drawable.contact2);
imageView4.setImageResource(R.drawable.config2);
textView1.setTextColor(Color.rgb(255, 255, 255));
textView2.setTextColor(Color.rgb(255, 255, 255));
textView3.setTextColor(Color.rgb(255, 255, 255));
textView4.setTextColor(Color.rgb(255, 255, 255));
switch (i){
case 0:
imageView1.setImageResource(R.drawable.wechat1);
textView1.setTextColor(Color.rgb(26,250,41));
break;
case 1:
imageView2.setImageResource(R.drawable.friend1);
textView2.setTextColor(Color.rgb(26,250,41));
break;
case 2:
imageView3.setImageResource(R.drawable.contact1);
textView3.setTextColor(Color.rgb(26,250,41));
break;
case 3:
imageView4.setImageResource(R.drawable.config1);
textView4.setTextColor(Color.rgb(26,250,41));
break;
default:
break;
}
}
这样的话,Onclick()也需要加上这个点击颜色变化的函数,即:
@Override
public void onClick(View v) {
switch (v.getId()){
case R.id.LinearLayout_wechat:
changeColor(0);
showfragment(0);
break;
case R.id.LinearLayout_friend:
changeColor(1);
showfragment(1);
break;
case R.id.LinearLayout_contact:
changeColor(2);
showfragment(2);
break;
case R.id.LinearLayout_config:
changeColor(3);
showfragment(3);
break;
default:
break;
}
}
这样就实现了点击切换图标和文本的颜色,效果图见文章开始的效果展示。
2.APP初始界面优化
使用以上的代码直接运行APP后,不进行任何操作的话中部四个tag页面会叠加在一起,类似于以下效果:
可以在初始化FragmentManager时,在提交前加上将四个tag隐藏的函数,即可实现APP初始界面为空白页,或者直接使用changeColor()和showfragment()函数,将其中一个tag显示:
private void initFragment(){
fragmentManager=getFragmentManager();
FragmentTransaction transaction=fragmentManager.beginTransaction(); //开始交互控制
transaction.add(R.id.id_content,wechatFragment); //把fragment加入到id_content
transaction.add(R.id.id_content,friendFragment);
transaction.add(R.id.id_content,contactFragment);
transaction.add(R.id.id_content,configFragment);
//隐藏4个tag
hideFragment(transaction);
//或者 changeColor(0);showfragment(0); 显示微信聊天页面
transaction.commit();
}
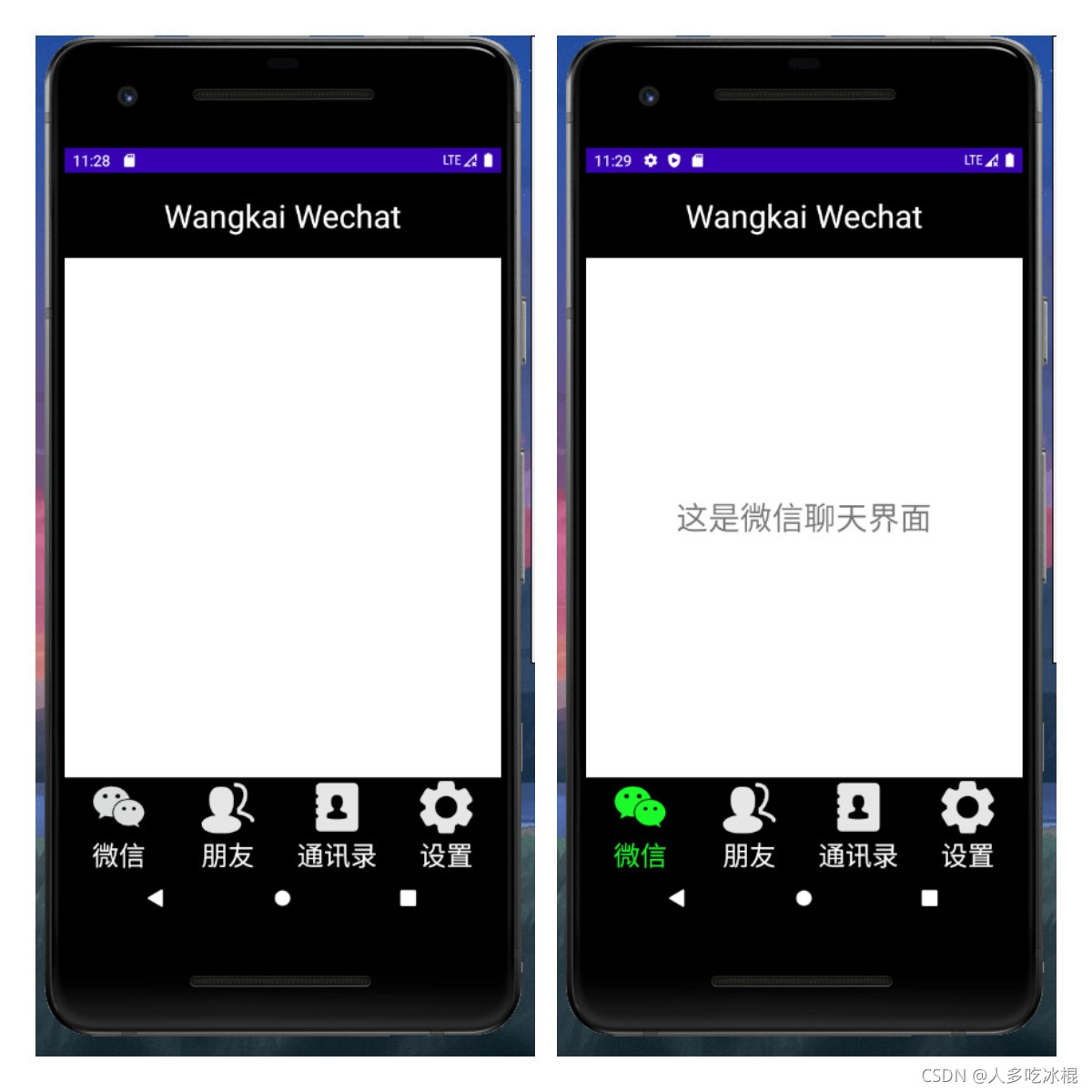
结语
本次作业大部分由老师指导完成,确实刚开始需要一段时间的学习,这次自己只独立完成了后面的外观结构调整部分,希望以后能自己独立完成更多内容。