This article mainly records the knowledge of using JDBC data sources in SpringBoot. If you are interested, learn it with the editor.
table of Contents
1. Code display
pom.xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jdbc</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
application.properties
spring.datasource.username=root
spring.datasource.password=123456
spring.datasource.url=jdbc:mysql://192.168.1.110:3306/jdbc
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
Test class, test the default connection data source
@SpringBootTest
class SpringBootJdbcApplicationTests {
@Autowired
DataSource dataSource;
@Test
void contextLoads() throws SQLException {
System.out.println(dataSource.getClass());
Connection connection = dataSource.getConnection();
System.out.println(connection);
connection.close();
}
}
Effect:
springboot 1.5.10 defaults to use org.apache.tomcat.jdbc.pool.DataSource as the data source;
springboot 2.4.3 defaults to use class com.zaxxer.hikari.HikariDataSource as the data source;
the relevant configuration of the data source is in Inside DataSourceProperties;
2. Principle of automatic configuration
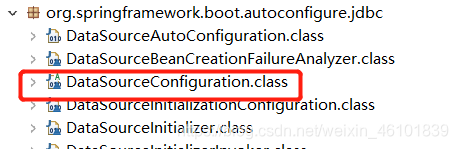
Refer to DataSourceConfiguration, create a data source according to the configuration, you can use spring.datasource.type to specify a custom data source type;
3. SpringBoot supports data sources by default
You can view DataSourceConfiguration in this configuration class
SpringBoot version 2.4.3 supports five data sources
1, org.apache.tomcat.jdbc.pool.DataSource
2, com.zaxxer.hikari.HikariDataSource
3, org.apache.commons.dbcp2.BasicDataSource
4, oracle.ucp. jdbc.PoolDataSource
5. You can also customize the data source
@Configuration(proxyBeanMethods = false)
@ConditionalOnMissingBean(DataSource.class)
@ConditionalOnProperty(name = "spring.datasource.type")
static class Generic {
@Bean
DataSource dataSource(DataSourceProperties properties) {
//使用DataSourceBuilder创建数据源,利用反射创建响应type的数据源,并且绑定相关属性
return properties.initializeDataSourceBuilder().build();
}
}
4. Commonly used mainstream open source database connection pool
Commonly used mainstream open source database connection pools include C3P0, DBCP, Tomcat Jdbc Pool, BoneCP, Druid, etc.
C3p0: The open source JDBC connection pool, which realizes the data source and JNDI binding, supports the JDBC3 specification and the standard extension of JDBC2. Open source projects currently using it include Hibernate, Spring, etc. Single thread, poor performance, suitable for small systems, the code is about 600KB.
DBCP (Database Connection Pool): A Java database connection pool project developed by Apache, Jakarta commons-pool object pool mechanism, the connection pool component used by Tomcat is DBCP. To use dbcp alone requires 3 packages: common-dbcp.jar, common-pool.jar, common-collections.jar. The database connection is placed in memory in advance. When the application needs to establish a database connection, it can directly apply for one in the connection pool. , Put it back when used up. Single thread, low concurrency, poor performance, suitable for small systems.
Tomcat Jdbc Pool: Tomcat used common-dbcp as the connection pool component before 7.0, but dbcp is single-threaded. In order to ensure thread safety, the entire connection pool is locked. The performance is poor. dbcp has more than 60 classes and is relatively complicated. . Tomcat has introduced a new connection pool module called Tomcat jdbc pool since 7.0. It is based on Tomcat JULI, uses the Tomcat log framework, is fully compatible with dbcp, obtains connections in an asynchronous manner, supports high concurrent application environments, and has only 8 super simple core files. JMX supports XA Connection.
BoneCP: The official statement BoneCP is an efficient, free, and open source Java database connection pool implementation library. The original intention of the design is to improve the performance of the database connection pool. According to some test data, BoneCP is the fastest, about 25 times faster than the second fastest connection pool at the time. It is perfectly integrated into some persistence products such as Hibernate and DataNucleus. in. BoneCP features: highly scalable and fast; callback mechanism for connection state switching; allows direct access to the connection; automatic reset capability; JMX support; lazy loading capability; support for XML and property file configuration methods; better Java code organization, 100% Unit test branch code coverage; the code is about 40KB.
Druid: Druid is the best database connection pool in the Java language. Druid can provide powerful monitoring and expansion functions. It is a high fault-tolerant, high-performance open source distributed system that can be used for big data real-time query and analysis, especially when it happens When code deployment, machine failure, and other product systems encounter downtime, Druid can still maintain 100% normal operation. Main features: Designed for analysis and monitoring; fast interactive query; high availability; extensible; Druid is an open source project, the source code is hosted on github.
5. Springboot starts to automatically execute sql
Add
schema.sql in application.properties to specify the file name
#spring某个版本之后需要加上这句,否则不会自动执行sql文件
spring.datasource.initialization-mode=always
# schema.sql中一般存放的是建表语句
spring.datasource.schema = classpath:schema.sql
# data.sql中一般存放的是需要插入更新等sql语句
spring.datasource.data = classpath:data.sql
6. Operate the database
JdbcTemplate operation database is automatically configured
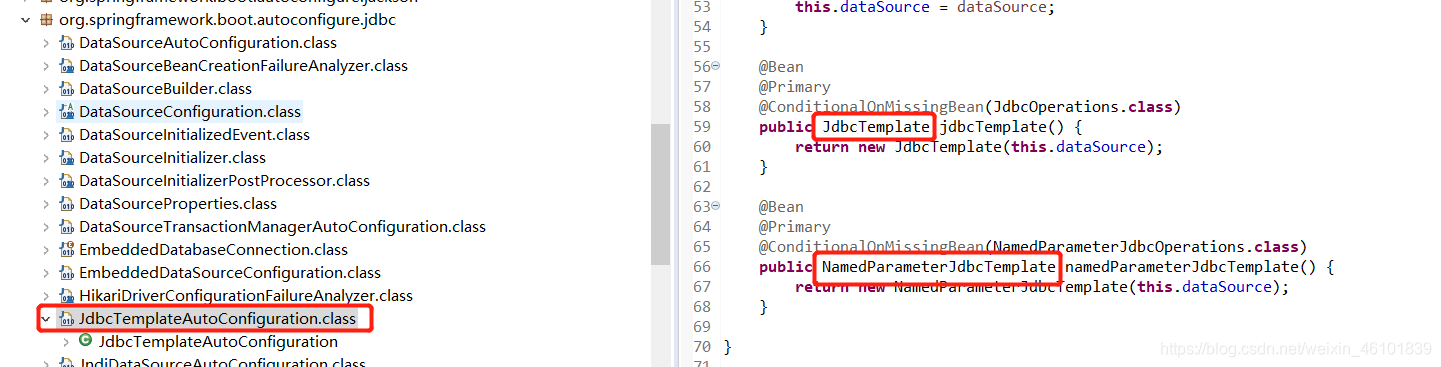
Simple usage
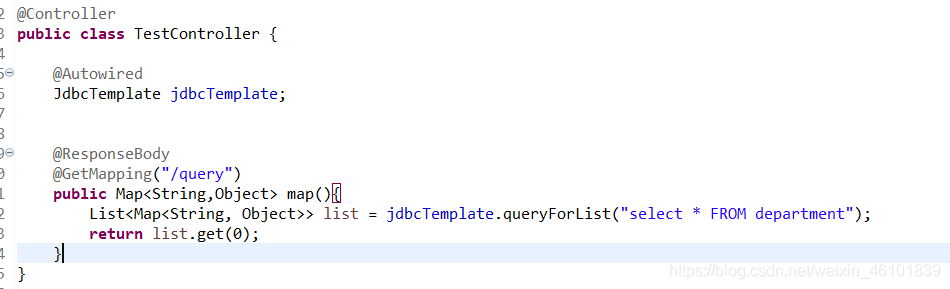