One: the characteristics of sequential storage of binary trees
Sequential binary trees usually only consider complete binary trees
- The left child node of the nth element is 2 * n + 1
- The right child node of the nth element is 2 * n + 2
- The parent node of the nth element is (n-1)/2
- n: represents the number of elements of the binary tree ( press 0 numbered as shown in Figure )
The sequential storage of a complete binary tree only needs to start from the root node, and store the nodes in the tree to the array in order according to the hierarchy.
From the perspective of data storage, the array storage method and the tree storage method can be converted to each other, that is, the array can be converted into a tree , and the tree can also be converted into an array.
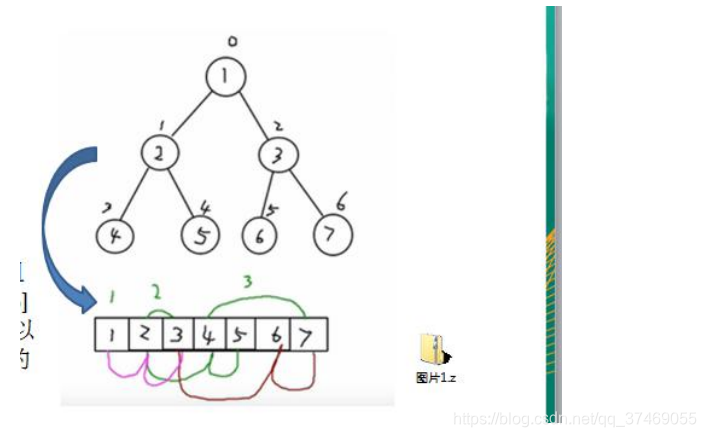
Request
:
- The node of the binary tree on the right requires an array to store arr: [1, 2, 3, 4, 5, 6, 6]
- It is required that when traversing the array arr , the nodes can still be traversed in the way of pre-order traversal, middle-order traversal and post-order traversal.
ArrayBinaryTree entity class
package com.tree;
/**
* @author lizhangyu
* @date 2021/3/11 22:19
*/
public class ArrayBinaryTree {
private int[] arr;
public ArrayBinaryTree(int[] arr) {
this.arr = arr;
}
/**
* 前序遍历顺序存储二叉树
* @param index
*/
public void preOrder(int index) {
if (arr == null || arr.length == 0) {
System.out.print("数组为空,不能按照二叉树的前序遍历");
return;
}
System.out.print(arr[index] + "\t");
if ((index * 2 + 1) < arr.length) {
preOrder(index * 2 + 1);
}
if (((index * 2 + 2) < arr.length)) {
preOrder(index * 2 + 2);
}
}
/**
* 中序遍历顺序存储二叉树
* @param index
*/
public void midOrder(int index) {
if (arr == null || arr.length == 0) {
System.out.print("数组为空,不能按照二叉树的前序遍历");
return;
}
if ((index * 2 + 1) < arr.length) {
midOrder(index * 2 + 1);
}
System.out.print(arr[index] + "\t");
if (((index * 2 + 2) < arr.length)) {
midOrder(index * 2 + 2);
}
}
/**
* 后序遍历顺序存储二叉树
* @param index
*/
public void postOrder(int index) {
if (arr == null || arr.length == 0) {
System.out.print("数组为空,不能按照二叉树的前序遍历");
return;
}
if ((index * 2 + 1) < arr.length) {
postOrder(index * 2 + 1);
}
if (((index * 2 + 2) < arr.length)) {
postOrder(index * 2 + 2);
}
System.out.print(arr[index] + "\t");
}
}
ArrayBinaryTreeDemo entity class
package com.tree;
/**
* @author lizhangyu
* @date 2021/3/11 22:27
*/
public class ArrayBinaryTreeDemo {
public static void main(String[] args) {
int[] arr = {1, 2, 3, 4, 5, 6, 7};
ArrayBinaryTree arrayBinaryTree = new ArrayBinaryTree(arr);
System.out.println("前序遍历结果为:");
arrayBinaryTree.preOrder(0);
System.out.println();
System.out.println("中序遍历结果为:");
arrayBinaryTree.midOrder(0);
System.out.println();
System.out.println("后序遍历结果为:");
arrayBinaryTree.postOrder(0);
}
}
operation result:
前序遍历结果为:
1 2 4 5 3 6 7
中序遍历结果为:
4 2 5 1 6 3 7
后序遍历结果为:
4 5 2 6 7 3 1