1. The method to stop the thread
There are 3 ways to stop the thread:
1. Use the exit flag to make the thread exit
2. Use the stop() method to forcefully terminate the thread, when the method is not recommended [expired method]
3. Use the interrupt() method to interrupt the thread
2.interrupt method
The effect of using the interrupt method is not to stop the thread immediately, but to make a stop mark
3. How to stop the thread specifically?
The following methods are described in the book "Java Multithreaded Programming Core Technology"
1. Abnormal method (recommended)
public class MyThread extends Thread {
@Override
public void run() {
try {
for (int i = 0; i < 500000; i++) {
if (MyThread.interrupted()){
System.out.println("已经是停止状态了,我要退出了!");
throw new InterruptedException();
}
System.out.println("i = " + (i+1));
}
System.out.println("如果我被输出了,则代表线程没有停止");
} catch (InterruptedException e) {
System.out.println("在MyThread类中的run方法中被捕获");
e.printStackTrace();
}
}
}
/**
* 根据中断状态退出for循环
*/
public class Main {
public static void main(String[] args) {
try {
MyThread myThread = new MyThread();
myThread.start();
Thread.sleep(100);
myThread.interrupt();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("end!");
}
}
The results are as follows: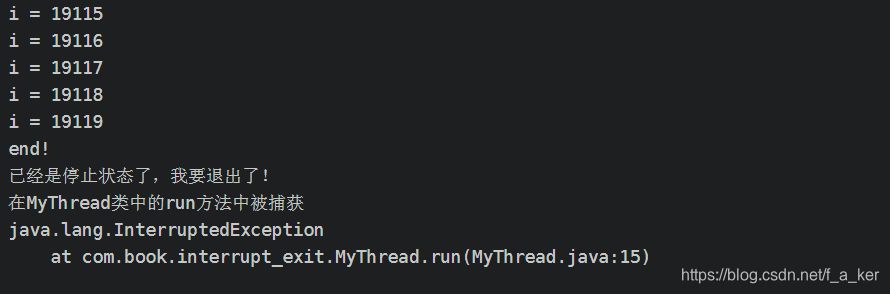
2. Stop the thread in sleep state
Sleep interruption is divided into two situations:
- Sleep the thread first, and then call interrupt to mark the interrupt state. Interrupt will interrupt the blocked thread. An interrupt exception will be thrown to achieve the effect of stopping the thread.
- The thread first calls interrupt to mark the interrupt state, and then the thread sleeps. An interrupt exception will be thrown to achieve the effect of stopping the thread.
1. Sleep first
public class MyThread extends Thread {
@Override
public void run() {
try {
System.out.println("run-----------start");
Thread.sleep(5000);
System.out.println("run-----------end");
} catch (InterruptedException e) {
System.out.println("在沉睡中被停止!进入catch,线程的是否处于停止状态:" + this.isInterrupted());
e.printStackTrace();
}
}
}
public class Main {
public static void main(String[] args) {
try {
MyThread myThread = new MyThread();
myThread.start();
Thread.sleep(2000);
System.out.println("状态:"+MyThread.interrupted());
myThread.interrupt();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
The results are as follows:
2. Mark the interrupt first
public class MyThread1 extends Thread {
@Override
public void run() {
try {
for (int i = 0; i < 100000; i++) {
System.out.println("i = " + (i+1));
}
System.out.println("run begin");
//interrupt是做一个中断标记,当时不会去中断正在运行的线程,当该线程处于阻塞状态时就会进行中断
//因此,先进行interrupt后,再遇到sleep阻塞时,才会进行中断
Thread.sleep(200000);
System.out.println("run end");
} catch (InterruptedException e) {
System.out.println("先停止,再遇到了sleep! 进入catch!");
e.printStackTrace();
}
}
}
public class Main1 {
public static void main(String[] args) {
MyThread1 myThread1 = new MyThread1();
myThread1.start();
myThread1.interrupt();
System.out.println("end!");
}
}
The results are as follows: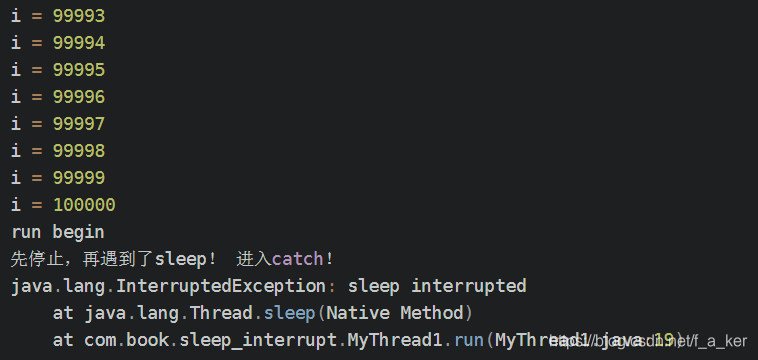
3.stop violent stop (not recommended)
4. The return statement stops the thread
- After calling interrupt marked as interrupted state, judge the current thread state in the run method, and return if it is interrupted state, which can achieve the effect of stopping the thread.
Remarks: It is recommended to use the "throwing exception" method to stop the thread, because the exception can also be thrown up in the catch block, so that the event of the thread stop can be propagated