Ready to work
vi sub1.c # 创建sub1.c文件
vi main1.c # 创建main1.c文件
Write sub1.c :
float x2x(int a, int b){
return a + b;
}
Write main1.c :
#include<stdio.h>
#include"sub1.c"
int main(){
int a = 2, b = 3;
printf("%f\n",x2x(a, b));
return 0;
}
Directly use gcc command to generate executable file
gcc -o main1 main.c # 将输出文件放入main1中
./main1 # 运行main1
The results are as follows:
Use MakeFile to compile and link
Create a makefile in the directory where you put the C program:
touch makefile # 创建makefile文件
Grammar rules:
target: prerequisites # target为需要生成的目标,prerequisites为依赖项
command # command为make需要执行的shell命令
Note: the command must start with the tab key
Edit the makefile:
#MakeFile
main1: main1.o sub1.o
main1.o main1.c sub1.h
cc -c main1.c
sub1.o: sub1.c sub1.h
cc -c sub1.c
clean:
rm *.o main1
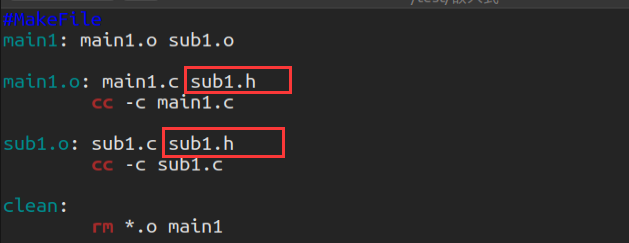
Note: When editing the makefile, the sub1.h header file is newly added, otherwise the following error will be reported when you run make to compile and link
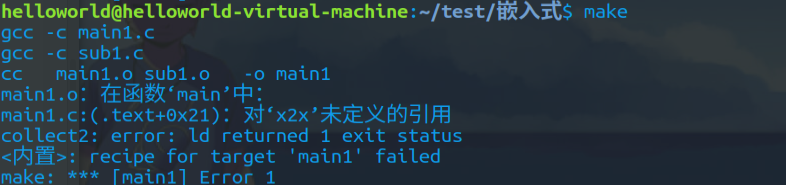
Add the sub1.h header file, as shown in the figure:

Modify the sub1.c source file, the content is as shown in the figure:
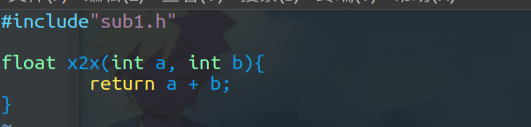
Modify the main1.c source file, the content is shown in the figure:
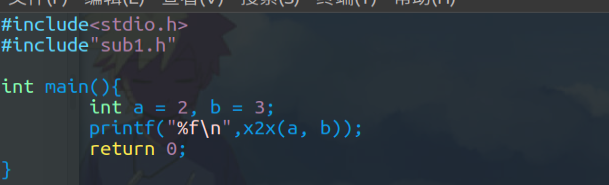
After completing the above modification, execute the following command:
make # 进行编译链接
./main1 # 运行main1

make clean # 删除所有 .o 文件和 main1 文件
Compile and link with the command line
gcc -c main1.c # 编译 main1.c 生成 main1.o
gcc -c sub1.c # 编译 sub1.c 生成 sub1.o
gcc -o main main1 mian1.o sub1.o # 链接 main1.o 和 sub1.o 生成 main1 可执行文件
./main1 # 运行 main1
The difference between -c and -o:
-c: Only compile and generate intermediate object files with the same name, without linking
- gcc -c main1.c (compile to generate main.o file)
-o: Specify the output file name, the file is an executable file, without -o will generate a.out by default
- gcc -o main1 main1.c (directly generate executable file main1)
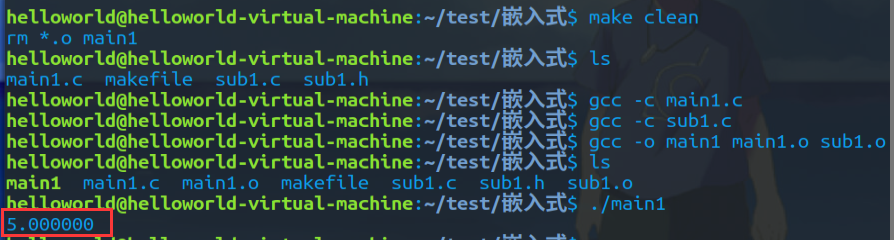
Use VS2017 to compile main1.c and run under Windows
-
Create an empty project
-
Create sub1.h, sub1.c, main1.c files respectively, the code content is the same as the content of the three pictures posted.
-
Click to run
At this time, an error of "error C4996" will be reported, which means that the scanf function or variable may be unsafe.
Solution: Define the macro before all include header files in main1.c: #define _CRT_SECURE_NO_DEPRECATE
-
After modification, re-run