Date: 2020-10-10
Author: Yi Dynasty 19th
Tag: JAVA fast and slow pointer
Title description
Given a linked list, return the first node where the linked list starts to enter the loop. If the linked list has no rings, null is returned.
In order to represent the rings in a given linked list, we use the integer pos to indicate the position where the end of the linked list is connected to the linked list (the index starts from 0). If pos is -1, there are no rings in the linked list. Note that pos is only used to identify the ring, and will not be passed to the function as a parameter.
Note: It is not allowed to modify the given linked list.
Advanced:
Can you solve this problem with O(1) (ie, constant) memory?
Example: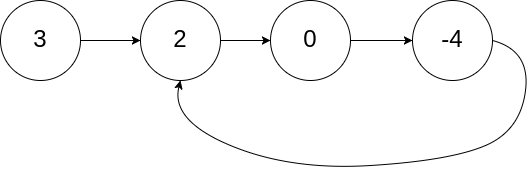
输入:head = [3,2,0,-4], pos = 1
输出:返回索引为 1 的链表节点
解释:链表中有一个环,其尾部连接到第二个节点。
输入:head = [1,2], pos = 0
输出:返回索引为 0 的链表节点
解释:链表中有一个环,其尾部连接到第一个节点。
Problem-solving ideas:
Method 1, establish a hash table, put the traversed nodes into the table. Output the first node that is repeatedly traversed. Space complexity O(n)
Method 2, fast and slow pointers , set up a fast pointer fast (move two at a time) Step), a slow pointer slow (moving one step at a time). If there is a ring, it will cross at one point. At this time, fast passes through a+n*(b+c)+b nodes, and slow passes through a+b nodes. Because of fast =2*slow simplifies to a=c+(n-1)(b+c). That is to say, at this time, a pos node is set up to start from the head node, and move backward step by step with slow, and the intersection is the first to enter the ring Nodes
Code
/**
* Definition for singly-linked list.
* class ListNode {
* int val;
* ListNode next;
* ListNode(int x) {
* val = x;
* next = null;
* }
* }
*/
public class Solution {
public ListNode detectCycle(ListNode head) {
if(head==null||head.next==null){
return null;
}
ListNode slow=head;
ListNode fast=head.next;
while(fast!=slow){
if(fast==null||fast.next==null){
return null;
}
fast=fast.next.next;
slow=slow.next;
}
ListNode pos=head;
while(pos!=slow.next){
pos=pos.next;
slow=slow.next;
}
return pos;
}
}