I learned to pack before. Learn how to package programs with resources today.
Previous tutorial link:
python is packaged into exe executable file. The tutorial
establishes a virtual environment to solve the problem of python packaging exe file is too large.
Today I will talk about how to pack the resource files together.
First, I will add the resource part of the code in the program, and write the absolute path of the resource. I found that the packaged exe can run, but when I moved the resource file, I found that the packaged exe could not run. This is definitely not working, which means that we cannot give the exe to other friends. Then I tried the new method.
Step 1: Establish a virtual environment and install related libraries.
This part refers to the
establishment of a virtual environment to solve the problem of python packaging exe file is too large.
Step 2: Create a resource folder
Basic principle: Pyinstaller can bundle resource files into an exe. When the exe is running, a temporary folder will be generated. The program can access the resources in the temporary folder through sys._MEIPASS
Official instructions: https://pythonhosted.org/PyInstaller/spec-files.html#spec-file-operation
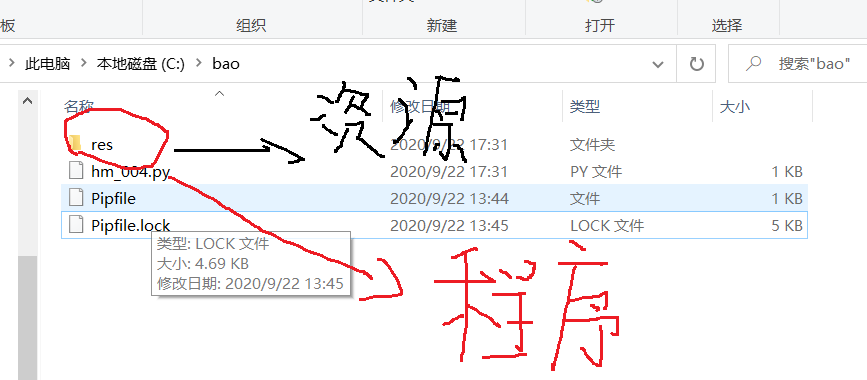
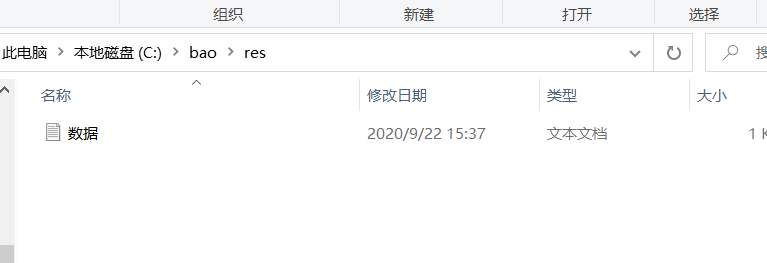
Step 3: Modify the .py file
Modify the code in the hm_004.py to read the resource data path part.
import sys
import os
#生成资源文件目录访问路径
def resource_path(relative_path):
if getattr(sys, 'frozen', False): #是否Bundle Resource
base_path = sys._MEIPASS
else:
base_path = os.path.abspath(".")
return os.path.join(base_path, relative_path)
#访问res文件夹下数据.txt的内容
filename = resource_path(os.path.join("res","数据.txt"))
The hm_004.py code is as follows. After
modifying the code, the hm_004.py code cannot run successfully on platforms such as pycharm, because the method of reading the path has changed. What does it matter? What we want is that the packaged exen can run.
#coding:utf-8
import sys
import os
#生成资源文件目录访问路径
def resource_path(relative_path):
if getattr(sys, 'frozen', False): #是否Bundle Resource
base_path = sys._MEIPASS
else:
base_path = os.path.abspath(".")
return os.path.join(base_path, relative_path)
#访问res文件夹下数据.txt的内容
filename = resource_path(os.path.join("res","数据.txt"))
print(filename)
with open(filename,encoding='utf-8') as f:
lines = f.readlines()
print(lines)
f.close()
Step 4: Package exe
result will generate build, dist folder and spec file.
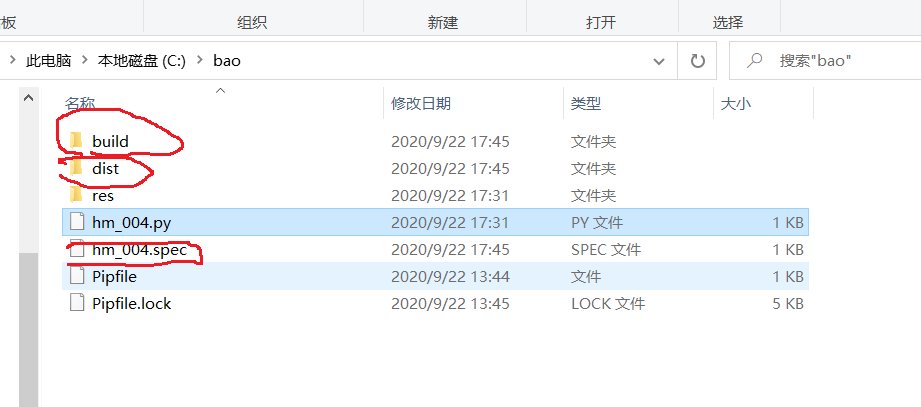
Step 5: Delete the build and dist folders. Modify the spec
spec and open it with Notepad. First open a notepad, and then drag the spec file into it.
Before the modification, datas=[], this article changes it to the following figure, which means
Add the res directory (and the files in the directory) in the current directory of hm_004.py to the target exe, and place it in the root directory of the zero-time file at runtime, with the name res.
If there are multiple resources
datas=[('res/bg.jpg','res'), ('exam.db','.')] There
are tuples in the list, the left side is the filename you want to add (relative path is OK), the right side It is the name of the folder after copying to the project.
For example: ('res/bg.jpg','res') in'res/bg.jpg' means that there is a bg.jpg picture in the res folder in the root directory of the project, which is copied to the res folder after the project.
'exam.db' in ('exam.db','.') is the file in the root directory of the project, and'.' means the root directory, that is, copied to the root directory in the project.
Still need to pay attention to the way to read the resource path, refer to the third step.
Step 6: Pack the exe again,
this time is to pack the spec file.
pyinstaller -F hm_004.spec
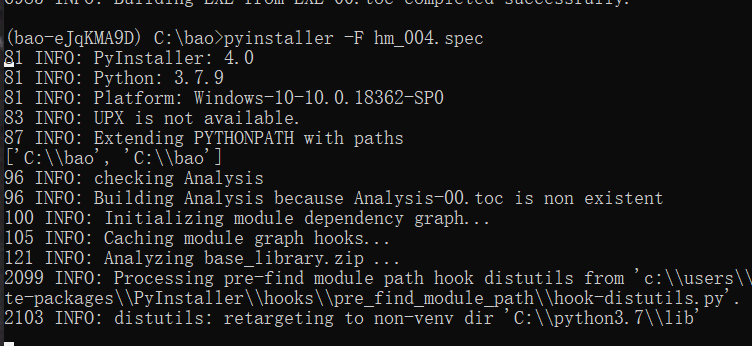
The build and dist files will be generated again. There is no new spec
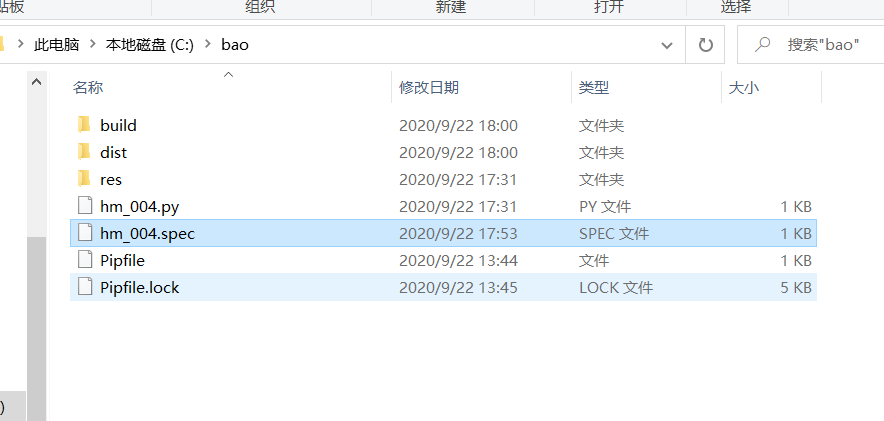
dist file
Step 7: Run exe
Since the example in this article is a simple program, not an interface, if you click exe directly, it will flash past because it is not an interface program, such as pyqt.
Non-interface, how do I need to run a python program, run
cmd into the dist folder, then enter the program name, and press Enter.
The result is as follows: It is found that the file path is different from the absolute path of the original data.txt.
Send it to other friends to try to run
I delete the res file.
And copy the dist folder to the desktop (if it is an interface program, you can only copy the exe)
the dist file on the desktop, (At this time, my computer does not have any data.txt resource file, because I have deleted it)
Run again:
other
In real life, our tasks are generally very complicated, such as involving models. Need to download tensorflow etc.
Some packages are too large to be installed directly in the virtual environment. You need to download them locally and install them.
Tutorial link: python install third-party packages
Which packaging steps are the same as the previous tutorial. The difference is that too many packages are needed, and the packages are not compatible with each other. For example, the keras library is required for packaging and tesorflow is required for Keras, but the tensorflow version must be greater than 2.0 during the packaging process.
The problem of compatibility between packages is exactly the drawback of python. Debug the package slowly when needed.
The new computer in electrical engineering: Yu Dengwu. Writing blog posts is not easy. If you think this article is useful to you, please give me a thumbs up and support, thank you.