One, Package
Package Version
------------ -------
click 7.1.2
Flask 1.1.2
Flask-MySQL 1.5.1
itsdangerous 1.1.0
Jinja2 2.11.2
MarkupSafe 1.1.1
pip 19.0.3
PyMySQL 0.9.3
setuptools 40.8.0
Werkzeug 1.0.1
2. Project structure
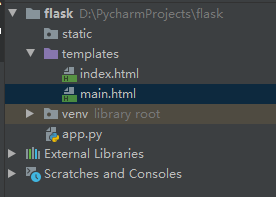
Three, app.py
from flask import Flask, json, render_template, request
from flaskext.mysql import MySQL
app = Flask(__name__)
app.secret_key = 'F12Zr47j\3yX R~X@H!jLwf/T'
@app.route('/')
def index():
return render_template("index.html")
@app.route('/login', methods=["post"])
def login():
form = request.form
conn = mysql.connect()
cursor = conn.cursor()
cursor.execute("SELECT * from users where username = %s and password = %s",
[form["username"], form["password"]])
user = cursor.fetchone()
if user is None:
return render_template('index.html', msg="用户名或者密码错误登录失败!")
else:
d = {
"id": user[0], "username": user[1], "password": user[2]}
session['user'] = user
return render_template('main.html', user=json.dumps(d))
if __name__ == '__main__':
app.run(port=5000, debug=True)
mysql = MySQL()
app.config['MYSQL_DATABASE_USER'] = 'root'
app.config['MYSQL_DATABASE_PASSWORD'] = '123456'
app.config['MYSQL_DATABASE_DB'] = 'demo'
mysql.init_app(app)
Fourth, the front end
1.index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>登录首页</title>
</head>
<body>
<form method="post" action="/login">
<input type="text" name="username" placeholder="请输入用户名:">
<input type="password" name="password" placeholder="请输入密码:">
<input type="submit" value="登录">
</form>
<h1>{
{
msg }}</h1>
</body>
</html>
2.main.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>后台主页</title>
</head>
<body>
<h1>登录成功,当前用户是{
{
user }}</h1>
</body>
</html>
Five, the database
DROP TABLE IF EXISTS `users`;
CREATE TABLE `users` (
`id` int(11) NOT NULL AUTO_INCREMENT COMMENT '主键',
`username` varchar(255) NOT NULL COMMENT '用户名',
`password` varchar(255) NOT NULL COMMENT '密码',
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=11 DEFAULT CHARSET=utf8;
LOCK TABLES `users` WRITE;
INSERT INTO `users` VALUES (1,'admin','123'),(3,'234','234'),(5,'456','123'),(6,'123','123'),(7,'789','789'),(10,'121','121');
UNLOCK TABLES;