1. What is the front-end background?
Wikipedia summary:
front-end and back-end are general terms that describe the start and end of a process. The front end acts to collect input information, and the back end processes it. The interface style and visual presentation of computer programs belong to the front end.
Second, how to achieve the front-end back-end to database interaction?
(1) Front-end static page-data submission and acquisition
There are generally two ways to submit front-end data to the server: 1. form submission; 2. AJAX submission
这是我的 directorys 文件夹下的 index.html 静态页面
// 1. 下面的form表单通过POST请求,提交一个基本信息到 /insert 路由中
<form action="/insert" method="POST">
<input type="text" name="name"><br>
<input type="text" name="age"><br>
<input type="radio" name="sex" value="男">男<br>
<input type="radio" name="sex" value="女">女<br>
<input type="submit" value="POST">
</form>
// 2. 通过一个button按钮发送一个AJAX的GET请求到/find 路由中
<button id="getdata">获取服务端数据</button>
<script>
let data;
document.getElementById("getdata").onclick = function(){
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function(){ //监听状态的变化的事件
if(xhr.readyState == 4 && xhr.status == 200){
data = JSON.parse(xhr.responseText)
console.log(data);
}
}
// xhr.open("get","/find?page=3&pageAmount=10", true);
xhr.open("get","/find", true);
xhr.send(null)
}
</script>
The difference between the two data submission / acquisition methods when the background server is turned on is:
form The information returned by the background received by the form will be displayed directly on the new page (refresh the page)
AJAX receives the background returned information through xhr.responseText
以下是以form表单发送一个POST请求的局部展示 (具体原理须结合后台理解)
以下是以Btn按钮 发送AJAX请求的局部展示 (具体原理须结合后台理解)
(2) Background Node.js-data reception and return
The way my Node server is built is through Express, which requires you to have a certain understanding of the Express module of Node.js.
这是 directorys文件夹同级目录下的 mongodb.js ,运行Node服务器即是运行该文件
// 调用./MongoModule 模块 ,该模块为自定义模块,其中封装着与数据库的具体操作
let db = require("./MongoModule");
// Express
let express = require("express");
let app = express();
// index.html 首页
app.use("/", express.static("./directorys"));
// 获取GET请求的search
let url = require("url");
/* 获取POST请求的search */
let bobyParser = require("body-parser");
app.use(bobyParser.urlencoded({extended: false}));
/*
* 以下调用insertOne、find等方法即是通过require("./MongoModule")模块封装的与数据库连接后的操作(下面会讲到)
*
* 通过app.post("/insert", (req, res) => { ... })路由即是获取前台的form表单提交的基本信息
* 这里的req.body中存储的即是前台发送信息,以对象存储
* eg: { name: 'codingScript', age: '18岁', sex: '男' }
* 通过app.get("/find", (req, res) => { ... }路由即是获取前端的AJAX请求信息
* ...... 删除、修改同理
*/
// 1. 插入
app.post("/insert", (req, res) => {
db.insertOne("home", "mine", req.body, (err, result) => res.send(result));
});
// 2. 查找
app.get("/find", (req, res) => {
let query = url.parse(req.url,true).query;
let pageAmount = parseInt(query.pageAmount) || 0;
let page = parseInt(query.pageAmount * (query.page-1)) || 0;
db.find({
"dbName": "home", // 数据库名
"collectionName": "mine", // 集合名
"pageAttr": {
"pageAmount":pageAmount, // 每页数据量
"page":page // 第page页
},
"json": {}, // 查询条件
"sort":{"num":1}, // 排序 1:升序 -1:降序
"callback": function(err, result) { // 查询结果
res.send(result); // 查询的内容返回到前台
}
});
});
// 3. 删除
app.get("/delete", (req, res) => {
let query = url.parse(req.url,true).query;
db.deleteMany("home", "mine", {"num":parseInt(query.num)}, (err, result) => res.send(result));
});
// 4. 修改
app.get("/update", (req, res) => {
db.updateMany("home", "mine", {"age":"23"},{$set:{"sex":"男"}}, (err, result) => res.send(result));
});
// 运行服务器 监听127.0.0.1:300端口 (端口号可以任意改)
app.listen(3000);
这是 directorys文件夹同级目录下的 MongoModule.js ,封装着与数据库的具体操作的模块
// 任何数据库的操作,都是先连接数据库!所以可以将连接数据库封装成函数
// 引入mongodb模块
let MongoClient = require('mongodb').MongoClient;
// 连接数据库 执行相应操作
function _connectDB(dbName, callback) {
let url = 'mongodb://localhost:27017/' + dbName;
MongoClient.connect(url, (err, db) => {
if(err) return "数据库连接失败";
console.log("Database Connect Success");
callback(err,db);
});
}
// 1. 插入数据
exports.insertOne = function(dbName, collectionName, json, callback) {
_connectDB(dbName, (err, db) => {
db.collection(collectionName).insertOne(json, (err, result) => {
if(err) return "插入失败";
callback(err, result);
db.close();
});
});
}
// 2. 删除数据
exports.deleteMany = function(dbName, collectionName, json, callback) {
_connectDB(dbName, (err, db) => {
db.collection(collectionName).deleteMany(json, (err, result) => {
if(err) return "插入失败";
callback(err, result);
db.close();
});
});
}
// 3. 修改数据
exports.updateMany = function(dbName, collectionName, jsonOld,jsonNew, callback) {
_connectDB(dbName, (err, db) => {
db.collection(collectionName).updateMany(jsonOld, jsonNew, (err, result) => {
if(err) return "插入失败";
callback(err, result);
db.close();
});
});
}
// 4. 查找数据
exports.find = function(option) {
_connectDB(option.dbName, (err, db) => {
db.collection(option.collectionName).find(option.json)
.limit(option.pageAttr.pageAmount) // 每页数据量
.skip(option.pageAttr.page) // 第page页
.sort(option.sort) // 排序
.toArray((err, result) => {
if(err) return "查询失败";
option.callback(err, result);
db.close();
});
});
}
(3)MongoDB
To send the data submitted by the foreground to the database and return the query data to the foreground, the server first needs to start the database:
mongod --dbpath The path where the data is stored (a folder for storing data)
MongoDB's commonly used SQL statements: ( Instruction on terminal operation)
use database mongo (restart a terminal) to
import data mongoimport
list all databases show dbs
use / create database use xxx
view the current database db
display the collection in the current database show collections
终端输出前台提交至数据库的数据
MongoDB图形化界面显示前台发送的数据
浏览器打印后台数据返回的数据
(The returned data is received through xhr.responseText in the foreground, and converted into an array / object using JOSN.paser ())
3. Illustration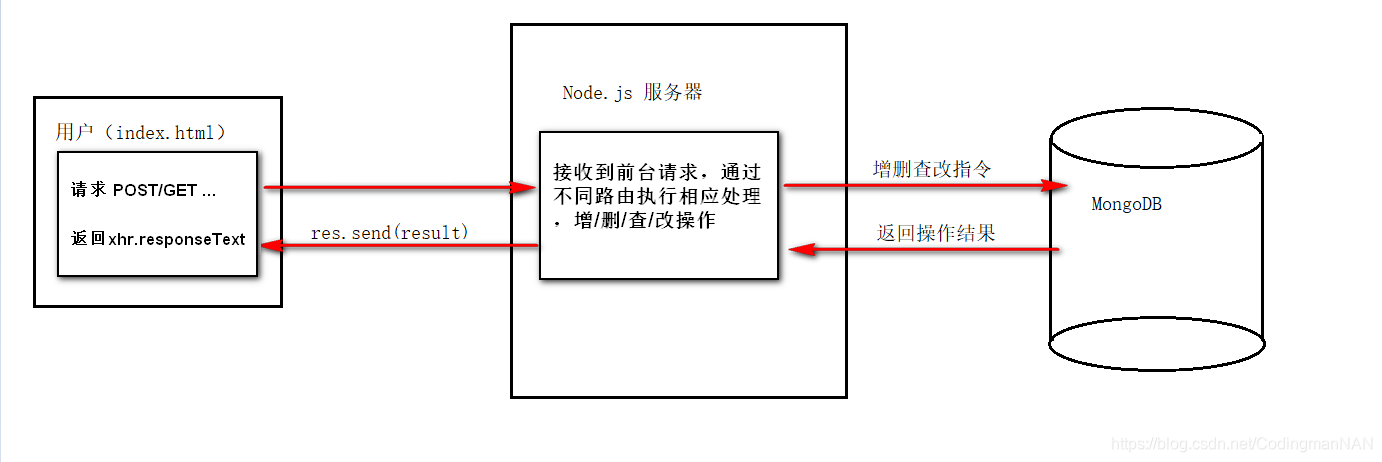
~~~~~~~~~~~~~ END ~~~~~~~~~~~~~~