This article is transferred from: http://m.biancheng.net/view/2811.html
In the previous section, we have understood the definition of members in the class. In this section, we will introduce how to access the members in the class, that is, the members of the calling class.
The members of the calling class actually use the objects of the class. For creating the objects of the class, you can first understand the class as a template, and the object of the class is an object customized according to this template.
For example, when creating a Word document, it will automatically create a file with the same style as the default document template (.dot) in Word.
The syntax for creating class objects is as follows.
Class name object name = new class name ();
The above grammatical form is a simple form, you can call the members of the class through the "object name". The syntax of the call is as follows.
Object name. Member of the class
The following example demonstrates how to use the object name to call members of the class.
[Example 1] Add a method in the Book class to output all the attributes in the class.
According to the title requirements, the code is as follows.
class Book { public int Id { get; set; } public string Name { get; set; } public double Price { get; set; } public void PrintMsg() { Console.WriteLine("图书编号:" + Id); Console.WriteLine("图书名称:" + Name); Console.WriteLine("图书价格:" + Price); } }
In the Main method in the Program.cs file of the project, add the code that calls the PrintMsg () method.
class Program { static void Main(string[] args) { Book book = new Book(); book.PrintMsg(); } }
Execute the above code, the effect is shown below.
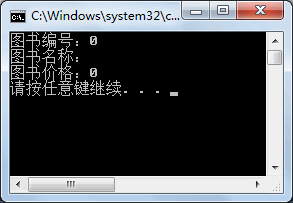
As can be seen from the above output effect, if no value is assigned to a custom attribute, the system will default to attribute assignment. If you need to do an output operation after assigning a value to an attribute, you must first assign a value to the attribute before calling PrintMsg (). The code for assigning and outputting is as follows.
class Program { static void Main (string [] args) { Book book = new Book (); // Assign property to book.Id = 1; book.Name = "Computer Basics"; book.Price = 34.5; book.PrintMsg (); } }
Execute the above code, the effect is shown in the figure below.
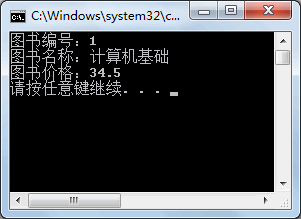
[Example 2] Add a method to the property assignment in the Book class and call it in the Main method.
According to the title requirements, the code is as follows.
class Book { public int Id { get; set; } public string Name { get; set; } public double Price { get; set; } public void SetBook(int id, string name, double price) { Id = id; Name = name; Price = price; } public void PrintMsg() { Console.WriteLine("图书编号:" + Id); Console.WriteLine("图书名称:" + Name); Console.WriteLine("图书价格:" + Price); } }
Call the SetBook and PrintMsg methods in the Main method, the code is as follows.
class Program { static void Main(string[] args) { Book book = new Book(); book.SetBook(1, "计算机基础", 34.5); book.PrintMsg(); } }
Execute the above code, the effect is consistent in [Example 1].
Through the above example, you can understand the basic usage of class object call attributes and methods. The code to assign values to attributes is as follows.
Object of class. Property name = value;
If you want to get the value of the property, just use "object of class. Property name". The code for calling a method using an object of a class is as follows.
Object of class. Method name (parameter);
Note: When calling a method, you need to pass the corresponding type of parameter.
If the members of the class are declared with the modifier static, then the "class name. Class member" method can be used directly when accessing the class members.
It should be noted that if a method is declared as static, only members of the static class can be accessed directly in the method, and non-static members can only be accessed through object calls of the class.
For example, if you change the method PrintMsg in [Example 2] to a static method, you cannot directly access the property, but you need to access the property through the class object or directly define the property as static.
[Example 3] Change the properties and methods in the Book class to static.
According to the requirements of the title, the properties and methods in the Book class are changed to static, and the changed code is as follows.
class Book { public static int Id { get; set; } public static string Name { get; set; } public static double Price { get; set; } public static void SetBook(int id, string name, double price) { Id = id; Name = name; Price = price; } public static void PrintMsg() { Console.WriteLine("图书编号:" + Id); Console.WriteLine("图书名称:" + Name); Console.WriteLine("图书价格:" + Price); } }
Change all the properties and methods in the Book class to static modifiers, and you can directly access the static properties in the static method.
The code to call the static method in the Main method is as follows.
class Program { static void Main (string [] args) { Book.SetBook (1, "Computer Basics", 34.5); Book.PrintMsg (); } }
Execute the above code, the effect is consistent with [Example 2]. It can be seen from the calling method that when calling the members of the Book class, there is no need to create objects of the class, but the static members in the class can be called directly by the class name. Usually the methods that are frequently called in the class are declared static.