This article is transferred from: http://m.biancheng.net/view/2809.html
Attributes are often used in conjunction with fields and provide get and set accessors, which are used to get or set the value of the field, respectively.
The use and methods of get accessor and set accessor are very similar, you can set or get the value of the field according to some rules and conditions when operating the field.
In addition, in order to ensure the security of the field, you can also choose to omit the get accessor or set accessor.
The syntax for defining attributes is as follows.
public data type attribute name
{
get
{
statement block to get attribute;
return value;
}
set
{
set attribute to get statement block;
}
}
among them:
1) get{}
The get accessor is used to get the value of an attribute. You need to use the return keyword at the end of the get statement to return a value compatible with the attribute data type.
If the accessor is omitted in the attribute definition, the private type field value cannot be obtained in other classes, so it is also called a write-only attribute.
2) set{}
The set accessor is used to set the value of the field. Here, a special value value is used, which is the value assigned to the field.
After the set accessor is omitted, you cannot assign values to fields in other classes, so it is also called a read-only attribute.
Usually the name of the attribute name is Pascal nomenclature. The first letter of the word is capitalized. If it is composed of multiple words, the first letter of each word is capitalized.
Since attributes are assigned to a field, the name of the attribute is usually the first letter of each word in the field. For example, a field named name is defined, and the attribute name is Name.
[Example] Define a book information class (Book), define the book number (id), book name (name), book price (price) 3 fields in the class, and set the attributes for these 3 fields, which will be the book The name is set to a read-only attribute.
According to the title requirements, the code is as follows.
namespace code_1 { class Book { private int id; private string name; private double price; // Set book number attribute public int Id { get { return id; } set { id = value; } } // Set book name attribute public string Name { get { return name; } } // Set book price attribute public double Price { get { return price; } set { price = value; } } } }
In the above example, when assigning a value to a field, the value is directly assigned to the field. If you want to limit the value assigned to the field, you can first determine whether the value meets the condition, if the condition is met, then assign the value, otherwise assign the default Value or perform other operations.
Assuming that the book price requirement in the above example is greater than 0, if the entered value does not satisfy the condition, the book price is set to 0. The attribute code of the revised book price field is as follows.
public double Price { get { return price; } set { if(value >= 0) { price = value; } else { price = 0; } } }
It can be seen from the above example that when defining field attributes, the role of the attribute is to provide get and set accessors for the field. Since the operations are similar, the definition of the attribute can be simplified to the following writing in C # language.
public data type attribute name {get; set;}
This method is also called automatic attribute setting. The code of attribute setting in the simplified book class is as follows.
public int Id{get; set;} public string Name{get; set;} public double Price{get; set;}
If you use the above method to set the property, you do not need to specify the field first. If you want to use automatic attribute to set the attribute means read-only attribute, you can directly omit the set accessor. The read-only attribute can be written as follows.
public int Id{get;}=1;
This is equivalent to setting the value of the Id attribute to 1 and ending with a semicolon. However, when using the method of automatically generating attributes, you cannot omit the get accessor. If you do not allow other classes to access the attribute value, you can add the access modifier private in front of the get accessor. The code is as follows.
public int Id{private get; set;}
In this way, the get accessor of the Id attribute can only be used in the current class.
In addition, the interface operation method provided by Visual Studio 2015 can also be used to generate properties of the field. Open the Book class and select the field to generate the property, then select "Edit"-"Refactor"-"Package field" in the menu bar. "Command, as shown below.
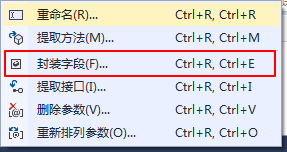
Select the "Package Field" command, and the dialog box shown below will pop up.
In this dialog box, if you need to make changes to the fields of the generated attributes, you can select or cancel through the check box, confirm the generated attributes, and click the "Apply" button to complete the operation of encapsulating the fields.
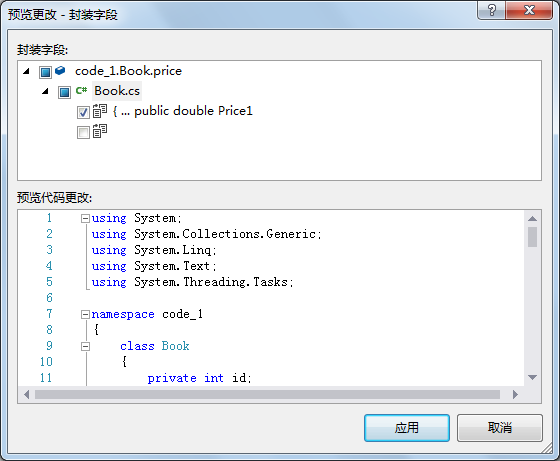
The user can also right-click the set field, and the menu shown below will pop up.
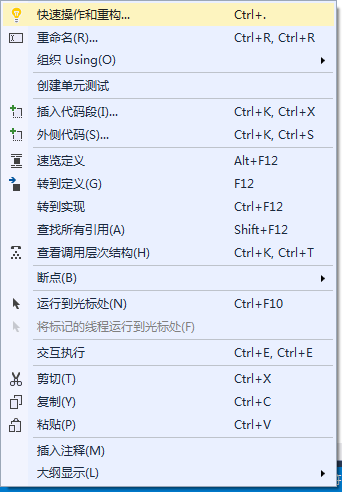
Select the "Quick Operation and Refactoring" command and then click the "Package Field" button to complete the field packing operation.