Flip the words in the string
Problem description:
Given a string, flip each word in the string one by one.
Example 1:
Input: "the sky is blue"
Output: "blue is sky the"
Example 2:
Input: "hello world!"
Output: "world! Hello"
Explanation: The input string can contain extra spaces before or after, but the reversed characters cannot be included.
Example 3:
Input: "a good example"
Output: "example good a"
Explanation: If there are extra spaces between two words, reduce the space between words after inversion to only one.
Description:
The characters without spaces form a word.
The input string can contain extra spaces before or after, but the reversed characters cannot be included.
If there are extra spaces between the two words, reduce the spaces between the words after inversion to only one.
Problem-solving ideas:
This question examines the basic operation of a string. There are two points to note: (1) there can be no spaces before and after the converted character; (2) multiple spaces in the middle should be compressed into one.
code show as below:
#include<iostream>
#include<vector>
using namespace std;
string reverseWords(string s) {
int i=0;
for(i=0;i<s.size();i++){//删除字符串前面的空格
if(s[i]!=' ')break;
}
s.erase(0,i);
for(i=s.size()-1;i>0;i--){//删除字符串后面的空格
if(s[i]!=' ')break;
}
s.erase(i+1,s.size()-i-1);
vector<string>v;
for(int i=0;i<s.size();i++){
string s1="";
while(i<s.size()){//遇到连续的字符保存在s1中
if(s[i]!=' '){
s1+=s[i];
i++;
}else{
break;
}
}
v.push_back(s1);//放进容器
int flag=0;
while(i<s.size()){
if(s[i]==' '){//出现空格则标志,然后跳过
flag=1;
i++;
}else{
break;
}
}
if(flag){//若出现空格则保存一个空格进容器
v.push_back(" ");
}
i--;
}
string s2="";
for(int i=v.size()-1;i>=0;i--){//将容器的字符串从后往前拿出
s2+=v[i];
}
return s2;
}
int main(){
string s=" the sky is blue ";
cout<<reverseWords(s)<<endl;
return 0;
}
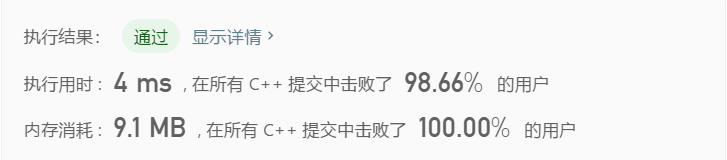