1. threads and processes
Relations and differences between processes and procedures
① program is an ordered set of instructions, it does not have any operational meaning, it is a static concept. The process is a process of program execution on the processor, it is a dynamic concept.
② software program can be used as a long-term data exist, but there is a certain process life cycle. Program is permanent, the process is temporary.
Note: The program can be seen as a recipe, but the process is the process in accordance with recipes for cooking.
③ the process and procedures of different: processes by a program, data, and process control block is composed of three parts.
④ correspondence between the process and procedures: by repeating, a program can have multiple processes; by calling relations, a process that may include more than one program.
2. The difference between the parallel and concurrent
- One explanation: refers to two or more parallel events occur at the same time; and refers to two or more concurrent events occur at the same time intervals.
- Explain two: Parallel multiple events on different entities, concurrent multiple events on the same entity.
- Explains three: on a parallel processor is "simultaneous" to handle multiple tasks, is concurrently handle multiple tasks simultaneously on multiple processors. As hadoop distributed cluster.
3. Create a way to thread
4. way to create a thread pool
ScheduledExecutorService scheduledThreadPool= Executors.newScheduledThreadPool(3); scheduledThreadPool.schedule(newRunnable(){ @Override public void run() { System.out.println ( "delayed three seconds" ); } }, 3, TimeUnit.SECONDS); scheduledThreadPool.scheduleAtFixedRate(newRunnable(){ @Override public void run() { System.out.println ( "delay one second every three seconds to perform a" ); } },1,3,TimeUnit.SECONDS);
5. Thread life cycle (state)
When the thread is created and started, it is neither a start to enter the execution state, nor is it has been in a state execution. In the thread of the life cycle, it has to go through New (New), Ready (Runnable), running (Running), blocked (Blocked) and death (Dead) 5 states. Especially when after the thread starts, it can not have been "occupied" the CPU to run alone, so the need to switch between multiple CPU threads, so thread state will run several times to switch between blocking
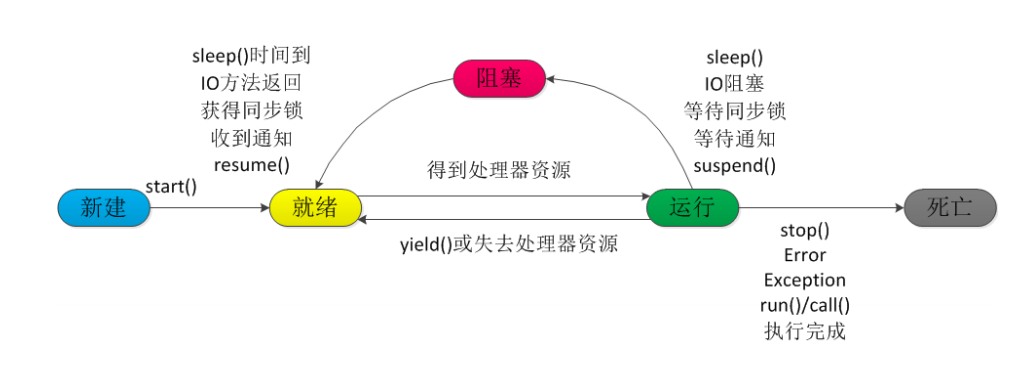
6.sleep and wait difference
7.start and run difference
The role of 8.volatile keyword (variable visibility, prohibit reordering)
9. The principle of first occurrence
1, the program sequence rules. In a thread EDITORIAL code book in advance occurred in the back. Rather it should be, according to the control flow of program order, because some branched structure.
2, Volatile variable rules. On a volatile variables, to write his first occurred in the read operation.
3, the thread start rule. start Thread object () method first occurrence in this thread every movement.
4, the thread terminates rules. All operations are ahead of thread termination occurs in the detection of this thread.
5, thread break rules. Call to thread interrupt () method to the first occurrence is detected interrupt thread code interrupt event.
6, the object termination rules. Initialize an object is completed (end of line constructor) begins issued finilize () method of the first occurrence.
7, transitive. A first occurrence of B, B C occurs first, then, C. A first occurs
8, the tube locking rules. The face of lock with a lock operation after a first unlock operation occurs.
10. The method calls between processes and threads
11.java common lock
12.synchronized principle underlying implementation
What 13.synchronized and ReentrantLock difference is?
- synchronized competition will always be waiting for a lock; ReentrantLock can try to acquire the lock and get get results
- synchronized acquiring the lock can not set time-out; ReentrantLock can set the timeout to acquire a lock
- synchronized fairness can not be achieved lock; ReentrantLock meet fair locks, which is to wait to get into the lock
- and control waits synchronized wakeup binding lock object wait () and notify (), notifyAll (); ReentrantLock and control waits for a wakeup Condition binding the await () and signal (), signalAll () method
- synchronized JVM level is achieved; ReentrantLock JDK is the code level to achieve
- executing the synchronized block of code in the lock or abnormal, automatically release the lock; of ReentrantLock not automatically release the lock, it is necessary finally {} block shows the release
14.ReentrantReadWriteLock read-write lock Detailed
Implementation 15.BlockingQueue blocking queue