Java, for a method of identifying error conditions and the consistency of response mechanisms - exception handling, exception handling can be effectively make the program more robust, easy to debug. This blog will detail the Java exception handling (Exception).
table of Contents:
☍ abnormal and abnormal Architecture Overview
☍ exception handling mechanism
☍ exception handling mechanism a: try-catch-finally
☍ exception handling mechanism two: throws an exception is thrown
User-defined exception classes ☍
In the process of using a computer language project development, even if the programmer to write code for perfection, still encounter some problems during the operation of the system, because there are many problems can not be avoided by the code, such as: customer input data format, read the file exists and that the network remains open and so on. Then you need to use Java exception handling mechanism to solve part of the problem, reasonable use exception handling mechanism can reduce the bug, increase the robustness of the program.
☍ abnormal and abnormal Architecture Overview
▴ abnormal Overview
☃ exception: In the Java language, will not normally occur in the implementation of the program called "anomaly." (Syntax errors and logic errors in the development process is not unusual)
☃ unusual event during the execution of Java programs that occur can be divided into two categories:
➢ Error: Serious problems can not be solved by the Java Virtual Machine. Such as: internal JVM system errors, resource depletion and other serious conditions. For example: a stack overflow error: StackOverflowError and heap overflow error: OOM (OutOfMemoryError). Generally do not write targeted exception handling code, modify code to cause errors.
public class ExceptionTest {
public static void main(String[] args) {
//栈(statck)溢出异常: java.lang.StackOverflowError
//main(args);
//堆(heap)溢出异常:java.lang.OutOfMemoryError
//Integer[] arr = new Integer[1020*1024*1024];
}
}
➢ Exception: other general problems caused by programming errors or accidental external factors, you can use the code targeted for processing. E.g:
✔ null pointer access
✔ trying to read a file that does not exist
✔ network connection is interrupted
✔ array subscript out of bounds
☃ for these errors, there are two solutions: First, it encounters an error terminate the program (default). Another method is by the programmer when writing programs to take into account the error
detection, an error message, and error handling (mainly to solve the unusual method).
☃ catch errors during compilation is the best, but some errors occur only at runtime, such as array bounds.
☃ different capture time can be divided into: 编译时异常
and 运行时异常
.
▴ Exception abnormal Category: abnormalities run & compile-time exception
Compile-time anomaly
☃ means the compiler requires that exceptions must be disposed of. That is the general program at run-time exception due to external factors. Abnormal compiler requires Java program must capture or declare all compilers.
☃ In Java, except RuntimeException Exception class and its subclasses are compiled in the abnormality, abnormality of the subject corresponding to the figure above. Compile-time unusual feature is the Java compiler will be checked, if unusual circumstances exist it is necessary to deal with exceptions, otherwise the program will not compile, such as abnormal reading empty files.
☃ for such an exception, if the program does not handle, it may bring unexpected results.
☃ exception processing compile time, there are two ways, as follows:
(1) using a try ... catch statement to capture the abnormal processing.
(2) using the throws keyword to declare an exception is thrown, the caller waits for its handling, processing continues if the caller does not throw an exception.
Runtime exception
☃ means that the compiler does not require unusual compulsory disposal. Generally it refers to a logical error when programming, programmers should actively avoid anomalies arise. Abnormal java.lang.RuntimeException class and its subclasses are running.
☃ runtime exception is characterized by a Java compiler will not be checked, that is, when an exception occurs when such a program, even without the use try ... catch statement to capture or throws keyword to declare thrown, the program can compile (run-time error may be), such as an array subscript bounds exception.
☃ for such exceptions, as the case may selection process (not sure logic is correct) or no treatment (when determining the correct logic), because such anomalies are common, if the whole process might be readability and efficiency of the program to produce influences.
☍ common abnormalities
▴ common abnormal run: java.lang.RuntimeException its subclasses
Null pointer exception NullPointerException
public void NullPointerExceptionDemo() {
Object obj = null;
System.out.println(obj.toString());
}

Array subscript bounds exception: ArrayIndexOutOfBoundsException
public void ArrayIndexOutOfBoundException1() {
int[] arr = new int[3];
System.out.println(arr[5]);
}
public void ArrayIndexOutOfBoundException2() {
String string = "abcd";
System.out.println(string.toCharArray()[5]);
}
String subscript bounds exception: StringIndexOutOfBoundsException
public void StringIndexOutOfBoundExceptionDemo() {
String str = "abcd";
System.out.println(str.charAt(5));
}
Type cast exception: java.lang.ClassCastException
public void ClassCastExceptionDemo() {
class Dog{
}
class Cat{
}
//Dog dog = new Cat(); 编译不通过,语法错误
Object obj = new Dog();
Cat cat = (Cat)obj;
}
Abnormal digital format: java.lang.NumberFormatException
public void NumberFormatExceptionDemo(){
String str = "abc";
//Integer number = new Integer(str);
int num = Integer.parseInt(str);
}
Console input format anomaly: java.util.InputMismatchExceptionDemo
public void InputMismatchExceptionDemo() {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入数字:");
int num = scanner.nextInt();
//控制台输入不符合规则时报的异常:如此时输入非数字字符
System.out.println(num);
}
Arithmetic anomaly: java.lang.ArithmeticException
public void ArithmeticExceptionDemo() {
int a = 4;
int b = 0;
System.out.println(a / b);
}
When ▴ common compiler exception: exception classes in addition to other outside RunTimeException
Exception to remind compiled ☃ compile time, it requires the programmer to handle the exception at compile time
☃ common compile-time anomalies are:
◌ SQL anomalies: java.sql.SQLException (an exception database access error or other error messages about)
Flow ◌ IO Exception: java.io.IOExeption (. When a certain I / O exception occurs, is thrown such failure or interruption of I / O operations generated generic class exception.)
• java.io.FileNotFoundException (when trying to open the file specified pathname failed Thrown)
• java.io.EOFException (represented in the input process unexpectedly reaches the end or the end of the file stream. This anomaly consists of data input streams to indicate the end of the stream. Please note that many other input operations return a special value in the end of the stream, instead of throwing an exception.)
◌ not find the specified class anomaly: java.lang.ClassNotFoundException
◌ data format anomalies: java.util.zip.DataFormatException (when the data format error This exception is thrown)
◌ not find method exception: NoSuchMethodException (when a particular method can not be found, Thrown)
◌ interrupt exception: java.lang.InterruptedException (thread is waiting, sleeping, or otherwise occupied when thrown, thread break between activities occasionally, a method may want to test whether the current thread has been interrupted, and if so, immediately thrown. the exception)
☍ exception handling mechanism
When programming, often coupled with the local code detection errors may occur,
such as performed when x / y operation, the denominator is 0 to be detected, the data is empty, the input data but not the characters and the like. Excessive if-else branch code of the program will lead to longer, bloated, poor readability. Thus using the exception handling mechanism.
☃ Java exception handling mechanism used, is the program code exception handling together, separate from the normal program code, makes the program simple, elegant, and easy to maintain.
☃ Java exception handling mode is divided into: try-catch-finally throws + mode and the abnormal mode type.
☃ Java provides is caught throwing exception handling model.
Execution ☃Java program such as abnormal, generates an exception class object, the exception object will be submitted to the Java runtime system, a process known as throw
(throw) exception.
☃ Once an exception is thrown when an object program, {} in the subsequent code are not executed.
Exception object generated:
☃ generated automatically by a virtual machine: the program is running, the program issues virtual machine detects the occurrence, if not found in the current handler code, automatically creates a corresponding instance of the object class exception is thrown in the background and - - automatic throw
☃ created manually by developers, such as: Exception exception = new ClassCastException (); create good does not throw an exception object does not have any influence on the program, and create a common objects, like
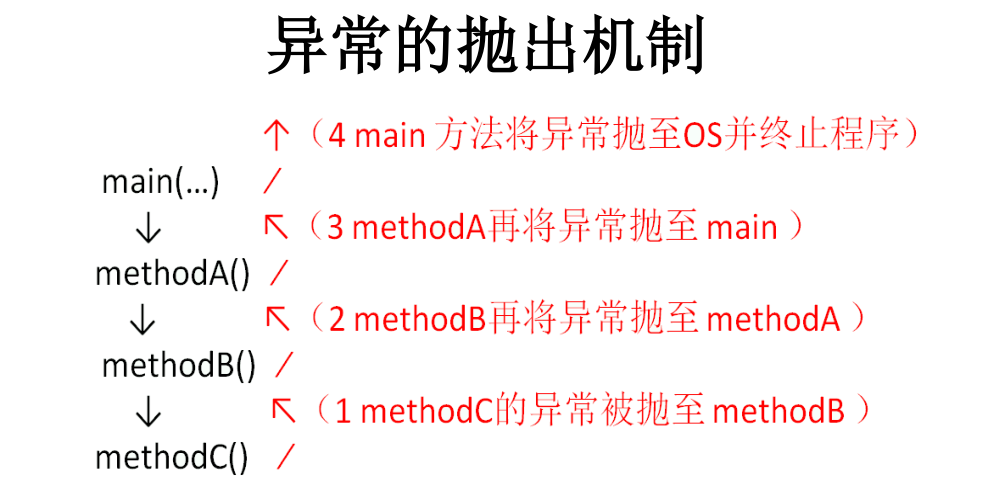
☃ if a method throws an exception, the exception object is thrown to the caller method of treatment. If the exception is not handled in the caller method, it continues to be thrown to the top of the method call this method. This process will continue indefinitely, until the exception is handled. This process is called capture (catch) exceptions.
☃ If an exception back to the main () method, and the main () does not handle, the program run is terminated.
☃ programmers usually only handle Exception, while Error powerless.
✦ To ensure the normal execution of the code must deal with exceptions that may arise during code execution can be an exception thrown to the caller, but exceptions must be handled at a time, rather than been thrown up without treatment .
☍ exception handling mechanism a: try-catch-finally
try-catch:
☃ exception handling is achieved through the try-catch-finally statement.
try {
//可能产生异常的代码
}
catch (异常类型1 变量名1) {
// 当产生指定类型(包括子类)的异常时的处理措施
}
catch (异常类型2 变量名2) {
// 当产生指定类型(包括子类)的异常时的处理措施
}
...
finally { //finally根据情况可加可不加
//无论是否发生异常,都会执行的语句
}
//其他代码
The first step is to catch exceptions ☃ {...} block with a selected range of the caught exception try, the abnormal code is possible in the try block. Once an exception occurs, the object will generate a respective exception classes, depending on the type of this object, to match the catch.
☃ the catch (Exceptiontype e) is a statement block exception object code processing. Each try block may be accompanied by one or more catch statement for exception handling of different types of objects may be generated.
Once ☃ abnormality try to match one catch, the catch enters the abnormality processing, the processing is completed once out of the current try-catch structure, the code continues down thereafter.
If ↪ know exactly what kind of exception is generated, the exception type can be used as a parameter of the catch; parent class can also be used as a parameter of the catch.
For example: where ArithmeticException class can be used as a parameter, it can be used as a parameter RuntimeException class or parent classes in all exception Exception class as an argument. But it can not be unrelated to the ArithmeticException class exceptions, such as NullPointerException (catch statements will not line).
☃ catch the exception type if there is no parent child relationship, the statement did not affect the order, if you catch the exception type if the child parent relationship is satisfied, a subclass of certain statements in the above require the parent class, otherwise an error.
Capture information about the anomalies:
☃ As with other objects, you can access an exception object's member variables or call its
methods.
➣ getMessage () Gets the abnormality information, return the string
➣ printStackTrace () Gets exception classes and exception information, as well as locations of the anomalies in the program. Return value void.

public static void tryCatchFinally() {
try {
//可能产生异常的代码
int arr[] = new int[3];
System.out.println("开始执行");
arr[4] = 232;
//出现异常后,{}其后的代码不再执行
System.out.println(arr[4]);
}catch (NumberFormatException e) {
//数字格式异常
System.out.println("数字格式异常");
}
/*子类异常一定声明在父类的上面
catch (IndexOutOfBoundsException e) {
}*/
catch (ArrayIndexOutOfBoundsException e) {
// 当产生指定类型(包括子类)的异常时的处理措施
System.out.println("数组越界异常");
//异常对象中的getMessage()方法,返回字符串类型的异常信息
System.out.println("getMessage方法:" + e.getMessage());
//异常对象中的printStackTrace()方法
e.printStackTrace(); //控制台输出详细的异常信息
} catch (Exception e) {
System.out.println("出现异常");
}finally {
//无论是否发生异常,都会执行的语句
System.out.println("ArrayIndexOutOfBoundsException Test");
}
//其他代码
//try-catch中定义的变量出了try-catch范围就不能使用了,除非将变量定义在try-catch前面
//arr[1] = 10;
System.out.println("orther code");
}
Output:

✦ use try-catch-finally compile exception handling, so that the program is no longer being given at compile time, but may still be an error at run time using the try-catch-finally exception may occur will be equivalent to a compile-time, delayed until run time appear.
finally:
☃ finally catch statement and the statement is optional, but at least one must be present.
The final step ☃ catch exceptions is to provide a unified statement by finally exception handling exports, so that the control flow goes to other parts of the program before, be able to
make a unified management of the state of the program.
☃ whether or not the abnormal event occurs in the try block, the catch statements whether, if there is an exception catch statement, whether there is return catch statement, finally statement in the block will be executed.
Since ☃ will finally performed, so if the method returns a value, finally also defined if the return value, the return value try-catch is overwritten
@SuppressWarnings("finally")
public int test1() {
try {
int a = 23;
int b = 0;
System.out.println(a / b);
return 0;
} catch (ArithmeticException e) {
e.printStackTrace();
return -1;
} catch (Exception e) {
e.printStackTrace();
return -1;
} finally {
System.out.println("执行到最后了");
//返回值会覆盖try-catch中的返回值
return 1;
}
}
Output:

☃ Because JVM can not automatically recover the resource database similar link, input and output streams, such as Socket network programming, we need to free up resources to manually shut down, then finally define the most appropriate (to prevent the influence due to abnormal cause code is not executed)
public void test2() {
//定义在try-catch前面,方便try-catch后面的使用
FileInputStream fis = null;
try {
File file = new File("abc.txt");
fis = new FileInputStream(file);
int data = fis.read();
while(data != -1) {
System.out.print((char)data);
data = fis.read();
}
} catch (FileNotFoundException e) {
e.printStackTrace();
}catch (IOException e) {
e.printStackTrace();
}finally {
//关闭流,try-catch可以嵌套使用
try {
if(fis != null) //避免空指针
fis.close();
} catch (IOException e2) {
e2.printStackTrace();
}
}
System.out.println("end");
}
Catch exceptions vs not catch the exception
☃ RuntimeException class or its subclasses, unusual these special classes
point is: even without the use try and catch capture, Java I could be captured and compiled by (a runtime exception will occur but makes the program run is terminated).
☃ If an exception is an exception, you must capture IOException and other types of non-operation, otherwise compilation errors. In other words, abnormal, abnormal capture when we have to deal compiled into a runtime exception.
☍ exception handling mechanism two: throws an exception is thrown
Statement thrown
☃ statement throws an exception is a Java exception handling in the second way
☄ (when the statement is executed) if a method could generate some kind of anomaly, but are not sure how to handle this
kind of anomalies, this method should explicitly declare an exception is thrown, indicating that the method will not deal with these anomalies, but by the caller of the method to deal with.
☄ throws in the methods of declaration statement that throw exception list, the exception type back throws exception type can be produced in the process, may be its parent.
When ☃ throws an exception is thrown way too early to write in a method declaration, indicate the implementation of this method, an exception once the method body type runtime exception may be thrown, and will generate an exception object in the code of the code and the method body does not continue down, this object is satisfied throws exception type is thrown, in vivo methods, the latter exception code is not executed
☃ if a method throws an exception, the exception object is thrown to the caller method of treatment. If the exception is not handled in the caller method, it continues to be thrown to the top of the method call this method. This process will continue indefinitely, until the final exception is handled or thrown JVM virtual machine. If an exception back to the main () method, and the main () does not handle, the program run is terminated.
↪ try-catch-finally: being really handle an exception; throws exception: only exception thrown the caller does not really handle the exception
public class ThrowsException {
static FileInputStream fis = null;
public static void main(String[] args) {
ThrowsException t = new ThrowsException();
//调用者处理异常
try {
t.getReadFile();;
} catch (IOException e) {
System.out.println("出现异常");
e.printStackTrace();
}finally {
//关闭io流
try {
if(fis != null)
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
System.out.println("finally");
}
}
//调用者继续抛出异常,IOException是FileFoundException父类,若对于两个异常不做区分处理,则抛出父类异常即可
public void getReadFile() throws IOException {
String filePath = "abc.txt";
readFile(filePath);
}
//声明抛出异常
public void readFile(String filePath) throws FileNotFoundException,IOException{
File file = new File(filePath);
// 读文件的操作可能产生FileNotFoundException 类型的异常
fis = new FileInputStream(file);
int data = fis.read();
while(data != -1) {
System.out.print((char)data);
data = fis.read();
}
System.out.println("方法体末尾");
}
}
Output:
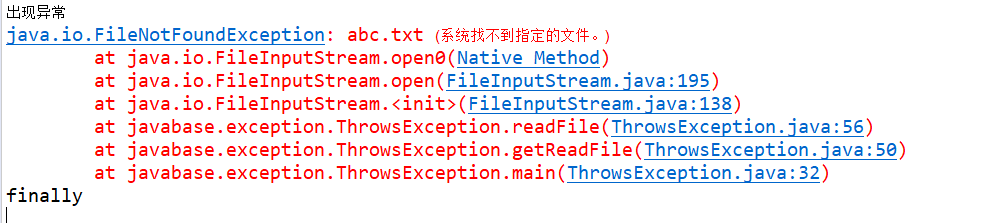
Thrown process
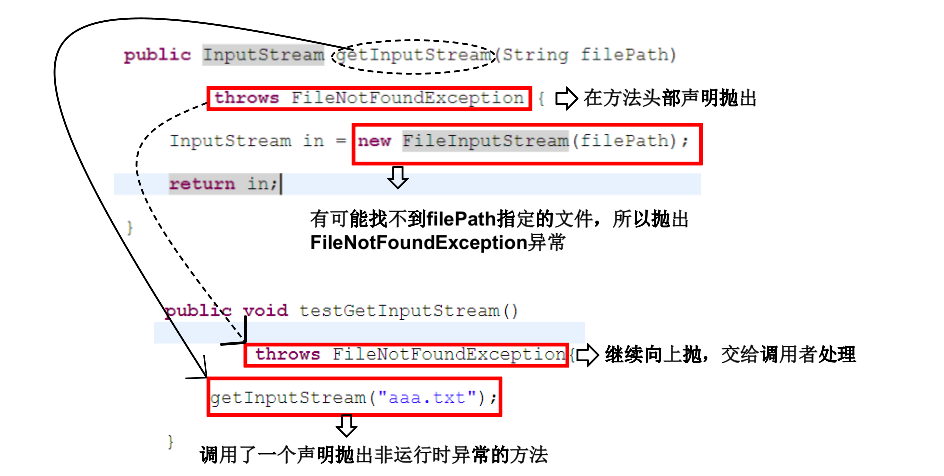
Principles override methods that throw the exception
☃ overridden method can not throw more exceptions than the type of method is overridden range. In the case of multi-state, a call to methodA () method - for catching an exception by exception handling parent class declaration.
☃ If the method is overridden parent class throws no way to handle exceptions, the subclass can not use the overridden method throws an exception is thrown, this time means that a subclass method if there is an abnormality rewriting, you must use the try- processing catch-finally embodiment.
public class A {
public void methodA() throws IOException {……}
public void methodB() {……}
}
public class B1 extends A {
public void methodA() throws FileNotFoundException {……}
//public void methodB() throws FileNotFoundException {……} 报错
}
public class B2 extends A {
public void methodA() throws Exception { // 报错……}
public void methodB(){
try{...}
catch(ExcptionType e){...}
finally{...}
}
}
Select the way of exception handling
A method of execution, and calls has several other methods, these methods in turn progressive relationship (eg: Method A calls Method B, B needs to obtain the data in Method C, Method C Method D to give the required data), these methods are recommended to be used with the called throws an exception is thrown while executing consider using method a try-catch-finally handled, it can guarantee when performing the method a, the other method is called If the abnormality is not disposed to affect the occurrence of a, while facilitating centralized exception processing.
try-catch-finally exception handling method and throws an exception handling only choose one to use, can not be used, because at the same time use, because the try-catch-finally has exception handling, and then thrown no sense.
☍ manually throw an exception
Java exception class object in addition to automatically generated when an abnormality occurs during program execution by the system and throw (the above are generated automatically JVM) can also be thrown manually create and make necessary.
☃ first class generates an exception object and then thrown operation (submitted to the Java runtime achieved by the throw statement
line environment).
public class ThrowTest {
public static void main(String[] args) {
ThrowTest t = new ThrowTest();
try {
t.method(-2);
} catch (Exception e) {
System.out.println(e.getMessage());
}
}
public void method(int num) throws Exception{
int i = 0;
if(num >= 0) {
i = num;
}else {
throw new Exception("数字不能小于0");
}
}
}
User-defined exception classes ☍
☃ Generally, user-defined class is a subclass of RuntimeException
☃ custom exception class usually need to write several overloaded constructors
☃ custom exception desirable to provide a serial number serialVersionUID
☃ defined custom exception thrown by throw
☃ different custom naming must be standardized, meaning able to see the name to know when an exception occurs, you can determine the type of exception by name
☃ your own exception classes must inherit the existing exception classes, usuallyException & RuntimeException
public class MyExceptionTest {
public void regist(int num) throws MyException {
if (num < 0)
throw new MyException("人数为负值,不合理", 3);
else
System.out.println("登记人数" + num);
}
public void manager() {
try {
regist(-1);
} catch (MyException e) {
System.out.println("登记失败," + e.getMessage());
System.out.print("出错种类" + e.getId());
}
System.out.print("本次登记操作结束");
}
public static void main(String args[]) {
MyExceptionTest t = new MyExceptionTest();
t.manager();
}
}
class MyException extends RuntimeException {
// 自定义异常序列标识号,用于区分异常
static final long serialVersionUID = 14232463463463535L;
private int idnumber;
public MyException() {}
public MyException(String message) {
super(message);
}
public MyException(String message, int id) {
super(message);
this.idnumber = id;
}
public int getId() {
return idnumber;
}
}
Output:

☍ summary
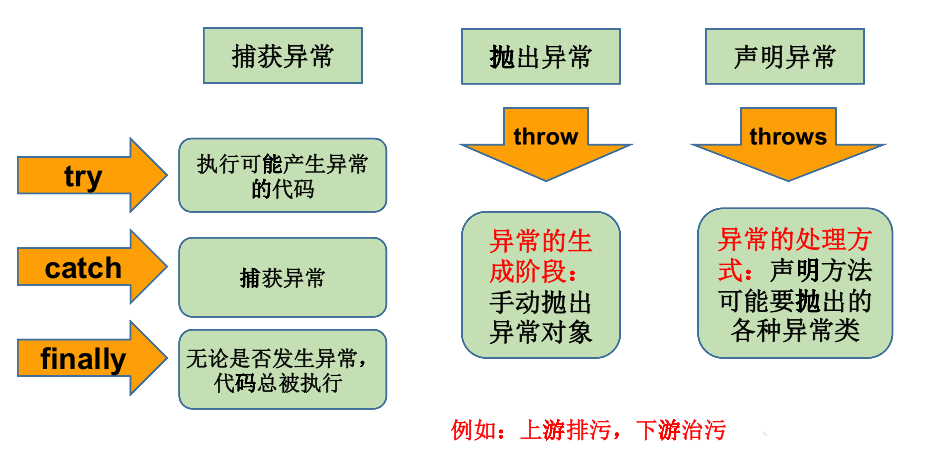
This blog and CSDN blog (ཌ ་. Jun ☠ fiber ་. ད) simultaneous release