1.使用ConversionService
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xmlns:p="http://www.springframework.org/schema/p"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc.xsd">
<!-- 配置处理器Handle,映射为“/hello”的请求 -->
<!-- <bean name="/hello" class="com.springmvc.controller.HelloController" /> -->
<mvc:annotation-driven conversion-service="conversionService"/>
<bean id="conversionService" class="org.springframework.context.support.ConversionServiceFactoryBean">
<property name="converters">
<list>
<bean class="com.springmvc.converter.StringToDateConverter" p:datePattern="yyyy-MM-dd"></bean>
</list>
</property>
</bean>
<!-- 配置自动扫描的基包 -->
<context:component-scan base-package="com.springmvc"/>
<!-- 配置视图解析器,将控制器方法返回的逻辑视图解析为物理视图 -->
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver" >
<!-- <property name="prefix" value="/ch06/"></property> -->
<!-- <property name="prefix" value="/ch07/" /> -->
<property name="prefix" value="/ch09/" />
<property name="suffix" value=".jsp"></property>
</bean>
<!-- 直接页面转发 -->
<mvc:view-controller path="success" view-name="success"/>
<mvc:view-controller path="index" view-name="index"/>
<bean class="org.springframework.web.servlet.view.BeanNameViewResolver">
<property name="order" value="50"></property>
</bean>
<mvc:default-servlet-handler/>
</beans>
package com.springmvc.converter;
import java.text.SimpleDateFormat;
import java.util.Date;
import org.springframework.core.convert.converter.Converter;
public class StringToDateConverter implements Converter<String, Date> {
private String datePattern;
public void setDatePattern(String datePattern) {
this.datePattern = datePattern;
}
@Override
public Date convert(String date) {
try {
SimpleDateFormat dateFormat=new SimpleDateFormat(datePattern);
return dateFormat.parse(date); //将字符串性转换成Date类型
} catch (Exception e) {
e.printStackTrace();
System.out.println("日期转换失败");
return null;
}
}
}
2.使用@InitBinder注解 和 WebDataBinder 类
@InitBinder
public void initBinder(WebDataBinder binder) {
SimpleDateFormat dateFormat=new SimpleDateFormat("yyyy-MM-dd");
binder.registerCustomEditor(Date.class, new CustomDateEditor(dateFormat, true));
}
3.使用@DateTimeFormat注解
import org.springframework.format.annotation.DateTimeFormat;
public class User {
@DateTimeFormat(pattern="yyyy-MM-dd")
private Date birthday;
...
附:数据类型转换图
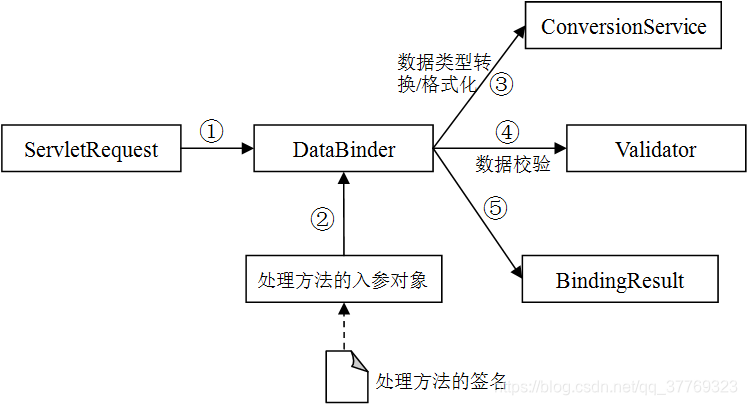