xml方式配置spring mvc
- 编写web.xml;注册listener、注册servlet、注册servlet-mapping
- 编写spring-mvc.xml
- 执行servlet规范:
- 在"根目录/resources/META-INF/services/"添加配置文件javax.servlet.ServletContainerInitializer
- 自定义一个类实现spring提供的WebApplicationInitializer接口,实例化&初始化spring容器
- 自定义controller类和业务逻辑
- 打包部署到web容器(通常使用tomcat)
零xml配置 & 内置tomcat 启动spring mvc
build.gradle添加依赖
compile group: 'org.springframework', name: 'spring-webmvc', version: '5.1.12.RELEASE'
compile group: 'org.apache.tomcat.embed', name: 'tomcat-embed-core', version: '8.5.31'
compile group: 'com.alibaba', name: 'fastjson', version: '1.2.47'
resources/META-INF/services/javax.servlet.ServletContainerInitializer
org.springframework.web.SpringServletContainerInitializer
UserEntity
public class UserEntity {
private String name;
private String age;
...
}
AppConfig 代替spring-mvc.xml
@Configuration
@ComponentScan("com.tbryant.springmvctest")
@EnableWebMvc
public class AppConfig implements WebMvcConfigurer {
@Override
public void configureViewResolvers(ViewResolverRegistry registry) {
}
@Override
public void configureMessageConverters(List<HttpMessageConverter<?>> converters) {
FastJsonHttpMessageConverter fastJsonHttpMessageConverter=new FastJsonHttpMessageConverter();
converters.add(fastJsonHttpMessageConverter);
}
}
MyWebApplicationInitializer 注册servlet和注册servlet-mapping
public class MyWebApplicationInitializer implements WebApplicationInitializer {
@Override
public void onStartup(ServletContext servletCxt) {
AnnotationConfigWebApplicationContext ac = new AnnotationConfigWebApplicationContext();
ac.register(AppConfig.class);
DispatcherServlet servlet = new DispatcherServlet(ac);
ServletRegistration.Dynamic registration = servletCxt.addServlet("DispatcherServlet", servlet);
registration.setLoadOnStartup(1);
registration.addMapping("/");
}
}
Application 内置tomcat & 注册listener
public class Application {
public static void main(String[] args) throws Exception {
Tomcat tomcat=new Tomcat();
tomcat.setPort(8080);
Context context=tomcat.addContext("/",System.getProperty("java.io.tmpdir"));
context.addLifecycleListener((LifecycleListener)Class.forName(tomcat.getHost().getConfigClass()).newInstance());
tomcat.start();
tomcat.getServer().await();
}
}
UserController
@Controller
public class UserController {
@RequestMapping("/getuser")
@ResponseBody
public UserEntity getUser() {
return new UserEntity("TBryant", "18");
}
}
运行main函数 执行结果
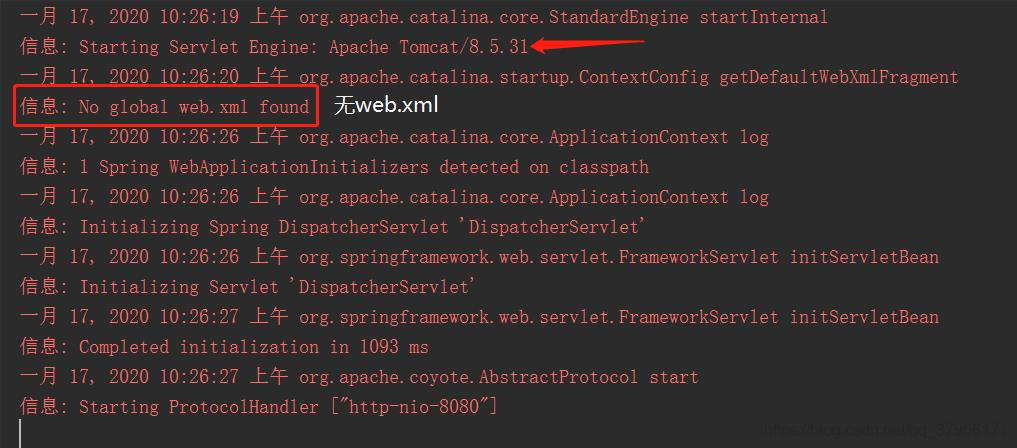