1.ThreadPoolExecutor创建线程池的构造函数
public ThreadPoolExecutor(int corePoolSize, int maximumPoolSize, long keepAliveTime, TimeUnit unit, BlockingQueue<Runnable> workQueue, ThreadFactory threadFactory, RejectedExecutionHandler handler) { if (corePoolSize < 0 || maximumPoolSize <= 0 || maximumPoolSize < corePoolSize || keepAliveTime < 0) throw new IllegalArgumentException(); if (workQueue == null || threadFactory == null || handler == null) throw new NullPointerException(); this.acc = System.getSecurityManager() == null ? null : AccessController.getContext(); this.corePoolSize = corePoolSize; this.maximumPoolSize = maximumPoolSize; this.workQueue = workQueue; this.keepAliveTime = unit.toNanos(keepAliveTime); this.threadFactory = threadFactory; this.handler = handler; }
参数简介:
- corePoolSize:核心线程数量
- maximumPoolSize:最大线程数量
- keepAliveTime:没有执行任务的线程,最大空闲时间
- unit:时间单位,TimeUnit.DAYS,TimeUnit.HOURS,TimeUnit.MINUTES,TimeUnit.SECONDS,TimeUnit.MILLISECONDS
TimeUnit.MICROSECONDS,TimeUnit.NANOSECONDS,七种
- BlockingQueue<Runnable> workQueue:一个阻塞队列,用来存储等待执行的任务, ArrayBlockingQueue,LinkedBlockingQueue,SynchronousQueue三种 - ThreadFactory threadFactory:创建线程的工厂类 - RejectedExecutionHandler handler:拒绝策略
2.拒绝策略 拒绝策略: 四种拒绝策略,实现:RejectedExecutionHandler接口 1.AbortPolicy(中止策略,抛出异常,默认的策略)
源码: /** * A handler for rejected tasks that throws a * {@code RejectedExecutionException}. / public static class AbortPolicy implements RejectedExecutionHandler { /* * Creates an {@code AbortPolicy}. */ public AbortPolicy() { }
/**
* Always throws RejectedExecutionException.
*
* [@param](https://my.oschina.net/u/2303379) r the runnable task requested to be executed * [@param](https://my.oschina.net/u/2303379) e the executor attempting to execute this task * [@throws](https://my.oschina.net/throws) RejectedExecutionException always */ public void rejectedExecution(Runnable r, ThreadPoolExecutor e) { throw new RejectedExecutionException("Task " + r.toString() + " rejected from " + e.toString()); } }
2.CallerRunsPolicy(由调用线程处理该任务)
源码: /** * A handler for rejected tasks that runs the rejected task * directly in the calling thread of the {@code execute} method, * unless the executor has been shut down, in which case the task * is discarded. / public static class CallerRunsPolicy implements RejectedExecutionHandler { /* * Creates a {@code CallerRunsPolicy}. */ public CallerRunsPolicy() { }
/**
* Executes task r in the caller's thread, unless the executor
* has been shut down, in which case the task is discarded.
*
* @param r the runnable task requested to be executed
* @param e the executor attempting to execute this task
*/
public void rejectedExecution(Runnable r, ThreadPoolExecutor e) { if (!e.isShutdown()) { r.run(); } } }
3.DiscardOldestPolicy(丢弃队列最前面的任务,然后重新提交被拒绝的任务)
源码: /** * A handler for rejected tasks that discards the oldest unhandled * request and then retries {@code execute}, unless the executor * is shut down, in which case the task is discarded. / public static class DiscardOldestPolicy implements RejectedExecutionHandler { /* * Creates a {@code DiscardOldestPolicy} for the given executor. */ public DiscardOldestPolicy() { }
/**
* Obtains and ignores the next task that the executor
* would otherwise execute, if one is immediately available,
* and then retries execution of task r, unless the executor
* is shut down, in which case task r is instead discarded.
*
* @param r the runnable task requested to be executed
* @param e the executor attempting to execute this task
*/
public void rejectedExecution(Runnable r, ThreadPoolExecutor e) {
if (!e.isShutdown()) { e.getQueue().poll(); e.execute(r); } } }
4.DiscardPolicy(丢弃任务,但是不抛出异常。如果线程队列已满,则后续提交的任务都会被丢弃,且是静默丢弃)
源码: /** * A handler for rejected tasks that silently discards the * rejected task. / public static class DiscardPolicy implements RejectedExecutionHandler { /* * Creates a {@code DiscardPolicy}. */ public DiscardPolicy() { }
/**
* Does nothing, which has the effect of discarding task r.
*
* @param r the runnable task requested to be executed
* @param e the executor attempting to execute this task
*/
public void rejectedExecution(Runnable r, ThreadPoolExecutor e) { } }
根据实际需求,制定合适的拒绝策略
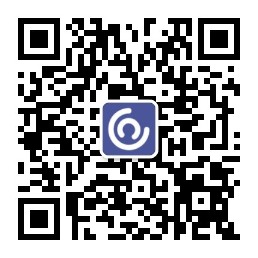
来源:江苏网站优化