一:分析随机读写程序的运行结果
/*将数据写到文本文件中,再从头到尾读取文件并输出到显示器*/
#include<stdio.h>
#include<stdlib.h>
int main()
{
int a[10], b[10], i;
FILE *iofile;
if((iofile=fopen("f1.dat","w+"))==NULL) /*用读写方式打开*/
{
printf("Cannot open file!");
exit(1);
}
printf("enter 10 integer numbers:\n");
for(i=0; i<10; i++)
{
scanf("%d", &a[i]);
fprintf(iofile, "%d ", a[i]);
}
printf("The numbers have been writen to file. \n");
printf("Display the data by read from file: \n");
fseek(iofile, 0, SEEK_SET); //亦可rewind(iofile);
for(i=0; i<10; i++)
{
fscanf(iofile, "%d", &b[i]);
printf("%d ", b[i]);
}
printf("\n");
fclose(iofile);
return 0;
}
运行结果:
fseek(iofile, 0, SEEK_SET);
fseek(iofile, 1, SEEK_SET); fseek(iofile, 2, SEEK_SET);
fseek(iofile, 3, SEEK_SET); fseek(iofile, 4, SEEK_SET);
分析:
首先明确,printf类都是以字符串形式打印(写到文本文件中),fseek(iofile,0,SEEK_SET);的位置指针是文件中的
将数据读到文件中后,文件中的数据是这种形式
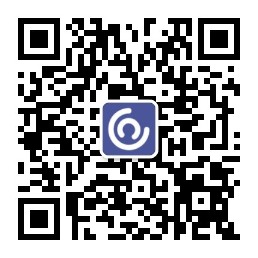
接下来进行fseek()操作,移动相应的字节,'1'是字符表示一个字节,文件中的空格也是字符表示一个字节
最后从文件指针指向的位置开始读取到缓冲区,再写到显示器上。
二:阅读以下程序,体会随机访问二进制文件中的数据块,数据在内存和文件间的流动
/*学生数据处理:有5个学生的数据,要求
(1)把它们保存到磁盘文件中;
(2)将磁盘文件中的第1,3,5个学生数据读入程序,并显示;
(3)将第3个学生的数据修改后存回磁盘文件中的原有位置;
(4)从磁盘文件读入5个学生的数据,并显示出来。
*/
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
typedef struct student
{
int num;
char name[20];
float score;
} Student;
int main( )
{
FILE *iofile;
int i;
if((iofile=fopen("stu_list.dat","wb+"))==NULL) //用读写方式打开
{
printf("Cannot open file!");
exit(1);
}
Student stud[5]= {{1001,"Li",85},{1002,"Fun",97.5},{1004,"Wang",54},{1006,"Tan",76.5},{1010,"ling",96}};
//(1)向磁盘文件输出5个学生的数据并显示出来
printf("(1)向磁盘文件输出5个学生的数据并显示出来\n");
for(i=0; i<5; i++)
{
fwrite((char*)&stud[i], sizeof(stud[i]), 1, iofile);
printf("%d %s %.1f\n", stud[i].num, stud[i].name, stud[i].score);
}
//(2)将磁盘文件中的第1,3,5个学生数据读入程序,并显示出来;
printf("(2)将磁盘文件中的第1,3,5个学生数据读入程序,并显示出来\n");
Student stud1[3]; //用来存放从磁盘文件读入的数据
for(i=0; i<5; i=i+2)
{
fseek(iofile, i*sizeof(stud[i]),SEEK_SET); //定位于第0,2,4学生数据开头
fread((char *)&stud1[i/2], sizeof(stud1[0]), 1, iofile);
//先后读入3个学生的数据,存放在stud1[0],stud[1]和stud[2]中
printf("%d %s %.1f\n", stud1[i/2].num, stud1[i/2].name, stud1[i/2].score);
//输出stud1[0],stud[1]和stud[2]各成员的值
}
//(3) 将第3个学生的数据修改后存回磁盘文件中的原有位置。
printf("(3)将第3个学生的数据修改后存回磁盘文件中的原有位置\n");
stud[2].num=1012; //修改第3个学生(序号为2)的数据
strcpy(stud[2].name, "Wu");
stud[2].score=60;
fseek(iofile, 2*sizeof(stud[0]),SEEK_SET); //定位于第3个学生数据的开头
fwrite((char *)&stud[2], sizeof(stud[2]), 1, iofile); //更新第3个学生数据
//(4)从磁盘文件读入修改后的5个学生的数据并显示出来。
printf("(4)从磁盘文件读入修改后的5个学生的数据并显示出来\n");
fseek(iofile, 0, SEEK_SET); //重新定位于文件指针位置
for(i=0; i<5; i++)
{
fread((char *)&stud[i],sizeof(stud[i]),1,iofile); //读入5个学生的数据
printf("%d %s %.1f\n", stud[i].num, stud[i].name, stud[i].score);
}
fclose(iofile);
return 0;
}
读写二进制文件相对于文本文件的优势:
fscanf()、fprintf()读写格式化数据,格式读写错误会影响输入输出的内容;
二进制文件是计算机的内部形式,不需要转换,
fread()、fwrite()直接读写数据块,不易出错,便于用feek()随机访问文件中的数据块。
三:文件的操作整理:
打开文件:fp=fopen("文件位置","读写方式");
文件指针的随机移动:
void rewind(FILE *stream);
int fseek(FILE *stream, long offset, int startPos);
对文件读或写:
1)读写格式化数据
int fscanf(文件指针,格式字符串,输出表列);
int fprintf(文件指针,格式字符串,输出表列);
2)以字符为单位
int fgetc(FILE *fp);
int fputs(int ch, FILE *fp);
3)以字符串为单位
char* fgets(char *str, int n, FILE *stream);
int fputs(char *str, FILE *stream);
4)二进制文件读写数据块
int fread(char *buffer, int size, int count, FILE *stream);
int fwrite(char *buffer, int size, int count, FILE *stream);
关闭文件:fclose(FILE *fp);
判断文件是否处于文件结束位置:
int feof(FILE *stream);