题目描述:
Given a string s1, we may represent it as a binary tree by partitioning it to two non-empty substrings recursively.
Below is one possible representation of s1 = "great"
:
great / \ gr eat / \ / \ g r e at / \ a t
To scramble the string, we may choose any non-leaf node and swap its two children.
For example, if we choose the node "gr"
and swap its two children, it produces a scrambled string "rgeat"
.
rgeat / \ rg eat / \ / \ r g e at / \ a t
We say that "rgeat"
is a scrambled string of "great"
.
Similarly, if we continue to swap the children of nodes "eat"
and "at"
, it produces a scrambled string "rgtae"
.
rgtae / \ rg tae / \ / \ r g ta e / \ t a
We say that "rgtae"
is a scrambled string of "great"
.
Given two strings s1 and s2 of the same length, determine if s2 is a scrambled string of s1.
Example 1:
Input: s1 = "great", s2 = "rgeat"
Output: true
Example 2:
Input: s1 = "abcde", s2 = "caebd"
Output: false
算法实现:
算法实现原理比较简单,就是如果两个字符串是题目中的这种关系,那么它们一定可以不断的执行一种操作:s1和s2可以分成两个部分,s1的某个部分和s2的某个部分满足这种关系(Scramble),s1的另一个部分和s2的另一个部分满足这种关系。而两个字符串要满足这种关系的前提是两个字符串具有完全相同的字符。具体的我们通过递归的方式实现。
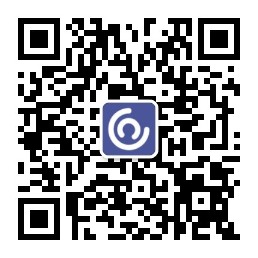
class Solution {
public:
bool isScramble(string s1, string s2) {
if(s1.empty() || s2.empty())return false;
if(s1.length() != s2.length())return false;
int n = s1.length();
return isScr(s1, s2);
}
bool isScr(string s1, string s2){
// bool flag = false;
// cout << s1 << "," << s2 << endl;
int n = s1.length();
if(n == 1)return s1 == s2;
if(n == 2)return s1 == s2 || (s1[0] == s2[1] && s1[1] == s2[0]);
unordered_map<char, int> m;
int i = 0;
int plus = 0;
//分两种情况考虑
//一是s1的根节点的两个子树没有交换,即s1的前一部分对应s2的前一部分,s1的后部分与s2的后部分对应
//二是s1的根节点的两个子树有交换,即s1的前一段对应s2的后半段,s1的后一段和s2的前一段对应
//第一种情况
while(i < n){
m[s1[i]] ++;
if(m[s1[i]] == 1)plus ++;
m[s2[i]] --;
if(m[s2[i]] == 0)plus --;
// cout << i << "," << plus << endl;
i ++;
if(plus == 0)break;
}
if(i < n && plus == 0)
if(isScr(s1.substr(0,i), s2.substr(0,i)) && isScr(s1.substr(i, n - i), s2.substr(i,n - i)))
{
return true;
}
//如果第一种情况不能成功,不意味着一定会失败,可能第二种情况会成功
i = 0, plus = 0;
m.clear();
while(i < n){
m[s1[i]] ++;
if(m[s1[i]] == 1)plus ++;
m[s2[n - 1 - i]] --;
if(m[s2[n - 1 - i]] == 0)plus --;
// cout << i << "," << plus << endl;
i ++;
if(plus == 0)break;
}
// cout << i << endl;
//两个字符串种字符不匹配
if(i == n && plus != 0)return false;
//虽然两个字符串字符匹配,但是其不能各自分成两个部分,并在两个字符串间匹配
if(i == n)return false;
return isScr(s1.substr(0,i), s2.substr(n - i)) && isScr(s1.substr(i, n - i), s2.substr(0,n - i));
}
};