- 服务器启动文件
1 import java.io.IOException;
2 import java.net.ServerSocket;
3 import java.net.Socket;
4
5 public class MyServer {
6
7 public static void main(String[] args) {
8
9 ServerSocket serverSocket = null;
10 Socket socket = null;
11
12 try {
13
14 //创建服务器套接字ServerSocket,并绑定服务器端口号,port:1-65536,>1024
15 serverSocket =new ServerSocket(9999);
16
17 while(true) {//可以响应多个各户端
18 System.out.println("服务器开始运行....");
19 //阻塞等待客户端
20 socket = serverSocket.accept();
21
22 //开启线程响应客户端,并将通信套接字socket转入子线程
23 new MyServerThread(socket).start();
24 }
25
26 } catch (IOException e) {
27
28 e.printStackTrace();
29
30 }finally {
31 //完成或者异常时,关闭套接字
32 try {
33
34 socket.close();
35 serverSocket.close();
36
37 } catch (IOException e) {
38
39 e.printStackTrace();
40
41 }
42
43 }
44
45 }
46 }
- 服务器线程文件
1 import java.io.IOException;
2 import java.io.InputStream;
3 import java.io.OutputStream;
4 import java.net.Socket;
5
6 public class MyServerThread extends Thread{
7
8 private Socket socket = null;
9 private InputStream in;
10 private OutputStream out;
11
12 public MyServerThread(Socket socket) {
13
14 this.socket = socket;
15
16 //客户端IP
17 String ip = socket.getInetAddress().getHostAddress();
18 int port = socket.getPort();
19 System.out.println("IP:" +ip +"port:"+port);
20 }
21
22 //发送和接收数据
23 @Override
24 public void run() { //响应客户端(数据收发)
25
26 try {
27 //获取输入输出流
28 in = socket.getInputStream();
29 out = socket.getOutputStream();
30
31 while(true) {//多次
32 //登陆协议 loginnamehellopass123456end
33
34 byte []b = new byte[32];//用于存放read到的数据流
35 in.read(b);//读取数据并存放到b数组中
36
37 String data = new String(b);//将字节流转为字符串
38
39 //读取数据解析登陆信息
40 String logo = data.substring(0,5);//login标志
41 String name = data.substring(data.indexOf("name")+4,data.indexOf("pass"));//data.indexOf("name")返回n的下标
42 String pass = data.substring(data.indexOf("pass")+4,data.indexOf("end"));//data.indexOf("name")返回n的下标
43
44 String msg ="";
45 if (logo.equals("login")) {//登陆?
46 if (name.equals("hello")) {
47 if (pass.equals("123456")) {
48 msg += "登录成功";
49 }
50 else {
51 msg += "密码错误";
52 }
53 }
54 else {
55 msg += "用户不存在";
56 }
57 }
58 else {
59 msg += "输入错误";
60 }
61
62 out.write(msg.getBytes());//将字符串转为字节数组发送到客户端
63 out.flush();
64
65 }
66 } catch (IOException e) {
67
68 e.printStackTrace();
69
70 }finally {
71
72 //g关闭流和套接字
73 try {
74
75 in.close();
76 out.close();
77 socket.close();
78
79 } catch (IOException e) {
80
81 e.printStackTrace();
82
83 }
84 }
85 }
86 }
1 import java.io.IOException;
2 import java.io.InputStream;
3 import java.io.OutputStream;
4 import java.net.Socket;
5 import java.util.Scanner;
6
7 public class MyClient {
8
9 public static void main(String[] args) {
10
11 Socket socket = null;
12 InputStream in = null;
13 OutputStream out = null;
14
15 try {
16 //1.创建通信套接字,并绑定IP和PORT
17 socket = new Socket("127.0.0.1", 9999);//127.0.0.1 B/C都在
18
19 //2.获取输入输出流
20 in = socket.getInputStream();
21 out = socket.getOutputStream();
22
23 Scanner input = new Scanner(System.in);//从控制台获取数据
24
25 while(true) {//循环发送和接收消息
26
27 String msg = input.next();
28
29 //fa
30 out.write(msg.getBytes());
31 out.flush();
32
33 //收
34 byte []b = new byte[32];
35 in.read(b);
36 String data = new String(b);
37 System.out.println(data.trim());//装不满时去空隔
38
39 }
40
41
42 } catch (IOException e) {
43 e.printStackTrace();
44 }finally {
45 try {
46 out.close();
47 in.close();
48 socket.close();
49 } catch (IOException e) {
50 e.printStackTrace();
51 }
52
53 }
54
55
56 }
57 }
- 运行服务端

- 运行客户端后服务器获得信息

- 客户端操作以及对应的服务端响应结果
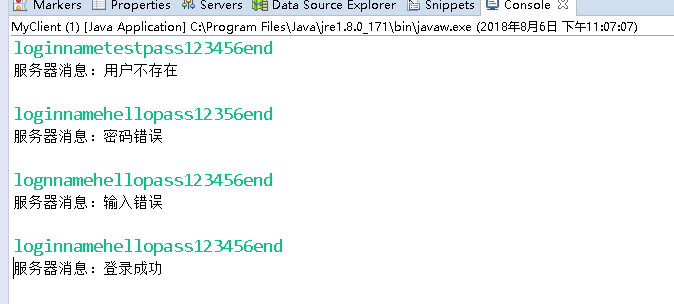