目录
图像读取主要用了两个库,不同的库是不同的对象:
# plt.imread和PIL.Image.open读入的都是RGB顺序
from PIL import Image
img = Image.open('xxxx.png') # 读取Image对象
img.save('xxx.png')
'''
print(img.mode) # 有'1', 'L', 'P', 'RGB', 'RGBA'等
'1': 表示黑白模式照片
'L': 表示灰度模式照片
'RGB': 表示RGB通道模式的彩色照片
'RGBA': 表示RGB通道及Alpha通道的照片
'''
img.show() # 显示图片
img.convert('L') # 转换为'L'模式
img.crop((20,30,300,200)) # 裁剪
# Image.eval(img, function) # 对每个像素/通道进行函数处理
import cv2
# opencv中cv2.imread读入的是BGR通道顺序
# flags=0是灰度模式,flags=1是默认的彩色模式
# im = cv2.imread('xxxx.png', flags=0) # 读取图像array对象、
im = cv2.imread("imgCode_grey200.jpg", flags=cv2.IMREAD_GRAYSCALE)
cv2.imwrite('imgCode_grey200.jpg', im)
plt.imshow(im) # 显示图片
# plt.show()
# plt.close()
# cv2.imshow('im', im) # 显示图片
## PIL.Image.open和cv2.imread的比较与相互转换的方法
# 当图片是png格式,读取结果是一致的;
# 当图片是jpg格式时,读取结果是不一致的。
# 这可能是因为Image.open 与 cv2.imread 在解码jpg时运算有差异。
# 简单转换
# im = np.array(img, np.uint8) # copy=True
# im = np.asarray(img, np.uint8) # copy=False
# 不设置dtype为数值的话,得到的可能是布尔值的数组,比如二值化后的图片
im = np.asarray(img)
# img = Image.fromarray(np.uint8(im))
img = Image.fromarray(im)
# 标准转换
def PILImageToCV(imagePath):
# PIL Image转换成OpenCV格式
img = Image.open(imagePath)
plt.imshow(img)
img = cv2.cvtColor(np.asarray(img), cv2.COLOR_RGB2BGR)
plt.imshow(img)
plt.show()
def CVImageToPIL(imagePath):
# OpenCV图片转换为PIL image
img = cv2.imread(imagePath)
plt.imshow(img)
img2 = Image.fromarray(cv2.cvtColor(img, cv2.COLOR_BGR2RGB))
plt.imshow(img2)
plt.show()
本次图像识别测试需要以下两个条件:
OCR软件:tesseract.exe,通过命令行调用来识别字符。
OCR软件的Python接口:pytesseract,内核是OCR软件tesseract
OCR:Optical Character Recognition (光学字符识别)
备注:另外的一个接口PyOCR,内核可包括tesseract或其他,但也得提前安装OCR软件。
import pytesseract
def get_result_by_imgCode_recognition(img):
# 进行验证码识别
result = pytesseract.image_to_string(img) # 接口默认返回的是字符串
# ''.join(result.split()) # 去掉全部空格和\n\t等
result = ''.join(list(filter(str.isalnum, result))) # 只保留字母和数字
return result
def pass_counter(img, img_value):
# 辨别是否识别正确
rst = get_result_by_imgCode_recognition(img)
if rst == img_value:
return 1
else:
return 0
def most_frequent(lst):
# 获取列表最频繁的元素,可用于集成投票获得识别结果
# return max(lst, key=lst.count)
return max(set(lst), key=lst.count)
1. 验证码获取
通过浏览器的开发者工具,发现验证码图片为base64编码的文件,通过解码后写入文件。
def fetch_imgCode():
# 获取验证码
url_imgCode = 'xxxx'
html = requests.post(url_imgCode)
'''
print(f'imgCode rsp: {html.text}')
imgCode rsp: {
"data":
{"image_buf_str": "/9j/4AAQ....KAP/9k=",
"image_code": "16501881494161"},
"error_code": 0, "msg": {"en-us": "Success", "zh-CN": "\u6210\u529f"},
"request": "POST /public/verificationCode/imgCode"}
'''
html = html.json()
image_buf_str = html['data']['image_buf_str']
image_code = html['data']['image_code']
# 保存base64编码的图片为图片文件
with open(f'./imgCode_png_raw/imgCode_{image_code}.png', 'wb') as f:
f.write(base64.b64decode(image_buf_str))
return image_code
2. 登录网站
通过向网站发起post请求,可登录网站,一般情况下:
输入image_code对应的正确的验证码的值image_value,即可登录成功。
反过来,如果登录成功,也意味着我们识别出来的验证码值image_value是正确。
HEADERS_PORTAL = {
'User-Agent': 'xxxx',
"Content-Type": "application/json",
}
def login(image_code, image_value):
login_flag = False
url_login = 'xxxx'
data_login = {"account": "DEMO_Tong",
"password": "9xdsaGcy",
"image_code": image_code,
"captcha": image_value,
"nickname": "DEMO_Tong", "client_type": 100}
html = requests.post(url_login, data=json.dumps(data_login), headers=HEADERS_PORTAL)
# print(f'login info: {html.text}')
html = html.json()
if html.get('data'):
if html.get('data').get('token'):
login_flag = True
return login_flag
3. 图像处理
灰度处理、二值处理、去噪、膨胀及腐蚀、倾斜矫正、字符切割、归一化等
# 灰度处理和二值处理
# lookup_table = [0 if i < 200 else 1 for i in range(256)]
def gray_processing(img, threshold = 127):
# 转为灰度模式
img = img.convert('L')
# 转为二值模式,阈值默认是 127,大于为白色,否则黑色。
# 为什么127呢,256/2=128, 2^8=256, 一个字节byte是8个比特bit
# image.convert('1') # 即 threshold = 127
# threshold = 125
lookup_table = [0 if i < threshold else 1 for i in range(256)]
img = img.point(lookup_table, '1')
return img
# 膨胀腐蚀法
def erode_dilate(im, threshold=2):
# im = cv2.imread('xxx.jpg', 0)
# cv2.imshow('xxx.jpg', im)
# (threshold, threshold) 腐蚀矩阵大小
kernel = np.ones((threshold, threshold), np.uint8)
# 膨胀
erosion = cv2.erode(im, kernel, iterations=1)
# cv2.imwrite('imgCode_erosion.jpg', erosion)
# Image.open('imgCode_erosion.jpg').show()
# # 腐蚀
# eroded = cv2.dilate(erosion, kernel, iterations=1)
# cv2.imwrite('imgCode_eroded.jpg', eroded)
# Image.open('imgCode_eroded.jpg').show()
return erosion
4.验证码识别测试
测试说明
根据不同的图像处理方式,进行验证码识别测试,积累成功识别示例的同时,观察不同处理方式的识别效果。测试中获取的验证码具有随机性,有容易识别的,也有不容易识别的,但客观上属于同一难度的验证码。
本次识别测试将分为3组,每次识别10000张,通过模拟登录网站来验证是否识别正确。
第一组直接识别原图片文件,标签为“raw”
第二组识别灰度处理和阈值为200的二值处理后的图片对象,标签为“gray”
第三组识别经灰度、二值和膨胀处理后的图片对象,标签为“erosion”
识别的结果根据图像处理方式和识别正确与否,放在不同文件夹,识别结果也追加到文件名:
imgCode_png_raw:存放从网站保存下来的原始图片
imgCode_png_raw_pass:存放raw测试识别正确的原始图片
imgCode_png_raw_fail:存放raw测试识别失败的原始图片
imgCode_png_raw_gray_pass:存放gray测试识别正确的原始图片
imgCode_png_raw_gray_fail:存放gray测试识别失败的已处理后的图片
imgCode_png_raw_gray_erosion_pass:存放erosion测试识别正确的原始图片
imgCode_png_raw_gray_erosion_fail:存放erosion测试识别失败的已处理后的图片
注意:通过浏览器的开发工具可以发现,验证码使用的字体应该是 element-icons.535877f5.woff
测试代码
from tqdm import tqdm, trange
from tqdm.contrib import tzip # tqdm是进度条模块,为了便于观察处理进度
TEST_TOTAL = 10000 # 测试数量1万张
def test_raw():
print('raw: ')
pass_count = 0
# for _ in range(TEST_TOTAL):
for _ in trange(TEST_TOTAL):
try:
image_code = fetch_imgCode()
img = Image.open(f'./imgCode_png_raw/imgCode_{image_code}.png')
result = get_result_by_imgCode_recognition(img)
login_flag = login(image_code, result)
if login_flag:
img.save(f'./imgCode_png_raw_pass/imgCode_{image_code}_{result}.png')
pass_count += 1
else:
img.save(f'./imgCode_png_raw_fail/imgCode_{image_code}_{result}.png')
except:
info = sys.exc_info()
print(info)
print(f'pass_rate: {pass_count/TEST_TOTAL*100}')
def test_gray():
print('gray: ')
pass_count = 0
for _ in trange(TEST_TOTAL):
try:
image_code = fetch_imgCode()
img = Image.open(f'./imgCode_png_raw/imgCode_{image_code}.png')
img_gray = gray_processing(img, threshold=200)
result = get_result_by_imgCode_recognition(img_gray)
login_flag = login(image_code, result)
if login_flag:
img.save(f'./imgCode_png_raw_gray_pass/imgCode_{image_code}_{result}.png')
pass_count += 1
else:
img_gray.save(f'./imgCode_png_raw_gray_fail/imgCode_{image_code}_{result}.png')
except:
info = sys.exc_info()
print(info)
print(f'pass_rate: {pass_count/TEST_TOTAL*100}')
def test_erosion():
print('erosion: ')
pass_count = 0
for _ in trange(TEST_TOTAL):
try:
image_code = fetch_imgCode()
img = Image.open(f'./imgCode_png_raw/imgCode_{image_code}.png')
img_gray = gray_processing(img, threshold=200)
im = np.asarray(img_gray, np.uint8) # gray之后变成array,值变为0和1,有效去噪点
erosion = erode_dilate(im, threshold=2)
img1 = Image.fromarray(erosion*255) # 值为0到1,整个图片都是黑色的。
result = get_result_by_imgCode_recognition(img1) # 这里用array也可以
login_flag = login(image_code, result)
if login_flag:
img.save(f'./imgCode_png_raw_gray_erosion_pass/imgCode_{image_code}_{result}.png')
pass_count += 1
else:
img1.save(f'./imgCode_png_raw_gray_erosion_fail/imgCode_{image_code}_{result}.png')
except:
info = sys.exc_info()
print(info)
print(f'pass_rate: {pass_count/TEST_TOTAL*100}')
测试结果
5. 成功示例的再识别测试
测试说明
将通过raw、gray、erosion识别测试正确的示例按照1:1:1的样本比例拷贝到imgCode_pass文件夹,此时的验证码样本都是有正确识别结果的,且数量一定和样本比例均衡,可以用三种处理方式进行再识别,比较三种处理方式的识别效果。
此次再识别测试的样本比例1:1:1,各8844张,共26532张。
测试代码
def test_pass_raw():
pass_list = os.listdir('./imgCode_pass')
pass_value_list = [img_file[-8:-4] for img_file in pass_list]
pass_cnt1 = 0
pass_amt = len(pass_list)
print(f'pass_amt: {pass_amt}')
# for img_file, img_value in zip(pass_list, pass_value_list):
for img_file, img_value in tzip(pass_list, pass_value_list):
# raw
img = Image.open(f'./imgCode_pass/{img_file}')
pass_cnt1 += pass_counter(img, img_value)
print(f'raw: \npass_rate:{pass_cnt1 / pass_amt * 100}')
def test_pass_gray():
pass_list = os.listdir('./imgCode_pass')
pass_value_list = [img_file[-8:-4] for img_file in pass_list]
pass_cnt2 = 0
pass_amt = len(pass_list)
print(f'pass_amt: {pass_amt}')
# for img_file, img_value in zip(pass_list, pass_value_list):
for img_file, img_value in tzip(pass_list, pass_value_list):
# raw
img = Image.open(f'./imgCode_pass/{img_file}')
# raw + grey200
img = gray_processing(img, threshold=200)
pass_cnt2 += pass_counter(img, img_value)
print(f'raw + grey200: \npass_rate:{pass_cnt2/pass_amt*100}')
def test_pass_erosion():
pass_list = os.listdir('./imgCode_pass')
pass_value_list = [img_file[-8:-4] for img_file in pass_list]
pass_cnt3 = 0
pass_amt = len(pass_list)
print(f'pass_amt: {pass_amt}')
# for img_file, img_value in zip(pass_list, pass_value_list):
for img_file, img_value in tzip(pass_list, pass_value_list):
# raw
img = Image.open(f'./imgCode_pass/{img_file}')
# raw + grey200
img = gray_processing(img, threshold=200)
# raw + grey200 + erosion
im = np.asarray(img, np.uint8) # gray之后变成array,值变为0和1,有效去噪点
erosion = erode_dilate(im, threshold=2)
img1 = Image.fromarray(erosion*255) # 值为0到1,整个图片都是黑色的。
pass_cnt3 += pass_counter(img1, img_value)
print(f'raw + grey200 + erosion(Image): \npass_rate:{pass_cnt3/pass_amt*100}')
测试结果
测试注意事项
此次测试特别需要注意样本比例,如果样本全为通过raw识别测试正确的来进行再识别,使用raw方式将为100%识别正确。下图是使用大部分为raw识别成功的示例来进行再识别的结果,发现不同处理方式的识别模型的识别能力呈下降趋势,越接近raw识别模型的模型精度越好,反正越差。
6. 集成融合投票模型,并使用多进程机制运行程序
测试说明
基于不同的模型的识别效果不一,考虑集成学习的模型融合,使用投票法,通过raw、gray、erosion三种模型进行识别预测投票,将票数多的识别结果作为集成融合投票模型的识别结果,来进行登录验证。
基于集成融合投票模型需要对同一张验证码示例进行3次识别,比较耗时,故使用多进程机制并行地运行程序,减少程序所消耗的时间。
测试代码
def test_ensemble_vote(kwargs):
result_list = []
image_code = fetch_imgCode()
img = Image.open(f'./imgCode_png_raw/imgCode_{image_code}.png')
result_list.append(get_result_by_imgCode_recognition(img))
img_gray = gray_processing(img, threshold=200)
result_list.append(get_result_by_imgCode_recognition(img_gray))
im = np.asarray(img_gray, np.uint8) # gray之后变成array,值变为0和1,有效去噪点
erosion = erode_dilate(im, threshold=2)
img1 = Image.fromarray(erosion*255) # 值为0到1,整个图片都是黑色的。
result_list.append(get_result_by_imgCode_recognition(img1))
result = max(result_list, key=result_list.count)
login_flag = login(image_code, result)
return login_flag
def test_ensemble_vote_multi():
print('test_ensemble_vote_multi: ')
from multiprocessing import Pool
pool = Pool()
pool_result_list = pool.map(test_ensemble_vote, trange(TEST_TOTAL))
pool.close()
pool.terminate()
pool.join()
pass_count = pool_result_list.count(True)
print(f'pass_rate: {pass_count/TEST_TOTAL*100}')
测试结果
单进程运行程序的结果
并行运行程序时的效果及结果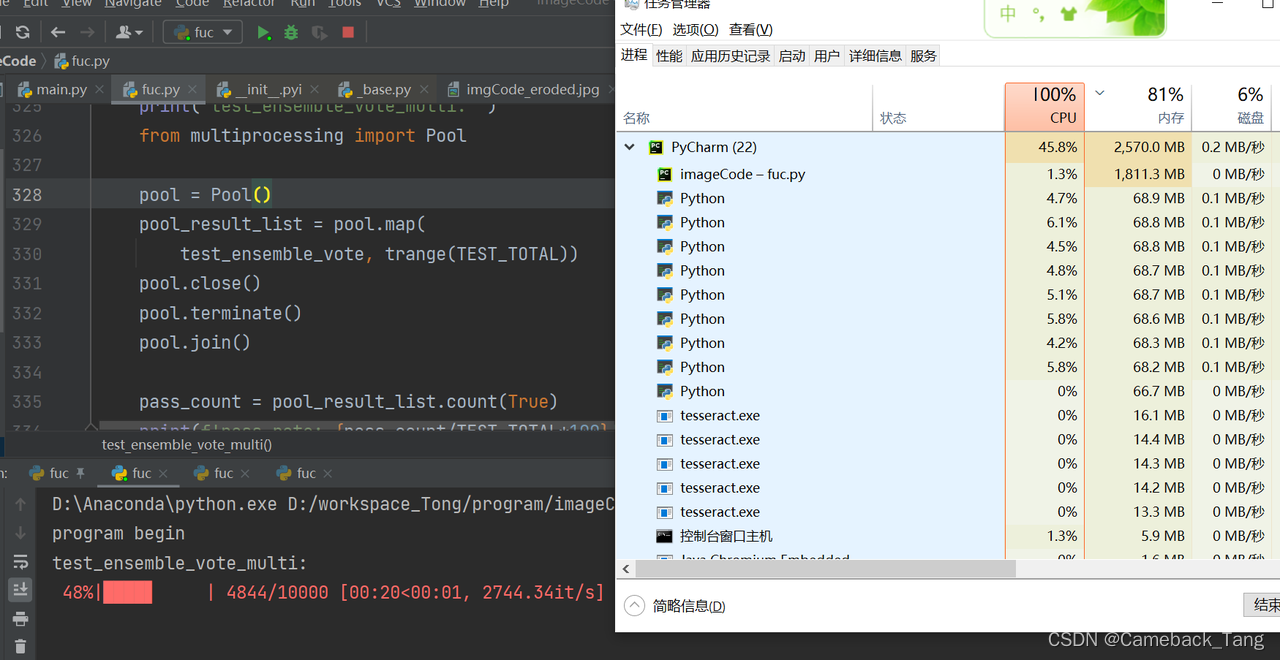
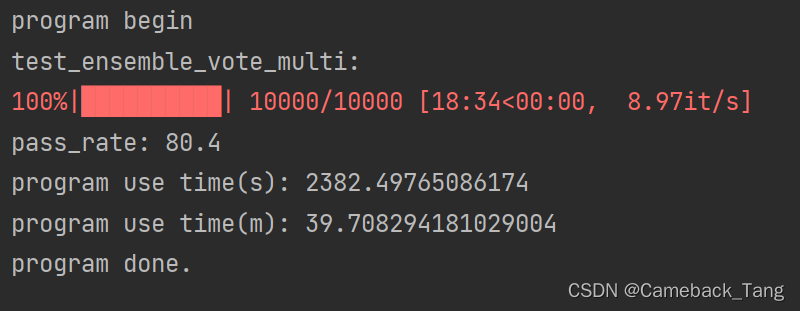
7. 失败示例的再识别
使用不同二值化阈值识别的融合投票模型对元模型(raw、gray或erosion)识别失败的例子进行再识别。
def test_fail():
## 单独一张图片,不同的二值化阈值,最频繁预测结果
# img = Image.open(f'./imgCode_fail/imgCode_16501101286728_359.png')
# img.show()
# result_list = []
# for i in trange(120,200,1):
# img_gray = gray_processing(img, threshold=i)
# img_gray.show()
# result = get_result_by_imgCode_recognition(img_gray)
# result_list.append(result)
# print(f'most_frequent(lst): {most_frequent(result_list)}')
## 多张图片,不同灰度阈值,最频繁预测结果,目的是寻找最佳阈值
fail_list = os.listdir('./imgCode_fail')
result_list_1 = []
for img_file in fail_list:
img = Image.open(f'./imgCode_fail/{img_file}')
result_list_2 = []
for i in trange(120,200,10):
img_gray = gray_processing(img, threshold=i)
result = get_result_by_imgCode_recognition(img_gray)
result_list_2.append(result)
result_list_1.append(result_list_2)
for img_file, lst in zip(fail_list, result_list_1):
print(f'{img_file}, most_frequent(lst): {most_frequent(lst)}')
8.其他