一、API 调用实现
cv2.resize(src, dst=None, size, fx=None, fy=None, interpolation=None)
参数解释:src (原图片),dst (输出图片),size (输出图片尺寸),fx (沿X轴的缩放系数),fy (沿Y轴的缩放系数),interpolation (插入方式)
size 输出图片尺寸格式为 (宽, 高)
interpolation 插值方法:
选项 | 说明 |
---|---|
cv2. INTER_LINEAR | 双线性插值(默认设置) |
cv2.INTER_NEAREST | 最近邻域插值 |
cv2. INTER_AREA | 像素关系重采样 |
cv2. INTER_CUBIC | 4x4像素邻域的双三次插值 |
cv2. INTER_LANCZOS4 | 8x8像素邻域的Lanczos插值 |
代码实现:
import cv2
img = cv2.imread('image.png', 1)
imageInfo = img.shape
height = imageInfo[0] # 图像高
width = imageInfo[1] # 图像宽
dstHeight = int(height*0.5)
dstWidth = int(width*0.5)
# 默认为双线性插值法
dst = cv2.resize(img, (dstWidth, dstHeight))
cv2.imshow('dst', dst)
cv2.waitKey(0)
cv2.destroyAllWindows()
二、最近临域插值法
确定图像的缩放比 — > 可知目标图像的尺寸 —> 目标图像像素位置除以缩放比可确定该点对应原图像的位置,如果结果为小数,则四舍五入。
假 设 : s r c ( 原 ) 10 × 20 缩 放 比 0.5 − − − > d s t ( 缩 ) 5 × 10 d s t ( 1 , 2 ) − − − > s r c ( 2 , 4 ) n e w X = x d s t / 0.5 = 1 / 0.5 = 2 n e w Y = y d s t / 0.5 = 2 / 0.5 = 4 假设:src(原) 10×20 缩放比0.5 ---> dst(缩) 5×10 \\ dst \quad (1,2)--->src \quad (2,4) \\ newX = x_{dst}/0.5=1/0.5=2 \\ newY = y_{dst}/0.5 = 2/0.5 =4 假设:src(原)10×20缩放比0.5−−−>dst(缩)5×10dst(1,2)−−−>src(2,4)newX=xdst/0.5=1/0.5=2newY=ydst/0.5=2/0.5=4
代码实现:
import cv2
import numpy as np
img = cv2.imread('image.png', 1)
imgInfo = img.shape
height = imgInfo[0] # 图片高
width = imgInfo[1] # 图片宽
Zratio = 0.5 # 缩放比
dstHeight = int(height * Zratio)
dstWidth = int(width * Zratio)
dstImage = np.zeros((dstHeight, dstWidth,3), np.uint8) # 创建一个画板
for i in range(dstHeight):
for j in range(dstWidth):
newX = round(i/Zratio)
newY = round(j/Zratio)
dstImage[i,j] = img[newX, newY]
cv2.imshow('dst', dstImage)
cv2.waitKey(0)
cv2.destroyAllWindows()
三、双线性插值法
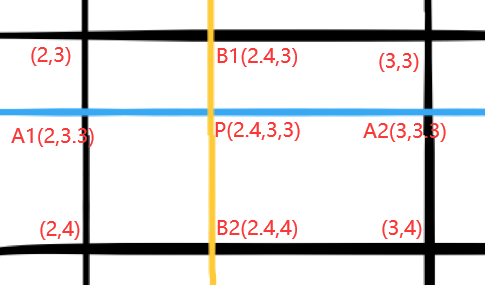
根据 A 推导:
A 1 = 0.7 × ( 2 , 3 ) + 0.3 × ( 2 , 4 ) = ( 2 , 3.3 ) A 2 = 0.7 × ( 3 , 3 ) + 0.3 × ( 3 , 4 ) = ( 3 , 3.3 ) P = A 1 × 0.6 + A 2 × 0.4 A1 = 0.7\times (2,3) + 0.3 \times (2,4) = (2,3.3) \\ A2 = 0.7\times (3,3) + 0.3 \times (3,4) = (3,3.3) \\ P = A1\times0.6 + A2\times0.4 \\ A1=0.7×(2,3)+0.3×(2,4)=(2,3.3)A2=0.7×(3,3)+0.3×(3,4)=(3,3.3)P=A1×0.6+A2×0.4
或者,根据 B 推导:
B 1 = 0.6 × ( 2 , 3 ) + 0.4 × ( 3 , 3 ) = ( 2.4 , 3 ) B 2 = 0.6 × ( 2 , 4 ) + 0.4 × ( 3 , 4 ) = ( 2.4 , 4 ) P = B 1 × 0.7 + B 2 × 0.3 B1 = 0.6\times (2,3) + 0.4 \times (3,3) = (2.4,3) \\ B2 = 0.6\times (2,4) + 0.4 \times (3,4) = (2.4,4) \\ P = B1\times0.7 + B2\times0.3 B1=0.6×(2,3)+0.4×(3,3)=(2.4,3)B2=0.6×(2,4)+0.4×(3,4)=(2.4,4)P=B1×0.7+B2×0.3
进一步推到,假设 (2,3) 为 (i,j),P为(i+u, j+v),则可得出公式:
f ( i + u , j + v ) = ( 1 − u ) ( 1 − v ) f ( i , j ) + ( 1 − u ) v f ( i , j + 1 ) + u ( 1 − v ) f ( i + 1 , j ) + u v f ( i + 1 , j + 1 ) f(i+u,j+v) = (1-u)(1-v)f(i,j) + (1-u)vf(i,j+1) + u(1-v)f(i+1,j) + uvf(i+1,j+1) f(i+u,j+v)=(1−u)(1−v)f(i,j)+(1−u)vf(i,j+1)+u(1−v)f(i+1,j)+uvf(i+1,j+1)
代码实现:
import cv2
import numpy as np
img = cv2.imread('image.png', 1)
imgInfo = img.shape
height = imgInfo[0] # 图片高
width = imgInfo[1] # 图片宽
Zratio = 0.5 # 缩放比
dstHeight = int(height * Zratio)
dstWidth = int(width * Zratio)
dstImage = np.zeros((dstHeight, dstWidth, 3), np.uint8) # 创建一个画板
for i in range(dstHeight):
for j in range(dstWidth):
P_x = i / Zratio
P_y = j / Zratio
u = P_x - int(P_x)
v = P_y - int(P_y)
dstImage[i,j] = (1-u)*(1-v)*img[int(P_x),int(P_y)] + (1-u)*v*img[int(P_x),int(P_y)+1]
+ u*(1-v)*img[int(P_x)+1,int(P_y)] + u*v*img[int(P_x)+1,int(P_y)+1]
cv2.imshow('dst',dstImage)
cv2.waitKey(0)
cv2.destroyAllWindows()
四、图片缩放前后对比
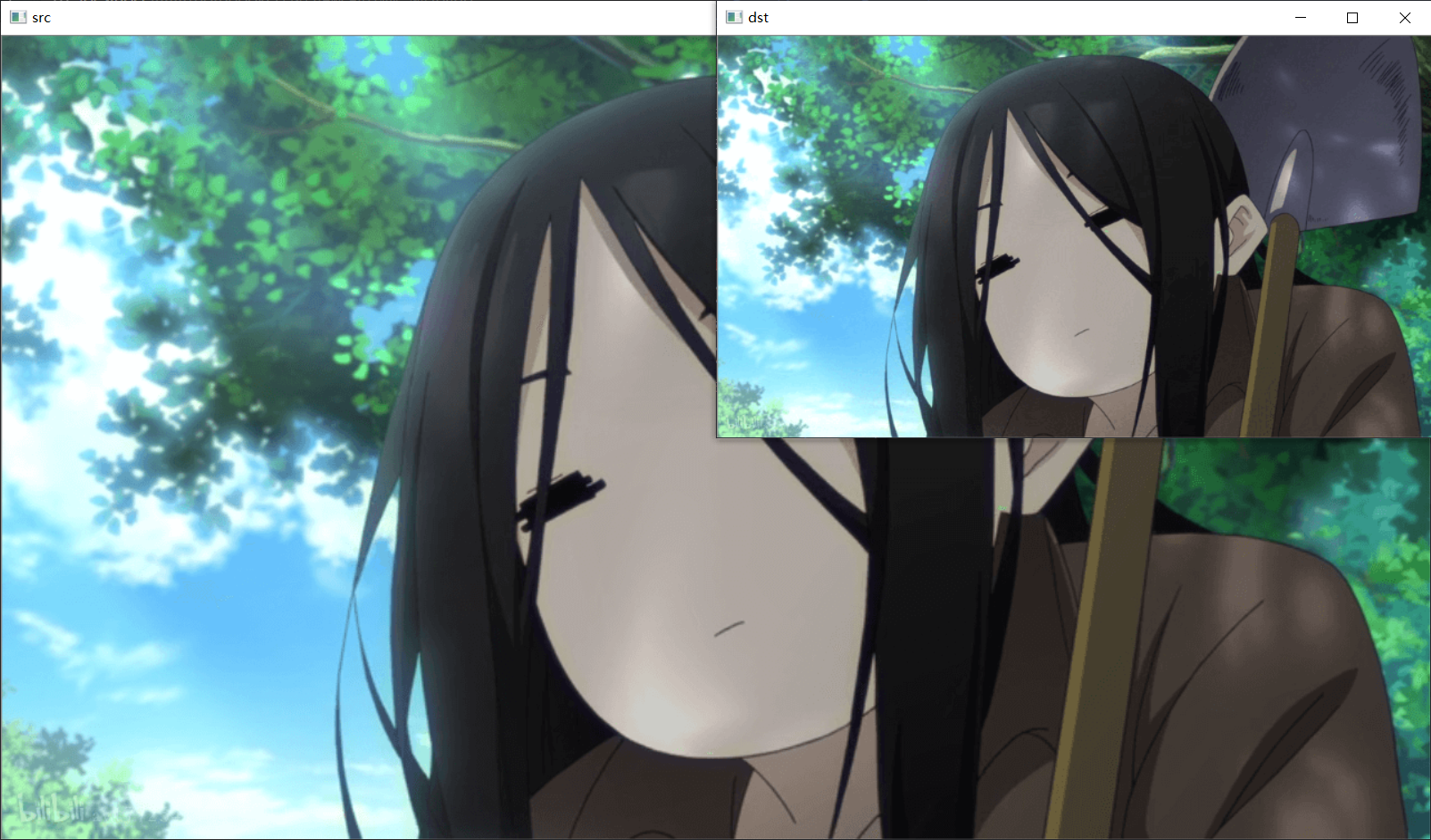