IOC:之前的代码类的初始化是通过new来实现的,比较麻烦,之前new的事情交给是工厂类来实现,我们直接调用工厂的数据。
IOC的用途就是用来作为工厂实现。
链接地址:https://spring.io/projects/spring-framework
操作文档地址:https://docs.spring.io/spring/docs/5.2.5.RELEASE/spring-framework-reference/
核心内容:https://docs.spring.io/spring/docs/5.2.5.RELEASE/spring-framework-reference/core.html#spring-core
使用步骤:
1.搭建maven项目工程
2.引入spring的jar包:
<!-- spring 核心 -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>5.2.5.RELEASE</version>
</dependency>
<!-- spring IOC -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-beans</artifactId>
<version>5.2.5.RELEASE</version>
</dependency>
<!-- spring 环境配置 -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.2.5.RELEASE</version>
</dependency>
3.创建application.xml文件作为配置文件(resource文件夹下)
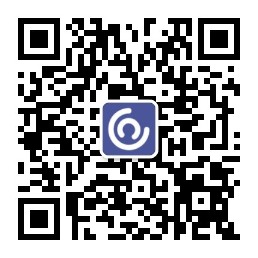
内容:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd">
</beans>
其中约束中需要下载文件,不然可能不能提示,这里下载的文件是叫做xsd的文件:
3.1 打开浏览器复制xsd文件链接,粘贴到地址栏,回车,另存,保存到自己文件夹中
3.2 eclipse -->window--> preferences -->xml -->xml catalog -->点击add按钮-->点击Location的File System按钮 -->选择xsd文件
-->key就会自动生成或者我们可以复制xsd上面一行链接其实就是key -->key type选择 Schema location -->保存即可
3.3 创建一个包,再创建一个类,作为测试,创建的是Student类
3.4 在xml中编写一个bean标签
<!--
id:唯一不为空即可
class:一个类的全限定名
-->
<bean id="stu" class="com.zcy.bean.Student"></bean>
3.5 测试
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext(
"application.xml");
Student stu = context.getBean(Student.class);
System.out.println(stu);
}
控制反转(IOC):spring帮助我们new数据,就像是一个工厂一样
4.spring虽然帮助我们初始化对象,默认是单例的
在bean标签中配置上 scope="prototype" 就可以是多例了(一般不会去配置,因为降低效率)
5. 设置对象的属性值:
5.1 创建类以及对应的属性
public class Student {
private String name;
private String sex;
private Integer age;
}
5.2 在配置文件中:
<bean id="stu" class="com.zcy.bean.Student">
这里的property就是设置属性,name就是属性名,value就是属性值
<property name="name" value="ZCY"></property>
<property name="sex" value="男"></property>
<property name="age" value="100"></property>
</bean>
这里是调用了set方法实现的
6.设置对象属性值(构造传递参数):(一般不这么用)
<bean id="stu" class="org.lgs.bean.Student">
<constructor-arg index="0" value="ZCY"></constructor-arg>
<constructor-arg index="1" value="男"></constructor-arg>
<constructor-arg index="2" value="100"></constructor-arg>
</bean>
7.设置较难的属性(Map,List,对象)
<bean id="stu" class="com.zcy.bean.Student">
<property name="name" value="ZCY"></property>
<property name="sex" value="男"></property>
<property name="age" value="100"></property>
<property name="parents">
<map>
<entry key="兄弟" value="左手"></entry>
<entry key="朋友" value="右手"></entry>
</map>
<!-- <list>
<value>香蕉</value>
<value>苹果</value>
<value>榴莲</value>
</list> -->
</property>
</bean>
对象的添加:
public class Student {
private String name;
private String sex;
private Integer age;
private Map<String, String> parents;
private ClassInfo cinfo;
}
让spring 创建班级类,id叫做class
<bean id="class" class="com.zcy.bean.ClassInfo">
<property name="cname" value="ZCY2020"></property>
</bean>
<bean id="stu" class="com.zcy.bean.Student">
<property name="name" value="ZCY"></property>
<property name="sex" value="男"></property>
<property name="age" value="100"></property>
<property name="parents">
<map>
<entry key="兄弟" value="左手"></entry>
<entry key="朋友" value="右手"></entry>
</map>
<!-- <list>
<value>香蕉</value>
<value>苹果</value>
<value>榴莲</value>
</list> -->
</property>
<!-- 这里的ref是引用的意思,调用其他spring自己new出来的对象 -->
<property name="cinfo" ref="class"></property>
</bean>
注入(DI):通过set或者构造方法设置任何类型的参数,到对应对象的属性中
8.属性文件配置:
参数写在properties文件中,例如:数据库
8.1.创建属性文件,例如:config.properties
8.2.在属性文件中编写参数:username=ZCY
8.3.在xml这边调用属性文件:
8.3.1 首先需要添加约束:
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:util="http://www.springframework.org/schema/util"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/util
https://www.springframework.org/schema/util/spring-util.xsd">
8.3.2 然后使用属性标签:classpath:告诉系统这个文件在src文件夹下
<util:properties id="config" location="classpath:config.properties"></util:properties>
8.3.3 属性文件在对应的bean里面使用,通过 #{属性的id.属性名}
<bean id="stu" class="com.zcy.bean.Student">
<property name="name" value="#{config.username}"></property>
<property name="sex" value="男"></property>
<property name="age" value="100"></property>
<property name="parents">
<map>
<entry key="兄弟" value="左手"></entry>
<entry key="朋友" value="右手"></entry>
</map>
</property>
<!-- 这里的ref是引用的意思,调用其他spring自己new出来的对象 -->
<property name="cinfo" ref="class"></property>
</bean>
9.xml这样配置还是太难了,如果service有100个类java文件,那么一个个bean去配置吗?
不,我们可以采用扫描的方式:
9.1 配置约束:
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:util="http://www.springframework.org/schema/util"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/util
https://www.springframework.org/schema/util/spring-util.xsd
http://www.springframework.org/schema/context
https://www.springframework.org/schema/context/spring-context.xsd">
9.2 配置扫描
扫描com.zcy.bean包下面的所有类(Java文件),把带注解的类全部new出来
<context:component-scan base-package=com.zcy.bean"></context:component-scan>
9.3 在对应需要new的类上添加注解
@Component
public class Student {}
9.4 属性注入:
方式1:
@Value("ZCY")
private String name;
方式2:
@Value("#{config.username}")
private String name;
方式3:重要
@Resource
private ClassInfo cinfo;
注解是企业中最常见的做法,因为好用