本文翻译自:Difference between HashMap, LinkedHashMap and TreeMap
What is the difference between HashMap
, LinkedHashMap
and TreeMap
in Java? Java中的HashMap
, LinkedHashMap
和TreeMap
什么区别? I don't see any difference in the output as all the three has keySet
and values
. 我没有看到输出有任何差异,因为所有三个都有keySet
和values
。 What are Hashtable
s? 什么是Hashtable
?
Map m1 = new HashMap();
m1.put("map", "HashMap");
m1.put("schildt", "java2");
m1.put("mathew", "Hyden");
m1.put("schildt", "java2s");
print(m1.keySet());
print(m1.values());
SortedMap sm = new TreeMap();
sm.put("map", "TreeMap");
sm.put("schildt", "java2");
sm.put("mathew", "Hyden");
sm.put("schildt", "java2s");
print(sm.keySet());
print(sm.values());
LinkedHashMap lm = new LinkedHashMap();
lm.put("map", "LinkedHashMap");
lm.put("schildt", "java2");
lm.put("mathew", "Hyden");
lm.put("schildt", "java2s");
print(lm.keySet());
print(lm.values());
#1楼
参考:https://stackoom.com/question/C7lJ/HashMap-LinkedHashMap和TreeMap之间的区别
#2楼
Just some more input from my own experience with maps, on when I would use each one: 从我自己的地图经验中获得更多的输入,当我使用每个地图时:
- HashMap - Most useful when looking for a best-performance (fast) implementation. HashMap - 在寻找最佳性能(快速)实现时最有用。
- TreeMap (SortedMap interface) - Most useful when I'm concerned with being able to sort or iterate over the keys in a particular order that I define. TreeMap(SortedMap接口) - 当我关心能够按照我定义的特定顺序对键进行排序或迭代时,最有用。
- LinkedHashMap - Combines advantages of guaranteed ordering from TreeMap without the increased cost of maintaining the TreeMap. LinkedHashMap - 结合TreeMap保证排序的优点,而不会增加维护TreeMap的成本。 (It is almost as fast as the HashMap). (它几乎与HashMap一样快)。 In particular, the LinkedHashMap also provides a great starting point for creating a Cache object by overriding the
removeEldestEntry()
method. 特别是,LinkedHashMap还为通过覆盖removeEldestEntry()
方法创建Cache对象提供了一个很好的起点。 This lets you create a Cache object that can expire data using some criteria that you define. 这使您可以使用您定义的某些条件创建可以使数据到期的Cache对象。
#3楼
HashMap makes absolutely not guarantees about the iteration order. HashMap绝对不保证迭代顺序。 It can (and will) even change completely when new elements are added. 当添加新元素时,它甚至可以(并且将)完全改变。 TreeMap will iterate according to the "natural ordering" of the keys according to their compareTo() method (or an externally supplied Comparator). TreeMap将根据其compareTo()方法(或外部提供的Comparator)根据键的“自然排序”进行迭代。 Additionally, it implements the SortedMap interface, which contains methods that depend on this sort order. 此外,它还实现了SortedMap接口,该接口包含依赖于此排序顺序的方法。 LinkedHashMap will iterate in the order in which the entries were put into the map LinkedHashMap将按照条目放入地图的顺序进行迭代
Look at how performance varying.. 看看性能如何变化..
Tree map which is an implementation of Sorted map. 树图是排序图的一种实现。 The complexity of the put, get and containsKey operation is O(log n) due to the Natural ordering 由于自然排序,put,get和containsKey操作的复杂性为O(log n)
#4楼
I prefer visual presentation: 我更喜欢视觉呈现:
╔══════════════╦═════════════════════╦═══════════════════╦═════════════════════╗
║ Property ║ HashMap ║ TreeMap ║ LinkedHashMap ║
╠══════════════╬═════════════════════╬═══════════════════╬═════════════════════╣
║ Iteration ║ no guarantee order ║ sorted according ║ ║
║ Order ║ will remain constant║ to the natural ║ insertion-order ║
║ ║ over time ║ ordering ║ ║
╠══════════════╬═════════════════════╬═══════════════════╬═════════════════════╣
║ Get/put ║ ║ ║ ║
║ remove ║ O(1) ║ O(log(n)) ║ O(1) ║
║ containsKey ║ ║ ║ ║
╠══════════════╬═════════════════════╬═══════════════════╬═════════════════════╣
║ ║ ║ NavigableMap ║ ║
║ Interfaces ║ Map ║ Map ║ Map ║
║ ║ ║ SortedMap ║ ║
╠══════════════╬═════════════════════╬═══════════════════╬═════════════════════╣
║ ║ ║ ║ ║
║ Null ║ allowed ║ only values ║ allowed ║
║ values/keys ║ ║ ║ ║
╠══════════════╬═════════════════════╩═══════════════════╩═════════════════════╣
║ ║ Fail-fast behavior of an iterator cannot be guaranteed ║
║ Fail-fast ║ impossible to make any hard guarantees in the presence of ║
║ behavior ║ unsynchronized concurrent modification ║
╠══════════════╬═════════════════════╦═══════════════════╦═════════════════════╣
║ ║ ║ ║ ║
║Implementation║ buckets ║ Red-Black Tree ║ double-linked ║
║ ║ ║ ║ buckets ║
╠══════════════╬═════════════════════╩═══════════════════╩═════════════════════╣
║ Is ║ ║
║ synchronized ║ implementation is not synchronized ║
╚══════════════╩═══════════════════════════════════════════════════════════════╝
#5楼
See where each class is in the class hierarchy in the following diagram ( bigger one ). 在下图中查看每个类在类层次结构中的位置( 较大的一个 )。 TreeMap implements SortedMap
and NavigableMap
while HashMap
doesn't. TreeMap实现了SortedMap
和NavigableMap
而HashMap
则没有。
HashTable
is obsolete and the corresponding ConcurrentHashMap
class should be used. HashTable
已过时,应使用相应的ConcurrentHashMap
类。
#6楼
These are different implementations of the same interface. 这些是同一界面的不同实现。 Each implementation has some advantages and some disadvantages (fast insert, slow search) or vice versa. 每种实现都有一些优点和一些缺点(快速插入,慢速搜索),反之亦然。
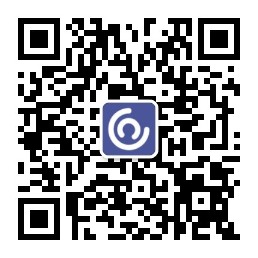
For details look at the javadoc of TreeMap , HashMap , LinkedHashMap . 有关详细信息,请查看TreeMap , HashMap , LinkedHashMap的javadoc。