データ構造及びアルゴリズムの分析は、P58変換サフィックスにコンジュゲートされています
#include<stdio.h>
#include <stdlib.h>
struct stackrecord {
int capacity;
int topofstack;
char *array;
};
typedef struct stackrecord* stack;
void makeempty(stack s) {
s->topofstack = -1;
}
int isempty(stack s) {
return s->topofstack == -1;
}
void disposestack(stack s) {
free(s->array);
free(s);
}
void push(char x, stack s) {
s->topofstack += 1;
s->array[s->topofstack] = x;
}
char top(stack s) {
return s->array[s->topofstack];
}
void pop(stack s) {
s->topofstack -= 1;
}
char topandpop(stack s) {
return s->array[s->topofstack--];
}
stack createstack(int maxelements) {
stack s;
s = (stack)malloc(sizeof(struct stackrecord));
if(s==NULL)
printf("out of space");
s->array = (char*)malloc(sizeof(int)*maxelements);
if (s->array == NULL)
printf("out ofspace");
s->capacity = maxelements;
makeempty(s);
return s;
}
void swc(char str[], stack s) {
int g = 0;
char b;
b = str[g];
while (b != '#') {
if (b >= 'a'&&b <= 'z') {
printf("%c", b);
}
else {
if (b == '+') {
while (top(s) == '+' || top(s) == '*') {
printf("%c", topandpop(s));
}
push('+',s);
}
if (b == '*') {
while (top(s) == '*') {
printf("%c", topandpop(s));
}
push('*',s);
}
if (b == '(') {
push('(', s);
}
if (b == ')') {
while (top(s) != '(') {
printf("%c", topandpop(s));
}
pop(s);
}
}
g++;
b = str[g];
}
while (isempty(s) == 0) {
printf("%c", topandpop(s));
}
}
int main() {
stack s = createstack(20);
char a;
char str[20];
a = getchar();
int k = 0;
while (a != '\n') {
str[k] = a;
a = getchar();
k++;
}
str[k] = '#';
swc(str, s);
return 0;
}
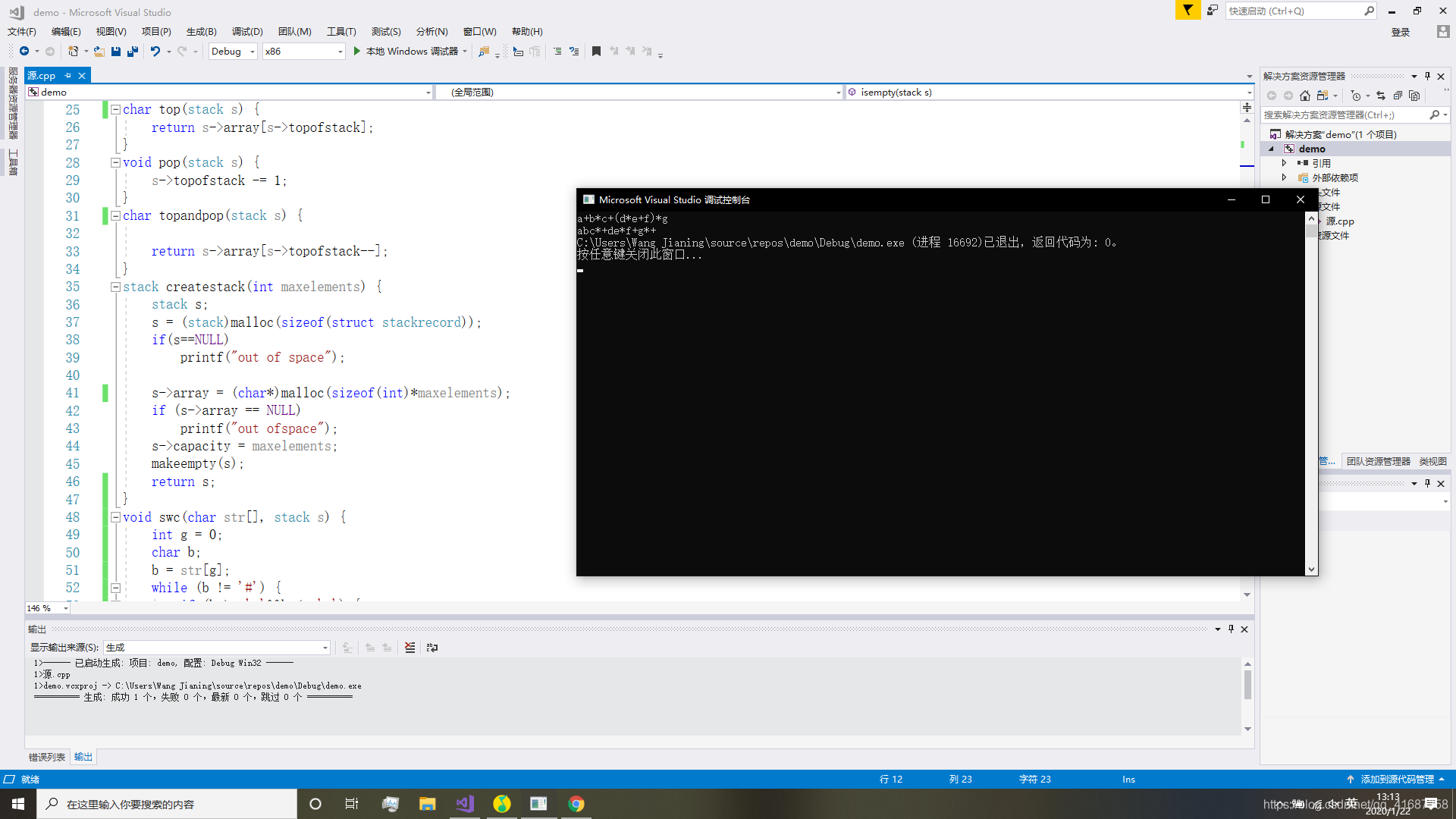