There is a catalog and you can choose freely.
- Remainder symbol %
The remainder sign is to find the remainder, such as 5%2=1, 17%6=5;
But it should be noted that the two numbers involved in the remainder must be integers.
- ampersand & - binary bitwise AND
like:
#include<stdio.h>
int main()
{
int a = 3, b = 5;
int c = a & b;
printf("%d\n", c);
return 0;
}
The ampersand sign is to write the numbers on the two sides of the symbol in binary, align them one by one, and the upper and lower numbers && will be true only if they are both true;
a=3 written in binary is 00000000000000000000000000000011;
b=5 written in binary is 00000000000000000000000000000101;
The operation of 2 numbers is 00000000000000000000000000000001;
Get the binary number 1. The binary number 1 is converted to decimal 1, so the output result is 1;
Summarize:
Perform operations in binary form and output the result in decimal form.
- or symbol |
The or symbol and the and symbol are similar, both operate in binary form and output in decimal. However, the | symbol is false only if 2 are false, and is true in other cases;
a=3 written in binary is 00000000000000000000000000000011;
a=5 written in binary is 00000000000000000000000000000101;
Perform OR operation 00000000000000000000000000000111;
111 is converted to decimal 7, that is, the output result is 7;
- XOR symbol ^
The XOR operation is also performed in binary form. The method of XOR operation is that the same is 0 and the difference is 1;
a=3 written in binary is 00000000000000000000000000000011;
a=5 written in binary is 00000000000000000000000000000101;
Perform XOR operation 000000000000000000000000000000110;
110 is converted to decimal 6, that is, the output result is 6;
- Right shift operator >>
#include<stdio.h>
int main()
{
int a = 8;
int c = a >>1;
printf("%d\n", c);
return 0;
}
The right shift operator is also performed in binary form, a>>1 means moving one bit to the right in binary form;
The binary representation of a=8 is 1000 as shown in the figure:
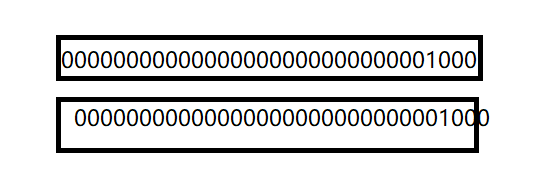
There are two types of right operators:
1. Arithmetic shift
The right side is discarded and the left side is filled with the original sign bit;
2. Logical shift
Discard the right side and add 0 to the left side;
However, most computers use arithmetic shifting. As shown in the figure above, the result obtained after shifting and filling is 100, which is converted to decimal 4; that is, the output result is 4;
It is important to pay special attention to the fact that negative numbers are stored in the memory as a complement code. You need to change the original code into a complement code and then perform the shift. I won’t go into details here. You can refer to
C language binary (original code, inverse code, complement code)
6. Left shift operator<<
The left shift operator shifts the binary number to the left by the corresponding number of digits and adds 0 to the right.
If a=8, c=a<<3; then adding 0 after shifting is 1000000, and converted to decimal is 64.
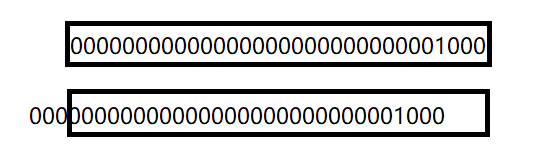
This article involves a lot of binary issues and does not go into details. You can refer to
C language binary http://t.csdn.cn/UilQ8