- jcefパッケージのインポート
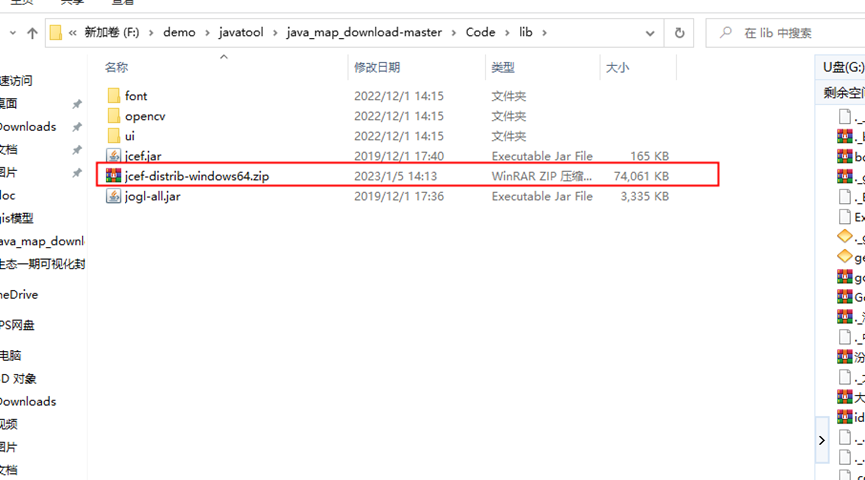
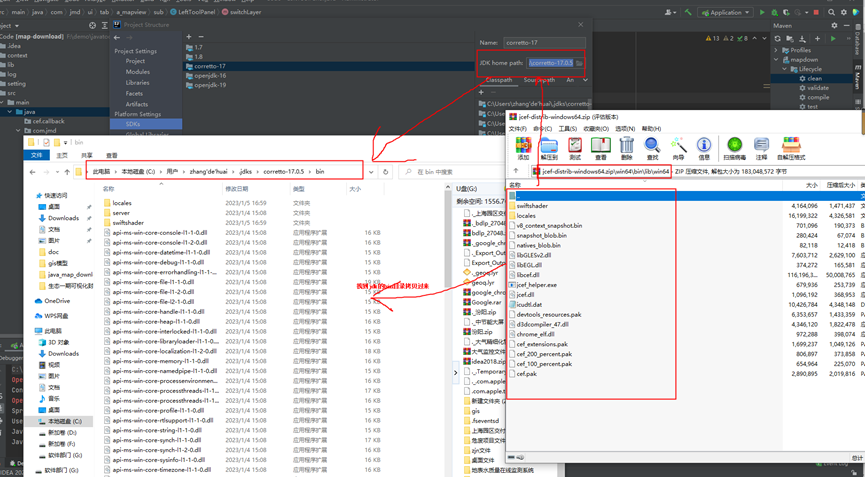
- テーブル構造設計
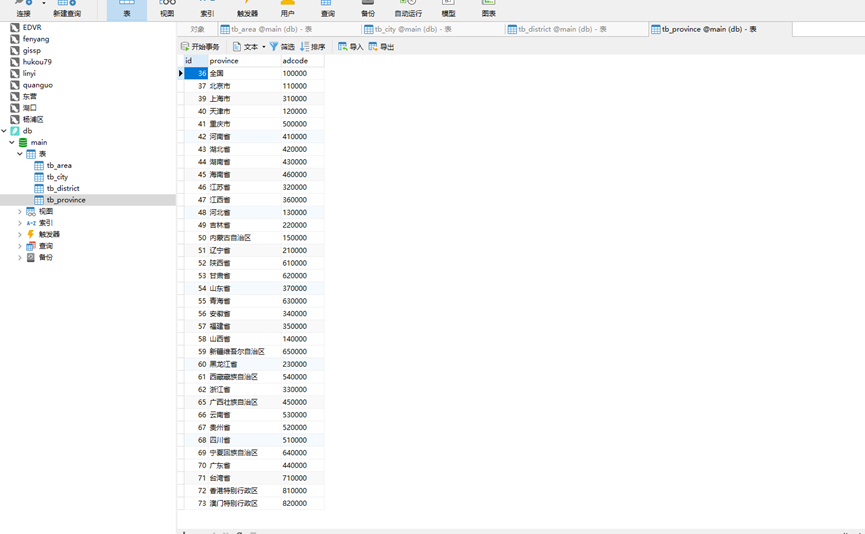
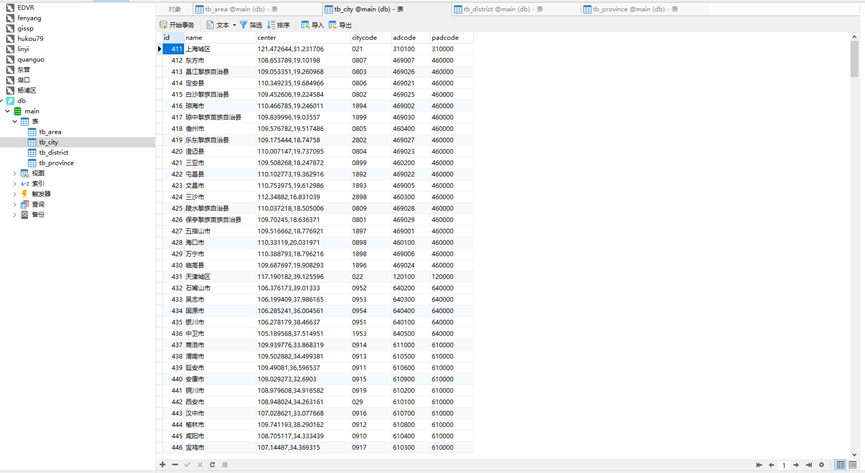
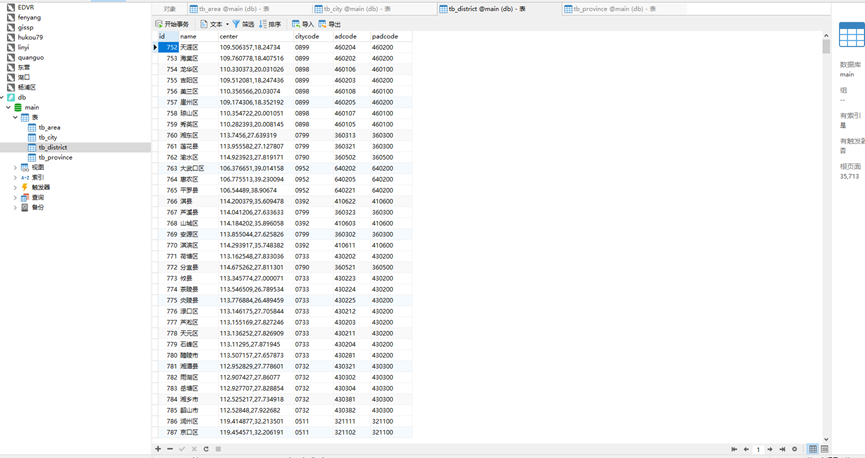
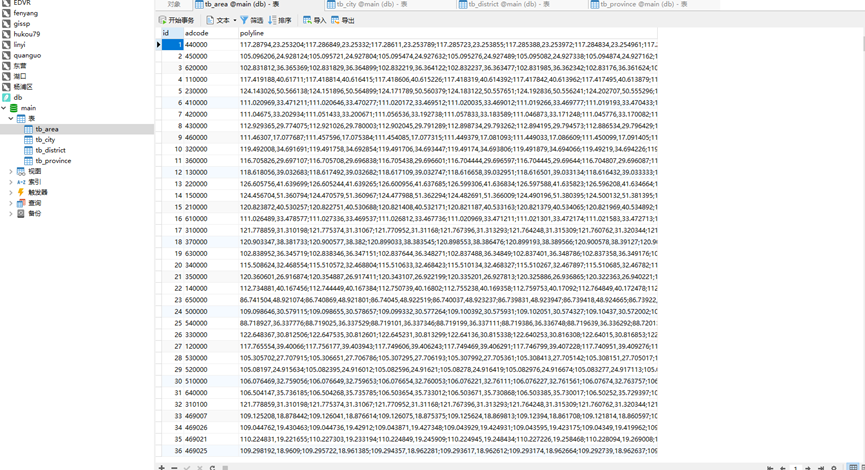
- 背景キーコード構造
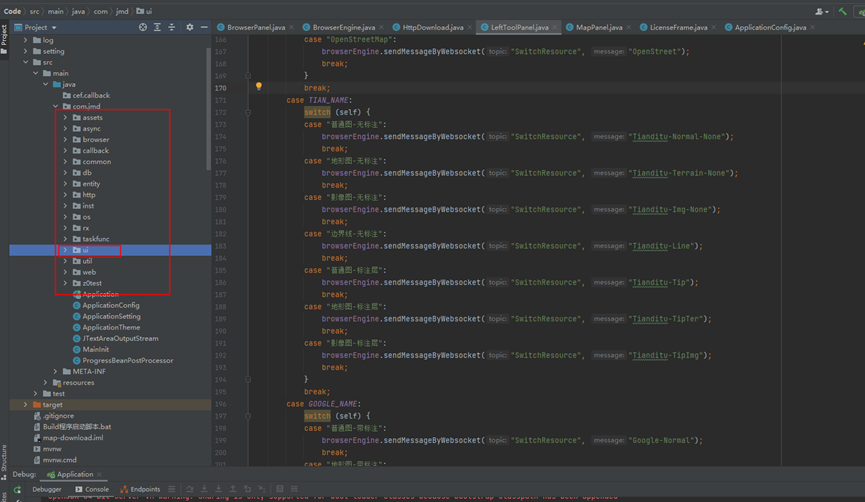
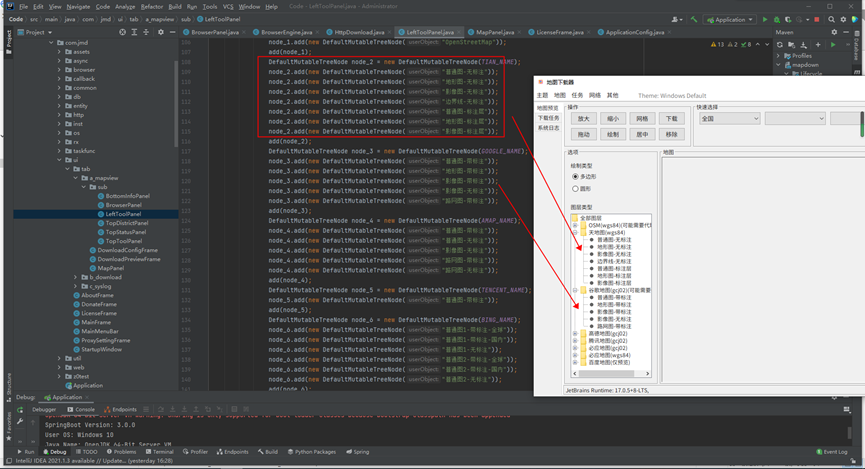
- フロントエンドのキーコード構造
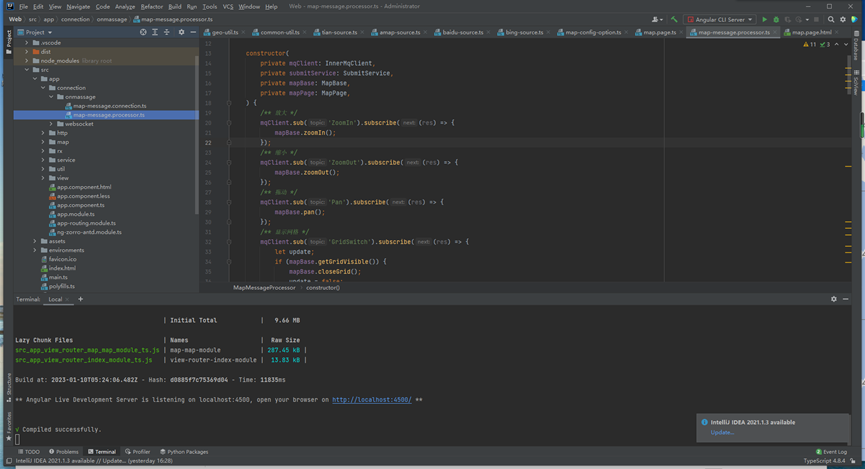
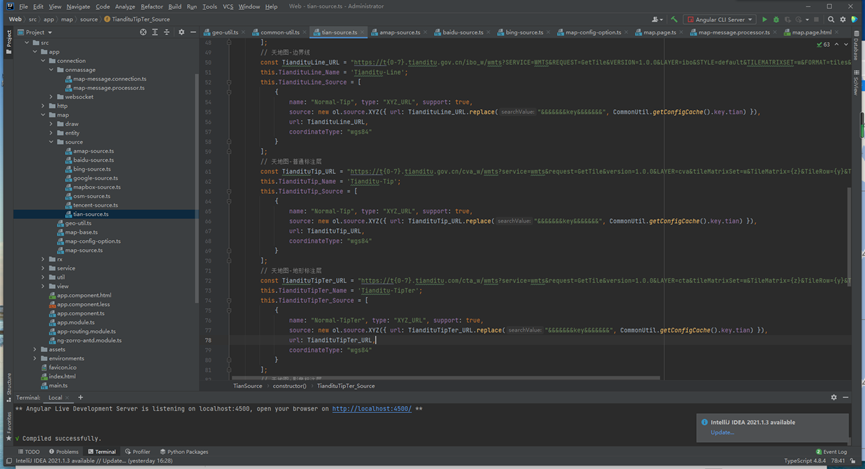
- 機能表示
- スタートページ
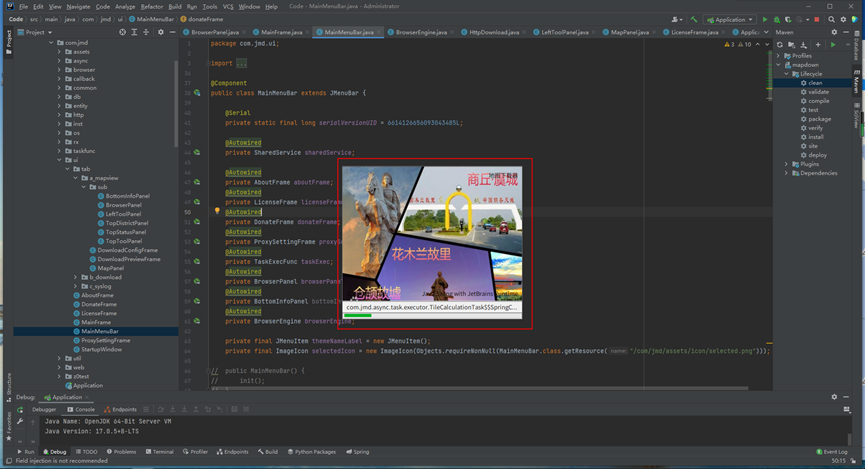
- ベースマップの切り替え
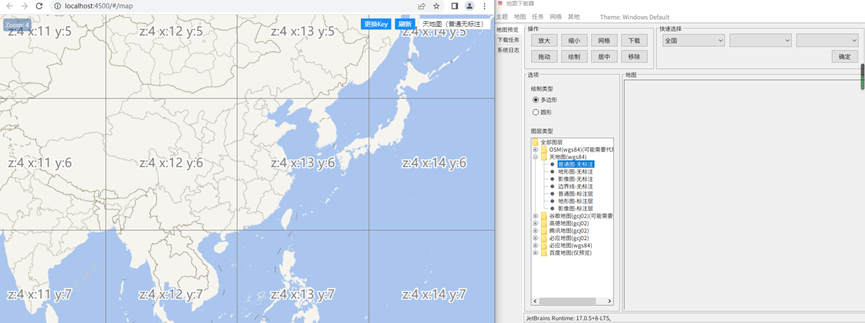
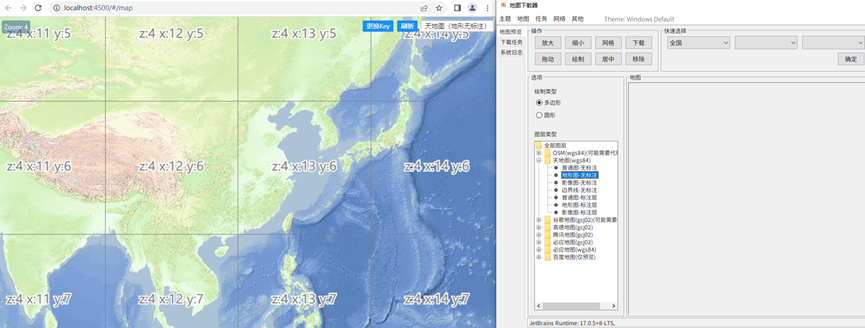
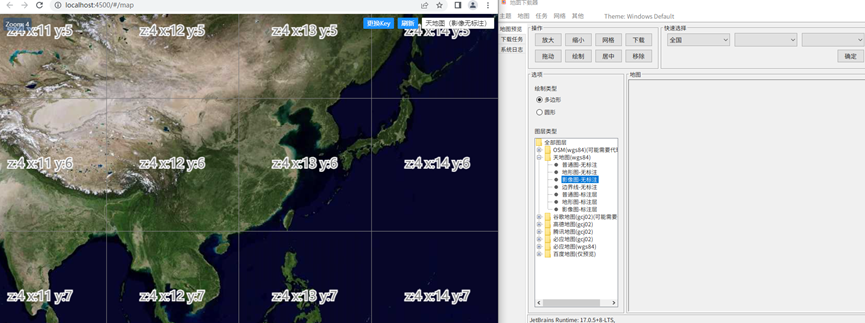
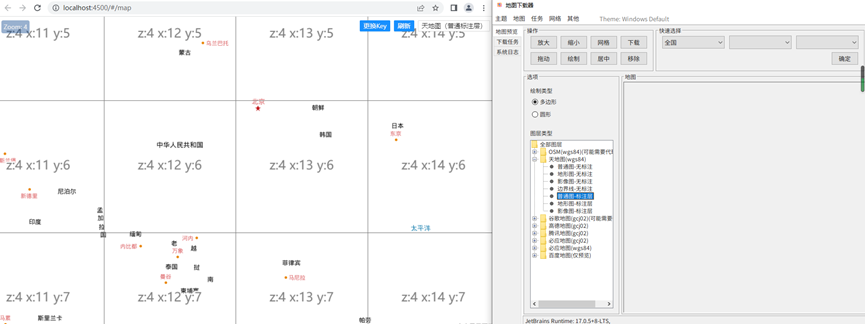
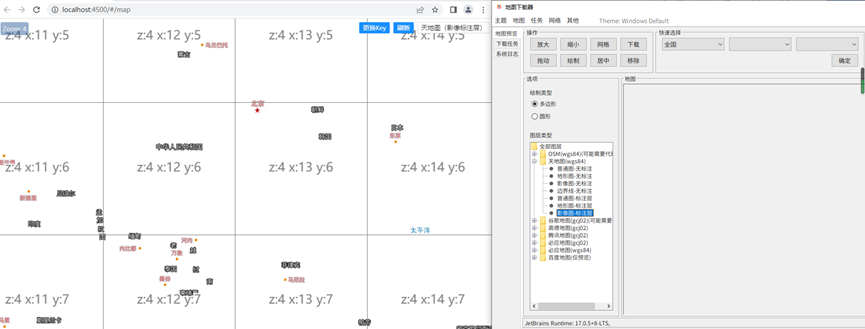
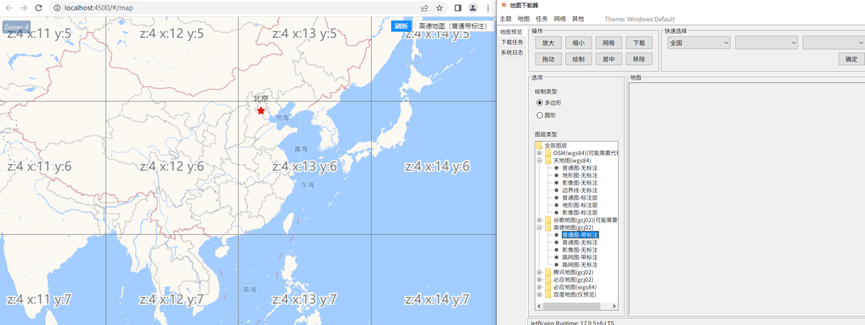
- ダウンロード領域を選択して描画
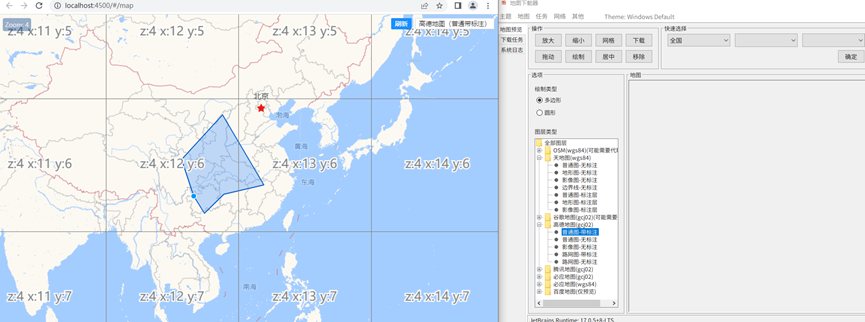
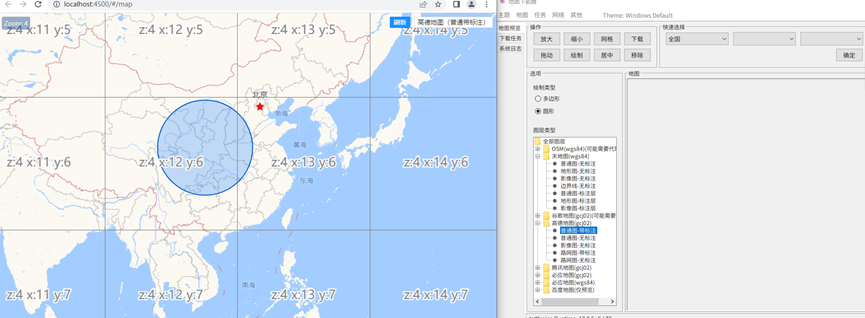
- 行政区分スイッチ選択ダウンロードエリア
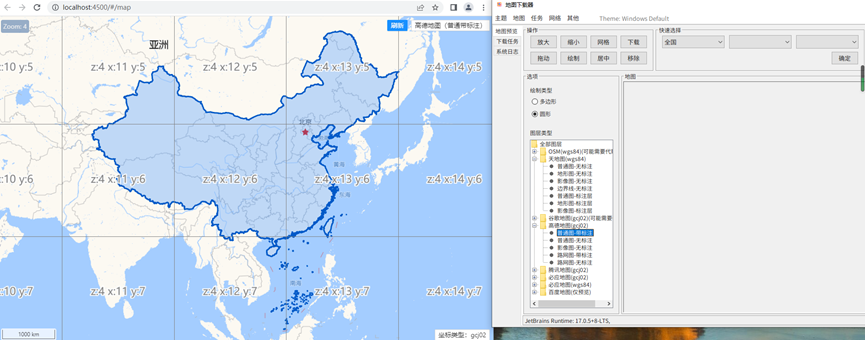
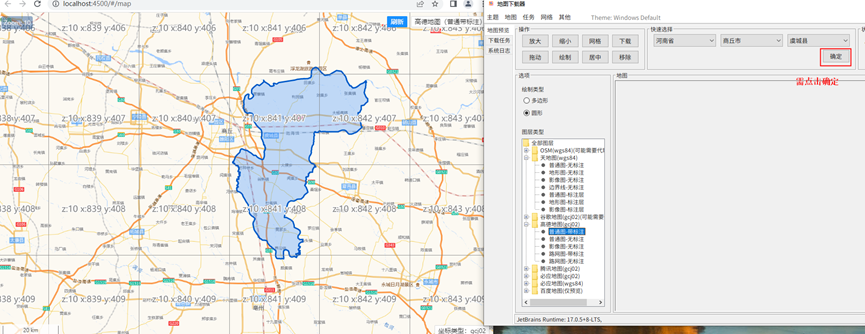
- ダウンロード
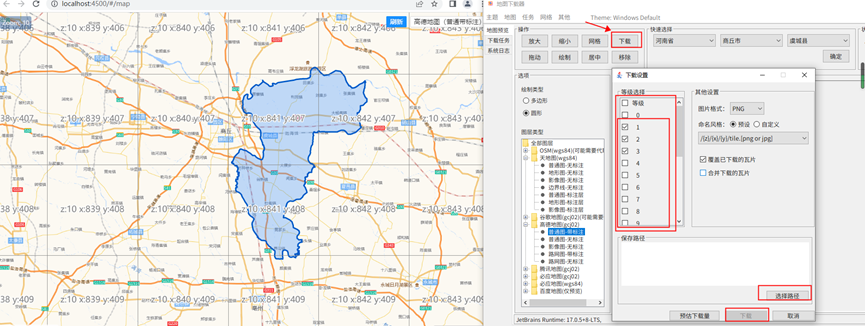
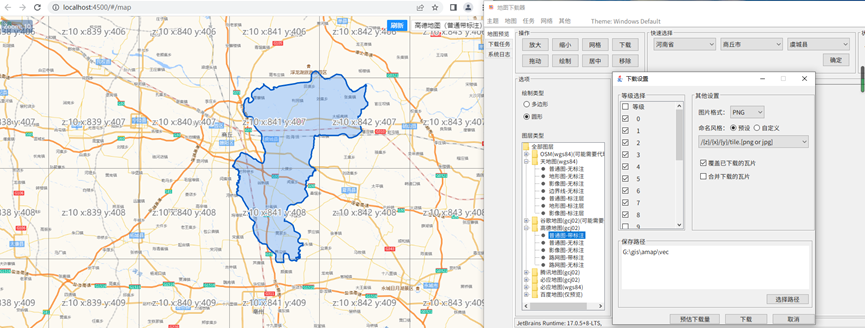
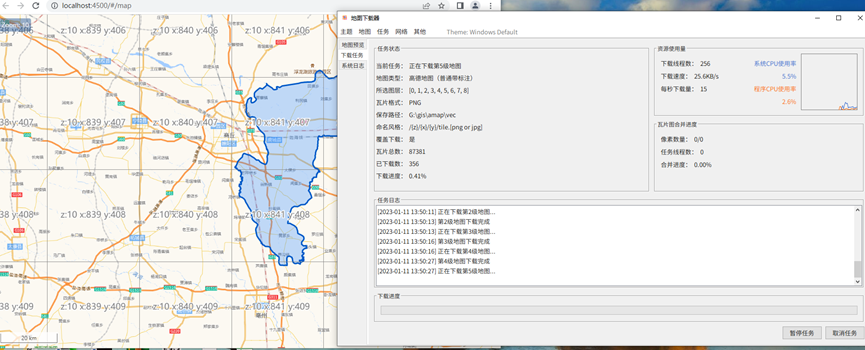
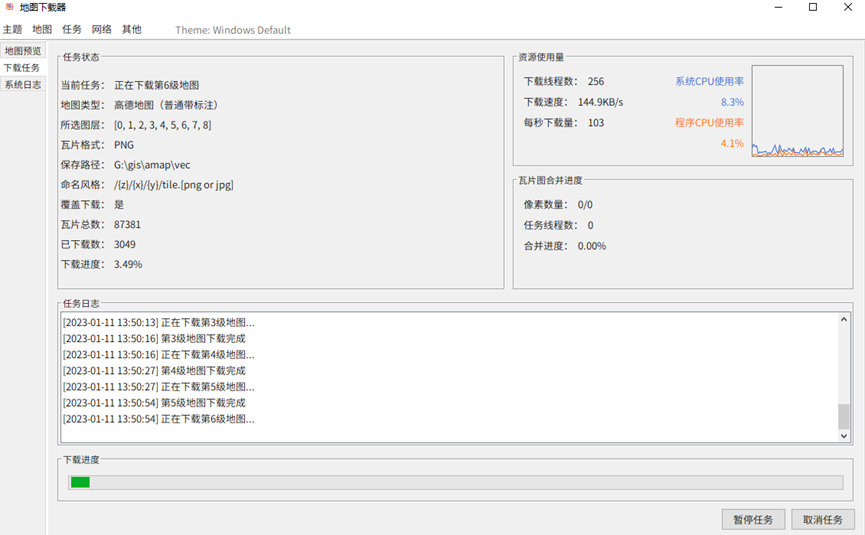
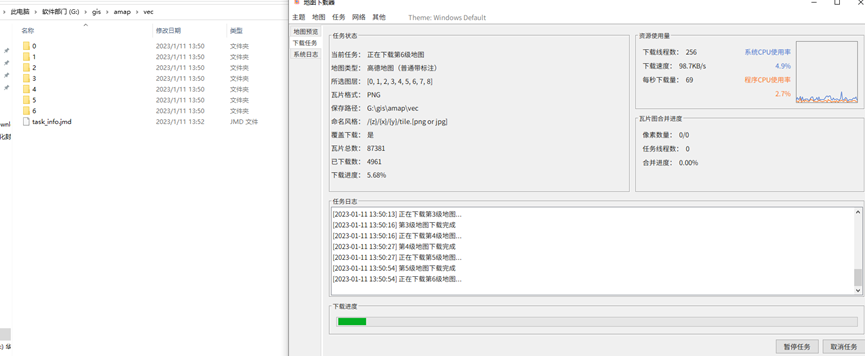
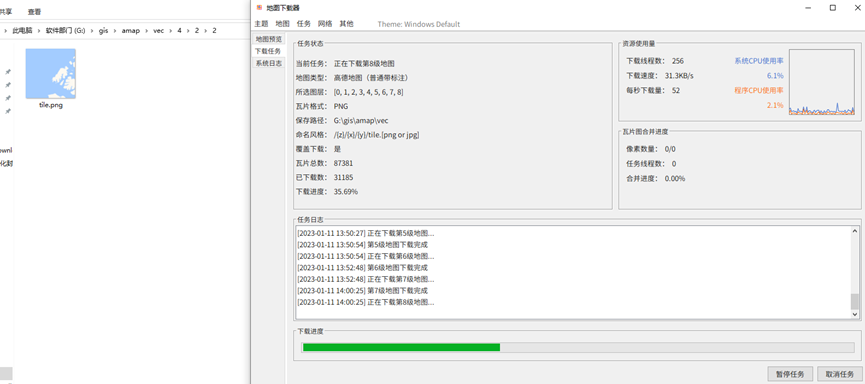
- キーコード
import { InnerMqClient } から '../../rx/inner-mq.service';
import { SubmitService } from '../../service/submit.service';
import { MapBase } から '../../map/map-base';
import { CommonUtil } から '../../util/common-util';
import { MapPage } from '../../view/page/map/map.page';
import { MapDraw } から '../../map/draw/map-draw';
import { MapWrap } から '../../map/draw/map-wrap';
import { GeoUtil } から "../../map/geo-util";
import { Point } from "../../map/entity/Point";
エクスポート クラス MapMessageProcessor {
コンストラクタ(
プライベート mqClient: InnerMqClient、
プライベート submitService: SubmitService、
プライベートマップベース: MapBase、
プライベートマップページ: マップページ、
) {
/** ズームイン */
mqClient.sub('ZoomIn').subscribe((res) => {
mapBase.zoomIn();
});
/** 缩小 */
mqClient.sub('ZoomOut').subscribe((res) => {
mapBase.zoomOut();
});
/** 拖动 */
mqClient.sub('Pan').subscribe((res) => {
mapBase.pan();
});
/** 显示网格 */
mqClient.sub('GridSwitch').subscribe((res) => {
let update;
if (mapBase.getGridVisible()) {
mapBase.closeGrid();
update = false;
} else {
mapBase.showGrid();
update = true;
}
let config = mapBase.getMapConfig();
if (config) {
config.grid = update;
CommonUtil.setConfigCache(config);
mapBase.setMapConfig(config);
}
});
/** 切换图层源 */
mqClient.sub('SwitchResource').subscribe((res) => {
// 切换图层
debugger
let lastType = mapBase.getCurrentCoordinateType();
mapBase.switchMapResource(res);
let currentType = mapBase.getCurrentCoordinateType();
// 保存设置
let config = mapBase.getMapConfig();
if (config) {
config.layer = res;
CommonUtil.setConfigCache(config);
mapBase.setMapConfig(config);
}
// 检查坐标类型
if (lastType != currentType) {
if (lastType == 'wgs84' && currentType == 'gcj02') {
mapBase.turnMapFeaturesFromWgs84ToGcj02();
} else if (lastType == 'gcj02' && currentType == 'wgs84') {
mapBase.turnMapFeaturesFromGcj02ToWgs84();
}
}
// 回调
setTimeout(() => {
mapPage.updateShowInfo();
});
});
/** 绘制类型切换 - */
mqClient.sub('SwitchDrawType').subscribe((res) => {
mapBase.setDrawType(res);
});
/** 绘制 - */
mqClient.sub('OpenDraw').subscribe((res) => {
mapBase.pan();
mapBase.removeDrawedFeatures();
mapBase.openDraw({
drawEnd: () => {
setTimeout(() => {
mapBase.removeDrawInteraction();
})
},
modifyEnd: () => {
}
});
});
/** 绘制指定多边形并定位 - */
mqClient.sub('DrawPolygonAndPositioning').subscribe((res) => {
mapBase.pan();
mapBase.removeDrawedFeatures();
let blocks = JSON.parse(res);
for (let i = 0; i < blocks.length; i++) {
let points: Array<Point> = [];
for (let j = 0; j < blocks[i].length; j++) {
let point = new Point(blocks[i][j].lng, blocks[i][j].lat);
if (mapBase.getCurrentCoordinateType() == 'wgs84') {
points.push(GeoUtil.gcj02_To_wgs84(point));
} else {
points.push(point);
}
}
let feature = MapDraw.createPolygonFeature(points);
MapWrap.addFeature(mapBase, mapBase.drawLayerName, feature);
}
mapBase.setFitviewFromDrawLayer();
});
/** fitview - */
mqClient.sub('Fitview').subscribe((res) => {
mapBase.setFitviewFromDrawLayer();
});
/** 删除绘制 - */
mqClient.sub('RemoveDrawedShape').subscribe((res) => {
mapBase.removeDrawedFeatures();
});
/** 提交区块下载 - */
mqClient.sub('SubmitBlockDownload').subscribe((res) => {
let data = {
tileName: this.mapBase?.getCurrentXyzName(),
mapType: CommonUtil.getMapType(this.mapBase?.getCurrentXyzName()),
tileUrl: this.mapBase?.getCurrentXyzUrlResources(),
points: this.mapBase?.getDrawedPoints(),
};
this.submitService.blockDownload(data).then((r) => {
});
});
/** 提交世界下载 - */
mqClient.sub('SubmitWorldDownload').subscribe((res) => {
let data = {
tileName: this.mapBase?.getCurrentXyzName(),
mapType: CommonUtil.getMapType(this.mapBase?.getCurrentXyzName()),
tileUrl: this.mapBase?.getCurrentXyzUrlResources()
};
this.submitService.worldDownload(data).then((r) => {
});
});
}
}
package com.jmd.http;
import java.io.File;
import java.io.IOException;
import java.util.HashMap;
import org.apache.commons.io.FileUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import com.jmd.entity.result.DownloadResult;
import com.jmd.inst.DownloadAmountInstance;
import com.jmd.util.CommonUtils;
@Component
public class HttpDownload {
@Autowired
private HttpClient http;
@Autowired
private DownloadAmountInstance downloadAmountInstance;
/** 通过URL下载文件 */
public DownloadResult downloadTile(String url, HashMap<String, String> headers, int imgType, String path,
int retry) {
DownloadResult result = new DownloadResult();
boolean success = false;
byte[] bytes = http.getFileBytes(url, headers);
if (null != bytes) {
byte[] imgData = imageSwitch(imgType, bytes);
try {
if (null != imgData) {
// result.setImgData(imgData);
File file = new File(path);
FileUtils.writeByteArrayToFile(file, imgData);
if (file.exists() && file.isFile()) {
downloadAmountInstance.add(file.length());
success = true;
}
}
} catch (IOException e) {
success = false;
e.printStackTrace();
}
}
if (success) {
result.setSuccess(true);
} else if (Thread.currentThread().isInterrupted()) {
result.setSuccess(false);
} else {
retry = retry - 1;
if (retry >= 0) {
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
}
return downloadTile(url, headers, imgType, path, retry);
} else {
result.setSuccess(false);
}
}
return result;
}
/** 下载的图片进行转码 */
private byte[] imageSwitch(int imgType, byte[] imgData) {
if (imgType == 0) {
// 保持PNG
return imgData;
} else if (imgType == 1 || imgType == 2 || imgType == 3) {
// 转换为JPG
float quality = 0.9f;
switch (imgType) {
case 1:
quality = 0.2f;
break;
case 2:
quality = 0.6f;
break;
case 3:
quality = 0.9f;
break;
default:
break;
}
try {
return CommonUtils.png2jpg(imgData, quality);
} catch (IOException e) {
e.printStackTrace();
}
}
return null;
}
}
如果对您有帮助
感谢支持技术分享,请扫码点赞支持:
技术合作交流qq:2401315930
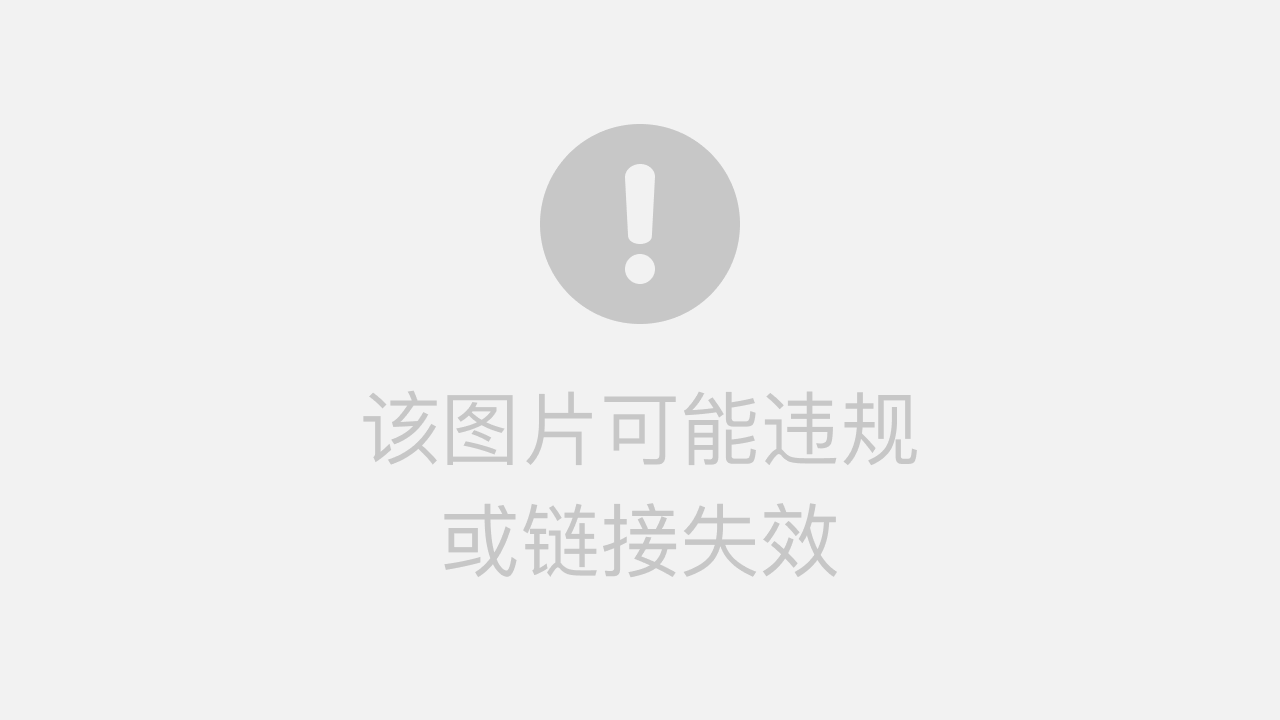
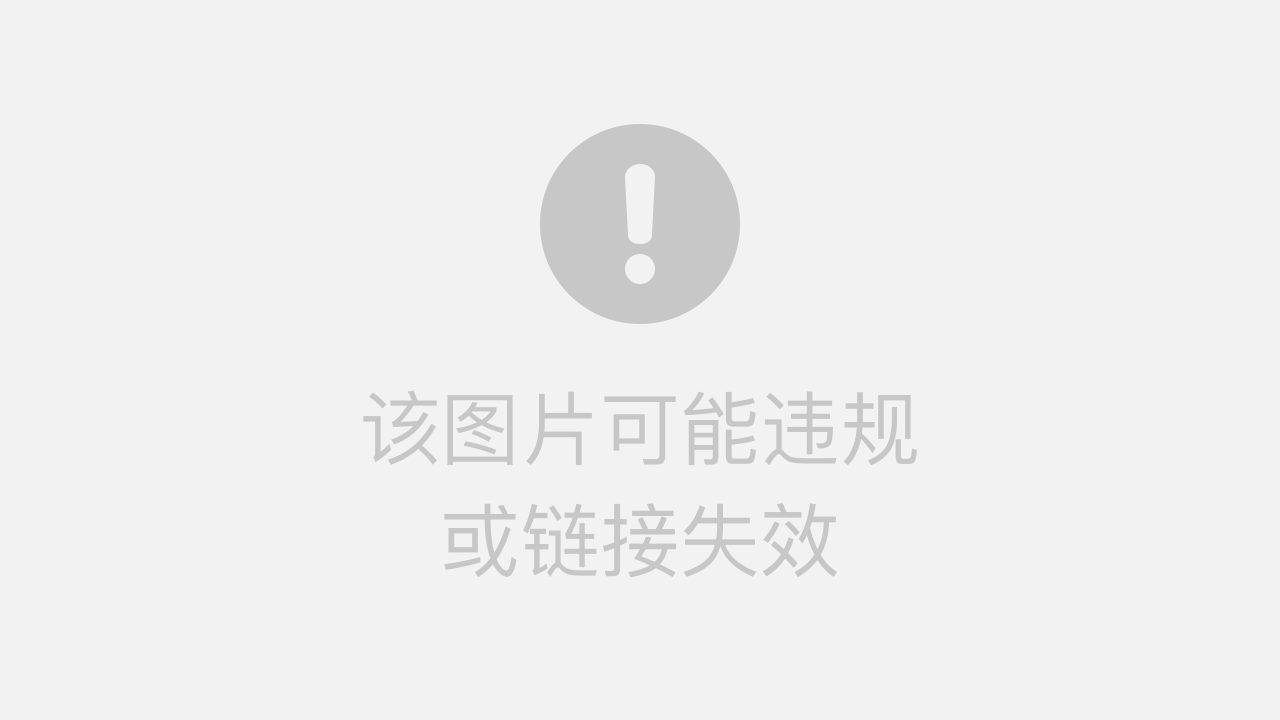
编辑
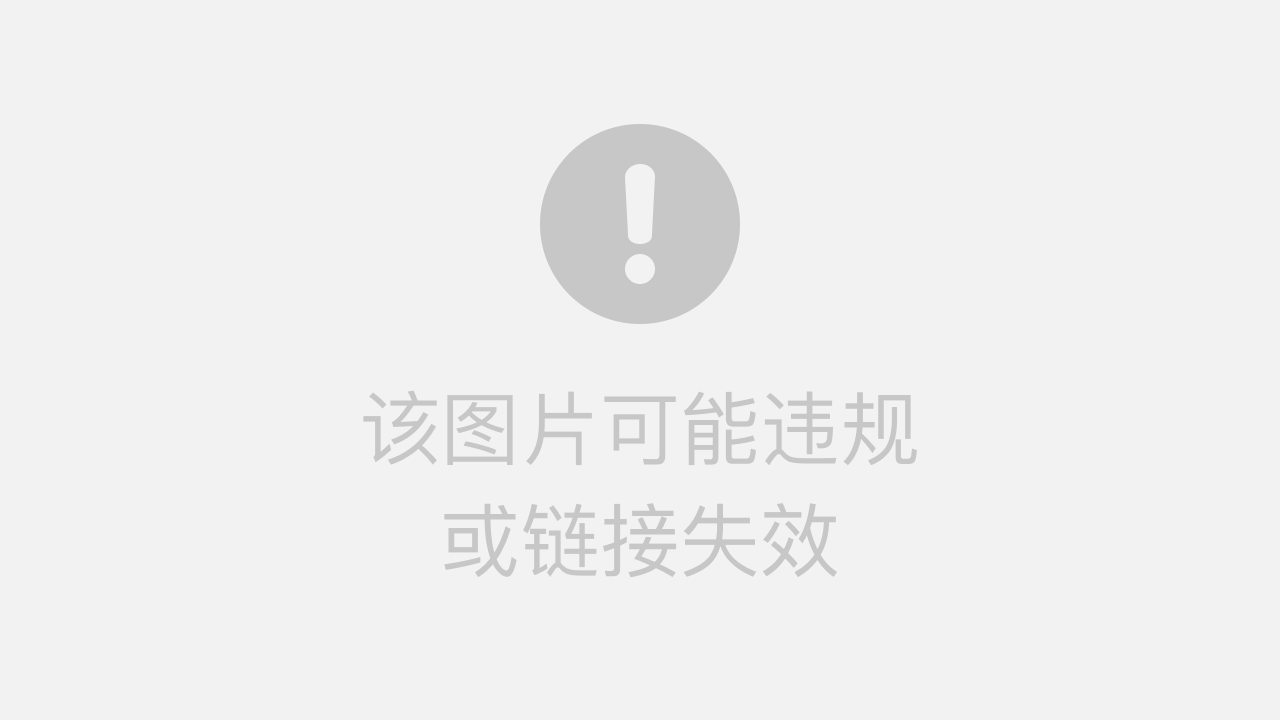
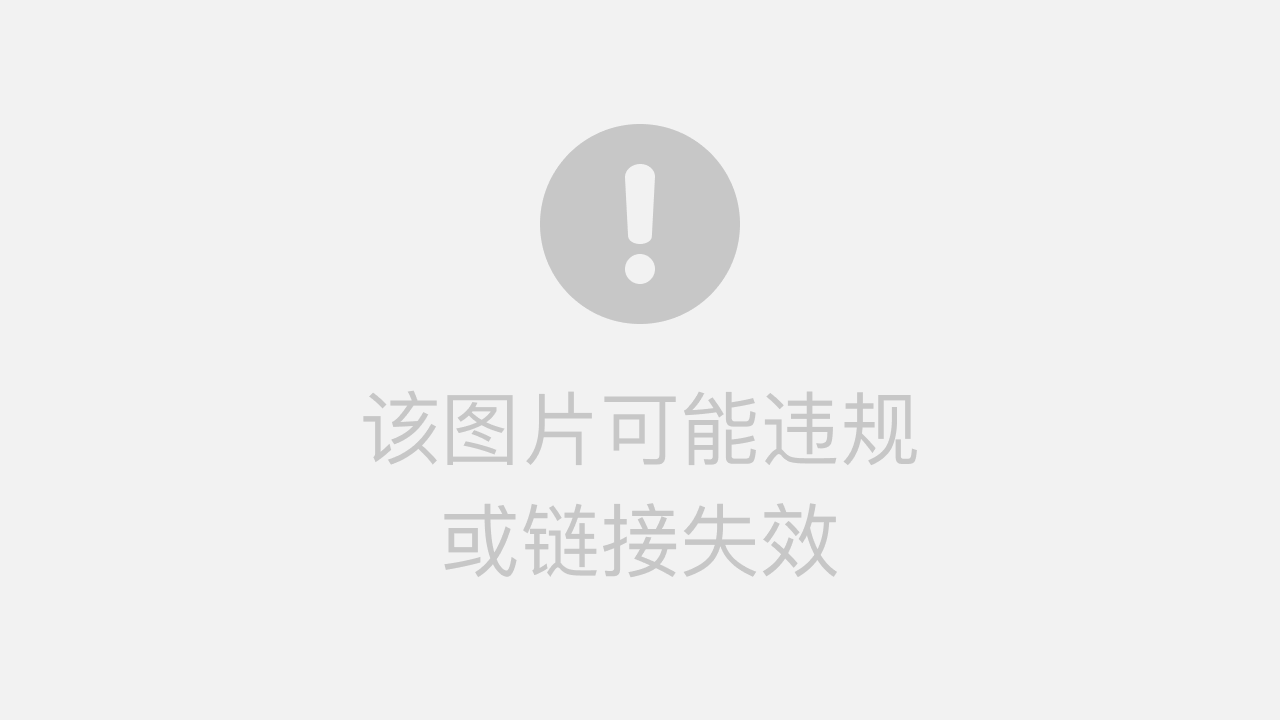
编辑
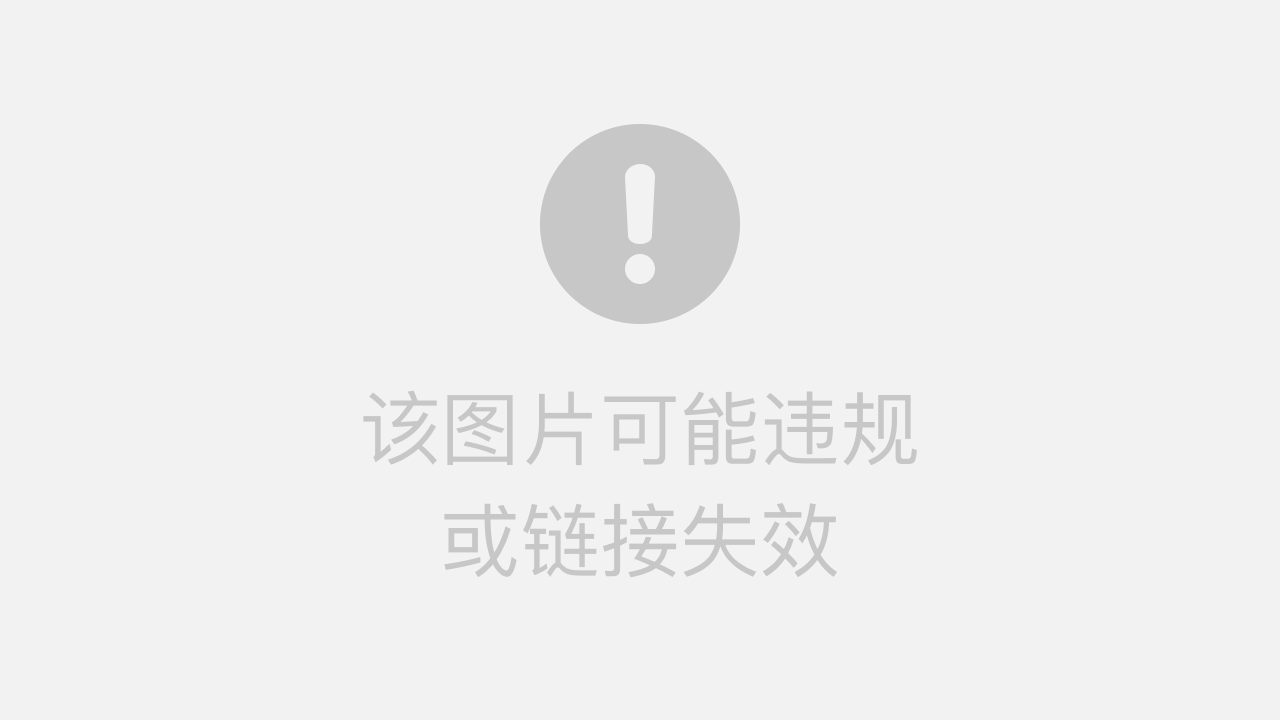
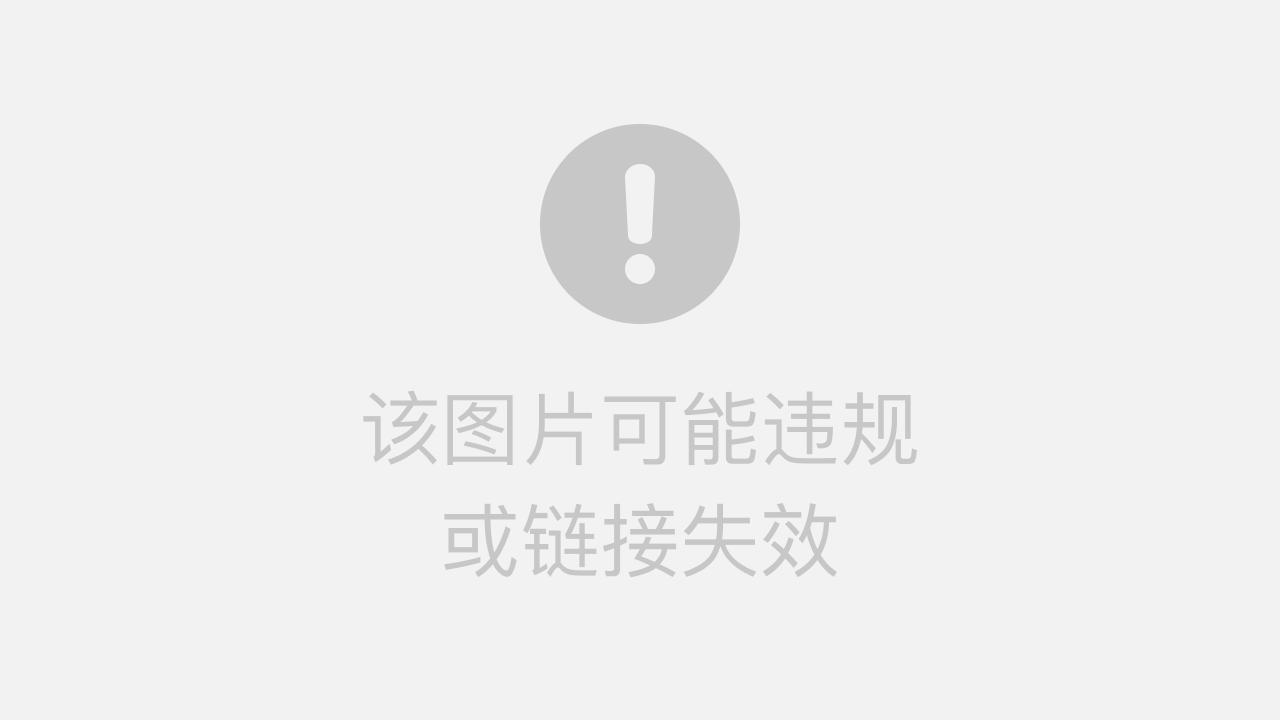
编辑