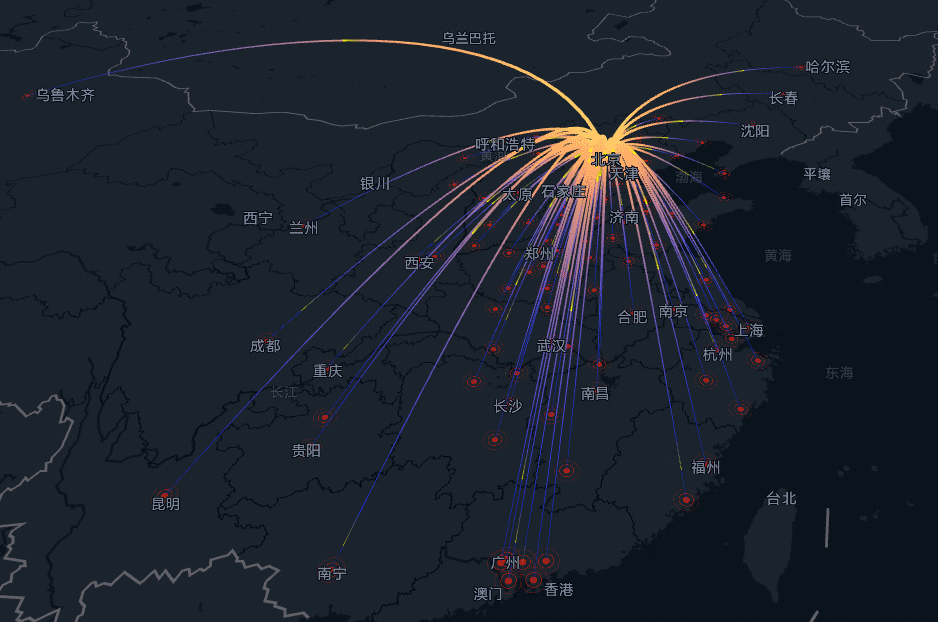
ラジアン フライライン レイヤーの実装には、マップ JS API と LOCA データ視覚化 API の組み合わせが必要です。
概要 - マップ JS API v2.0 | Amap API (amap.com)
概要 - LOCA Data Visualization API 2.0 | Gaode Map API (amap.com)
このレイヤーを初めて導入したとき、LOCA のマニュアルをよく読まなかったため、エラーを報告し続けました。
ラジアンフライングラインレイヤーのデモコードでのAPIの導入方法が違うので、しばらく導入方法が分からず、LOCAサンプルマニュアルをよく読んで理解しました。
北京人口流出フライライン - ラジアン フライライン レイヤー - 例の詳細 - Loca API 2.0 | Gaode Map API (amap.com)
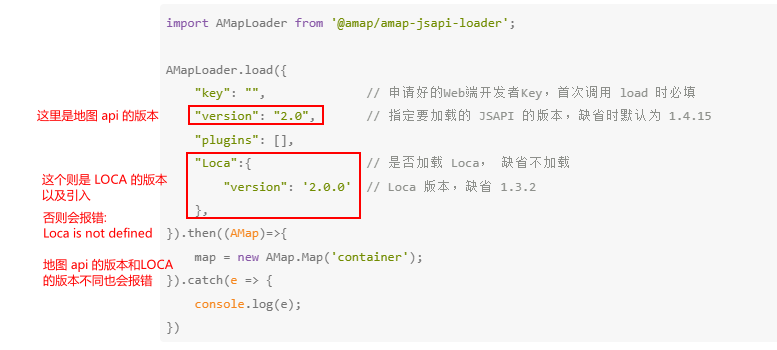
次は完全なコードの説明です。
<template>
<div id="map" style="width: 100%;height: 100%;"></div>
</template>
<script setup>
import AMapLoader from '@amap/amap-jsapi-loader';
function initMap() {
window._AMapSecurityConfig = {
securityJsCode: '8e920f73eb2e6880a92ea6662eefc476',
}
AMapLoader.load({
key:"e4e3d44a98350790a1493450032bbec5", // 申请好的Web端开发者Key,首次调用 load 时必填
/*
* 刚开始没有引入下面代码时,会报错: Loca is not defined
* Loca: {
version: '2.0.0' // Loca 版本,缺省 1.3.2
},
而且 JSAPI的version(版本)要和Loca的version(版本)一致才可以,否则也会报错
不懂的可以去看官方文档: https://lbs.amap.com/api/loca-v2/intro
* */
version:"2.0", // 指定要加载的 JSAPI 的版本,缺省时默认为 1.4.15
Loca: {
version: '2.0.0' // Loca 版本,缺省 1.3.2
},
plugins:[], // 需要使用的的插件列表,如比例尺'AMap.Scale'等
}).then((AMap) => {
const map = new AMap.Map("map", {
resizeEnable: true, // 是否监控地图容器尺寸变化
viewMode: '3D',
pitch: 48,// 地图俯仰角度,有效范围 0 度- 83 度
center:[104.780269, 34.955403], // 地图中心点
zoom:5, // 地图显示的缩放级别
mapStyle:"amap://styles/grey", // 设置地图的显示样式
});
// Container: Loca 的核心控制类,可以控制光源、视角变换、图层渲染等。
var loca = new Loca.Container({ // 创建一个容器
map, // 传入地图实例 还可以这样写: map: map (ES6语法)
});
// 呼吸点
// ScatterLayer: 带有动画效果的图层类型,用于展示散点数据,支持动画效果,如呼吸点、闪烁点等。大地面上的点,可展示三种类型:颜色圆、图标、动画图标
var scatter = new Loca.ScatterLayer({ // 创建一个散点图层
loca, // 传入容器实例 (相当于把散点图层添加到地图上)
zIndex: 10, // 图层层级
opacity: 0.6, // 图层透明度
visible: true, // 图层是否可见
zooms: [2, 22], // 图层显示的缩放级别范围
});
/*
* geojson 格式的数据源,一个数据源可以给多个图层同时提供数据。一个geojson数据源可以同时拥有点、线、面的数据类型,每个图层绘制的时候会自动获取 合适的数据类型进行渲染
* */
var pointGeo = new Loca.GeoJSONSource({ // 创建一个 GeoJSON 对象
url: 'https://a.amap.com/Loca/static/loca-v2/demos/mock_data/pulselink-china-city-point.json', // 数据源的链接地址,一般是接口地址,返回的数据必须是 geojson 格式
});
scatter.setSource(pointGeo); // 给上面创建的散点图层设置数据源
scatter.setStyle({ // 设置样式
unit: 'meter', // 设置单位为米
size: [100000, 100000], // 设置大小范围
borderWidth: 0, // 设置边框宽度
texture: 'https://a.amap.com/Loca/static/loca-v2/demos/images/breath_red.png', // 设置纹理
duration: 2000, // 设置动画时长
animate: true, // 设置动画
});
loca.add(scatter); // 将散点图层(scatter)添加到容器中
// 弧线
// PulseLinkLayer: 连接飞线图层,可以支持弧度,宽度,过渡色等能力。 并且还支持脉冲动画,可以表达数据的朝向
var pulseLink = new Loca.PulseLinkLayer({ // 创建一个弧线图层
// loca,
zIndex: 10, // 设置图层的层级
opacity: 1, // 设置图层的透明度
visible: true, // 设置图层是否可见
zooms: [2, 22], // 设置图层可见的缩放级别范围
depth: true, // 设置是否开启深度检测
});
// GeoJSONSource: 图层的数据源,可以是本地数据,也可以是远程数据,数据格式必须是 geojson 格式
var geo = new Loca.GeoJSONSource({ // 创建一个 GeoJSON 对象
url: 'https://a.amap.com/Loca/static/loca-v2/demos/mock_data/data-line-out.json', // 数据源的链接地址,一般是接口地址,返回的数据必须是 geojson 格式
});
pulseLink.setSource(geo); // 给上面的弧线图层设置数据源
pulseLink.setStyle({ // 设置样式
unit: 'meter', // 设置单位为米
dash: [40000, 0, 40000, 0], // 设置虚线样式
lineWidth: function () { // 设置线宽
return [20000, 1000]; // 设置线宽范围
},
height: function (index, feat) { // 设置高度
return feat.distance / 3 + 10; // 设置高度范围
},
altitude: 1000, // 设置高度
smoothSteps: 30, // 设置平滑度
speed: function (index, prop) { // 设置速度
return 1000 + Math.random() * 200000; // 设置速度范围
},
flowLength: 100000, // 设置流动长度
lineColors: function (index, feat) { // 设置线颜色
return ['rgb(255,228,105)', 'rgb(255,164,105)', 'rgba(1, 34, 249,1)']; // 设置线颜色范围
},
maxHeightScale: 0.3, // 弧顶位置比例
headColor: 'rgba(255, 255, 0, 1)', // 设置头部颜色
trailColor: 'rgba(255, 255,0,0)', // 设置尾部颜色
});
loca.add(pulseLink); // 将图层添加到容器中
loca.animate.start(); // 开始动画
// 点击事件处理
var clickInfo = new AMap.Marker({ // 点标记
anchor: 'bottom-center', // 设置点标记锚点,可选值:'top-left','top-center','top-right'等详细请看官方文档: https://lbs.amap.com/api/jsapi-v2/documentation#marker
position: [116.396923, 39.918203, 0], // 点标记在地图上显示的位置
});
clickInfo.setMap(map); // 将点标记添加到地图上
clickInfo.hide(); // 隐藏点标记
map.on("click", function (e) {
var feat = pulseLink.queryFeature(e.pixel.toArray());
if (feat) {
clickInfo.show();
var props = feat.properties;
clickInfo.setPosition(feat.coordinates[1]);
clickInfo.setContent(
'<div style="text-align: center; height: 20px; width: 150px; color:#fff; font-size: 14px;">' +
'速率: ' + feat.properties['ratio'] +
'</div>'
);
} else {
clickInfo.hide();
}
});
/*
以下为:
样式调试工具,仅用于开发阶段方便调试样式
可以将可视化图层添加到调试工具中使用
请勿在生产环境中使用
* */
/*var dat = new Loca.Dat(); // 创建一个 Dat 对象
dat.addLayer(pulseLink); // 将图层添加到 Dat 对象中*/
});
}
initMap();
</script>
https://a.amap.com/Loca/static/loca-v2/demos/mock_data/pulselink-china-city-point.jsonのデータ:
{
"type": "FeatureCollection",
"features": [
{
"type": "Feature",
"properties": {
"type": 0,
"ratio": 0.0369,
"lineWidthRatio": 1
},
"geometry": {
"type": "Point",
"coordinates": [
115.482331,
38.867657
]
}
},
{
"type": "Feature",
"properties": {
"type": 1,
"ratio": 0.035,
"lineWidthRatio": 0.9447674418604651
},
"geometry": {
"type": "Point",
"coordinates": [
117.190182,
39.125596
]
}
},
{
"type": "Feature",
"properties": {
"type": 2,
"ratio": 0.0189,
"lineWidthRatio": 0.47674418604651164
},
"geometry": {
"type": "Point",
"coordinates": [
121.472644,
31.231706
]
}
},
{
"type": "Feature",
"properties": {
"type": 3,
"ratio": 0.0167,
"lineWidthRatio": 0.41279069767441856
},
"geometry": {
"type": "Point",
"coordinates": [
113.280637,
23.125178
]
}
},
{
"type": "Feature",
"properties": {
"type": 4,
"ratio": 0.0148,
"lineWidthRatio": 0.35755813953488375
},
"geometry": {
"type": "Point",
"coordinates": [
106.504962,
29.533155
]
}
},
{
"type": "Feature",
"properties": {
"type": 5,
"ratio": 0.0147,
"lineWidthRatio": 0.35465116279069764
},
"geometry": {
"type": "Point",
"coordinates": [
114.884091,
40.811901
]
}
},
{
"type": "Feature",
"properties": {
"type": 6,
"ratio": 0.0137,
"lineWidthRatio": 0.32558139534883723
},
"geometry": {
"type": "Point",
"coordinates": [
113.746262,
23.046237
]
}
},
{
"type": "Feature",
"properties": {
"type": 7,
"ratio": 0.0136,
"lineWidthRatio": 0.3226744186046511
},
"geometry": {
"type": "Point",
"coordinates": [
104.065735,
30.659462
]
}
},
{
"type": "Feature",
"properties": {
"type": 0,
"ratio": 0.0134,
"lineWidthRatio": 0.3168604651162791
},
"geometry": {
"type": "Point",
"coordinates": [
114.502461,
38.045474
]
}
},
{
"type": "Feature",
"properties": {
"type": 1,
"ratio": 0.0116,
"lineWidthRatio": 0.2645348837209302
},
"geometry": {
"type": "Point",
"coordinates": [
118.175393,
39.635113
]
}
},
{
"type": "Feature",
"properties": {
"type": 2,
"ratio": 0.011,
"lineWidthRatio": 0.24709302325581392
},
"geometry": {
"type": "Point",
"coordinates": [
118.767413,
32.041544
]
}
},
{
"type": "Feature",
"properties": {
"type": 3,
"ratio": 0.0108,
"lineWidthRatio": 0.24127906976744187
},
"geometry": {
"type": "Point",
"coordinates": [
120.153576,
30.287459
]
}
},
{
"type": "Feature",
"properties": {
"type": 4,
"ratio": 0.0101,
"lineWidthRatio": 0.2209302325581395
},
"geometry": {
"type": "Point",
"coordinates": [
116.857461,
38.310582
]
}
},
{
"type": "Feature",
"properties": {
"type": 5,
"ratio": 0.0097,
"lineWidthRatio": 0.20930232558139533
},
"geometry": {
"type": "Point",
"coordinates": [
117.939152,
40.976204
]
}
},
{
"type": "Feature",
"properties": {
"type": 6,
"ratio": 0.0087,
"lineWidthRatio": 0.18023255813953484
},
"geometry": {
"type": "Point",
"coordinates": [
114.490686,
36.612273
]
}
},
{
"type": "Feature",
"properties": {
"type": 7,
"ratio": 0.0081,
"lineWidthRatio": 0.1627906976744186
},
"geometry": {
"type": "Point",
"coordinates": [
113.665412,
34.757975
]
}
},
{
"type": "Feature",
"properties": {
"type": 0,
"ratio": 0.008,
"lineWidthRatio": 0.15988372093023254
},
"geometry": {
"type": "Point",
"coordinates": [
114.085947,
22.547
]
}
},
{
"type": "Feature",
"properties": {
"type": 1,
"ratio": 0.0077,
"lineWidthRatio": 0.1511627906976744
},
"geometry": {
"type": "Point",
"coordinates": [
120.619585,
31.299379
]
}
},
{
"type": "Feature",
"properties": {
"type": 2,
"ratio": 0.0074,
"lineWidthRatio": 0.14244186046511628
},
"geometry": {
"type": "Point",
"coordinates": [
108.948024,
34.263161
]
}
},
{
"type": "Feature",
"properties": {
"type": 3,
"ratio": 0.0074,
"lineWidthRatio": 0.14244186046511628
},
"geometry": {
"type": "Point",
"coordinates": [
117.000923,
36.675807
]
}
},
{
"type": "Feature",
"properties": {
"type": 4,
"ratio": 0.0071,
"lineWidthRatio": 0.13372093023255813
},
"geometry": {
"type": "Point",
"coordinates": [
114.508851,
37.0682
]
}
},
{
"type": "Feature",
"properties": {
"type": 5,
"ratio": 0.0063,
"lineWidthRatio": 0.11046511627906977
},
"geometry": {
"type": "Point",
"coordinates": [
115.665993,
37.735097
]
}
},
{
"type": "Feature",
"properties": {
"type": 6,
"ratio": 0.006,
"lineWidthRatio": 0.10174418604651163
},
"geometry": {
"type": "Point",
"coordinates": [
120.355173,
36.082982
]
}
},
{
"type": "Feature",
"properties": {
"type": 7,
"ratio": 0.0055,
"lineWidthRatio": 0.08720930232558138
},
"geometry": {
"type": "Point",
"coordinates": [
116.307428,
37.453968
]
}
},
{
"type": "Feature",
"properties": {
"type": 0,
"ratio": 0.0055,
"lineWidthRatio": 0.08720930232558138
},
"geometry": {
"type": "Point",
"coordinates": [
112.982279,
28.19409
]
}
},
{
"type": "Feature",
"properties": {
"type": 1,
"ratio": 0.0054,
"lineWidthRatio": 0.08430232558139536
},
"geometry": {
"type": "Point",
"coordinates": [
115.469381,
35.246531
]
}
},
{
"type": "Feature",
"properties": {
"type": 2,
"ratio": 0.0053,
"lineWidthRatio": 0.08139534883720931
},
"geometry": {
"type": "Point",
"coordinates": [
119.107078,
36.70925
]
}
},
{
"type": "Feature",
"properties": {
"type": 3,
"ratio": 0.0051,
"lineWidthRatio": 0.07558139534883722
},
"geometry": {
"type": "Point",
"coordinates": [
123.429096,
41.796767
]
}
},
{
"type": "Feature",
"properties": {
"type": 4,
"ratio": 0.0049,
"lineWidthRatio": 0.06976744186046512
},
"geometry": {
"type": "Point",
"coordinates": [
117.184811,
34.261792
]
}
},
{
"type": "Feature",
"properties": {
"type": 5,
"ratio": 0.0049,
"lineWidthRatio": 0.06976744186046512
},
"geometry": {
"type": "Point",
"coordinates": [
112.549248,
37.857014
]
}
},
{
"type": "Feature",
"properties": {
"type": 6,
"ratio": 0.0048,
"lineWidthRatio": 0.06686046511627905
},
"geometry": {
"type": "Point",
"coordinates": [
113.122717,
23.028762
]
}
},
{
"type": "Feature",
"properties": {
"type": 7,
"ratio": 0.0048,
"lineWidthRatio": 0.06686046511627905
},
"geometry": {
"type": "Point",
"coordinates": [
112.540918,
32.999082
]
}
},
{
"type": "Feature",
"properties": {
"type": 0,
"ratio": 0.0048,
"lineWidthRatio": 0.06686046511627905
},
"geometry": {
"type": "Point",
"coordinates": [
114.649653,
33.620357
]
}
},
{
"type": "Feature",
"properties": {
"type": 1,
"ratio": 0.0047,
"lineWidthRatio": 0.06395348837209303
},
"geometry": {
"type": "Point",
"coordinates": [
118.326443,
35.065282
]
}
},
{
"type": "Feature",
"properties": {
"type": 2,
"ratio": 0.0047,
"lineWidthRatio": 0.06395348837209303
},
"geometry": {
"type": "Point",
"coordinates": [
120.301663,
31.574729
]
}
},
{
"type": "Feature",
"properties": {
"type": 3,
"ratio": 0.0047,
"lineWidthRatio": 0.06395348837209303
},
"geometry": {
"type": "Point",
"coordinates": [
117.283042,
31.86119
]
}
},
{
"type": "Feature",
"properties": {
"type": 4,
"ratio": 0.0046,
"lineWidthRatio": 0.061046511627906974
},
"geometry": {
"type": "Point",
"coordinates": [
119.586579,
39.942531
]
}
},
{
"type": "Feature",
"properties": {
"type": 5,
"ratio": 0.0044,
"lineWidthRatio": 0.05523255813953489
},
"geometry": {
"type": "Point",
"coordinates": [
112.434468,
34.663041
]
}
},
{
"type": "Feature",
"properties": {
"type": 6,
"ratio": 0.0044,
"lineWidthRatio": 0.05523255813953489
},
"geometry": {
"type": "Point",
"coordinates": [
115.980367,
36.456013
]
}
},
{
"type": "Feature",
"properties": {
"type": 7,
"ratio": 0.0044,
"lineWidthRatio": 0.05523255813953489
},
"geometry": {
"type": "Point",
"coordinates": [
126.642464,
45.756967
]
}
},
{
"type": "Feature",
"properties": {
"type": 0,
"ratio": 0.0044,
"lineWidthRatio": 0.05523255813953489
},
"geometry": {
"type": "Point",
"coordinates": [
121.549792,
29.868388
]
}
},
{
"type": "Feature",
"properties": {
"type": 1,
"ratio": 0.0043,
"lineWidthRatio": 0.05232558139534883
},
"geometry": {
"type": "Point",
"coordinates": [
113.883991,
35.302616
]
}
},
{
"type": "Feature",
"properties": {
"type": 2,
"ratio": 0.0043,
"lineWidthRatio": 0.05232558139534883
},
"geometry": {
"type": "Point",
"coordinates": [
113.295259,
40.09031
]
}
},
{
"type": "Feature",
"properties": {
"type": 3,
"ratio": 0.0042,
"lineWidthRatio": 0.04941860465116278
},
"geometry": {
"type": "Point",
"coordinates": [
102.712251,
25.040609
]
}
},
{
"type": "Feature",
"properties": {
"type": 4,
"ratio": 0.0042,
"lineWidthRatio": 0.04941860465116278
},
"geometry": {
"type": "Point",
"coordinates": [
116.587245,
35.415393
]
}
},
{
"type": "Feature",
"properties": {
"type": 5,
"ratio": 0.0041,
"lineWidthRatio": 0.04651162790697675
},
"geometry": {
"type": "Point",
"coordinates": [
114.352482,
36.103442
]
}
},
{
"type": "Feature",
"properties": {
"type": 6,
"ratio": 0.004,
"lineWidthRatio": 0.0436046511627907
},
"geometry": {
"type": "Point",
"coordinates": [
114.075031,
32.123274
]
}
},
{
"type": "Feature",
"properties": {
"type": 7,
"ratio": 0.004,
"lineWidthRatio": 0.0436046511627907
},
"geometry": {
"type": "Point",
"coordinates": [
114.024736,
32.980169
]
}
},
{
"type": "Feature",
"properties": {
"type": 0,
"ratio": 0.004,
"lineWidthRatio": 0.0436046511627907
},
"geometry": {
"type": "Point",
"coordinates": [
114.298572,
30.584355
]
}
},
{
"type": "Feature",
"properties": {
"type": 1,
"ratio": 0.0039,
"lineWidthRatio": 0.04069767441860465
},
"geometry": {
"type": "Point",
"coordinates": [
115.650497,
34.437054
]
}
},
{
"type": "Feature",
"properties": {
"type": 2,
"ratio": 0.0039,
"lineWidthRatio": 0.04069767441860465
},
"geometry": {
"type": "Point",
"coordinates": [
114.940278,
25.85097
]
}
},
{
"type": "Feature",
"properties": {
"type": 3,
"ratio": 0.0038,
"lineWidthRatio": 0.0377906976744186
},
"geometry": {
"type": "Point",
"coordinates": [
125.3245,
43.886841
]
}
},
{
"type": "Feature",
"properties": {
"type": 4,
"ratio": 0.0037,
"lineWidthRatio": 0.034883720930232565
},
"geometry": {
"type": "Point",
"coordinates": [
111.670801,
40.818311
]
}
},
{
"type": "Feature",
"properties": {
"type": 5,
"ratio": 0.0036,
"lineWidthRatio": 0.031976744186046506
},
"geometry": {
"type": "Point",
"coordinates": [
115.819729,
32.896969
]
}
},
{
"type": "Feature",
"properties": {
"type": 6,
"ratio": 0.0035,
"lineWidthRatio": 0.029069767441860465
},
"geometry": {
"type": "Point",
"coordinates": [
111.517973,
36.08415
]
}
},
{
"type": "Feature",
"properties": {
"type": 7,
"ratio": 0.0035,
"lineWidthRatio": 0.029069767441860465
},
"geometry": {
"type": "Point",
"coordinates": [
120.864608,
32.016212
]
}
},
{
"type": "Feature",
"properties": {
"type": 0,
"ratio": 0.0035,
"lineWidthRatio": 0.029069767441860465
},
"geometry": {
"type": "Point",
"coordinates": [
118.956806,
42.275317
]
}
},
{
"type": "Feature",
"properties": {
"type": 1,
"ratio": 0.0035,
"lineWidthRatio": 0.029069767441860465
},
"geometry": {
"type": "Point",
"coordinates": [
112.736465,
37.696495
]
}
},
{
"type": "Feature",
"properties": {
"type": 2,
"ratio": 0.0034,
"lineWidthRatio": 0.026162790697674413
},
"geometry": {
"type": "Point",
"coordinates": [
111.003957,
35.022778
]
}
},
{
"type": "Feature",
"properties": {
"type": 3,
"ratio": 0.0033,
"lineWidthRatio": 0.023255813953488372
},
"geometry": {
"type": "Point",
"coordinates": [
118.016974,
37.383542
]
}
},
{
"type": "Feature",
"properties": {
"type": 4,
"ratio": 0.0033,
"lineWidthRatio": 0.023255813953488372
},
"geometry": {
"type": "Point",
"coordinates": [
121.391382,
37.539297
]
}
},
{
"type": "Feature",
"properties": {
"type": 5,
"ratio": 0.0033,
"lineWidthRatio": 0.023255813953488372
},
"geometry": {
"type": "Point",
"coordinates": [
113.114543,
41.034126
]
}
},
{
"type": "Feature",
"properties": {
"type": 6,
"ratio": 0.0033,
"lineWidthRatio": 0.023255813953488372
},
"geometry": {
"type": "Point",
"coordinates": [
119.649506,
29.089524
]
}
},
{
"type": "Feature",
"properties": {
"type": 7,
"ratio": 0.0033,
"lineWidthRatio": 0.023255813953488372
},
"geometry": {
"type": "Point",
"coordinates": [
106.713478,
26.578343
]
}
},
{
"type": "Feature",
"properties": {
"type": 0,
"ratio": 0.0032,
"lineWidthRatio": 0.020348837209302327
},
"geometry": {
"type": "Point",
"coordinates": [
115.892151,
28.676493
]
}
},
{
"type": "Feature",
"properties": {
"type": 1,
"ratio": 0.0032,
"lineWidthRatio": 0.020348837209302327
},
"geometry": {
"type": "Point",
"coordinates": [
108.705117,
34.333439
]
}
},
{
"type": "Feature",
"properties": {
"type": 2,
"ratio": 0.0032,
"lineWidthRatio": 0.020348837209302327
},
"geometry": {
"type": "Point",
"coordinates": [
114.879365,
30.447711
]
}
},
{
"type": "Feature",
"properties": {
"type": 3,
"ratio": 0.0032,
"lineWidthRatio": 0.020348837209302327
},
"geometry": {
"type": "Point",
"coordinates": [
109.99029,
39.817179
]
}
},
{
"type": "Feature",
"properties": {
"type": 4,
"ratio": 0.0032,
"lineWidthRatio": 0.020348837209302327
},
"geometry": {
"type": "Point",
"coordinates": [
120.672111,
28.000575
]
}
},
{
"type": "Feature",
"properties": {
"type": 5,
"ratio": 0.0031,
"lineWidthRatio": 0.017441860465116275
},
"geometry": {
"type": "Point",
"coordinates": [
108.320004,
22.82402
]
}
},
{
"type": "Feature",
"properties": {
"type": 6,
"ratio": 0.0031,
"lineWidthRatio": 0.017441860465116275
},
"geometry": {
"type": "Point",
"coordinates": [
117.129063,
36.194968
]
}
},
{
"type": "Feature",
"properties": {
"type": 7,
"ratio": 0.0031,
"lineWidthRatio": 0.017441860465116275
},
"geometry": {
"type": "Point",
"coordinates": [
120.139998,
33.377631
]
}
},
{
"type": "Feature",
"properties": {
"type": 0,
"ratio": 0.0031,
"lineWidthRatio": 0.017441860465116275
},
"geometry": {
"type": "Point",
"coordinates": [
120.856394,
40.755572
]
}
},
{
"type": "Feature",
"properties": {
"type": 1,
"ratio": 0.0031,
"lineWidthRatio": 0.017441860465116275
},
"geometry": {
"type": "Point",
"coordinates": [
114.412599,
23.079404
]
}
},
{
"type": "Feature",
"properties": {
"type": 2,
"ratio": 0.0031,
"lineWidthRatio": 0.017441860465116275
},
"geometry": {
"type": "Point",
"coordinates": [
119.946973,
31.772752
]
}
},
{
"type": "Feature",
"properties": {
"type": 3,
"ratio": 0.003,
"lineWidthRatio": 0.014534883720930232
},
"geometry": {
"type": "Point",
"coordinates": [
118.589421,
24.908853
]
}
},
{
"type": "Feature",
"properties": {
"type": 4,
"ratio": 0.003,
"lineWidthRatio": 0.014534883720930232
},
"geometry": {
"type": "Point",
"coordinates": [
113.132855,
29.37029
]
}
},
{
"type": "Feature",
"properties": {
"type": 5,
"ratio": 0.003,
"lineWidthRatio": 0.014534883720930232
},
"geometry": {
"type": "Point",
"coordinates": [
118.047648,
36.814939
]
}
},
{
"type": "Feature",
"properties": {
"type": 6,
"ratio": 0.0029,
"lineWidthRatio": 0.011627906976744179
},
"geometry": {
"type": "Point",
"coordinates": [
119.306239,
26.075302
]
}
},
{
"type": "Feature",
"properties": {
"type": 7,
"ratio": 0.0029,
"lineWidthRatio": 0.011627906976744179
},
"geometry": {
"type": "Point",
"coordinates": [
112.607693,
26.900358
]
}
},
{
"type": "Feature",
"properties": {
"type": 0,
"ratio": 0.0029,
"lineWidthRatio": 0.011627906976744179
},
"geometry": {
"type": "Point",
"coordinates": [
115.041299,
35.768234
]
}
},
{
"type": "Feature",
"properties": {
"type": 1,
"ratio": 0.0029,
"lineWidthRatio": 0.011627906976744179
},
"geometry": {
"type": "Point",
"coordinates": [
109.502882,
34.499381
]
}
},
{
"type": "Feature",
"properties": {
"type": 2,
"ratio": 0.0029,
"lineWidthRatio": 0.011627906976744179
},
"geometry": {
"type": "Point",
"coordinates": [
115.992811,
29.712034
]
}
},
{
"type": "Feature",
"properties": {
"type": 3,
"ratio": 0.0029,
"lineWidthRatio": 0.011627906976744179
},
"geometry": {
"type": "Point",
"coordinates": [
111.134335,
37.524366
]
}
},
{
"type": "Feature",
"properties": {
"type": 4,
"ratio": 0.0028,
"lineWidthRatio": 0.008720930232558138
},
"geometry": {
"type": "Point",
"coordinates": [
114.341447,
34.797049
]
}
},
{
"type": "Feature",
"properties": {
"type": 5,
"ratio": 0.0028,
"lineWidthRatio": 0.008720930232558138
},
"geometry": {
"type": "Point",
"coordinates": [
121.618622,
38.91459
]
}
},
{
"type": "Feature",
"properties": {
"type": 6,
"ratio": 0.0028,
"lineWidthRatio": 0.008720930232558138
},
"geometry": {
"type": "Point",
"coordinates": [
113.826063,
34.022956
]
}
},
{
"type": "Feature",
"properties": {
"type": 7,
"ratio": 0.0028,
"lineWidthRatio": 0.008720930232558138
},
"geometry": {
"type": "Point",
"coordinates": [
106.937265,
27.706626
]
}
},
{
"type": "Feature",
"properties": {
"type": 0,
"ratio": 0.0028,
"lineWidthRatio": 0.008720930232558138
},
"geometry": {
"type": "Point",
"coordinates": [
87.617733,
43.792818
]
}
},
{
"type": "Feature",
"properties": {
"type": 1,
"ratio": 0.0028,
"lineWidthRatio": 0.008720930232558138
},
"geometry": {
"type": "Point",
"coordinates": [
120.750865,
30.762653
]
}
},
{
"type": "Feature",
"properties": {
"type": 2,
"ratio": 0.0028,
"lineWidthRatio": 0.008720930232558138
},
"geometry": {
"type": "Point",
"coordinates": [
113.307718,
33.735241
]
}
},
{
"type": "Feature",
"properties": {
"type": 3,
"ratio": 0.0027,
"lineWidthRatio": 0.0058139534883720955
},
"geometry": {
"type": "Point",
"coordinates": [
103.823557,
36.058039
]
}
},
{
"type": "Feature",
"properties": {
"type": 4,
"ratio": 0.0027,
"lineWidthRatio": 0.0058139534883720955
},
"geometry": {
"type": "Point",
"coordinates": [
109.741193,
38.290162
]
}
},
{
"type": "Feature",
"properties": {
"type": 5,
"ratio": 0.0027,
"lineWidthRatio": 0.0058139534883720955
},
"geometry": {
"type": "Point",
"coordinates": [
112.23813,
30.326857
]
}
},
{
"type": "Feature",
"properties": {
"type": 6,
"ratio": 0.0026,
"lineWidthRatio": 0.0029069767441860417
},
"geometry": {
"type": "Point",
"coordinates": [
113.382391,
22.521113
]
}
},
{
"type": "Feature",
"properties": {
"type": 7,
"ratio": 0.0025,
"lineWidthRatio": 0
},
"geometry": {
"type": "Point",
"coordinates": [
112.144146,
32.042426
]
}
},
{
"type": "Feature",
"properties": {
"type": 0,
"ratio": 0.0025,
"lineWidthRatio": 0
},
"geometry": {
"type": "Point",
"coordinates": [
113.113556,
36.191112
]
}
},
{
"type": "Feature",
"properties": {
"type": 1,
"ratio": 0.0025,
"lineWidthRatio": 0
},
"geometry": {
"type": "Point",
"coordinates": [
114.391136,
27.8043
]
}
},
{
"type": "Feature",
"properties": {
"type": 2,
"ratio": 0.0025,
"lineWidthRatio": 0
},
"geometry": {
"type": "Point",
"coordinates": [
111.691347,
29.040225
]
}
}
]
}