[LeetCode] 697.配列の次数(簡単)(JAVA)
件名アドレス:https://leetcode.com/problems/degree-of-an-array/
タイトル説明:
非負の整数numsの空でない配列が与えられた場合、この配列の次数は、その要素のいずれか1つの最大頻度として定義されます。
あなたの仕事は、numsと同じ次数を持つnumsの(連続した)サブ配列の可能な限り最小の長さを見つけることです。
例1:
Input: nums = [1,2,2,3,1]
Output: 2
Explanation:
The input array has a degree of 2 because both elements 1 and 2 appear twice.
Of the subarrays that have the same degree:
[1, 2, 2, 3, 1], [1, 2, 2, 3], [2, 2, 3, 1], [1, 2, 2], [2, 2, 3], [2, 2]
The shortest length is 2. So return 2.
例2:
Input: nums = [1,2,2,3,1,4,2]
Output: 6
Explanation:
The degree is 3 because the element 2 is repeated 3 times.
So [2,2,3,1,4,2] is the shortest subarray, therefore returning 6.
制約:
- nums.lengthは1から50,000の間です。
- nums [i]は、0から49,999までの整数になります。
一般的なアイデア
非負の数のみを含む空でない整数配列numが与えられた場合、配列の次数は、指数グループ内の任意の要素の最大値として定義されます。
あなたの仕事は、numsと同じ次数のnumsで最も短い連続したサブ配列を見つけ、その長さを返すことです。
問題解決方法
- 配列の次数を調べるには、マップを使用して各要素の次数を記録できます。
- マップをもう一度トラバースして、最も頻繁に発生するものを見つけ、最小の次数が結果になります
class Solution {
public int findShortestSubArray(int[] nums) {
if (nums.length <= 0) return 0;
Map<Integer, int[]> map = new HashMap<>();
for (int i = 0; i < nums.length; i++) {
int[] pre = map.get(nums[i]);
if (pre != null) {
map.put(nums[i], new int[]{pre[0] + 1, pre[1], i});
} else {
map.put(nums[i], new int[]{1, i, i});
}
}
int min = 0;
int maxCount = 0;
for (Map.Entry<Integer, int[]> entry : map.entrySet()) {
int[] temp = entry.getValue();
if (temp[0] > maxCount) {
maxCount = temp[0];
min = temp[2] - temp[1] + 1;
} else if (maxCount == temp[0]) {
min = Math.min(min, temp[2] - temp[1] + 1);
}
}
return min;
}
}
実行時間:17ミリ秒、Javaユーザーの84.89%を打ち負かす
メモリ消費量:44 MB、Javaユーザーの7.13%を打ち負かす
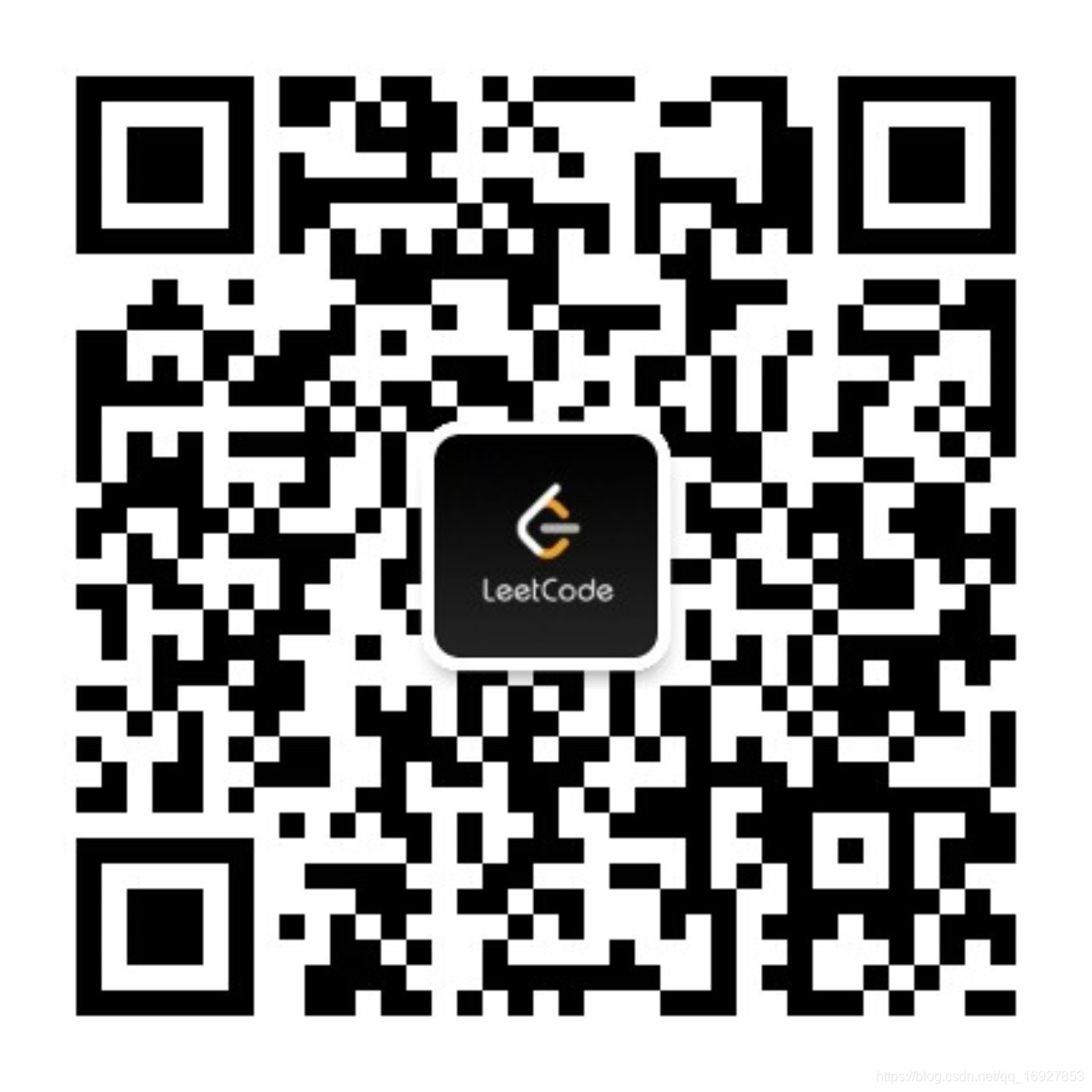