GridView receives the following optional parameter attributes:
scrollDirection
:Scroll methodpadding
:Paddingresolve
: Components sorted in reverse ordercrossAxisSpacing
: Spacing between horizontal sub-WidgetsmainAxisSpacing
: Spacing between vertical sub-WidgetscrossAxisCount
: Number of Widgets in a rowchildAspectRatio
: Child Widget width-to-height ratiochildren
:gridDelegate
: Control layout is mainly usedGridView.builder
inside
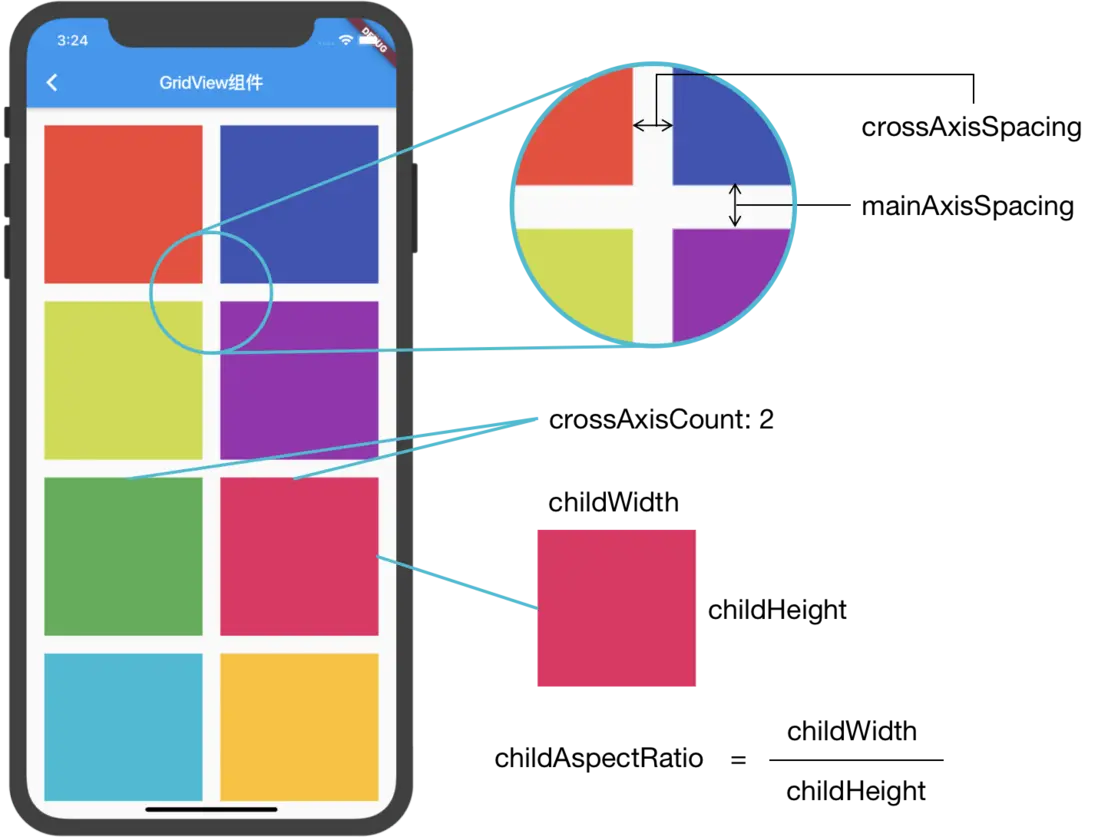
To implement the code, I won’t say more
: 1. Grid data. I chose seven data: icons and subtitles corresponding to them.
import 'package:flutter/material.dart';
List listData = [
{
"title": "信息列表1",
"author": 'Mohamed Chahin',
// "imageUrl": 'https://www.itying.com/images/flutter/1.png',
// "imageUrl": 'images/json.png',
"imageUrl": Icons.account_tree_rounded,
},
{
"title": '信息列表2',
"author": 'Mohamed Chahin',
// "imageUrl": 'https://www.itying.com/images/flutter/1.png',
// "imageUrl": 'images/statistics.png',
"imageUrl": Icons.ad_units_rounded,
},
{
"title": '信息列表3',
"author": 'Mohamed Chahin',
// "imageUrl": 'https://www.itying.com/images/flutter/1.png',
// "imageUrl": 'images/statistics.png',
"imageUrl": Icons.adb,
},
{
"title": '信息列表4',
"author": 'Mohamed Chahin',
// "imageUrl": 'https://www.itying.com/images/flutter/1.png',
// "imageUrl": 'images/statistics.png',
"imageUrl": Icons.add_alert_sharp,
},
{
"title": '信息列表5',
"author": 'Mohamed Chahin',
// "imageUrl": 'https://www.itying.com/images/flutter/1.png',
// "imageUrl": 'images/statistics.png',
"imageUrl": Icons.add_a_photo_rounded,
},
{
"title": '信息列表6',
"author": 'Mohamed Chahin',
// "imageUrl": 'https://www.itying.com/images/flutter/1.png',
// "imageUrl": 'images/json.png',
"imageUrl": Icons.add_a_photo_outlined,
},
{
"title": '信息列表7',
"author": 'Mohamed Chahin',
// "imageUrl": 'images/statistics.png',
"imageUrl": Icons.add_photo_alternate_sharp,
}
];
2. View main.dart
code:
import 'package:flutter/material.dart';
import 'package:flutter_test_app/res/listData.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({
super.key});
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({
super.key, required this.title});
final String title;
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: Text(widget.title),
),
body: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
//新增代码
Expanded(
child: GridViewTest(),
),
],
),
);
}
}
class GridViewTest extends StatefulWidget {
LayoutDemo createState() => LayoutDemo();
}
class LayoutDemo extends State<GridViewTest> {
List<Widget> _getListData() {
var tempList = listData.map((value) {
return Center(
child: InkWell( //InkWell:GridView的item背景点击变色,松开恢复原色
onTap: () {
},
child: Container(
alignment: Alignment.bottomCenter,
//设置一个外边框
decoration: BoxDecoration(
border: Border.all(
color: Colors.black12,
width: 1.0, //默认
)),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const SizedBox(width: 10, height: 10),
Icon(
value['imageUrl'],
color: Colors.green,
size: 60,
),
Text(value['title'])
],
),
),
),
);
});
return tempList.toList();
}
Widget build(BuildContext context) {
return GridView.count(
crossAxisCount: 3,
children: _getListData(),
// mainAxisSpacing:9.0,
// crossAxisSpacing:9.0,
// padding: const EdgeInsets.all(10),
);
}
}
running result:
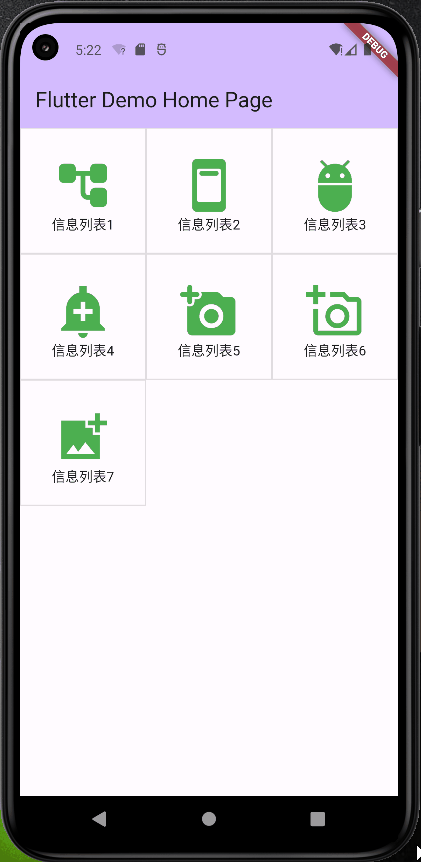