Prefacio: El uso de código para generar Excel se usa principalmente para un diseño relativamente simple de Excel. Si necesita usar una exportación de informes de Excel más compleja, puede leer otro artículo
1. Importar el paquete jar
<dependency>
<groupId>cn.afterturn</groupId>
<artifactId>easypoi-base</artifactId>
<version>3.0.3</version>
<exclusions>
<exclusion>
<artifactId>commons-lang3</artifactId>
<groupId>org.apache.commons</groupId>
</exclusion>
<exclusion>
<artifactId>guava</artifactId>
<groupId>com.google.guava</groupId>
</exclusion>
</exclusions>
</dependency>
2. Aplicación de código
Propiedades de clase de entidad exportadas (puede usar @Excel para establecer propiedades de celda)
package com.xinke.sunshine_ebid.dal.entity;
import cn.afterturn.easypoi.excel.annotation.Excel;
import lombok.Data;
@Data
public class TestEntity {
@Excel(name = "姓名", orderNum = "1", width = 15)
private String name;
@Excel(name = "电话", orderNum = "2", width = 20)
private String phone;
@Excel(name = "地址", orderNum = "3", width = 30)
private String address;
}
capa de control
package com.xinke.sunshine_ebid.webapp;
import cn.afterturn.easypoi.excel.ExcelExportUtil;
import cn.afterturn.easypoi.excel.entity.TemplateExportParams;
import com.xinke.sunshine_ebid.common.utils.EasyPoiUtils;
import com.xinke.sunshine_ebid.dal.entity.TestEntity;
import com.xinke.sunshine_ebid.service.TestService;
import org.apache.poi.ss.usermodel.Workbook;
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import javax.annotation.Resource;
import javax.servlet.http.HttpServletResponse;
import java.util.List;
import java.util.Map;
@CrossOrigin
@RestController
@RequestMapping(value = "/Test")
public class TestController {
@Resource
private TestService testService;
/**
* 使用过代码直接生成excel表格
* @param response
* @throws Exception
*/
@GetMapping("/test")
public void test(HttpServletResponse response) throws Exception{
List<TestEntity> data = testService.test();
EasyPoiUtils.exportExcel(data, null, "设置的表格名称", TestEntity.class,"设置的文件名称",response);
}
}
capa de lógica de negocios
package com.xinke.sunshine_ebid.service;
import com.xinke.sunshine_ebid.dal.entity.TestEntity;
import org.springframework.stereotype.Service;
import java.util.ArrayList;
import java.util.List;
@Service
public class TestService {
public List<TestEntity> test() throws Exception{
// 封装参数
List<TestEntity> list = new ArrayList<>();
TestEntity test = new TestEntity();
test.setName("瓜");
test.setPhone("18888888888");
test.setAddress("PD");
list.add(test);
TestEntity test2 = new TestEntity();
test2.setName("瓜2");
test2.setPhone("1999999999");
test2.setAddress("PD2");
list.add(test2);
return list;
}
}
3. prueba
interfaz de llamada
localhost:8087/Prueba/prueba
Exportación exitosa
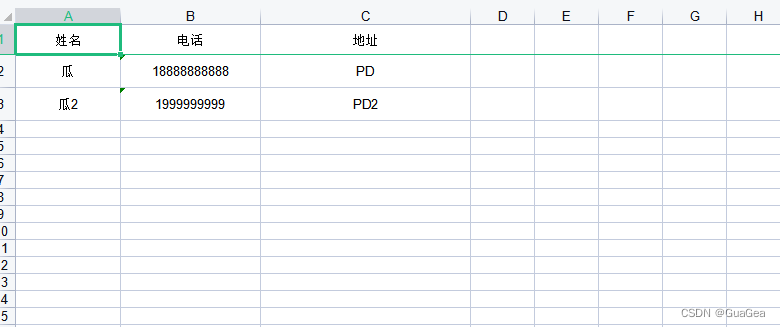