1. En el proceso de desarrollo de un proyecto web, la legalidad de los datos enviados por los usuarios es el método de verificación más básico. En SpringBoot, el paquete de componentes hibernate-vidator se puede usar directamente para lograr el procesamiento de verificación y las anotaciones de verificación admitidas en este paquete de componentes, como Como se muestra en la figura.
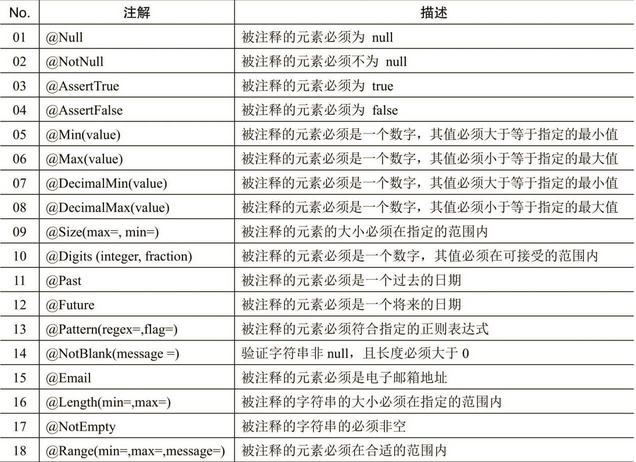
2. Cree un archivo ValidationMessages.properties (el nombre del archivo es el valor predeterminado y no se puede cambiar) en el directorio src / main / resources. Todos los mensajes de error deben guardarse en este archivo.
1 userinfo.username.notnull.error=用户账号不能为空!
2 userinfo.email.from.error=请输入正确格式的用户邮箱!
3 userinfo.username.length.error=用户账号长度错误!
4 userinfo.age.notnull.error=用户年龄不能为空!
5 userinfo.age.digits.error=用户年龄必须是合法的数字!
6 userinfo.salary.notnull.error=用户工资不能为空!
7 userinfo.salary.digits.error=用户工资必须是合法的数字!
8 userinfo.birthday.notnull.error=用户生日不允许为空!
Cree una clase de entidad UserInfo y use anotaciones de validación en esta clase. Al mismo tiempo, el mensaje de error cuando se produce el error de validación hace referencia a la definición del archivo ValidationMessages.properties anterior.
1 package com.demo.po;
2
3 import java.io.Serializable;
4 import java.util.Date;
5
6 import javax.validation.constraints.Digits;
7 import javax.validation.constraints.Email;
8 import javax.validation.constraints.NotNull;
9
10 /**
11 *
12 * @author
13 *
14 */
15 public class UserInfo implements Serializable {
16
17 /**
18 *
19 */
20 private static final long serialVersionUID = 1L;
21
22 @NotNull(message = "{userinfo.username.notnull.error}")
23 private String userName;
24
25 @Email(message = "{userinfo.email.from.error}")
26 private String email;
27
28 @NotNull(message = "{userinfo.age.notnull.error}")
29 @Digits(integer = 3, fraction = 0, message = "{userinfo.age.digits.error}")
30 private Integer age;
31
32 @NotNull(message = "{userinfo.salary.notnull.error}")
33 @Digits(integer = 20, fraction = 2, message = "{userinfo.salary.digits.error}")
34 private Integer salary;
35
36 @NotNull(message = "{userinfo.birthday.notnull.error}")
37 private Date birthday;
38
39 public String getUserName() {
40 return userName;
41 }
42
43 public void setUserName(String userName) {
44 this.userName = userName;
45 }
46
47 public String getEmail() {
48 return email;
49 }
50
51 public void setEmail(String email) {
52 this.email = email;
53 }
54
55 public Integer getAge() {
56 return age;
57 }
58
59 public void setAge(Integer age) {
60 this.age = age;
61 }
62
63 public Integer getSalary() {
64 return salary;
65 }
66
67 public void setSalary(Integer salary) {
68 this.salary = salary;
69 }
70
71 public Date getBirthday() {
72 return birthday;
73 }
74
75 public void setBirthday(Date birthday) {
76 this.birthday = birthday;
77 }
78
79 }
Construya un controlador y comience a verificar y juzgar los campos, como se muestra a continuación:
1 package com.demo.controller;
2
3 import java.text.SimpleDateFormat;
4 import java.util.Iterator;
5
6 import javax.validation.Valid;
7
8 import org.springframework.beans.propertyeditors.CustomDateEditor;
9 import org.springframework.stereotype.Controller;
10 import org.springframework.validation.BindingResult;
11 import org.springframework.validation.ObjectError;
12 import org.springframework.web.bind.WebDataBinder;
13 import org.springframework.web.bind.annotation.InitBinder;
14 import org.springframework.web.bind.annotation.PostMapping;
15 import org.springframework.web.bind.annotation.ResponseBody;
16
17 import com.demo.po.UserInfo;
18
19 @Controller
20 public class ValidationController {
21
22 /**
23 *
24 *
25 * @param userInfo
26 * @param result
27 * @return
28 */
29 @PostMapping(value = "member_add")
30 @ResponseBody
31 public Object add(@Valid UserInfo userInfo, BindingResult result) {
32 // 执行的验证出现错误
33 if (result.hasErrors()) {
34 Iterator<ObjectError> iterator = result.getAllErrors().iterator();
35 // 获取全部的错误
36 while (iterator.hasNext()) {
37 // 取出每一个错误
38 ObjectError error = iterator.next();
39 System.out.println("【错误信息】 code = " + error.getCode() + ", message = " + error.getDefaultMessage());
40 }
41 return result.getAllErrors();
42 } else {
43 return userInfo;
44 }
45 }
46
47 /**
48 * 本程序需要对日期格式进行处理
49 *
50 * @param binder
51 */
52 @InitBinder
53 public void initBinder(WebDataBinder binder) {
54 // 建立一个可以将字符串转换为日期的程序类
55 SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
56 // 明确的描述此时需要注册一个日期格式的转换处理程序
57 binder.registerCustomEditor(java.util.Date.class, new CustomDateEditor(sdf, true));
58 }
59
60 }
Luego, cree un cartero para probar si su interfaz de verificación es normal, como se muestra a continuación:
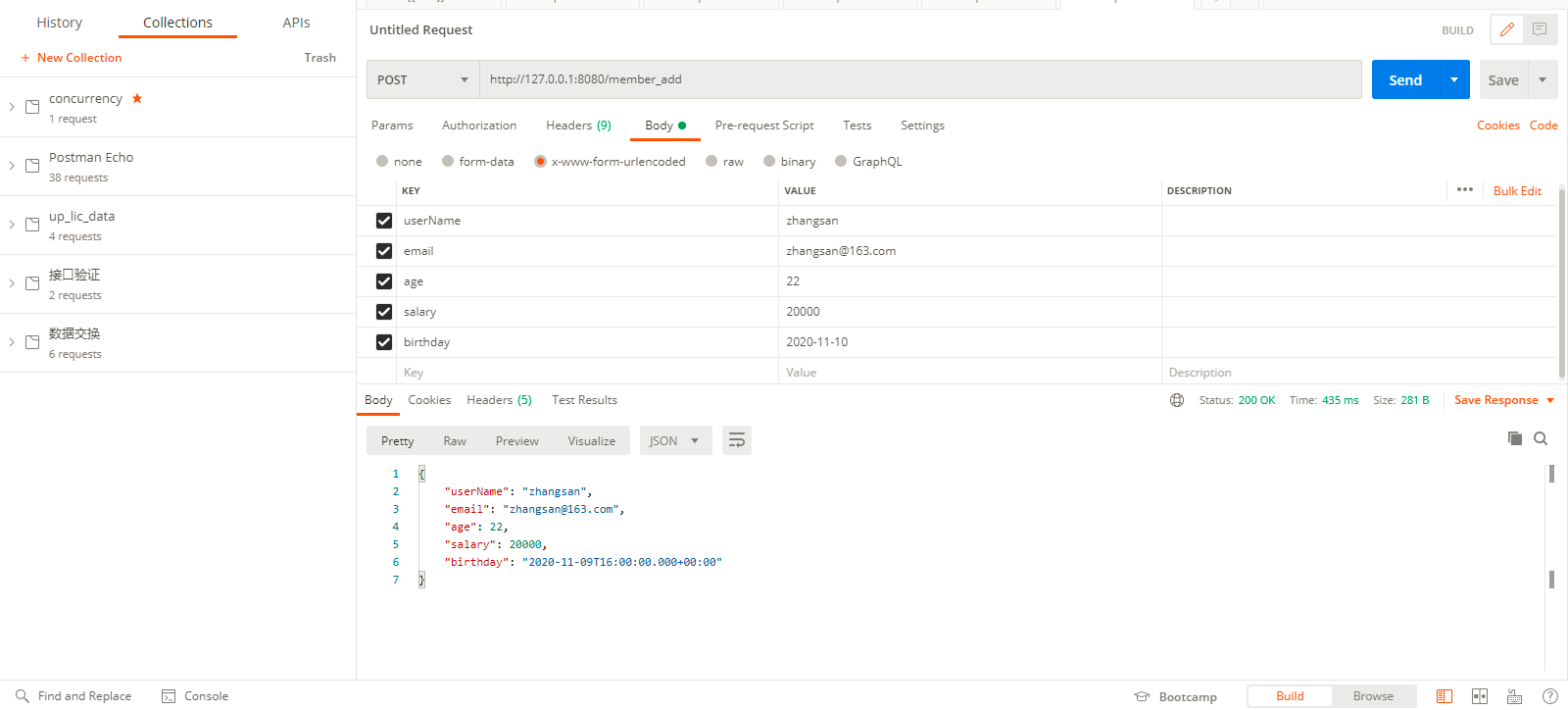