El método también se denomina método. El lenguaje Go admite la herencia y reescritura de métodos.
Uno, herencia de método
El método se puede heredar. Si el campo anónimo implementa un método, la estructura que contiene el campo anónimo también puede llamar al método en la estructura anónima.
El caso es el siguiente:
//myMethod02.go
// myMehthodJicheng2 project main.go
package main
import (
"fmt"
)
type Human struct {
name, phone string
age int
}
type Student struct {
Human
school string
}
type Employee struct {
Human
company string
}
func (h *Human) SayHi() {
fmt.Printf("大家好! 我是%s, 我%d岁, 我的电话是: %s\n",
h.name, h.age, h.phone)
}
func main() {
s1 := Student{Human{"Anne", "15012349875", 16}, "武钢三中"}
e1 := Employee{Human{"Sunny", "18045613416", 35}, "1000phone"}
s1.SayHi()
e1.SayHi()
}
El efecto es el siguiente:
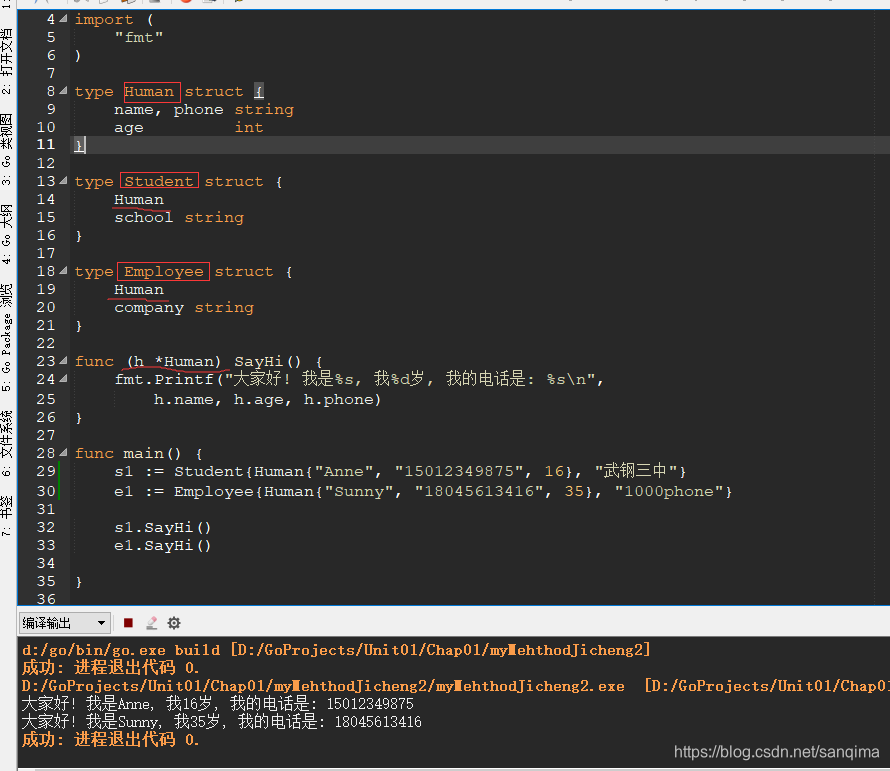
Dos, método de reescritura
La subclase reescribe la función del método de la clase principal con el mismo nombre; al llamar, se llama primero al método de la subclase, si la subclase no existe, se llama al método de la clase principal.
El caso es el siguiente:
//myMethod2.go
// myMehthodJicheng2 project main.go
package main
import (
"fmt"
)
type Human struct {
name, phone string
age int
}
type Student struct {
Human
school string
}
type Employee struct {
Human
company string
}
func (h *Human) SayHi() {
fmt.Printf("大家好! 我是%s, 我%d岁, 我的电话是: %s\n",
h.name, h.age, h.phone)
}
func (s *Student) SayHi() {
fmt.Printf("大家好! 我是%s, 我%d岁, 我在%s上学, 我的电话是: %s\n",
s.name, s.age, s.school, s.phone)
}
func (e *Employee) SayHi() {
fmt.Printf("大家好! 我是%s, 我%d岁, 我在%s工作, 我的电话是: %s\n",
e.name, e.age, e.company, e.phone)
}
func main() {
s1 := Student{Human{"Anne", "15012349875", 16}, "武钢三中"}
e1 := Employee{Human{"Sunny", "18045613416", 35}, "1000phone"}
s1.SayHi()
e1.SayHi()
}
El efecto es el siguiente:
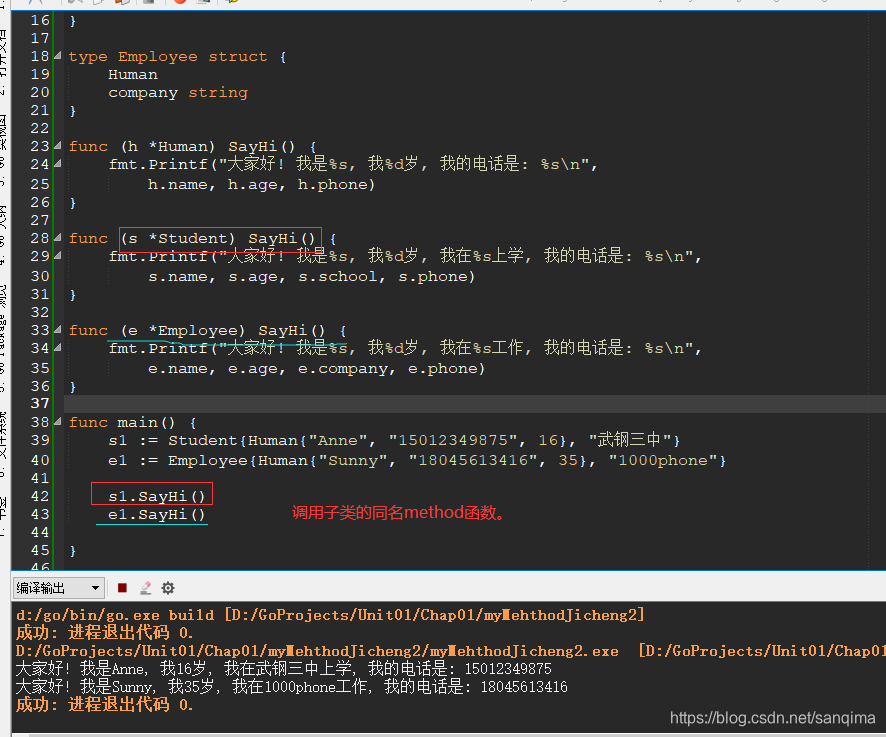