Day 2 studies online CMS front-end development
1 Vue.js research and Webpack
2 CMS to create a front-end engineering
2.1 Import system management front-end engineering
CMS system using Vue-cli scaffolding to create, Vue-cli scaffolding is provided to quickly build Vue official one-page application, github address: https://github.com/vuejs/vue-cli (interested students can refer to the official guide to create a front-end engineering using vue-cli), the project engineering Vue-cli-created second package, the following briefing CMS project.
2.2.1 engineering structures
if I have to be developed based on engineering Vue-Cli created also need to make some packaged in it on the basis of the import course materials provided Vue-Cli package engineering. The xc-ui-pc-sysmanage.7z course materials copied to the UI project directory, and unpack, open xc-ui-pc-sysmanage directory with WebStorm
Front-end tests have demo
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script src="vue.min.js"></script>
</head>
<body>
<div id="app">
{{name}}
</div>
<script>
var Vm=new Vue({
el:"#app",
data:{
name: "我这个是第一次进行前端得开发"
}
})
</script>
</body>
</html>
V-model: a test case to open a small demo:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script src="vue.min.js"></script>
</head>
<body>
<div id="app">
{{name}}
<input v-model="num1">+
<input v-model="num2">=
{{Number.parseInt(num1)+Number.parseInt(num2)}}
<button value="计算">计算</button>
</div>
</body>
<script>
var vm=new Vue({
el:'#app',
data:{
name:"黑马程序员",
num1:1,
num2:1
}
})
</script>
</html>
V-text solve the problem of flicker difference
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div id="app">
<span v-text="name"></span>
<input value="1" type="text" v-model="num1">+
<input value="1" type="text" v-model="num2">=
<span v-text="Number.parseInt(num1)+Number.parseInt(num2)"></span>
<button>计算</button>
</div>
<script src="vue.min.js"></script>
<script>
var vue=new Vue({
el:'#app',
data:{
name:"黑马程序员",
num1:1,
num2:1
}
})
</script>
</body>
</html>
v-on Test Examples small demo
<!DOCTYPE html>
<html lang="en" xmlns:v-on="http://www.w3.org/1999/xhtml">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div id="app">
<span v-text="name"></span>
<input type="text" v-model="num1">+
<input type="text" v-model="num2">=
<span v-text="result"></span>
<button v-on:click="change">计算</button>
</div>
</body>
<script src="vue.min.js"></script>
<script>
var vm=new Vue({
el:'#app',
data:{
name:"黑马程序员",
num1:0,
num2:0,
result:0
},
methods:{
change(){
this.result=Number.parseInt(this.num1)+Number.parseInt(this.num2);
}
}
})
</script>
</html>
V-bind small test case
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div id="app">
<a :href="url">
<span v-text="name"></span>
</a>
<input type="text" v-model="num1">+
<input type="text" v-model="num2">=
<span v-text="result"></span>
<button v-on:click="change">计算</button>
<span :style="{fontSize:size+'px'}">JavaEE工程师</span>
</div>
</body>
<script src="vue.min.js"></script>
<script>
var vue=new Vue({
el:'#app',
data:{
name:"黑马程序员",
num1:0,
num2:0,
result:0,
size:33,
url:"http://www.baidu.com"
},
methods:{
change(){
this.result=Number.parseInt(this.num1)+Number.parseInt(this.num2);
}
}
})
</script>
</html>
v-for and -if basis demo
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div id="app">
<ul>
<span>数组遍历测试==============</span>
<li v-for="(item,index) in arrayList" :key="index">{{item}}----{{index}}</li>
<span>对象遍历测试==============</span>
<li v-for="(value,key) in user">{{key}}-----{{value}}</li>
<span>集合遍历测试==============</span>
<li v-for="(item,key) in userlist" :key="item.user.username">
<div v-if="item.user.username=='wangdachui'" style="background-color: #00b3ee">
{{item.user.username}}----{{item.user.age}}
</div>
<div v-else="">
{{item.user.username}}----{{item.user.age}}
</div>
</li>
</ul>
</div>
</body>
<script src="vue.min.js"></script>
<script>
var vue=new Vue({
el:'#app',
data:{
arrayList:[1,2,3,4,5,6],
user:{username:"wangdachui",age:22},
userlist:[
{user:{username:"wangdachui",age:22}},{user:{username:"wangjiejie",age:90}}
]
}
})
</script>
</html>
About WebPack first demo program quiz
1.modl1.js
function add(x,y) {
return x+y;
}
module.exports.add=add;
2.main.js
var {add}=require("./module")
var Vue=require("./vue.min");
var vue=new Vue({
el:'#app',
data:{
name:"黑马程序员",
num1:0,
num2:0,
result:0,
size:33,
url:"http://www.baidu.com"
},
methods:{
change(){
this.result=add(Number.parseInt(this.num1),Number.parseInt(this.num2));
}
}
})
3.webpack.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div id="app">
<a :href="url">
<span v-text="name"></span>
</a>
<input type="text" v-model="num1">+
<input type="text" v-model="num2">=
<span v-text="result"></span>
<button v-on:click="change">计算</button>
<span :style="{fontSize:size+'px'}">JavaEE工程师</span>
</div>
</body>
<script src="vue.min.js"></script>
<script src="builds.js">
</script>
</html>
Complete the normal test and development process
Webpack using automated build tools for this process to complete the hot deployment front-end learning new to me, it is quite strange, but after complete understanding of the process is actually not difficult to find, first of all to separate, fixed file structure
After completion of the file structure is divided started fixed npm command, for the convenience of packaging to use, and will command a stationary hot deployment package.json added after packing on the inside
{
"scripts": {
"dev": "webpack-dev-server --inline --hot --open --port 5008"
},
"devDependencies": {
"html-webpack-plugin": "^2.30.1",
"webpack": "^3.6.0",
"webpack-dev-server": "^2.9.1"
}
}
After creating a webpack.config order to complete the corresponding development process, the development of this document webpack
//引用html-webpack-plugin插件,作用是根据html模板在内存生成html文件,它的工作原理是根据模板文件在内存中生成一个index.html文件。
var htmlwp = require('html-webpack-plugin');
module.exports={
entry:'./src/main.js', //指定打包的入口文件
output:{
path : __dirname+'/dist', // 注意:__dirname表示webpack.config.js所在目录的绝对路径
filename:'build.js' //输出文件
},
devtool: 'eval-source-map',
plugins:[
new htmlwp({
title: '首页', //生成的页面标题<head><title>首页</title></head>
filename: 'index.html', //webpack-dev-server在内存中生成的文件名称,自动将build注入到这个页面底部,才能实现自动刷新功能
template: 'webpack2.html' //根据vue_02.html这个模板来生成(这个文件请程序员自己生成)
})
]
}
After all the files you've created a command can be run direct-mail this hot deployment operation, very fast and convenient
接下来就是对CMS的前端工程的应用的开发导入相应的前端工程执行run dev
运行可能会出现这个错误
解决办法:
说明node sass版本在当前环境运行不了,解决方法如下:
1.卸载当前版本node sass
npm uninstall --save node-sass
2.重新安装node sass
cnpm install --save node-sass
完成运行
2.2 单页面应用介绍
什么是单页应用?
引用百度百科:
单页面应用的优缺点:
优点:
1、用户操作体验好,用户不用刷新页面,整个交互过程都是通过Ajax来操作。
2、适合前后端分离开发,服务端提供http接口,前端请求http接口获取数据,使用JS进行客户端渲染。
缺点:
1、首页加载慢 单页面应用会将js、 css打包成一个文件,在加载页面显示的时候加载打包文件,如果打包文件较大或者网速慢则 用户体验不好。
2、SEO不友好 SEO(Search Engine Optimization)为搜索引擎优化。它是一种利用搜索引擎的搜索规则来提高网站在搜索引擎 排名的方法。目前各家搜索引擎对JS支持不好,所以使用单页面应用将大大减少搜索引擎对网站的收录。
总结:
本项目的门户、课程介绍不采用单页面应用架构去开发,对于需要用户登录的管理系统采用单页面开发。
3 CMS前端页面查询开发
3.1 页面原型
3.1.1 创建页面
3.1.1.1 页面结构
在model目录创建 cms模块的目录结构
关于对CMS前端工程的CMS的页面导入和路由配置,
先建一个和home目录类似的文件结构
该文件结构构建完成之后即可配置路由,路由的导入则遵循在头部导入具体的页面路径
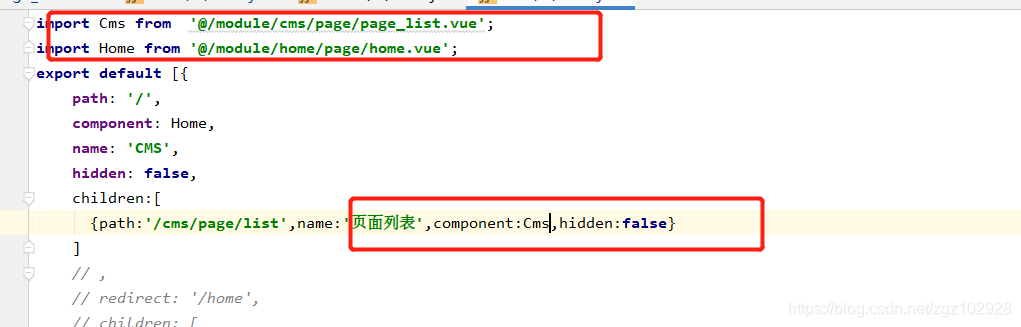
之后在base基础功能中,导入全局路由
路由配置完成,进行测试:
开始导入elemetnUi中的组件进行相应的布局只用去ElementUI的官网进行相应的复制,就可以了
<template>
<div>
<el-button>查询</el-button>
<el-table
:data="tableData"
stripe
style="width: 100%">
<el-table-column
prop="date"
label="日期"
width="180">
</el-table-column>
<el-table-column
prop="name"
label="姓名"
width="180">
</el-table-column>
<el-table-column
prop="address"
label="地址">
</el-table-column>
</el-table>
</div>
</template>
<script>
export default {
data() {
return {
tableData: [{
date: '2016-05-02',
name: '王小虎',
address: '上海市普陀区金沙江路 1518 弄'
}, {
date: '2016-05-04',
name: '王小虎',
address: '上海市普陀区金沙江路 1517 弄'
}, {
date: '2016-05-01',
name: '王小虎',
address: '上海市普陀区金沙江路 1519 弄'
}, {
date: '2016-05-03',
name: '王小虎',
address: '上海市普陀区金沙江路 1516 弄'
}]
}
}
}
</script>
<style scoped>
</style>
关于ElementUi得页面组件得导入及其分页插件导入得相关得demo案例
<template>
<div>
<el-button @click="query">查询</el-button>
<el-table :data="list" stripe style="width: 100%">
<el-table-column type="index" width="60">
</el-table-column>
<el-table-column prop="pageName" label="页面名称" width="120">
</el-table-column>
<el-table-column prop="pageAliase" label="别名" width="120">
</el-table-column>
<el-table-column prop="pageType" label="页面类型" width="150">
</el-table-column>
<el-table-column prop="pageWebPath" label="访问路径" width="250">
</el-table-column>
<el-table-column prop="pagePhysicalPath" label="物理路径" width="250">
</el-table-column>
<el-table-column prop="pageCreateTime" label="创建时间" width="180" >
</el-table-column>
</el-table>
<el-pagination
style="float:right"
background
layout="prev, pager, next"
:total="total"
:page-size="param.page"
:current-page="param.size"
@current-change="change"
>
</el-pagination>
</div>
</template>
<script>
export default {
data() {
return {
list: [ {
"siteId": "5a751fab6abb5044e0d19ea1",
"pageId": "5ad92f5468db52404cad0f7c",
"pageName": "402885816243d2dd016243f24c030002.html",
"pageAliase": "课程详情页面",
"pageWebPath": "/course/",
"pageParameter": null,
"pagePhysicalPath": "F:/eduprojects/xc-edu-snapshotv1.0/xc-ui-pc-static-portal/course/detail/",
"pageType": "1",
"pageTemplate": null,
"pageHtml": null,
"pageStatus": null,
"pageCreateTime": "2018-05-26T08:49:33.332+0000",
"templateId": "5aec5dd70e661808240ab7a6",
"pageParams": null,
"htmlFileId": "5b091f97c5e9b7070c94a2bb",
"dataUrl": null
},
{
"siteId": "5a751fab6abb5044e0d19ea1",
"pageId": "5ad94b9168db5243ec846e8e",
"pageName": "preview_4028858162e0bc0a0162e0bfdf1a0000.html",
"pageAliase": "课程预览页面",
"pageWebPath": "/coursepre/",
"pageParameter": null,
"pagePhysicalPath": "F:\\develop\\xc_portal_static\\course\\preview\\",
"pageType": "1",
"pageTemplate": null,
"pageHtml": null,
"pageStatus": null,
"pageCreateTime": "2018-04-20T02:08:17.621+0000",
"templateId": "5a925be7b00ffc4b3c1578b5",
"pageParams": null,
"htmlFileId": "5ad94b9168db5243ec846e8f",
"dataUrl": "http://localhost:40200/portalview/course/get/4028858162e0bc0a0162e0bfdf1a0000"
}],
total:50,
param:{
page:1,
size:3
}
}
},
methods:{
query(){
alert("查询")
},
change(){
this.query()
}
}
}
</script>
<style scoped>
</style>
3.2.2 Api调用
前端页面导入cms.js,调用js方法请求服务端页面查询接口。
1)导入cms.js
开始编写服务端得请求,出现跨域得错误
开始解决:处理方法
改变此访问地址前缀
import http from './../../../base/api/public'
import querystring from 'querystring'
let sysConfig = require('@/../config/sysConfig')
let apiUrl = sysConfig.xcApiUrlPre;
export const page_list=(page,size,queryparam)=>{
return http.requestQuickGet(apiUrl+'/cms/page/list/'+page+'/'+size+'');
}
methods:{
query(){
page_list(this.param.page,this.param.size).then(res=>{
this.list=res.queryResult.list;
this.total=res.queryResult.total;
});
},
change(){
this.query()
}
进行mounted的分页初始化查询
methods:{
query(){
page_list(this.param.page,this.param.size).then(res=>{
this.list=res.queryResult.list;
this.total=res.queryResult.total;
});
},
change(page){
this.param.page=page;
this.query();
}
},
mounted() {
this.query();
}
完成: