List of Formula
That list of Formula List Comprehensions, Python is a very simple but powerful built-in can be used to create a list of the formula.
For example, to generate a list [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
can be used list(range(1, 11))
:
>>> list(range(1, 11))
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
But if you want to generate [1x1, 2x2, 3x3, ..., 10x10]
how to do? The first loop method:
>>> L = []
>>> for x in range(1, 11): ... L.append(x * x) ... >>> L [1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
However, the cycle too complicated, and the list of the formula may be replaced with a circulation line list generated above statement:
>>> [x * x for x in range(1, 11)] [1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
When writing a list of the formula, the element to be generated x * x
to the front, followed by for
recycling, you can create a list put out, is useful to write a few times, you will soon be familiar with this syntax.
can also add back loop for determining if, so that we can filter out only the even square of:
>>> [x * x for x in range(1, 11) if x % 2 == 0] [4, 16, 36, 64, 100]
May also be used two cycles, the whole arrangement can be generated:
>>> [m + n for m in 'ABC' for n in 'XYZ'] ['AX', 'AY', 'AZ', 'BX', 'BY', 'BZ', 'CX', 'CY', 'CZ']
More than three and a triple loop is rarely used.
Use the formula list, you can write very simple code. For example, to list all files and directories in the current directory, can be achieved through a line of code:
>>> import os # 导入os模块,模块的概念后面讲到
>>> [d for d in os.listdir('.')] # os.listdir可以列出文件和目录 ['.emacs.d', '.ssh', '.Trash', 'Adlm', 'Applications', 'Desktop', 'Documents', 'Downloads', 'Library', 'Movies', 'Music', 'Pictures', 'Public', 'VirtualBox VMs', 'Workspace', 'XCode']
for
In fact, recycling can simultaneously use two or more variables, such as dict
the items()
possible iterations key and value at the same time:
>>> d = {'x': 'A', 'y': 'B', 'z': 'C' } >>> for k, v in d.items(): ... print(k, '=', v) ... y = B x = A z = C
Thus, the list formula may be used to generate two variables list:
>>> d = {'x': 'A', 'y': 'B', 'z': 'C' } >>> [k + '=' + v for k, v in d.items()] ['y=B', 'x=A', 'z=C']
Finally a list of all the strings to lowercase:
>>> L = ['Hello', 'World', 'IBM', 'Apple'] >>> [s.lower() for s in L] ['hello', 'world', 'ibm', 'apple']
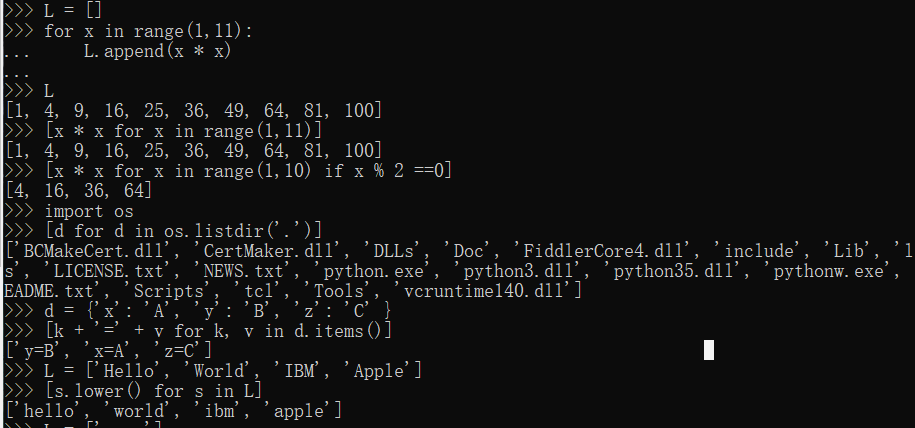
Builder
List by the formula, we can create a list directly. However, subject to memory limitations, the list is certainly limited capacity. Also, create a list containing one million elements, not only take up much storage space, if we only need to access the first few elements behind the elements that the vast majority of occupied space are wasted.
So, if the list element can be calculated out in accordance with an algorithm that if we can continue to calculate in the process cycle in a subsequent elements? This eliminates the need to create a complete list, thus saving a lot of space. In Python, while circulating mechanism for this calculation, known as a generator: generator.
To create a generator, there are many ways. The first method is very simple, as long as a list generation type of []
change ()
, creates a generator:
>>> L = [x * x for x in range(10)]
>>> L [0, 1, 4, 9, 16, 25, 36, 49, 64, 81] >>> g = (x * x for x in range(10)) >>> g <generator object <genexpr> at 0x1022ef630>
Creating L
and g
differ only in the outermost layer []
and ()
, L
a list, and g
a generator.
We can directly print out each element of the list, but how we print out each element of the generator it?
If you want to print out one by one, by next()
obtaining a return value is a function of the generator:
>>> next(g)
0
>>> next(g)
1
>>> next(g) 4 >>> next(g) 9 >>> next(g) 16 >>> next(g) 25 >>> next(g) 36 >>> next(g) 49 >>> next(g) 64 >>> next(g) 81 >>> next(g) Traceback (most recent call last): File "<stdin>", line 1, in <module> StopIteration
We talked, generator algorithm is stored in each call next(g)
, will calculate the g
value of the next element until the calculated to the last element, when no more elements, throws StopIteration
an error.
Of course, this constant invocation above next(g)
is too sick, the correct approach is to use a for
loop because the generator is iterable:
>>> g = (x * x for x in range(10))
>>> for n in g: ... print(n) ... 0 1 4 9 16 25 36 49 64 81
So, we created after a generator, basically never called next()
, but through for
to the iterative loop it, and do not care about StopIteration
the error.
generator is very powerful. If the projection algorithm is complex, with a similar type of list generation for
time cycle can not be achieved, it can also be implemented as a function.
For example, the number of columns known Feibolaqi (the Fibonacci), except for the first and second number, any number can be obtained by the first two numbers together:
1, 1, 2, 3, 5, 8, 13, 21, 34, ...
Feibolaqi formula list by the number of columns to write, however, a function is very easy to print it out:
def fib(max):
n, a, b = 0, 0, 1 while n < max: print(b) a, b = b, a + b n = n + 1 return 'done'
Note that the assignment statement:
a, b = b, a + b
It is equivalent to:
t = (b, a + b) # t是一个tuple
a = t[0] b = t[1]
But you do not have to explicitly write the temporary variable t can be assigned.
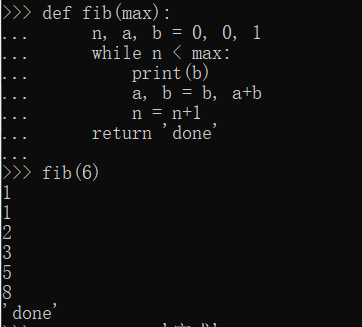