1, IO flow
The data different operations can be divided into:
1) byte stream: byte stream can operate any data, since any data in a computer is stored in the form of bytes
2) character stream: pure character stream operations only character data, more convenient.
According to the flow of points, they can be divided into
1) the input stream
2) the output stream
2, the byte stream
2.1 byte output stream
2.1.1 byte output stream OutputStream
OutputStream abstract class, all the output stream of bytes superclass. Data byte operations are, class defines the basic functionality common method output stream of bytes.
2.1.2 FileOurputStream class
That file output stream for writing data to the output stream File.
package com.Output;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import org.junit.Test;
public class FileOutputStreamDemo00 {
@Test
public void t1() throws IOException {
File f1=new File("test.txt");
//case1:实例化
//FileOutputStream fos=new FileOutputStream(f1);
//case2:直接接受字符串
FileOutputStream fos=new FileOutputStream("test.txt");
//FileOutputStream 具备向文件写入的能力
fos.write(97); //传入:a
//case3:数组写入
byte[] b= {98,99,100,101};
fos.write(b); //传入:bcde
//case4:输出byte选择的部分
fos.write(b,1,3); //传入cde
//关闭流,释放资源
fos.close();
}
}
2.1.3 in writing to the file and line feed
newFileOutputStream (file) to create an output stream object to write data to a file, it will overwrite the contents of the original file. Solution Use other constructors:
@Test
//使用追加内容方式国建输出流对象
public void t2() throws FileNotFoundException {
//true:需要追加
FileOutputStream fos=new FileOutputStream("test.txt",true);
//输出内容
try {
fos.write(97);
} catch (IOException e) {
e.printStackTrace();
}finally {
try {
if(fos!=null)
fos.close();
}catch(IOException e) {
e.printStackTrace();
}
}
}
2.2 input stream of bytes
2.2.1 input stream of bytes InputStream
The data file into memory
2.2.2 FileInputStream class
FileInputStream obtains input bytes from a file system in a file.
When reading data file, calls the read method to achieve read data from a file
package com.Input;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.util.Arrays;
import org.junit.Test;
public class FileInputStreamDemo00 {
public void t1() throws IOException {
File f=new File("test.txt");
//实例化对象
FileInputStream fis=new FileInputStream(f);
//case1: read() 每次只读一个字节
System.out.println(fis.read()); //97
//一次性全部读取
int n=0;
while((n = fis.read()) != -1) {
System.out.print((char)n+" ");
}
}
@Test
public void t2() throws IOException {
File f=new File("test.txt");
//实例化对象
FileInputStream fis=new FileInputStream(f);
//case2: 读取byte[] 10:来确定读取的长度
byte[] b=new byte[10];
//fis.read(b);
//System.out.println(Arrays.toString(b)); //[98, 99, 100, 101, 99, 100, 101, 97, 0, 0]
//一次性读取
byte[] b1=new byte[3];
int count=fis.read(b1);
while(count!=-1) {
for(int i=0;i<count;i++) {
System.out.print(b1[i]+" "); //97 98 99 97 98 99
}
count=fis.read(b);//修改
}
//释放资源
fis.close();
}
}
2.3 Copying Files
A stream of bytes to copy files
package com.Input;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
/**
* 文件复制
* @author Administrator
*步骤1、将指定路径的目标文件内容读取到内存中
*2、将内存中的写入另一个同名文件
*缺点:效率低,一个字节一个字节度
*/
public class Demo {
public static void main(String[] args) {
//1、实例化输入输出流
FileInputStream fis=null;
FileOutputStream fos=null;
try {
fis=new FileInputStream("G:\\demo1.txt");
fos=new FileOutputStream("demo1.txt");
//调用输入流read()把握目标读取到内存中
int n=0;
while((n=fis.read())!=-1) {
fos.write(n);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}finally {
try {
if( fis!=null)
fis.close();
if(fos!=null)
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
Buffer array way to copy files
package com.Input;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
/**
* 文件复制
* @author Administrator
*步骤1、将指定路径的目标文件内容读取到内存中
*2、将内存中的写入另一个同名文件
*/
public class Demo00 {
public static void main(String[] args) {
//1、实例化
FileInputStream fis=null;
FileOutputStream fos=null;
try {
fis=new FileInputStream("G:\\demo1.txt");
fos=new FileOutputStream("demo1.txt");
//复制2、创建数组
byte[] b=new byte[1024];
int n=0;
while((n=fis.read(b))!=-1) {
fos.write(b,0,n);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}finally {
if(fis!=null) {
try {
if( fis!=null)
fis.close();
if(fos!=null)
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
3. coding
package com.编码;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.util.Arrays;
public class Demo00 {
public static void main(String[] args) throws IOException {
//文件中写入内容,默认编码GBK
writeText();
//读取文件中的内容,默认编码
readText();
//文件写入编码,指定编码 utf-8
writeTextEncoding();
readTextEncoding();
}
private static void readTextEncoding() throws IOException {
FileInputStream fis=new FileInputStream("2.txt");
byte[] bytes=new byte[12];
int n=fis.read(bytes);
System.out.println(Arrays.toString(bytes)); //[-28, -67, -96, -27, -91, -67, 0, 0, 0, 0, 0, 0]
//解码
String str=new String(bytes,"utf-8");
System.out.println(str); //你好
}
private static void writeTextEncoding() throws FileNotFoundException, IOException {
FileOutputStream fos=new FileOutputStream("2.txt");
//编码
byte[] bytes="你好".getBytes("utf-8");
fos.write(bytes);
fos.close();
}
//写入内容
private static void writeText() throws IOException {
FileOutputStream fos=new FileOutputStream("1.txt");
fos.write("你好吗?".getBytes());
fos.close();
}
//读取
private static void readText() throws IOException {
FileInputStream fis=new FileInputStream("1.txt");
int b=0;
while((b=fis.read())!=-1) {
System.out.print(b+" "); //输出默认编码:196 227 186 195 194 240 163 191
}
}
}
Code: source object content into a standard format of the content in accordance with a standard.
Decoding: using the same encoding standard and encoded content is reduced to the original target content.
4, character-input stream Reader
Normal view text file, you must use the same character set and save the file when you open the file.
4.1 FileRead Deliverable
package com.FileReader类;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.FileReader;
import java.io.IOException;
public class Demo00 {
public static void main(String[] args) throws IOException {
//写入中文 -字节码的方式写入
writeText();
//读取中文 -字符流的方式
readText();
}
private static void readText() throws IOException {
FileReader fr=new FileReader("3.txt");
int ch=0;
while((ch=fr.read())!=-1) {
//将编码转为中文
System.out.print((char)ch);
}
}
private static void writeText() throws IOException {
FileOutputStream fos=new FileOutputStream("3.txt");
fos.write("好棒的人类啊".getBytes());
fos.close();
}
}
5, the output character stream Writer
5.1 FileWriter class
package com.FileReader类;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
public class Demo00 {
public static void main(String[] args) throws IOException {
//写入中文 -字节码的方式写入
//writeText();
//字符流的方式写入
writeTextByWrite();
//读取中文 -字符流的方式
readText();
}
//字符流的方式写入
private static void writeTextByWrite() throws IOException {
FileWriter fw=new FileWriter("3.txt");
fw.write("你现在在吃饭是吗");
fw.close();
//fw.flush();
}
private static void readText() throws IOException {
FileReader fr=new FileReader("3.txt");
int ch=0;
while((ch=fr.read())!=-1) {
//将编码转为中文
System.out.print((char)ch);
}
}
// private static void writeText() throws IOException {
// FileOutputStream fos=new FileOutputStream("3.txt");
// fos.write("好棒的人类啊".getBytes());
// fos.close();
// }
}
The difference between flush () and close () of
6, copy the text file character stream
package com.FileReader类;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import org.junit.Test;
public class Test00 {
@Test
public void t1() throws IOException {
//构建字符流
FileReader fr=new FileReader("3.txt");
FileWriter fw=new FileWriter("test.txt");
//复制
//way1:效率低
// int len=0;
// while((len=fr.read())!=-1) {
// fw.write(len);
// }
//way2:数组
char[] chars=new char[1024];
int l=0;
while((l=fr.read(chars))!=-1) {
fw.write(chars, 0, l);
}
fr.close();
fw.close();
}
}
package com.FileReader类;
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.IOException;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Set;
import org.junit.Test;
/**
*
* @author Administrator
*集合中的数据存储到文件
定义一个 Map 集合,里面包含下列数据:
摩卡 30
卡布奇诺 27
拿铁 27
香草拿铁 30
*/
public class Test01 {
@Test
public void t1() throws IOException {
Map<String,Integer> map=new LinkedHashMap<>();
map.put("摩卡",30);
map.put("卡布奇诺",27);
map.put("拿铁", 27);
map.put("香草拿铁 ",30);
//2.将集合按格式存入
BufferedWriter fw=new BufferedWriter(new FileWriter("test1.txt"));
Set<String> keys=map.keySet();
for(String key:keys) {
fw.write(key.toString() + "=" + map.get(key));
fw.write("\r\n");
}
fw.close();
}
}
7, commutations
7.1 .OutputStreamWriter
Converted into a string of bytes according to the specified code tables, and then use these bytes to write the byte stream out.
package com.转换流;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.io.OutputStreamWriter;
import java.io.UnsupportedEncodingException;
import org.junit.Test;
public class Demo {
@Test
//将字符串按照指定的编码表转成字节,再使用字节流将这些字节写出去。
public void t1() throws IOException {
//创建输出流对象
FileOutputStream fos=new FileOutputStream("5.txt");
//创建转换流对象,将字符转换成字节,并制定编码
OutputStreamWriter osw=new OutputStreamWriter(fos,"utf-8");
//使用转换流提供的方法写出字符
osw.write("转换流");
osw.close();
}
}
7.2 InputStreamReader
@Test
public void t2() throws IOException {
//创建输入流对象,关联文件
FileInputStream fis=new FileInputStream("5.txt");
//使用转换流进行转换
InputStreamReader isr=new InputStreamReader(fis,"utf-8");
int ch=0;
while((ch=isr.read())!=-1) {
System.out.println((char)ch);
}
}
8, the buffer flow
You can increase the speed of read and write IO stream
8.1 byte stream buffer
8.1.1 字节缓冲输出流 BufferedOutputStream 和 字节缓冲输入流 BufferedInputStream
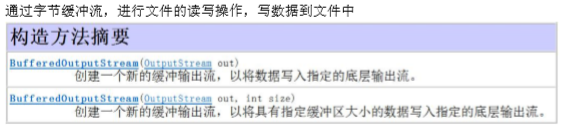
package com.缓冲流;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import org.junit.Test;
public class Demo00 {
@Test
public void t1() throws IOException {
//构建字节流对象
FileOutputStream fos=new FileOutputStream("6.txt");
//国建缓冲流对象,将字节流对象传入
BufferedOutputStream bos=new BufferedOutputStream(fos);
//使用缓冲流写数据
bos.write("dasdaa".getBytes());
bos.close();
fos.close();
}
@Test
public void t2() throws IOException {
//构建输入流,关联文件
FileInputStream fis=new FileInputStream("6.txt");
//构建缓冲流对象
BufferedInputStream bis=new BufferedInputStream(fis);
int ch=0;
while((ch=bis.read())!=-1) {
System.out.println((char)ch);
}
bis.close();
fis.close();
}
}
8.2 字符缓冲流
8.2.1 字符缓冲输入流 BufferedReader 和 字符缓冲输入流 BufferedReader
BufferedReader新增:
BufferedReader:
package com.缓冲流;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import org.junit.Test;
public class Demo01 {
@Test
public void t1() throws IOException {
//构建输出流
FileWriter fw=new FileWriter("7.txt");
//实例化缓冲流对象,将基本输入流传入
BufferedWriter bw=new BufferedWriter(fw);
bw.write("hello world");
bw.newLine();
bw.write("hello");
//关闭
bw.close();
}
@Test
public void t2() throws IOException {
//创建输入流
FileReader fr=new FileReader("7.txt");
//传入缓冲流,传入基本字符输入流对象
BufferedReader br=new BufferedReader(fr);
//使用缓冲流读取
String str=null;
while((str=br.readLine())!=null) {
System.out.println(str);
}
}
}
例题:
package com.缓冲流;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
/**
* 缓冲流复制文件
* @author Administrator
*
*/
public class Test00 {
public static void main(String[] args) throws IOException {
//使用基本流一次复制一个字符
//t1();
//使用基本流一次复制一个数组
//t2();
//使用缓冲流一次复制一个字符
//t3();
//使用缓冲流一次复制一个数组
t4();
}
private static void t4() throws IOException {
//构建缓冲流
BufferedReader br=new BufferedReader(new FileReader("8.txt"));
BufferedWriter bw=new BufferedWriter(new FileWriter("copy8.txt"));
String str=null;
while((str=br.readLine())!=null) {
bw.write(str);
bw.newLine();
}
bw.close();
br.close();
}
private static void t3() throws IOException {
//构建缓冲流
BufferedReader br=new BufferedReader(new FileReader("8.txt"));
BufferedWriter bw=new BufferedWriter(new FileWriter("copy8.txt"));
int len=0;
while((len=br.read())!=-1) {
bw.write(len);
}
bw.close();
br.close();
}
private static void t2() throws IOException {
//构建输入流
FileReader fr=new FileReader("8.txt");
FileWriter fw=new FileWriter("copy8.txt");
int b=0;
char[] ch=new char[1024];
while((b=fr.read(ch))!=-1) {
fw.write(ch, 0, b);
}
fw.close();
fr.close();
}
private static void t1() throws IOException {
//构建输入流
FileReader fr=new FileReader("8.txt");
FileWriter fw=new FileWriter("copy8.txt");
//复制,一次复制一个
int len=0;
while((len=fr.read())!=-1) {
fw.write(len);
}
fw.close();
fr.close();
}
}
9.其他流
9.1 对象序列化流
序列化:是指将一个"对象(包含属性值)"存储到一个文件中,或者通过网络进行传输
构造方法:ObjectOutputStream(OutputStreamout)
序列化的方法:
void writeObject(Objectobj) :将指定的对象写入 ObjectOutputStream 注意:当某个对象需要被"序列化"时,此类必须实现:Serializable(接口)
反序列化:是指将一个文本文件转成一个 Java 对象
类介绍:ObjectInputStream
构造方法 ObjectInputStream(InputStreamin)
反序列化的方法 ObjectreadObject() :从 ObjectInputStream 读取对象。
package com.其他流;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import org.junit.Test;
public class Demo {
@Test
public void t1() throws IOException, ClassNotFoundException {
Student stu=new Student("xixi",12);
//构建对象序列化流对象
ObjectOutputStream oos=new ObjectOutputStream(new FileOutputStream("ot.txt"));
oos.writeObject(stu);
oos.close();
System.out.println("写入成功");
//反序列化
ObjectInputStream ois=new ObjectInputStream(new FileInputStream("ot.txt"));
Object obj=ois.readObject();
Student stu1=(Student)obj;
System.out.println(stu1);
}
}