Fragment creation
- Creating a layout for the fragment
- Define a class that inherits fragment
- Rewrite oncreatView method
Fragment class definitions
Frament.java
public class MyFragment extends Fragment {
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
View view = View.inflate(getContext(), R.layout.fragment_layout, null);
return view;
}
}
fragment_layout.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="This is a Fragment"/>
</LinearLayout>
XML directly introduced
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<fragment
android:id="@+id/one_frament_one"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:name="com.godc.fragmenttest.MyFragment"/>
</LinearLayout>
Introduced in Java code
FragmentManager fragmentManager = getSupportFragmentManager();
FragmentTransaction transaction = fragmentManager.beginTransaction();
MyFragment myFragment = new MyFragment();
/* *
*参数1:Fragment容器id
* 参数2:Fragment
* 参数3:给添加的Fragment设置标签名
*/
transaction.add(R.id.one_frament_one, myFragment, "FragmentB");
transaction.commit();
effect
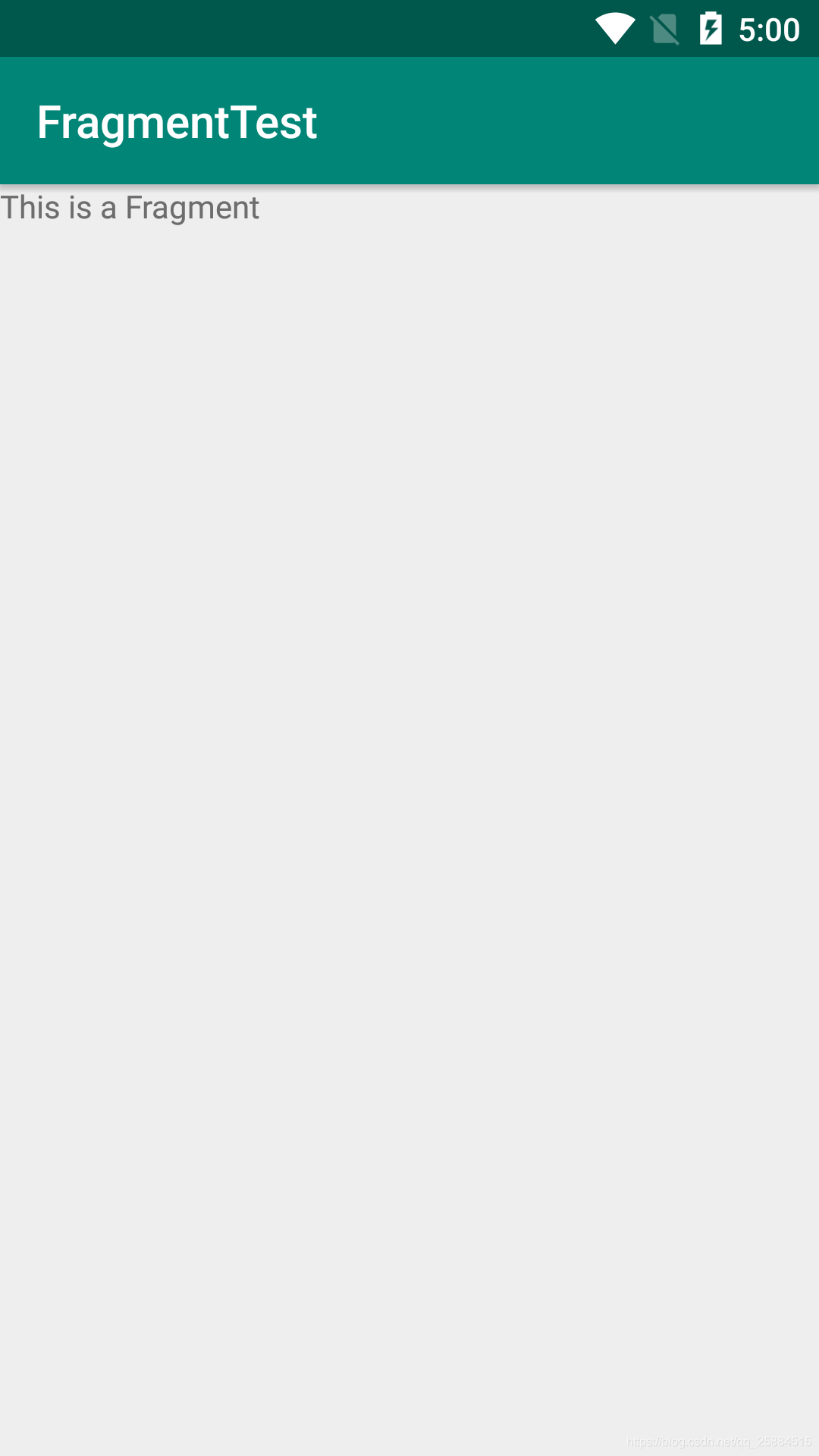
to sum up
Fragment life cycle
Life-cycle approach introduced
method | meaning |
---|---|
onAttach() | When the association's activities and calls Fragment |
onCreate() | When the call is established Fragment |
onCreateView() | Fragment call is create a view |
onActivityCreated() | Called when the activity associated with the Fragment has been created |
onDestroyView () | When Fragment management views are removed call |
onDetach() | When Fragment and call activity disassociated |
FragmentTransaction method summary
method | meaning | Life Cycle call |
---|---|---|
add(id, fragment) | Fragment was added to a queue | A fragment can not be added twice |
show(fragment) | Add a fragment | When the fragment is not present in the queue, the callback onAttach (), onCreate (). When the presence of the fragment in the queue and call through the hide (fragment), a callback onHiddenChange (boolean). Other cases no callback function. |
replace(id,fragment) | Queue already exists in crash, will enter the queue does not exist and the other fragment and clear the queue, the last show of the fragment to the specified layout. | 同add(id, fragment) |
remove(fragment) | The destruction of the queue specified fragment | When the fragment is not present in the queue, nothing happens. When the fragment is present in the queue, the life cycle of the implementation depends mainly on whether the current fragment into the fragment return stack. |
hide(fragment) | Hide queue specified fragment, equivalent to calling view .setVisibility (View.GONE) | 当队列中存在该fragment时,回调onHiddenChange(boolen)当队列中不存在该fragment时,回调onAttach()、onCreate()、onHiddenChange(boolen)。 |
detach(fragment) | 销毁指定frament的视图,并且该fragment的onCreateVieew(……)不能再被调用(除非调用attach(fragment)重新连接) | 当队列中存在该fragment时,回调onDestroyView()当队列中不存在该fragment时,回调onAttach()、onCreate()。 |
attach(fragment) | 创建指定fragment的视图。标识该fragment的onCreateView(……)能被调用。 | 当队列中存在该fragment时且被调用detach(fragment)时,回调createView()、onActivityCreated()、onResume()。当队列中不存在该fragment时,回调onAttach()、onCreate()。 |
addToBackStack(string) | 使本次事务增加的fragment进入当前activity的返回栈中。当前参数是对返回栈的描述,没什么实际用途。传入null即可。 | |
commit() | 提交本次事务,可在非主线程中被调用。主要用于多线程处理情况。 | |
commitNow() | 提交本次事务,只在主线程中被调用。 这时候addToBackStack(string)不可用。 |