Refer to the official document: https:? //Selenium.dev/selenium/docs/api/py/webdriver_support/selenium.webdriver.support.select.html highlight = all_selected_options # selenium.webdriver.support.select.Select.all_selected_options
An import (Import)
from selenium.webdriver.support.select import Select
Second, choose (select)
Select class provides three methods to select an option:
select_by_index(index)
select_by_value(value)
select_by_visible_text(text)
note:
- index from 0 to start
- value is the value of a property option label, not the value displayed in the drop-down box
- visible_text option tag is in the middle value, the value is displayed in the drop box
Third, anti-election (deselect)
Selectively bound to invert the selection and cancels selection. Select offers four methods to cancel our original choice:
deselect_by_index(index)
deselect_by_value(value)
deselect_by_visible_text(text)
deselect_all()
The first three correspond respectively to select, the fourth is the abolition of all options, yes, you're not wrong, it is canceled.
There is a special select tag, i.e. set select multiple = "multiple" attribute, which can be a multi-select box is selected,
By repeatedly select, select a number of options, and by deselect_all () to cancel them all.
Note: the following two, the same intention of
# Return the first or sub-item currently selected
print(Select(driver.find_element_by_xpath("//select[@id='nr']")).first_selected_option.text)
# Currently selected output of the first item, i.e., the second display
now the Select = (driver.find_element_by_css_selector ( "body> form> SELECT")). First_selected_option
Print (now.text)
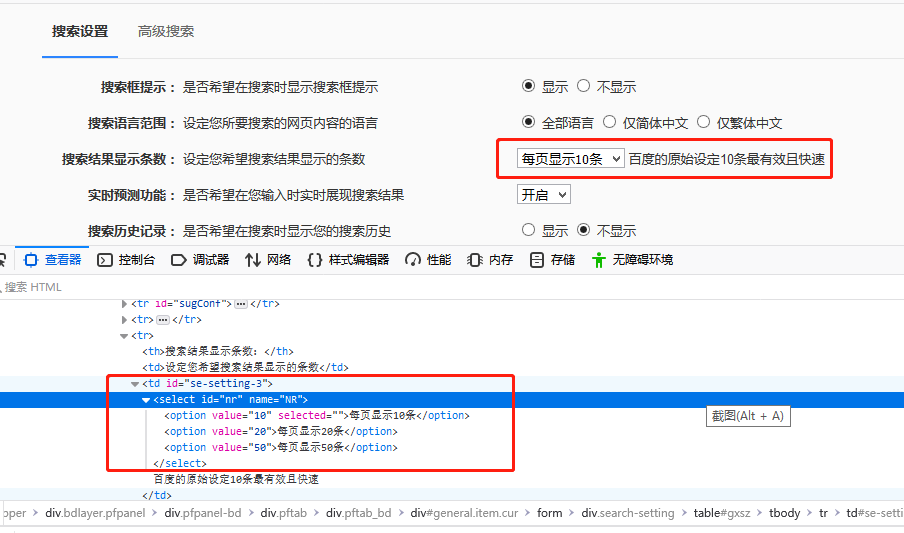
================================================================================
from selenium import webdriver
from selenium.webdriver.common.action_chains import ActionChains
#from selenium.webdriver.support.select import Select
from selenium.webdriver.support.ui import Select
from time import sleep
= webdriver.Firefox Driver ()
driver.implicitly_wait (10)
driver.get ( 'http://www.baidu.com')
# -------------------- -------------------------------------------------- ----------
Link driver.find_element_by_link_text = ( "settings")
ActionChains (Driver) .move_to_element (Link) .perform () # hover above
SLEEP (. 5)
driver.find_element_by_class_name ( "setpref" ) .click () # open the Search settings
SLEEP (2)
# ------------------------------------ -------------------------------------
SLEEP (. 3)
Print ( 'display the second sub-items ( 2): ')
. the select (driver.find_element_by_xpath ( "sELECT // [@ ID =' NR ']")) select_by_index (. 1) # options selected second child, 20 per
print (Select (driver.find_element_by_xpath ( "// select [@ id = 'nr']")). first_selected_option.text) # Returns the first child or currently selected item
SLEEP (. 3)
Print ( 'third display sub-item (50): ')
the select (driver.find_element_by_xpath ( "sELECT // [@ ID =" NR']. ")) select_by_value ('50 ') # select value is' 50 per page' child that the third child of the selected
print (select (driver.find_element_by_xpath ( "// select [@ id = 'nr']")). first_selected_option.text) # returns the first child or currently selected item
sleep (3 )
Print ( 'Show the second child (20 is):')
the select (driver.find_element_by_xpath ( "sELECT // [@ ID =" NR '] ")) select_by_visible_text (' 20 per ') # selected text. value '20 per' sub-item
print (Select (driver.find_element_by_xpath ( "// select [@ id = 'nr']")). first_selected_option.text) # Returns the first child or currently selected item
SLEEP (. 3)
Print ( 'Show current child (the second child 20 selected):')
print (Select (driver.find_element_by_xpath ( "// select [@ id = 'nr']")). first_selected_option.text) # returns to the first or the currently selected page displayed child # 20
# --- -------------------------------------------------- --------------------------------
= driver.find_ Options Elements _by_xpath ( '// * [@ ID = "NR"] / Option')
Options [. 1] .click () # the second sub-item is selected
SLEEP (2)
Options [2] .click ( ) # third sub-item is selected
SLEEP (2)
Options [0] .click () # first sub-item is selected
SLEEP (2)
# ----------------- -------------------------------------------------- ----
Print (Options [0] .text)
Print (Options [. 1] .text)
Print (Options [2] .text)
#-----------------------------------------------------------------------
driver.quit()
Displaying the second sub-item (2):
20 per
display the third sub-items (50):
Showing 50
to display the second sub-items (20):
20 per
subkeys currently selected ( The second sub-item 20):
20 per page
10 per
page shows 20
per page 50
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
===============================================================================================================
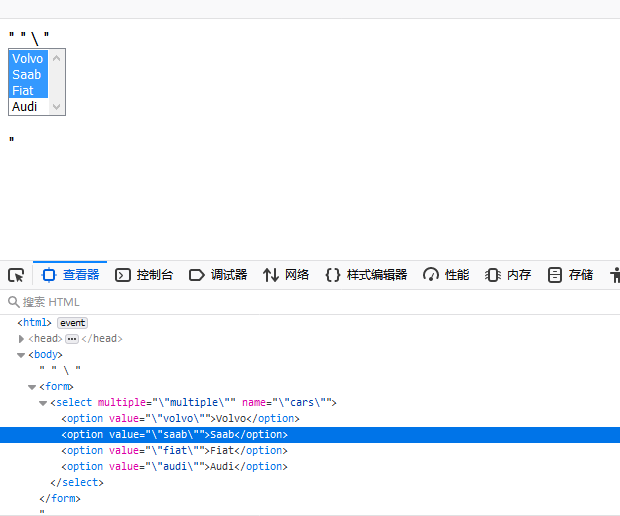
from selenium import webdriver
from selenium.webdriver.support.ui import Select
from time import sleep
driver = webdriver.Firefox()
driver.get("https://www.runoob.com/runcode") # 打开浏览器,进入演示页面
driver.find_element_by_css_selector("#codeinp").clear() # 定位输入框文本域 # 清空文本域
# 输入多选下拉框的演示源码 (multiple="multiple\")
texts = "<html> " \
"<body><form><select multiple=\"multiple\" name=\"cars\"><option value=\"volvo\">Volvo</option>" \
"<option value=\"saab\">Saab</option><option value=\"fiat\">Fiat</option>\" \
\"<option value=\"audi\">Audi</option></select></form></body></html>"
driver.find_element_by_css_selector("#codeinp").send_keys(texts)
driver.find_element_by_css_selector("#btrun").click() # 点击提交代码
sleep(2)
#--------------------------------------------------------------------------------------------------------
all_handles = driver.window_handles
driver.switch_to.window(all_handles[1]) #切换到新的打开的运行页面
#-------------------------------------------------------------------------------------------------------
# 选择全部的选项(多选),一个接着一个选择,直到全部选择
Select(driver.find_element_by_css_selector("body > form > select")).select_by_index(0)
sleep(1)
Select(driver.find_element_by_css_selector("body > form > select")).select_by_index(1)
sleep(1)
Select(driver.find_element_by_css_selector("body > form > select")).select_by_index(2)
sleep(1)
Select(driver.find_element_by_css_selector("body > form > select")).select_by_index(3)
sleep(1)
#----------------------------------------------------------------------------------------------------------------
# 取消选择第一项选项(页面上可以观察到变化)
Select(driver.find_element_by_css_selector("body > form > select")).deselect_by_index(0)
sleep(1)
# 输出当前选择的第一项,即显示第二个
now = Select(driver.find_element_by_css_selector("body > form > select")).first_selected_option
print(now.text)
#取消第二个选项
#Select(driver.find_element_by_css_selector("body > form > select")).deselect_by_value('\"saab\"')
Select(driver.find_element_by_css_selector("body > form > select")).deselect_by_visible_text('Saab')
sleep(3)
#即取消三、四选项
Select(driver.find_element_by_css_selector("body > form > select")).deselect_all() #取消所有选项
sleep(3)
#---------------------------------------------------------------------------------------------------------
driver.close()