- I. Overview
- Second, the custom binding using the property editor
- Third, the use of custom parameter binding converter
I. Overview
1.3 parameter binding process
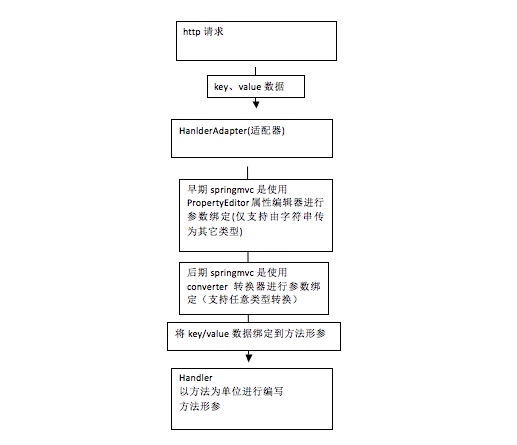
1.2 @RequestParam
- If the shape parameter name parameter controller REQUEST request and consistent method name, parameters automatically adapter binding. If not specified parameter name can be requested by the request to which method @RequestParam bound parameter on.
- For the parameters must pass, required by the property @RequestParam set to true, if you do not pass this parameter error.
For some parameters, if not passed, also you need to set the default value, use the property @RequestParam defaultvalue set the default value.
You can bind simple types: integer, string, single precision / double precision, date, boolean.
Simple types can be bound pojo
- Simple pojo types include only a simple type of property.
- The binding process: attribute name parameter name and pojo the request of the same request, it can be bound to succeed.
problem:
- If the controller has a plurality of parameter method and pojo pojo has duplicate properties, simple binding pojo not targeted binding,
- For example: The method of parameter items and has User, pojo simultaneous presence attribute name, HTTP request from the name of the process can not be bound to the targeted items or user.
Second, the custom binding using the property editor
- springmvc did not provide default binding type of date, you need a custom date type of binding.
2.1 WebDataBinder (understand)
- Class is defined in the controller:
//自定义属性编辑器
// @InitBinder
// public void initBinder(WebDataBinder binder) throws Exception {
// // Date.class必须是与controler方法形参pojo属性一致的date类型,这里是java.util.Date
// binder.registerCustomEditor(Date.class, new CustomDateEditor(
// new SimpleDateFormat("yyyy-MM-dd HH-mm-ss"), true));
// }
- Using this approach is not common to a plurality of controller.
2.2 WebBindingInitializer (understand)
- Let WebBindingInitializer controller using a plurality of common property editor.
- Custom WebBindingInitializer, injected into the processor adapter.
- If you want more controller need to work together to register the same property editor, you can achieve PropertyEditorRegistrar interface and inject webBindingInitializer in.
public class CustomPropertyEditor implements PropertyEditorRegistrar {
@Override
public void registerCustomEditors(PropertyEditorRegistry binder) {
binder.registerCustomEditor(Date.class, new CustomDateEditor(
new SimpleDateFormat("yyyy-MM-dd HH-mm-ss"), true));
}
}
- Configuration is as follows:
<!-- 注册属性编辑器 -->
<!-- 注册属性编辑器 -->
<bean id="customPropertyEditor"
class="com.hao.ssm.controller.propertyeditor.CustomPropertyEditor"></bean>
<!-- 自定义webBinder -->
<bean id="customBinder"
class="org.springframework.web.bind.support.ConfigurableWebBindingInitializer">
<property name="propertyEditorRegistrars">
<list>
<ref bean="customPropertyEditor"/>
</list>
</property>
</bean>
<!--注解适配器 -->
<bean
class="org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerAdapter">
<property name="webBindingInitializer" ref="customBinder"></property>
</bean>
Third, the use of custom parameter binding converter
3.1 implement the Converter interface
- Define the date string type converter and the converter space before and after removal.
public class CustomDateConverter implements Converter<String, Date> {
@Override
public Date convert(String source) {
try {
//进行日期转换
return new SimpleDateFormat("yyyy-MM-dd HH:mm:ss").parse(source);
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
}
public class StringTrimConverter implements Converter<String, String> {
@Override
public String convert(String source) {
try {
//去掉字符串两边空格,如果去除后为空设置为null
if(source!=null){
source = source.trim();
if(source.equals("")){
return null;
}
}
} catch (Exception e) {
e.printStackTrace();
}
return source;
}
}
3.2 Configuration converter
<!-- 转换器 -->
<bean id="conversionService"
class="org.springframework.format.support.FormattingConversionServiceFactoryBean">
<property name="converters">
<list>
<bean class="com.hao.ssm.controller.converter.CustomDateConverter" />
<bean class="com.hao.ssm.controller.converter.StringTrimConverter" />
</list>
</property>
</bean>