Introduction:
Spring Boot committed to rapid application development booming (rapid application development) to be a leader.
Features:
I recommend you to build springboot project with idea, I am using the idea, so I used the idea to build the next project
First, create a project
After filling out Group and Atrifact click Next, here I chose the jar, because official documentation recommends that packaged into JAR, so there is not too much to explain.
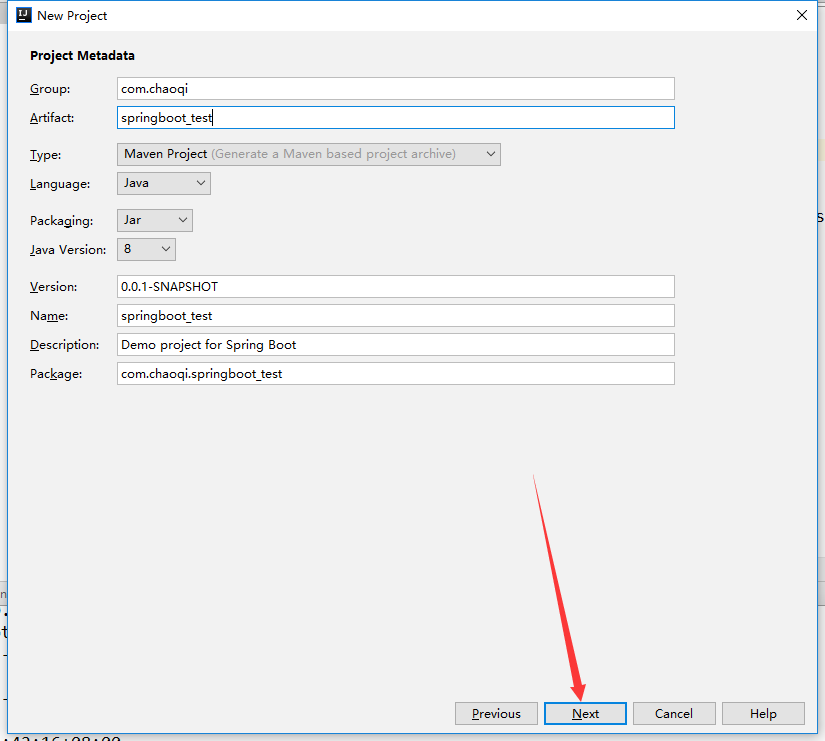
Here check on the web
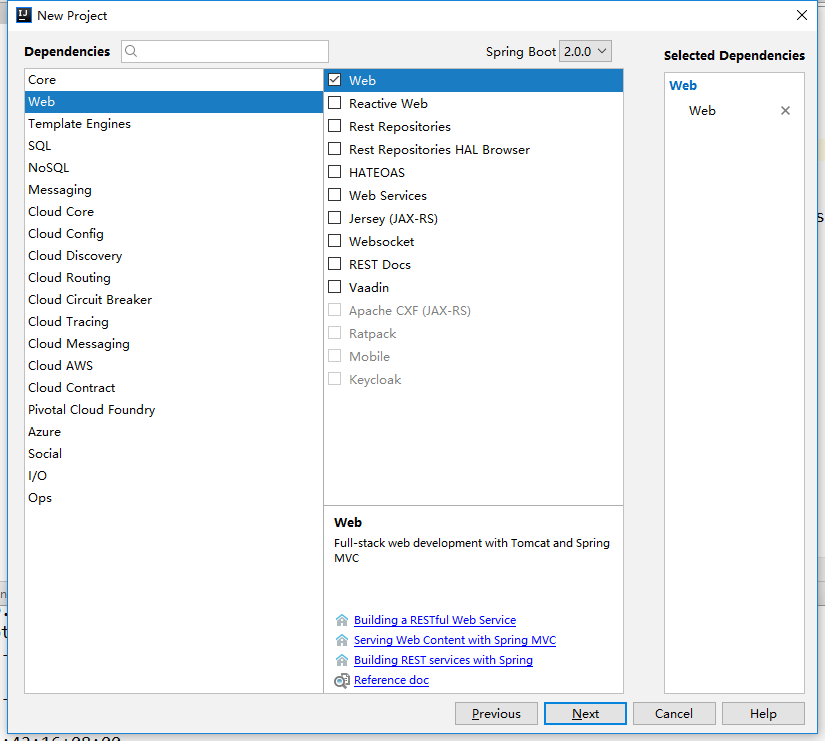
Check here on MySQL, JDBC and click Next Mybatis
Enter here the name of the project after the project path and click Finish
This is a new structure after the completion of the project
Second, add pom.xml dependence
Because springboot do not recommend the use of jsp page, depending on if you want to use to add jsp necessary for
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.chaoqi</groupId> <artifactId>springboot_demo2</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>springboot_demo2</name> <description>Demo project for Spring Boot</description> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.0.0.RELEASE</version> <relativePath/> </parent> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-jdbc</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.mybatis.spring.boot</groupId> <artifactId>mybatis-spring-boot-starter</artifactId> <version>1.3.2</version> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <scope>runtime</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <!--添加jsp依赖 --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-tomcat</artifactId> </dependency> <dependency> <groupId>org.apache.tomcat.embed</groupId> <artifactId>tomcat-embed-jasper</artifactId> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
三、springboot整合springmvc
因为在创建项目的时候我们选择了mybatis和jdbc所以在这里也要把他们两也给配置进去
编辑application.properties
# 页面默认前缀目录
spring.mvc.view.prefix=/WEB-INF/jsp/
# 响应页面默认后缀
spring.mvc.view.suffix=.jsp
#开发配置
spring.datasource.driverClassName = com.mysql.jdbc.Driver
spring.datasource.url = jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=utf-8
spring.datasource.username = root
spring.datasource.password = 123456
# mybatis接口文件位置
mybatis.mapper-locations: classpath:mapper/*.xml
mybatis.type-aliases-package: com.chaoqi.springboot_demo2.domain
如果大家习惯用application.yml那也可以用,但是用application.yml在第一次启动项目的时候一定要maven clean一下,不然会报错。
server: port: 8080 spring: mvc: view: prefix: /WEB-INF/jsp/ suffix: .jsp datasource: url: jdbc:mysql://localhost:3306/test?characterEncoding=UTF-8&useUnicode=true&useSSL=false username: root password: 123456 driver-class-name: com.mysql.jdbc.Driver mybatis: mapper-locations: classpath:mapping/*.xml type-aliases-package: com.chaoqi.springboot_demo2.domain
编辑完application.properties之后再src/mian下创建webapp目录,结构如下
新建IndexController
package com.chaoqi.springboot_test.web; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; @Controller public class IndexController { private static final String INDEX = "index"; @RequestMapping("/show") public String getIndex() { return INDEX; } }
运行main函数
访问页面,成功
四、springboot整合mybatis
创建数据库表
-- ---------------------------- -- Table structure for music_info -- ---------------------------- DROP TABLE IF EXISTS `music_info`; CREATE TABLE `music_info` ( `id` int(10) NOT NULL AUTO_INCREMENT COMMENT '主键id', `singer_name` varchar(100) NOT NULL COMMENT '歌手名', `music_size` varchar(100) NOT NULL COMMENT '歌曲大小', `music_name` varchar(100) NOT NULL COMMENT '歌曲名', PRIMARY KEY (`id`) ) ENGINE=InnoDB AUTO_INCREMENT=4 DEFAULT CHARSET=utf8; -- ---------------------------- -- Records of music_info -- ---------------------------- INSERT INTO `music_info` VALUES ('1', '小三', '3.2M', '起风了'); INSERT INTO `music_info` VALUES ('2', '刘德华', '3.0M', '忘情水'); INSERT INTO `music_info` VALUES ('3', '猪点点', '5.0M', '会写程序的小猪');
创建pojo
package com.chaoqi.springboot_test.dao.domain; public class MusicInfo { // 主键id private Integer id; // 歌手名 private String singerName; // 歌曲大小 private String musicSize; // 歌曲名 private String musicName; /** * 获取 主键id music_info.id * * @return 主键id */ public Integer getId() { return id; } /** * 设置 主键id music_info.id * * @param id 主键id */ public void setId(Integer id) { this.id = id; } /** * 获取 歌手名 music_info.singer_name * * @return 歌手名 */ public String getSingerName() { return singerName; } /** * 设置 歌手名 music_info.singer_name * * @param singerName 歌手名 */ public void setSingerName(String singerName) { this.singerName = singerName == null ? null : singerName.trim(); } /** * 获取 歌曲大小 music_info.music_size * * @return 歌曲大小 */ public String getMusicSize() { return musicSize; } /** * 设置 歌曲大小 music_info.music_size * * @param musicSize 歌曲大小 */ public void setMusicSize(String musicSize) { this.musicSize = musicSize == null ? null : musicSize.trim(); } /** * 获取 歌曲名 music_info.music_name * * @return 歌曲名 */ public String getMusicName() { return musicName; } /** * 设置 歌曲名 music_info.music_name * * @param musicName 歌曲名 */ public void setMusicName(String musicName) { this.musicName = musicName == null ? null : musicName.trim(); } @Override public String toString() { return "MusicInfo{" + "id=" + id + ", singerName='" + singerName + '\'' + ", musicSize='" + musicSize + '\'' + ", musicName='" + musicName + '\'' + '}'; } }
创建mapper.xml
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.chaoqi.springboot_test.dao.mapper.MusicInfoMapper"> <resultMap id="BaseResultMap" type="com.chaoqi.springboot_test.dao.domain.MusicInfo"> <id column="id" jdbcType="INTEGER" property="id" /> <result column="singer_name" jdbcType="VARCHAR" property="singerName" /> <result column="music_size" jdbcType="VARCHAR" property="musicSize" /> <result column="music_name" jdbcType="VARCHAR" property="musicName" /> </resultMap> </mapper>
创建mapper
package com.chaoqi.springboot_test.dao.mapper; import com.chaoqi.springboot_test.dao.domain.MusicInfo; import org.apache.ibatis.annotations.ResultMap; import org.apache.ibatis.annotations.Select; import java.util.List; public interface MusicInfoMapper { @ResultMap("BaseResultMap") @Select("select * from music_info") List<MusicInfo> selectAll(MusicInfo musicInfo); }
service接口
package com.chaoqi.springboot_test.service; import com.chaoqi.springboot_test.dao.domain.MusicInfo; import java.util.List; public interface MusicInfoService { public List<MusicInfo> getMusicInfo(MusicInfo musicInfo); }
service实现类
package com.chaoqi.springboot_test.service.impl; import com.chaoqi.springboot_test.dao.domain.MusicInfo; import com.chaoqi.springboot_test.dao.mapper.MusicInfoMapper; import com.chaoqi.springboot_test.service.MusicInfoService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import java.util.List; @Service public class MusicInfoServiceImpl implements MusicInfoService { @Autowired private MusicInfoMapper musicInfoMapper; @Override public List<MusicInfo> getMusicInfo(MusicInfo musicInfo) { List<MusicInfo> musicInfos = musicInfoMapper.selectAll(null); return musicInfos; } }
创建完成后的结构如下
编辑indexController
package com.chaoqi.springboot_test.web; import com.chaoqi.springboot_test.dao.domain.MusicInfo; import com.chaoqi.springboot_test.service.MusicInfoService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.ResponseBody; import java.util.List; @Controller public class IndexController { private static final String INDEX = "index"; @Autowired private MusicInfoService musicInfoService; @RequestMapping("/show") public String getIndex() { return INDEX; } @RequestMapping("/music") @ResponseBody public List<MusicInfo> getMusicInfo(MusicInfo musicInfo) { List<MusicInfo> musicInfoList = musicInfoService.getMusicInfo(null); return musicInfoList; } }
给SpringbootTestApplication类加上注解@MapperScan("com.chaoqi.springboot_test.dao.mapper")
package com.chaoqi.springboot_test; import org.mybatis.spring.annotation.MapperScan; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; @SpringBootApplication @MapperScan("com.chaoqi.springboot_test.dao.mapper") public class SpringbootTestApplication { public static void main(String[] args) { SpringApplication.run(SpringbootTestApplication.class, args); } }
运行项目,成功,springboot+springmvc+mybatis整合完成(源码下载地址:https://github.com/caicahoqi/ChaoqiIsPrivateLibrary)