Programming ideas:
The first is the login page, registration page, and home page
Data may then be stored in the table, i.e. registered user can enter data in the graphics frame
Retrieve all the data in the back end, to see if there is data
Corresponding to randomly generated codes, and requires the input of the verification code display
Finally, to complete the login
Source:
package yanzhengma;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JPasswordField;
import javax.swing.JTextField;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JPasswordField;
import javax.swing.JTextField;
public class Interface extends JFrame {
private static final long serialVersionUID = 1L;
private static final long serialVersionUID = 1L;
private static String record;
public static void main(String[] args) {
new Interface();
}
new Interface();
}
Interface public () {
// create instance of JFrame
JFrame frame = new JFrame ( "please login:");
// create instance of JFrame
JFrame frame = new JFrame ( "please login:");
// 设置frame大小
frame.setLocation(500, 300);
frame.setSize(400, 200);
frame.setResizable(false);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLocation(500, 300);
frame.setSize(400, 200);
frame.setResizable(false);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Create a panel
JPanel panel = new JPanel ();
JPanel panel = new JPanel ();
// add panel
frame.add (panel);
frame.add (panel);
// Set the panel particular forms
placeComponents (panel);
placeComponents (panel);
// Set interface is visible
frame.setVisible (true);
frame.setVisible (true);
}
public void placeComponents(JPanel panel) {
// clear the panel
panel.setLayout (null);
panel.setLayout (null);
// Create user component
JLabel userLabel = new JLabel ( "User Name:");
userLabel.setBounds (10, 20 is, 80, 25);
panel.add (userLabel);
JLabel userLabel = new JLabel ( "User Name:");
userLabel.setBounds (10, 20 is, 80, 25);
panel.add (userLabel);
// Create a text field for the user to input
the JTextField = new new userText the JTextField (20 is);
userText.setBounds (100, 20 is, 165, 25);
panel.add (userText);
the JTextField = new new userText the JTextField (20 is);
userText.setBounds (100, 20 is, 165, 25);
panel.add (userText);
// create a password component
JLabel passwordLabel = new JLabel ( "Password:");
passwordLabel.setBounds (10, 50, 80, 25);
panel.add (passwordLabel);
JLabel passwordLabel = new JLabel ( "Password:");
passwordLabel.setBounds (10, 50, 80, 25);
panel.add (passwordLabel);
// Create a password text field
JPasswordField PasswordText = new new JPasswordField (20);
passwordText.setBounds (100, 50, 165, 25);
panel.add (PasswordText);
JPasswordField PasswordText = new new JPasswordField (20);
passwordText.setBounds (100, 50, 165, 25);
panel.add (PasswordText);
// Create the component verification code used
JLabel verificationLabel = new JLabel ( "verification code:");
verificationLabel.setBounds (10, 80, 80, 25);
panel.add (verificationLabel);
JLabel verificationLabel = new JLabel ( "verification code:");
verificationLabel.setBounds (10, 80, 80, 25);
panel.add (verificationLabel);
// Create a text field codes
the JTextField = new new verificationText the JTextField (20 is);
verificationText.setBounds (100, 80, 80, 25);
panel.add (verificationText);
the JTextField = new new verificationText the JTextField (20 is);
verificationText.setBounds (100, 80, 80, 25);
panel.add (verificationText);
// create a random string
String Result = "";
for (int I = 0; I <. 6; I ++) {
int intVal = (int) (Math.random () * 26 is + 97);
Result = Result + ( char) intVal;
}
Record = Result;
String Result = "";
for (int I = 0; I <. 6; I ++) {
int intVal = (int) (Math.random () * 26 is + 97);
Result = Result + ( char) intVal;
}
Record = Result;
// Create verification code display components used
the JLabel verificationShowLabel the JLabel new new = (Result);
verificationShowLabel.setBounds (200 is, 80, 80, 25);
panel.add (verificationShowLabel);
the JLabel verificationShowLabel the JLabel new new = (Result);
verificationShowLabel.setBounds (200 is, 80, 80, 25);
panel.add (verificationShowLabel);
// Create the login button
JButton loginButton = new JButton ( "login");
loginButton.setBounds (10, 120, 80, 25);
panel.add (loginButton);
JButton loginButton = new JButton ( "login");
loginButton.setBounds (10, 120, 80, 25);
panel.add (loginButton);
// button to monitor
the ActionListener = new new ourListener1 the ActionListener () {
public void the actionPerformed (the ActionEvent E) {
IF (e.getSource () == loginButton) // determines whether or not the login button click
{
IF (record.equalsIgnoreCase (verificationText.getText ())) // determines whether the correct verification code
{
iF (StoreroomManager.datelist.size () == 0) // determines whether there is data memory space
{
JOptionPane.showMessageDialog (null, "you have not registered");
userText. the setText ( "");
passwordText.setText ( "");
the ActionListener = new new ourListener1 the ActionListener () {
public void the actionPerformed (the ActionEvent E) {
IF (e.getSource () == loginButton) // determines whether or not the login button click
{
IF (record.equalsIgnoreCase (verificationText.getText ())) // determines whether the correct verification code
{
iF (StoreroomManager.datelist.size () == 0) // determines whether there is data memory space
{
JOptionPane.showMessageDialog (null, "you have not registered");
userText. the setText ( "");
passwordText.setText ( "");
} Else // existing assignment date, and retrieves whether there is a user registered
{
Storeroom Storeroom date = new new ();
date.setUser (userText.getText ()); // get the username entered by the user
date. setPassword (passwordText.getText ()); // get the password entered by the user
StoreroomManager.confirmDate (DATE);
passwordText.setText ( "");
verificationText.setText ( "");
}
} // this code is not correct the else
{
PasswordText .setText ( "");
verificationText.setText ( "");
JOptionPane.showMessageDialog (null, "code is incorrect, please re-enter");
// Create a random string
string Result = "";
for (int I = 0; i <6; i ++ ) {
int intVal = (int) (Math.random() * 26 + 97);
result = result + (char) intVal;
}
record = result;
verificationShowLabel.setText(result);
{
Storeroom Storeroom date = new new ();
date.setUser (userText.getText ()); // get the username entered by the user
date. setPassword (passwordText.getText ()); // get the password entered by the user
StoreroomManager.confirmDate (DATE);
passwordText.setText ( "");
verificationText.setText ( "");
}
} // this code is not correct the else
{
PasswordText .setText ( "");
verificationText.setText ( "");
JOptionPane.showMessageDialog (null, "code is incorrect, please re-enter");
// Create a random string
string Result = "";
for (int I = 0; i <6; i ++ ) {
int intVal = (int) (Math.random() * 26 + 97);
result = result + (char) intVal;
}
record = result;
verificationShowLabel.setText(result);
}
}
}
};
loginButton.addActionListener(ourListener1);
}
}
};
loginButton.addActionListener(ourListener1);
// Create the register button
JButton registerButton = new JButton ( "Register");
registerButton.setBounds (100, 120, 80, 25);
panel.add (registerButton);
JButton registerButton = new JButton ( "Register");
registerButton.setBounds (100, 120, 80, 25);
panel.add (registerButton);
// 对按钮进行监视
ActionListener ourListener2 = new ActionListener() {
public void actionPerformed(ActionEvent e) {
if (e.getSource() == registerButton) {
userText.setText("");
passwordText.setText("");
verificationText.setText("");
new RegisterInterface();
}
}
};
registerButton.addActionListener(ourListener2);
ActionListener ourListener2 = new ActionListener() {
public void actionPerformed(ActionEvent e) {
if (e.getSource() == registerButton) {
userText.setText("");
passwordText.setText("");
verificationText.setText("");
new RegisterInterface();
}
}
};
registerButton.addActionListener(ourListener2);
// Create the refresh button
JButton refreshButton = new JButton ( "refresh");
refreshButton.setBounds (250, 80, 80, 25);
panel.add (refreshButton);
JButton refreshButton = new JButton ( "refresh");
refreshButton.setBounds (250, 80, 80, 25);
panel.add (refreshButton);
// button to monitor
the ActionListener = new new ourListener3 the ActionListener () {
public void the actionPerformed (the ActionEvent E) {
IF (e.getSource () == to refreshButton) {
passwordText.setText ( "");
verificationText.setText ( "");
// create a random string
string Result = "";
for (int I = 0; I <. 6; I ++) {
int intVal = (int) (Math.random () * 26 is + 97);
Result = Result + ( char) intVal;
}
Record = Result;
verificationShowLabel.setText (Result);
the ActionListener = new new ourListener3 the ActionListener () {
public void the actionPerformed (the ActionEvent E) {
IF (e.getSource () == to refreshButton) {
passwordText.setText ( "");
verificationText.setText ( "");
// create a random string
string Result = "";
for (int I = 0; I <. 6; I ++) {
int intVal = (int) (Math.random () * 26 is + 97);
Result = Result + ( char) intVal;
}
Record = Result;
verificationShowLabel.setText (Result);
}
}
};
refreshButton.addActionListener(ourListener3);
}
}
}
};
refreshButton.addActionListener(ourListener3);
}
}
// This file is equivalent to a database for storing data
package yanzhengma;
import java.util.ArrayList;
import java.util.List;
import java.util.List;
import javax.swing.JOptionPane;
class Storeroom {
private String User;
private String password;
private String User;
private String password;
void setUser(String str) {
User = str;
}
User = str;
}
String getUser() {
return User;
return User;
}
void setPassword(String str) {
password = str;
}
password = str;
}
String getpassword() {
return password;
return password;
}
}
class StoreroomManager // operate on the data classes
{
static List <Storeroom> = new new datelist the ArrayList <Storeroom> (0);
{
static List <Storeroom> = new new datelist the ArrayList <Storeroom> (0);
static void confirmDate (Storeroom date) // log through the data
{
int In Flag = 0;
{
int In Flag = 0;
for (int i = 0; i < datelist.size(); i++) {
if (date.getUser().equalsIgnoreCase(datelist.get(i).getUser())) {
if (date.getpassword().equalsIgnoreCase(datelist.get(i).getpassword())) {
JOptionPane.showMessageDialog(null, "登录成功");
flag++;
}
if (date.getUser().equalsIgnoreCase(datelist.get(i).getUser())) {
if (date.getpassword().equalsIgnoreCase(datelist.get(i).getpassword())) {
JOptionPane.showMessageDialog(null, "登录成功");
flag++;
}
}
}
( "Data entry:") System.out.println;
System.out.println ( "User Name:" + date.getUser ());
System.out.println ( "Password:" + date.getpassword ( ));
for (int I = 0; I <datelist.size (); I ++) {
System.out.println ( "table" + (i + 1) + " data:");
the System.out. println ( "user name:" + datelist.get (I) .getUser ());
System.out.println ( "password:" + datelist.get (I) .getpassword ());
}
IF (In Flag == 0 ) {
JOptionPane.showMessageDialog (null, "user name or password is wrong!");
}
}
( "Data entry:") System.out.println;
System.out.println ( "User Name:" + date.getUser ());
System.out.println ( "Password:" + date.getpassword ( ));
for (int I = 0; I <datelist.size (); I ++) {
System.out.println ( "table" + (i + 1) + " data:");
the System.out. println ( "user name:" + datelist.get (I) .getUser ());
System.out.println ( "password:" + datelist.get (I) .getpassword ());
}
IF (In Flag == 0 ) {
JOptionPane.showMessageDialog (null, "user name or password is wrong!");
}
}
static boolean searchDate(Storeroom date)// 登录,遍历数据
{
int flag = 0;
for (int i = 0; i < datelist.size(); i++) {
if (date.getUser().equals(datelist.get(i).getUser()))
if (date.getpassword().equals(datelist.get(i).getpassword()))
flag++;
}
if (flag == 0)
return false;
else
return true;
{
int flag = 0;
for (int i = 0; i < datelist.size(); i++) {
if (date.getUser().equals(datelist.get(i).getUser()))
if (date.getpassword().equals(datelist.get(i).getpassword()))
flag++;
}
if (flag == 0)
return false;
else
return true;
}
}
// This document is about the same as the class Interface
package yanzhengma;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JPasswordField;
import javax.swing.JTextField;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JPasswordField;
import javax.swing.JTextField;
public class RegisterInterface {
private static String record;
registerInterface public () {
// create instance of JFrame
JFrame frame = new JFrame ( "Welcome to the registration page:");
// create instance of JFrame
JFrame frame = new JFrame ( "Welcome to the registration page:");
// 设置frame大小
frame.setLocation(500, 300);
frame.setSize(400, 200);
frame.setResizable(false);
frame.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
frame.setLocation(500, 300);
frame.setSize(400, 200);
frame.setResizable(false);
frame.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
// Create a panel
JPanel panel = new JPanel ();
JPanel panel = new JPanel ();
// add panel
frame.add (panel);
frame.add (panel);
// Set the panel particular forms
placeComponents (panel, frame);
placeComponents (panel, frame);
// Set interface is visible
frame.setVisible (true);
frame.setVisible (true);
}
void placeComponents public (the JPanel Panel, the JFrame Frame) {
// Clear panel
panel.setLayout (null);
// Clear panel
panel.setLayout (null);
// Create user component
JLabel userLabel = new JLabel ( "User Name:");
userLabel.setBounds (10, 20 is, 80, 25);
panel.add (userLabel);
JLabel userLabel = new JLabel ( "User Name:");
userLabel.setBounds (10, 20 is, 80, 25);
panel.add (userLabel);
// Create a text field for the user to input
the JTextField = new new userText the JTextField (20 is);
userText.setBounds (100, 20 is, 165, 25);
panel.add (userText);
the JTextField = new new userText the JTextField (20 is);
userText.setBounds (100, 20 is, 165, 25);
panel.add (userText);
// create a password component
JLabel passwordLabel = new JLabel ( "Password:");
passwordLabel.setBounds (10, 50, 80, 25);
panel.add (passwordLabel);
JLabel passwordLabel = new JLabel ( "Password:");
passwordLabel.setBounds (10, 50, 80, 25);
panel.add (passwordLabel);
// Create a password text field
JPasswordField PasswordText = new new JPasswordField (20);
passwordText.setBounds (100, 50, 165, 25);
panel.add (PasswordText);
JPasswordField PasswordText = new new JPasswordField (20);
passwordText.setBounds (100, 50, 165, 25);
panel.add (PasswordText);
// Create the component verification code used
JLabel verificationLabel = new JLabel ( "verification code:");
verificationLabel.setBounds (10, 80, 80, 25);
panel.add (verificationLabel);
JLabel verificationLabel = new JLabel ( "verification code:");
verificationLabel.setBounds (10, 80, 80, 25);
panel.add (verificationLabel);
// Create a text field codes
the JTextField = new new verificationText the JTextField (20 is);
verificationText.setBounds (100, 80, 80, 25);
panel.add (verificationText);
the JTextField = new new verificationText the JTextField (20 is);
verificationText.setBounds (100, 80, 80, 25);
panel.add (verificationText);
// create a random string
String Result = "";
for (int I = 0; I <. 6; I ++) {
int intVal = (int) (Math.random () * 26 is + 97);
Result = Result + ( char) intVal;
}
Record = Result;
String Result = "";
for (int I = 0; I <. 6; I ++) {
int intVal = (int) (Math.random () * 26 is + 97);
Result = Result + ( char) intVal;
}
Record = Result;
// Create verification code display components used
the JLabel verificationShowLabel the JLabel new new = (Result);
verificationShowLabel.setBounds (200 is, 80, 80, 25);
panel.add (verificationShowLabel);
the JLabel verificationShowLabel the JLabel new new = (Result);
verificationShowLabel.setBounds (200 is, 80, 80, 25);
panel.add (verificationShowLabel);
// Create the confirmation button
JButton confirmButton = new JButton ( "OK");
confirmButton.setBounds (10, 120, 80, 25);
panel.add (ConfirmButton);
JButton confirmButton = new JButton ( "OK");
confirmButton.setBounds (10, 120, 80, 25);
panel.add (ConfirmButton);
// button on the monitor (confirmed, i.e., the registration button)
the ActionListener ourListener1 the ActionListener new new = () {
public void the actionPerformed (the ActionEvent E) {
the ActionListener ourListener1 the ActionListener new new = () {
public void the actionPerformed (the ActionEvent E) {
if (e.getSource() == confirmButton) {
if (record.equalsIgnoreCase(verificationText.getText())) {
Storeroom date = new Storeroom();
date.setUser(userText.getText());
date.setPassword(passwordText.getText());
if (StoreroomManager.searchDate(date))
frame.dispose();
else {
StoreroomManager.datelist.add(date);
frame.dispose();
}
} else {
JOptionPane.showMessageDialog(null, "验证码错误,请重新输入");
verificationText.setText("");
passwordText.setText("");
if (record.equalsIgnoreCase(verificationText.getText())) {
Storeroom date = new Storeroom();
date.setUser(userText.getText());
date.setPassword(passwordText.getText());
if (StoreroomManager.searchDate(date))
frame.dispose();
else {
StoreroomManager.datelist.add(date);
frame.dispose();
}
} else {
JOptionPane.showMessageDialog(null, "验证码错误,请重新输入");
verificationText.setText("");
passwordText.setText("");
// create a random string
String Result = "";
for (int I = 0; I <. 6; I ++) {
int intVal = (int) (Math.random () * 26 is + 97);
Result = Result + ( char) intVal;
}
Record = Result;
verificationShowLabel.setText (Result);
}
String Result = "";
for (int I = 0; I <. 6; I ++) {
int intVal = (int) (Math.random () * 26 is + 97);
Result = Result + ( char) intVal;
}
Record = Result;
verificationShowLabel.setText (Result);
}
}
}
}
};
confirmButton.addActionListener(ourListener1);
confirmButton.addActionListener(ourListener1);
// Create a cancel button
JButton cancelButton = new JButton ( "Cancel");
cancelButton.setBounds (100, 120, 80, 25);
panel.add (By cancelButton);
JButton cancelButton = new JButton ( "Cancel");
cancelButton.setBounds (100, 120, 80, 25);
panel.add (By cancelButton);
// 对按钮进行监视
ActionListener ourListener2 = new ActionListener() {
public void actionPerformed(ActionEvent e) {
if (e.getSource() == cancelButton) {
JOptionPane.showMessageDialog(null, "are you kidding me?!");
frame.dispose();
}
}
};
cancelButton.addActionListener(ourListener2);
ActionListener ourListener2 = new ActionListener() {
public void actionPerformed(ActionEvent e) {
if (e.getSource() == cancelButton) {
JOptionPane.showMessageDialog(null, "are you kidding me?!");
frame.dispose();
}
}
};
cancelButton.addActionListener(ourListener2);
// Create the refresh button
JButton refreshButton = new JButton ( "refresh");
refreshButton.setBounds (250, 80, 80, 25);
panel.add (refreshButton);
JButton refreshButton = new JButton ( "refresh");
refreshButton.setBounds (250, 80, 80, 25);
panel.add (refreshButton);
// button to monitor
the ActionListener = new new ourListener3 the ActionListener () {
public void the actionPerformed (the ActionEvent E) {
IF (e.getSource () == to refreshButton) {
passwordText.setText ( "");
verificationText.setText ( "");
// create a random string
string Result = "";
for (int I = 0; I <. 6; I ++) {
int intVal = (int) (Math.random () * 26 is + 97);
Result = Result + ( char) intVal;
}
Record = Result;
verificationShowLabel.setText (Result);
the ActionListener = new new ourListener3 the ActionListener () {
public void the actionPerformed (the ActionEvent E) {
IF (e.getSource () == to refreshButton) {
passwordText.setText ( "");
verificationText.setText ( "");
// create a random string
string Result = "";
for (int I = 0; I <. 6; I ++) {
int intVal = (int) (Math.random () * 26 is + 97);
Result = Result + ( char) intVal;
}
Record = Result;
verificationShowLabel.setText (Result);
}
}
};
refreshButton.addActionListener(ourListener3);
}
};
refreshButton.addActionListener(ourListener3);
}
}
}
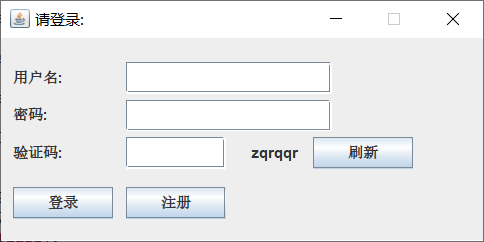