2.Spring case of IOC
Create a maven project
Import dependence
pom.xml
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
4.0.0
cn.cqu
xmlIOC
1.0-SNAPSHOT
jar
org.springframework
spring-context
5.0.2.RELEASE
commons-dbutils
commons-dbutils
1.4
mysql
mysql-connector-java
5.1.6
c3p0
c3p0
0.9.1.2
junit
junit
4.12
Create a database
CREATE TABLE account(
id INT PRIMARY KEY AUTO_INCREMENT,
NAME VARCHAR(40),
money FLOAT
)CHARACTER SET utf8 COLLATE utf8_general_ci;
INSERT INTO account(NAME,money)VALUES('aaa',1000);
INSERT INTO account(NAME,money)VALUES('bbb',1000);
INSERT INTO account(NAME,money)VALUES('ccc',1000);
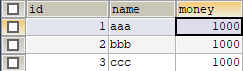
Account.java
package cn.cqu.domain;
public class Account {
private Integer id;
private String name;
private float money;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public float getMoney() {
return money;
}
public void setMoney(float money) {
this.money = money;
}
@Override
public String toString() {
return "Account{" +
"id=" + id +
", name='" + name + '\'' +
", money=" + money +
'}';
}
}
IAccountDao.java
package cn.cqu.dao;
import cn.cqu.domain.Account;
import java.util.List;
/**
* Account persistence layer interface
*/
public interface IAccountDao {
/**
* All inquiries
* @return
*/
List findAllAccount();
/**
* Query a
* @param accountId
* @return
*/
Account findById(Integer accountId);
/**
* Insert
* @param account
*/
void saveAccount(Account account);
/**
* Updates
* @param account
*/
void updateAccount(Account account);
/**
* Delete
* @param accountId
*/
void deleteAccount(Integer accountId);
}
AccountDaoImpl.java
package cn.cqu.dao.impl;
import cn.cqu.dao.IAccountDao;
import cn.cqu.domain.Account;
import org.apache.commons.dbutils.QueryRunner;
import org.apache.commons.dbutils.handlers.BeanHandler;
import org.apache.commons.dbutils.handlers.BeanListHandler;
import java.util.List;
public class AccountDaoImpl implements IAccountDao {
private QueryRunner runner;
public Query Runner getRunner () {
return runner;
}
public void setRunner(QueryRunner runner) {
this.runner = runner;
}
public List findAllAccount() {
try {
return runner.query("select * from account",new BeanListHandler(Account.class));
} catch (Exception e) {
throw new RuntimeException(e);
}
}
public Account findById(Integer accountId) {
try {
return runner.query("select * from account where id = ?",new BeanHandler(Account.class),accountId);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
public void saveAccount(Account account) {
try {
runner.update("insert into account(name,money)values(?,?)",account.getName(),account.getMoney());
} catch (Exception e) {
throw new RuntimeException(e);
}
}
public void updateAccount(Account account) {
try {
runner.update("update account set name=?,money=? where id=?",account.getName(),account.getMoney(),account.getId());
} catch (Exception e) {
throw new RuntimeException(e);
}
}
public void deleteAccount(Integer accountId) {
try {
runner.update("delete from account where id=?",accountId);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
IAccountService.java
package cn.cqu.service;
import cn.cqu.domain.Account;
import java.util.List;
public interface IAccountService {
/**
* All inquiries
* @return
*/
List findAllAccount();
/**
* Query a
* @param accountId
* @return
*/
Account findById(Integer accountId);
/**
* Insert
* @param account
*/
void saveAccount(Account account);
/**
* Updates
* @param account
*/
void updateAccount(Account account);
/**
* Delete
* @param accountId
*/
void deleteAccount(Integer accountId);
}
AccountServiceImpl.java
package cn.cqu.service.impl;
import cn.cqu.domain.Account;
import cn.cqu.service.IAccountService;
import cn.cqu.dao.IAccountDao;
import java.util.List;
public class AccountServiceImpl implements IAccountService {
private IAccountDao dao;
public IAccountDao getDao() {
return dao;
}
public void setDao(IAccountDao dao) {
this.dao = dao;
}
public List findAllAccount() {
return dao.findAllAccount();
}
public Account findById(Integer accountId) {
return dao.findById(accountId);
}
public void saveAccount(Account account) {
dao.saveAccount(account);
}
public void updateAccount(Account account) {
dao.updateAccount(account);
}
public void deleteAccount(Integer accountId) {
dao.deleteAccount(accountId);
}
}
bean.xml
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
AccountServiceTest.java
package cn.cqu.test;
import cn.cqu.domain.Account;
import cn.cqu.service.IAccountService;
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import java.util.List;
/**
* Use Junit unit testing: testing our configuration
*/
public class AccountServiceTest {
@Test
public void testFindAll()
{
1 // Get vessel
ApplicationContext ac = new ClassPathXmlApplicationContext("bean.xml");
// 2 to obtain business layer objects
IAccountService as = ac.getBean("accountService",IAccountService.class);
// 3. Execution method
List accounts = as.findAllAccount();
for (Account account:accounts)
{
System.out.println(account);
}
}
@Test
public void testFindById()
{
1 // Get vessel
ApplicationContext ac = new ClassPathXmlApplicationContext("bean.xml");
// 2 to obtain business layer objects
IAccountService as = ac.getBean("accountService",IAccountService.class);
// 3. Execution method
Account account = as.findById(1);
System.out.println(account);
}
@Test
public void Save test account ()
{
Account account =new Account();
account.setName("testFindById");
account.setMoney(1314);
1 // Get vessel
ApplicationContext ac = new ClassPathXmlApplicationContext("bean.xml");
// 2 to obtain business layer objects
IAccountService as = ac.getBean("accountService",IAccountService.class);
// 3. Execution method
as.saveAccount(account);
}
@Test
public void testUpdate()
{
1 // Get vessel
ApplicationContext ac = new ClassPathXmlApplicationContext("bean.xml");
// 2 to obtain business layer objects
IAccountService as = ac.getBean("accountService",IAccountService.class);
// 3. Execution method
Account account = as.findById(1);
account.setMoney(234);
as.updateAccount(account);
}
@Test
public void testDelete()
{
1 // Get vessel
ApplicationContext ac = new ClassPathXmlApplicationContext("bean.xml");
// 2 to obtain business layer objects
IAccountService as = ac.getBean("accountService",IAccountService.class);
// 3. Execution method
as.deleteAccount(3);
}
}
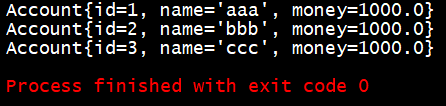

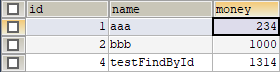
Use annotations to transform part of the code above
AccountDaoImpl.java
package cn.cqu.dao.impl;
import cn.cqu.dao.IAccountDao;
import cn.cqu.domain.Account;
import org.apache.commons.dbutils.QueryRunner;
import org.apache.commons.dbutils.handlers.BeanHandler;
import org.apache.commons.dbutils.handlers.BeanListHandler;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Repository;
import java.util.List;
@Repository("accountDao")
public class AccountDaoImpl implements IAccountDao {
@Autowired
private QueryRunner runner;
public List findAllAccount() {
try {
return runner.query("select * from account",new BeanListHandler(Account.class));
} catch (Exception e) {
throw new RuntimeException(e);
}
}
public Account findById(Integer accountId) {
try {
return runner.query("select * from account where id = ?",new BeanHandler(Account.class),accountId);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
public void saveAccount(Account account) {
try {
runner.update("insert into account(name,money)values(?,?)",account.getName(),account.getMoney());
} catch (Exception e) {
throw new RuntimeException(e);
}
}
public void updateAccount(Account account) {
try {
runner.update("update account set name=?,money=? where id=?",account.getName(),account.getMoney(),account.getId());
} catch (Exception e) {
throw new RuntimeException(e);
}
}
public void deleteAccount(Integer accountId) {
try {
runner.update("delete from account where id=?",accountId);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
AccountServiceImpl.java
package cn.cqu.service.impl;
import cn.cqu.domain.Account;
import cn.cqu.service.IAccountService;
import cn.cqu.dao.IAccountDao;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service("accountService")
public class AccountServiceImpl implements IAccountService {
@Autowired
private IAccountDao dao;
public List findAllAccount() {
return dao.findAllAccount();
}
public Account findById(Integer accountId) {
return dao.findById(accountId);
}
public void saveAccount(Account account) {
dao.saveAccount(account);
}
public void updateAccount(Account account) {
dao.updateAccount(account);
}
public void deleteAccount(Integer accountId) {
dao.deleteAccount(accountId);
}
}
bean.xml
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
https://www.springframework.org/schema/context/spring-context.xsd">
Operating results above
The new notes 3.Spring
We still can not do without spring xml configuration file, then can we not write this bean.xml, all configurations use annotations to achieve it?
We found that the reason why we can not do without xml configuration file, because:
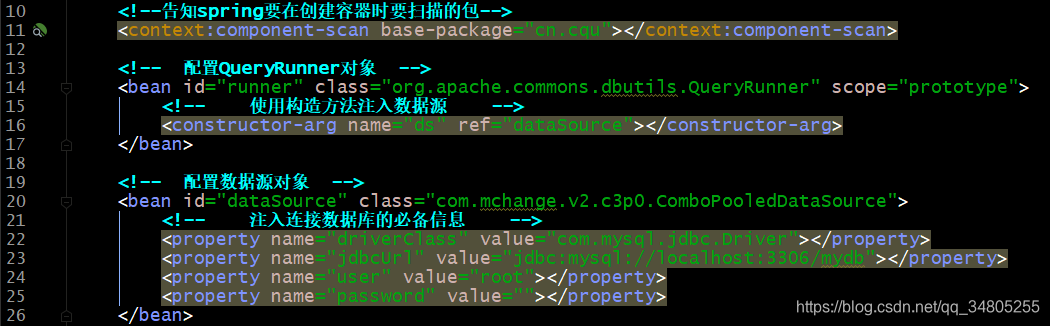
Reason 1:
If he wants to be able to configure annotation, then we are away from the xml file one step further.
Reason 2:
Data sources and QueryRunner configuration also need to rely on notes to achieve.
Because QueryRunner is a jar package under dbutils, we wanted to give it a plus that can not be annotated
The same is true dataSource
1. First, the transformation of the notes follows

Create a special category, as follows
package cn.cqu.config;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
/**
* Its class name and the name of the package in which it can be named by their own
*
* This class is a class configuration, and its role is the same bean.xml
*/
@Configuration
@ComponentScan(basePackages = "cn.cqu")
public class SpringConfiguration {
}
(1) @Configuration
Role: Wuxi abortion how much money http://www.chnk120.com/
The current class is used to specify a spring class configuration, will be loaded from the class notes when creating container. When the vessel is required to obtain
AnnotationApplicationContext (@Configuration have annotated classes .class)
Attributes:
value: for the configuration specified bytecode class
detail:
When configured as an argument AnnotationConfigApplicationContext class object created, you can not write this comment
(2)@ComponentScan或@ComponentScans
effect:
@ComponentScan used to specify a Spring specified when creating a container to be scanned through the notes package
@ComponentScans for designating a plurality of annotation specified by Spring container when creating a packet to be scanned
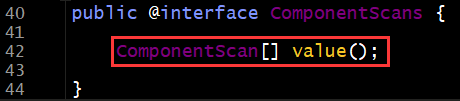
Attributes:
value or basePackages: specifies when creating the container package to be scanned
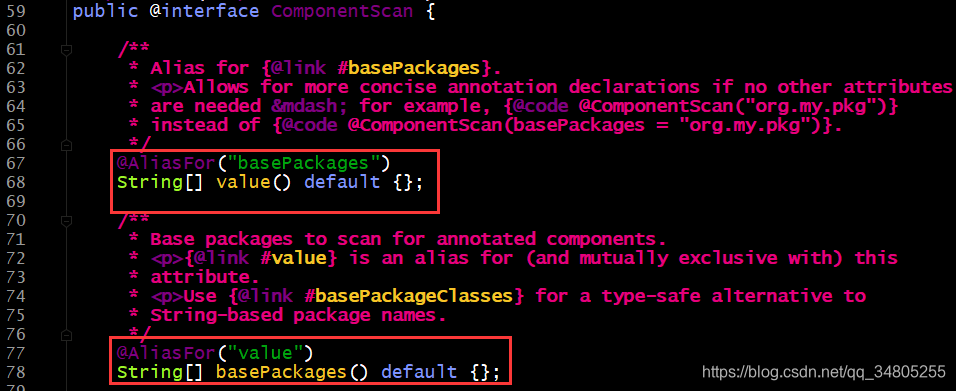
We use this annotation is equivalent to the configuration in bean.xml

2. Next follows the transformation of our

In fact, the above configuration has two steps:
1. Create an object QueryRunner
2. The IOC container into Sping
Add this class to create QueryRunner object methods, namely the completion of the first step in creating an object QueryRunner
Use the following to complete the second step Bean
(3)@Bean
effect:
Method for the return value of the current as the bean object into Spring IOC container
Attributes:
name: id is used to specify the bean, when not writing the name of the current method
detail:
When we use the annotation configuration method, if the method has parameters, spring framework will have to find the bean container object is not available, the mode of action to find and Autowired notes is the same
Similarly, we can also use to specify the scope @Scope
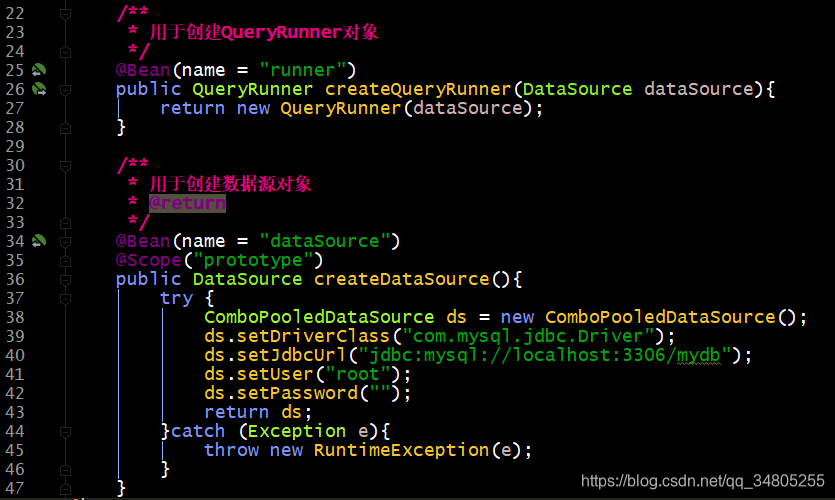
(4)@Import
effect:
Other configurations for introducing the class
By this method, may be disposed in a plurality of classes written among the class provided a master configuration, and other configurations will be introduced to the master configuration classes by the class corresponds to a coming together annotation @Import bean.xml
Attributes:
value: other configuration is used to specify the class bytecode
When we use Import, Import annotated classes there is the master configuration or parent class configuration, while the import of all sub-class configuration
同时它也支持并列的配置关系,我们只需要在使用AnnotationConfigApplicationContext创建容器时,将多个配置类的字节码都作为它的参数

4.SpringIOC总结
对Spring依赖总结:
变化集中转移到配置(配置文件或注解)中
Spring框架内部依赖于配置
自定义类依赖于不变(String)
从而编译时依赖转运行时依赖,降低耦合