Finishing array of methods currently used to learn a new es6 method.
Length 1 arr.push () additive element from the rear, the return value is added after the array
let arr = [1,2,3,4,5] console.log(arr.push(5)) // 6 console.log(arr) // [1,2,3,4,5,5]
2 arr.pop () remove elements from behind, is only one element of the return value is deleted
let arr = [1,2,3,4,5] console.log(arr.pop()) // 5 console.log(arr) //[1,2,3,4]
3 arr.shift () Returns the value of a front delete elements, it can only be deleted from the deleted elements
let arr = [1,2,3,4,5] console.log(arr.shift()) // 1 console.log(arr) // [2,3,4,5]
Length 4 arr.unshift () to add elements from the foregoing, the return value is added after the array
let arr = [1,2,3,4,5] console.log(arr.unshift(2)) // 6 console.log(arr) //[2,1,2,3,4,5]
5 arr.splice (i, n) after the start deleting that element from i (index value). The return value is removed elements
Argument: i index number n
let arr = [1,2,3,4,5] console.log(arr.splice(2,2)) //[3,4] console.log(arr) // [1,2,5]
6 arr.concat () returns an array of two new array is connected to the connection
let arr = [1,2,3,4,5] console.log(arr.concat([1,2])) // [1,2,3,4,5,1,2] console.log(arr) // [1,2,3,4,5]
7 str.split () will be converted to an array of strings
let str = '123456' console.log(str.split('')) // ["1", "2", "3", "4", "5", "6"]
8 arr.sort () to sort the array, good return value is an array of rows, the default is sorted by the leftmost digit is not, see examples in numerical sorting size.
let arr = [2,10,6,1,4,22,3] console.log(arr.sort()) // [1, 10, 2, 22, 3, 4, 6] let arr1 = arr.sort((a, b) =>a - b) console.log(arr1) // [1, 2, 3, 4, 6, 10, 22] let arr2 = arr.sort((a, b) =>b-a) console.log(arr2) // [22, 10, 6, 4, 3, 2, 1]
9 arr.reverse () array reversed, the return value is an array of the inverted
let arr = [1,2,3,4,5] console.log(arr.reverse()) // [5,4,3,2,1] console.log(arr) // [5,4,3,2,1]
10 arr.slice (start, end) to cut the index start end of the array index value, the index does not contain the end value, the return value is an array of cut out
let arr = [1,2,3,4,5] console.log(arr.slice(1,3)) // [2,3] console.log(arr) // [1,2,3,4,5]
11 arr.forEach (callback) through the array, no return
callback parameters: value - the current value of the index
index - the index
array - the original array
let arr = [1,2,3,4,5] arr.forEach( (value,index,array)=>{ console.log(`value:${value} index:${index} array:${array}`) }) // value:1 index:0 array:1,2,3,4,5 // value:2 index:1 array:1,2,3,4,5 // value:3 index:2 array:1,2,3,4,5 // value:4 index:3 array:1,2,3,4,5 // value:5 index:4 array:1,2,3,4,5 let arr = [1,2,3,4,5] arr.forEach( (value,index,array)=>{ value = value * 2 console.log(`value:${value} index:${index} array:${array}`) }) console.log(arr) // value:2 index:0 array:1,2,3,4,5 // value:4 index:1 array:1,2,3,4,5 // value:6 index:2 array:1,2,3,4,5 // value:8 index:3 array:1,2,3,4,5 // value:10 index:4 array:1,2,3,4,5 // [1, 2, 3, 4, 5]
12 arr.map (callback) mapping array (iterate), there is return to return a new array
callback parameters: value - the current value of the index
index - the index
array - the original array
Press Ctrl + C to copy the code
Press Ctrl + C to copy the code
ps: the difference arr.forEach () and arr.map () of
1. arr.forEach () is the for loop, is replaced for. arr.map () is an array where the modified data, and returns the new data.
2. arr.forEach () does not return arr.map () has return
13 arr.filter (callback) with arrays, returns an array to meet the requirements of
let arr = [1,2,3,4,5] let arr1 = arr.filter( (i, v) => i < 3) console.log(arr1) // [1, 2]
14 arr.every (callback) is determined according to the condition, whether the whole element of the array is satisfied, if yes return ture
let arr = [1,2,3,4,5] let arr1 = arr.every( (i, v) => i < 3) console.log(arr1) // false let arr2 = arr.every( (i, v) => i < 10) console.log(arr2) // true
15 arr.some () Analyzing element according to the condition, whether there is an array satisfied, if a return to meet ture
let arr = [1,2,3,4,5] let arr1 = arr.some( (i, v) => i < 3) console.log(arr1) // true let arr2 = arr.some( (i, v) => i > 10) console.log(arr2) // false
16 arr.reduce (callback, initialValue) an array of all iterations, the accumulator, each value (left to right) were combined in the array, a finally calculated value
Parameters: callback: previousValue Required - callback return value on a first call, or the initial value (the initialValue) provided
currentValue Required - array item currently being processed array
Optional index - the current array item index values in the array
Optional array - the original array
initialValue: Optional - Initial value
Carry out the method: when the first execution of the callback function, and curValue preValue may be a value to be provided if the reduce initialValue call (), then the first preValue initialValue equal, and equal to the first value curValue array; if initialValue not provided, then preValue equal to the first value in the array.
let arr = [0,1,2,3,4] let arr1 = arr.reduce((preValue, curValue) => preValue + curValue ) console.log(arr1) // 10
let arr2 = arr.reduce((preValue,curValue)=>preValue + curValue,5) console.log(arr2) // 15
17 arr.reduceRight Like (callback, initialValue) and arr.reduce () function, except that, reduceRight () from the forward end of the array of array of items in the array do accumulate.
Implementation methods: reduceRight () the first call to the callback function callbackfn, prevValue and curValue can be one of two values. Providing initialValue If the call parameters reduceRight (), is equal to the prevValue initialValue, curValue equal to the last value in the array. InitialValue If no parameter is equal to the array prevValue last value, equal to the array curValue penultimate value.
let arr = [0,1,2,3,4] let arr1 = arr.reduceRight((preValue, curValue) => preValue + curValue ) console.log(arr1) // 10
let arr2 = arr.reduceRight((preValue,curValue)=>preValue + curValue,5) console.log(arr2) // 15
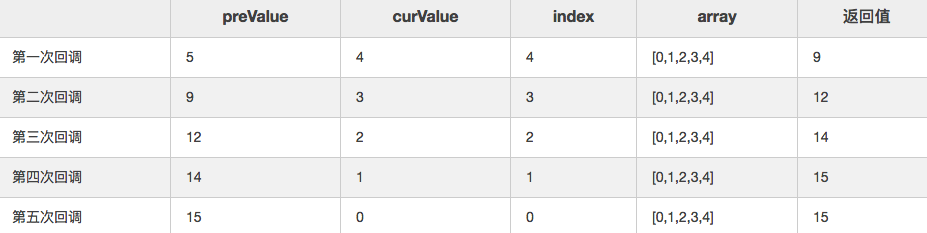
ps:
(If these two methods do not understand, you can view the desert teacher instance
http://www.w3cplus.com/javascript/array-part-8.html
)
18 arr.indexOf () to find an element index value, if repeated, is found in the first index value if there returns -1
let arr = [1,2,3,4,5,2] let arr1 = arr.indexOf(2) console.log(arr1) // 1 let arr2 = arr.indexOf(9) console.log(arr2) // -1
19 arr.lastIndexOf() 和arr.indexOf()的功能一样,不同的是从后往前查找
let arr = [1,2,3,4,5,2] let arr1 = arr.lastIndexOf(2) console.log(arr1) // 5 let arr2 = arr.lastIndexOf(9) console.log(arr2) // -1
20 Array.from() 将伪数组变成数组,就是只要有length的就可以转成数组。 ---es6
let str = '12345' console.log(Array.from(str)) // ["1", "2", "3", "4", "5"] let obj = {0:'a',1:'b',length:2} console.log(Array.from(obj)) // ["a", "b"]
21 Array.of() 将一组值转换成数组,类似于声明数组 ---es6
let str = '11' console.log(Array.of(str)) // ['11']
等价于
console.log(new Array('11')) // ['11]
ps:
但是new Array()有缺点,就是参数问题引起的重载
console.log(new Array(2)) //[empty × 2] 是个空数组 console.log(Array.of(2)) // [2]
22 arr.copyWithin() 在当前数组内部,将制定位置的数组复制到其他位置,会覆盖原数组项,返回当前数组
参数: target --必选 索引从该位置开始替换数组项
start --可选 索引从该位置开始读取数组项,默认为0.如果为负值,则从右往左读。
end --可选 索引到该位置停止读取的数组项,默认是Array.length,如果是负值,表示倒数
let arr = [1,2,3,4,5,6,7] let arr1 = arr.copyWithin(1) console.log(arr1) // [1, 1, 2, 3, 4, 5, 6] let arr2 = arr.copyWithin(1,2) console.log(arr2) // [1, 3, 4, 5, 6, 7, 7] let arr3 = arr.copyWithin(1,2,4) console.log(arr3) // [1, 3, 4, 4, 5, 6, 7]
23 arr.find(callback) 找到第一个符合条件的数组成员
let arr = [1,2,3,4,5,2,4] let arr1 = arr.find((value, index, array) =>value > 2) console.log(arr1) // 3
24 arr.findIndex(callback) 找到第一个符合条件的数组成员的索引值
let arr = [1,2,3,4,5] let arr1 = arr.findIndex((value, index, array) => value > 3) console.log(arr1) // 3
25 arr.fill(target, start, end) 使用给定的值,填充一个数组,ps:填充完后会改变原数组
参数: target -- 待填充的元素
start -- 开始填充的位置-索引
end -- 终止填充的位置-索引(不包括该位置)
let arr = [1,2,3,4,5] let arr1 = arr.fill(5) console.log(arr1) // [5, 5, 5, 5, 5] console.log(arr) // [5, 5, 5, 5, 5] let arr2 = arr.fill(5,2) console.log(arr2) let arr3 = arr.fill(5,1,3) console.log(arr3)
26 arr.includes() 判断数中是否包含给定的值
let arr = [1,2,3,4,5] let arr1 = arr.includes(2) console.log(arr1) // ture let arr2 = arr.includes(9) console.log(arr2) // false let arr3 = [1,2,3,NaN].includes(NaN) console.log(arr3) // true
ps:与indexOf()的区别:
1 indexOf()返回的是数值,而includes()返回的是布尔值
2 indexOf() 不能判断NaN,返回为-1 ,includes()则可以判断
27 arr.keys() 遍历数组的键名
let arr = [1,2,3,4] let arr2 = arr.keys() for (let key of arr2) { console.log(key); // 0,1,2,3 }
28 arr.values() 遍历数组键值
let arr = [1,2,3,4] let arr1 = arr.values() for (let val of arr1) { console.log(val); // 1,2,3,4 }
29 arr.entries () key names and values through the array
let arr = [1,2,3,4] let arr1 = arr.entries() for (let e of arr1) { console.log(e); // [0,1] [1,2] [2,3] [3,4] }
entries () method returns an array of iterations.
Before each iteration array index value is a value as a key, a value as the array value.