A data type
Second, input and output statements
Example:
scanf("%d",&a); printf("%d",a);
Different types of data have their corresponding format type:
. 1, int % D takes an integer value and it is represented as a signed decimal integer
long% ld is a long integer
2 , % O unsigned octal integer (no output prefix 0)
3,% u unsigned decimal integer
4,% x / X unsigned hexadecimal integer Example:
printf("%x\n", i); printf("%X\n", i); printf("%#x\n", i); printf("%#X\n", i);
Respectively output 2f 2F 0x2f 0X2F
. 5, a float , and double single-precision floating-point ( % F ) and double precision floating point ( % LF)
. 6 , % .mf output real decimal places of m bits, m has a front attention points.
. 7 , % E / E number, represented here scientific notation "e" is represented by a case when the output "e" capitalization
8,% c character. Can according to the digital input ASCII code into corresponding respective character
Example:
#include <stdio.h> int main() { char i='A'; printf("%c",i); return 0; }
A character output
If the output is a control character '% d', the output value of the ASCII code corresponding to 'A'
9,% s string. Output string of characters until a null character string (string '\ 0' ends, this '\ 0' i.e. null character)
Example:
#include <stdio.h> int main() { char i[]="ABC"; printf("%s",i); return 0; }
Output string of ABC
10,% p to the output pointer, output variable address in hexadecimal notation
Example:
#include <stdio.h> int main () { int I = . 5 ; the printf ( " % D \ n- " , I); // output decimal integer the printf ( " % P " , I); // hexadecimal output pointer form the printf ( " % P " , & I); // output variable memory address return 0 ; }
11,% n number of characters before this character until the total output, no output text
12 %% without conversion, outputting the character '%' (percent) itself
13,% m print error errno value corresponding to the content, (Example: printf ( "% m \ n ");
Third, the escape character
Escape character
|
significance
|
ASCII value (decimal)
|
\a
|
Bell (BEL)
|
007
|
\b
|
Backspace (BS), the current position to the previous column
|
008
|
\f
|
Feed (FF), the current position to the beginning of the page
|
012
|
\n
|
Line feed (LF), the current position to the beginning of the next line
|
010
|
\r
|
A carriage return (CR), the current position to the beginning of the line
|
013
|
\t
|
Horizontal tab (the HT) (TAB jumps to the next position)
|
009
|
\ v
|
Vertical tabulation (VT)
|
011
|
\\
|
Represent a backslash character '\'
|
092
|
\'
|
On behalf of a single quote (apostrophe) character
|
039
|
\"
|
It represents a double quote character
|
034
|
\? | On behalf of a question mark | 063 |
\0
|
Null character (NUL)
|
000
|
\ooo
|
Any character 1-3 octal number represented
|
Three octal
|
\ socialization
|
Hexadecimal character represents any
|
Hex
|
String needs to be used to represent \\ \ first \ represents the escape, the second \ character represents the
Example:
#include <stdio.h> main() { printf("hello world"); printf("\n"); printf("hello world"); printf("\a"); //响铃 printf("\b"); //退格 printf("\n"); printf("\f"); //翻页 printf("\n"); printf("hello world"); printf("\r"); //回车 printf("\t"); //水平制表,跳到下一个TAB位置 printf("hello world"); system("\"C:\\Program Files\\Tencent\\QQ\\Bin\\QQScLauncher.exe\""); // ‘\\’和‘"""’表示不出来用\来转义 getchar(); //等待响应 return 0; }
四、常量与变量
1、变量的命名规则
• 标识符:
程序中用于标识常量、变量、函数的字符序列组成
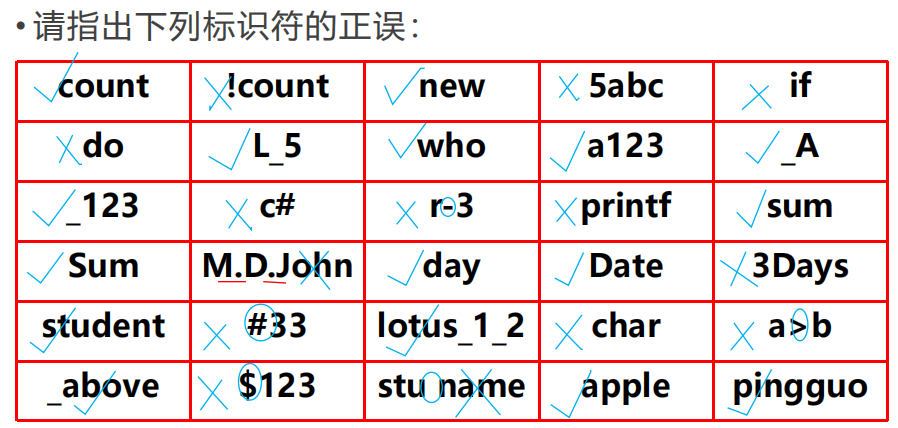
变量使用之前一定要初始化,如果变量不初始化,就会默认读取垃圾数据, 有些垃圾数据
会导致程序崩溃。所以,变量使用之前,必须初始化。
2、定义常量
• 定义常量PI的两种方式:
#define PI 3.14159; const float PI=3.14159; //const定义的常量不能修改,是只读数据
例:
#include <stdio.h> int main() { #define NAME "朱健康" const int AGE=22; printf("我的名字: %s",NAME); printf("\n"); printf("我的年龄:%d",AGE); return 0; }
#include <stdio.h> //自由落体运动 g=9.8 //输入时间,输出自由落体运动的距离 int main() { #define g 9.8 float t,s; printf("自由落体时间:\n"); scanf("%f",&t); s=1/2.0*g*t*t; printf("自由落体的距离: %f",s); return 0; }
五、进制的计算
1、二进制、八进制转换
• 例 (1101001)2=(001,101,001)2=(151)8
• 例 (246)8=(010,100,110)2=(10100110)2
2、十进制、二进制转换
- 0.625 * 2 = 1.25 取整1
- 0.25 * 2 = 0.5 取整0
- 0.5 * 2 = 1 取整1
3、位、字节、字
六、整型常量
• 十进制整数:

• 八进制整数:
• 十六进制整数:
• 长整型常量:
七、整型变量
八、浮点型常量
• 十进制小数形式:
• 指数形式:
• 实型常量的类型细分:
九、浮点型变量
#include <stdio.h> #include <math.h> //给出三角形的三边长,求三角形面积。 int main() { double a,b,c; double p,s; scanf("%lf %lf %lf",&a,&b,&c); //double的格式化类型为%lf if(a+b>c && a+c>b && b+c>a) { p=(a+b+c)/2.0; s=sqrt(p*(p-a)*(p-b)*(p-c)); printf("a=%lf\tb=%lf\tc=%lf\n",a,b,c); printf("三角形面积:%f",s); } else { printf("三边不能构成三角形"); } return 0; }