
Article Directory
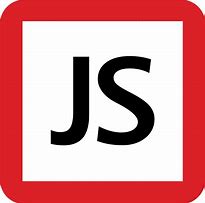
JS Object Model
JavaScript is a prototype-based (the Prototype) object-oriented language, rather than object-oriented language class
C ++, Java has class and instance Instance Class concept, abstract class is a class of things, but it is kind of entity instances
JS is a prototype-based language, it is only the concept prototype object. Prototype object is a template, new object from the template constructed in order to obtain the original property. Any object at runtime can dynamically add attributes. Moreover, any object can be used as a prototype of another object, so that the latter can share the properties of the former
The definition of class
Declare literal way
var obj = {
property_1: value_1, // property_# may be an identifier...
property_2: value_2, // or a number...
...,
"property n": value_n // or a string
};
var obj = {
x : 1,
1 : 'abc',
'y':'123'
}
for (let s in obj)
console.log(s, typeof(s));
This method is also known as literals create objects
Js 1.2 started to support
Before ES6 - constructor
- The definition of a function (constructor) objects, function names capitalized
- Use this attribute definitions
- Create a new object using the new and constructors
// 定义类,构造器 function Point(x, y) { this.x = x; this.y = y; this.show = () => {console.log(this,this.x,this.y)}; console.log('Point~~~~~~~~'); } console.log(Point); p1 = new Point(4,5); console.log(p1); console.log('----------------'); // 继承 function Point3D(x,y,z) { Point.call(this,x,y); // "继承" this.z = z; console.log('Point3D~~~~~~~~'); } console.log(Point3D); p2 = new Point3D(14,15,16); console.log(p2); p2.show(); 运行结果: Info: Start process (下午8:31:59) [Function: Point] Point~~~~~~~~ Point { x: 4, y: 5, show: [Function] } ---------------- [Function: Point3D] Point~~~~~~~~ Point3D~~~~~~~~ Point3D { x: 14, y: 15, show: [Function], z: 16 } Point3D { x: 14, y: 15, show: [Function], z: 16 } 14 15 Info: End process (下午8:31:59)
Construction of a new new generic object, the new operator will pass this value to the new object Point3D constructor function, function to create the object properties for z
From the words know, after getting a new object, use this object to call the constructor, then how to perform a "base class' constructor methods? Point3D object is used to perform this constructor Point, the use of the method call, passing this subclass. Eventually, after construction is completed, the object is assigned to p2
Note: If you do not use the new keyword, is a normal function call, this does not mean instances
ES6 in class
ES6 from the beginning, providing a new class keyword, makes creating objects more simple, clear
- Class is defined using the class keyword. In essence create or function is a special function
- A class can have a constructor method named constructor. If no explicit definition of a constructor, is added a default method constuctor
- Use extends keyword to inherit
- A constructor can use the super keyword to call a parent class constructor
- Class is not a private property
// 基类定义 class Point { constructor(x,y) /*构造器*/ { this.x = x; this.y = y; } show() /*方法*/ { console.log(this,this.x,this.y); } } let p1 = new Point(10,11) p1.show() // 继承 class Point3D extends Point { constructor (x,y,z) { super(x,y); this.z = z; } } let p2 = new Point3D(20,21,22); p2.show() 运行结果: Info: Start process (下午8:38:01) Point { x: 10, y: 11 } 10 11 Point3D { x: 20, y: 21, z: 22 } 20 21 Info: End process (下午8:38:01)
Overriding methods
Subclass method Point3D the show, you need to be rewritten
// 基类定义
class Point {
constructor(x,y) /*构造器*/ {
this.x = x;
this.y = y;
//this.show = function() {console.log(this,this.x)};
}
show() /*方法*/ {
console.log(this,this.x,this.y);
}
}
let p1 = new Point(10,11)
p1.show()
// 继承
class Point3D extends Point {
constructor (x,y,z) {
super(x,y);
this.z = z;
}
show(){ // 重写
console.log(this,this.x,this.y, this.z);
}
}
let p2 = new Point3D(20,21,22);
p2.show();
运行结果:
Info: Start process (下午8:42:59)
Point { x: 10, y: 11 } 10 11
Point3D { x: 20, y: 21, z: 22 } 20 21 22
Info: End process (下午8:42:59)
Direct overwrite method in the subclass to the parent
, if the parent class is required, the use of super.method () call way
const person = {
age:'20多了',
name(){
return this.age;
}
}
const man = {
age:'18岁了',
sayName(){
return super.name();
}
}
Object.setPrototypeOf( man, person );
let n = man.sayName();
console.log( n ) //18岁了
运行结果:
Info: Start process (上午10:06:35)
18岁了
Info: End process (上午10:06:35)
Arrow functions also support covering subclass
Summary: parent class, subclass, class definition used in the same way property or method, the child class overrides parent. When accessing the same name property or method, preferably used property (with different sub-genus)
Static properties
Static properties not yet well supported
Static method
Although Js and C ++, Java, like there is this, but the performance is different Js
The reason is that, C ++, Java is statically compiled language, this is a compile time binding, and Js is a dynamic language runtime bindings
class Add {
constructor(x, y) {
this.x = x;
this.y = y;
}
static print(){
console.log(this.x); // ? this是什么
}
}
add = new Add(40, 50);
console.log(Add);
Add.print();
//add.print(); // 实例不能访问直接访问静态方法,和C++、Java一致
add.constructor.print(); // 实例可以通过constructor访问静态方法
运行结果:
Info: Start process (下午9:03:09)
[Function: Add]
undefined //console.log()没有返回结果
undefined
Info: End process (下午9:03:09)
This is a static method Add class, rather than the examples Add
Note:
static concept and Python static different, the equivalent of class variables in Python
Points to note this
Although Js and C ++, Java, like there is this, but the performance is different Js
The reason is that, C ++, Java is statically compiled language, this is a compile time binding, and Js is a dynamic language runtime bindings
var school = {
name : 'magedu',
getNameFunc : function () {
console.log(this.name);
console.log(this);
return function () {
console.log(this === global); // this是否是global对象
return this.name;
};
}
};
console.log(school.getNameFunc()());
/* 运行结果
magedu{ name: 'magedu', getNameFunc: [Function: getNameFunc] }
true
undefined*/
In order to analyze the above procedures, first learn some knowledge:
when the function is executed, it will open a new execution context ExecutionContext
Creating this property, but this is what we should look at how the function is called up
- myFunction (1,2,3), an ordinary function call, this points to the global object. Nodejs global object is the global or browser
window - myObject.myFunction (1,2,3), is called object method, this method comprises the pointing object
- call and apply the method call, who look at the first parameter is
Analysis of the Examples
magedu and {name: 'magedu', getNameFunc : [Function: getNameFunc]} is well understood
True third-line printing is the result of the implementation of the console.log (this == global), it shows that the current is global, because the call returned by this function
is called directly, this is a normal function call, so this is a global object
The fourth line undefined, because this is global, no name attribute
This is the time function call, call different ways, this corresponds to a different object, it is not C ++, Java point to an instance of itself, the problem is this, and this is a problem left over from history, and is compatible with the new version retains only
And we use, sometimes need to be clear so that this must be our desired objects, how to solve this problem?
Explicit Incoming
var school = {
name : 'magedu',
getNameFunc : function () {
console.log(this.name);
console.log(this);
return function (that) {
console.log(this == global); // this是否是global对象
return that.name;
};
}
}
console.log(school.getNameFunc()(school));
/* 运行结果
magedu
{ name: 'magedu', getNameFunc: [Function: getNameFunc] }
false
magedu
*/
By actively incoming object, thus avoiding the problem of this
ES3 (ES-262 third edition) introduced apply, call method
var school = {
name : 'magedu',
getNameFunc : function () {
console.log(this.name);
console.log(this);
return function () {
console.log(this == global); // this是否是global对象
return this.name;
};
}
}
console.log(school.getNameFunc().call(school)); // call方法显式传入this对应的对象
/* 运行结果
magedu
{ name: 'magedu', getNameFunc: [Function: getNameFunc] }
false
magedu
*/
apply, call the object's methods are a function of the method, the first parameter is passed in the object introduced
apply pass other parameters need to use an array
call transfer other parameters need to use variable parameters collection
function Print() {
this.print = function(x,y) {console.log(x + y)};
}
p = new Print(1,2)
p.print(10, 20)
p.print.call(p, 10, 20);
p.print.apply(p, [10, 20]);
运行结果:
Info: Start process (上午9:42:22)
30
30
30
Info: End process (上午9:42:22)
ES5 introduces a bind method
bind this method to set the value of the function
var school = {
name : 'magedu',
getNameFunc : function () {
console.log(this.name);
console.log(this);
return function () {
console.log(this == global); // this是否是global对象
return this.name;
};
}
}
console.log(school.getNameFunc().bind(school)); // bind方法绑定
/* 运行结果
magedu
{ name: 'magedu', getNameFunc: [Function: getNameFunc] }
[Function: bound ]
*/
Only print three lines, indicating that where there are problems, the problem lies in the wrong bind method
var school = {
name : 'magedu',
getNameFunc : function () {
console.log(this.name);
console.log(this);
return function () {
console.log(this == global); // this是否是global对象
return this.name;
};
}
}
var func = school.getNameFunc();
console.log(func);
var boundfunc = func.bind(school); // bind绑定后返回新的函数
console.log(boundfunc);
console.log(boundfunc());
/* 运行结果
magedu
{ name: 'magedu', getNameFunc: [Function: getNameFunc] }
[Function]
[Function: bound ]
false
magedu
*/
apply, call methods, different parameters, passed this time call
bind method is a function to bind this, the direct use when calling
ES6 introduced support this function arrow
ES6 new technology, they do not compatible with this problem
var school = {
name : 'magedu',
getNameFunc : function () {
console.log(this.name);
console.log(this);
return () => {
console.log(this == global); // this是否是global对象
return this.name;
};
}
}
console.log(school.getNameFunc()());
/* 运行结果
magedu
{ name: 'magedu', getNameFunc: [Function: getNameFunc] }
false
magedu
*/
ES6 new definition as follows
class school{
constructor(){
this.name = 'magedu';
}
getNameFunc() {
console.log(this.name);
console.log(this, typeof(this));
return () => {
console.log(this == global); // this是否是global对象
return this.name;
};
}
}
console.log(new school().getNameFunc()());
/* 运行结果
magedu
school { name: 'magedu' } 'object'
false
magedu
*/
The method above to solve this problem, bind the most common method