1.Map Profile
1.java.util package the Map <K, V>
K - type this map maintained bond, V - type of mapped values (can be understood as one relationship)
individuals dry point (FIG):
1.2 personal focus on explaining several methods (PUT, entrySet, keySet)
1.2.1 PUT method
to run the following code:
package com.wangcong.map;
import java.util.HashMap;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
public class MapTest {
public static void main(String[] args) {
Map<Object, Object> map=new HashMap();
map.put("zs", 12);
map.put("ww", 23);
map.put("ls", 33);
map.put("zl", 22);
map.put("zs", 42);
Object old=map.put("ls", 37);
System.out.println("原来的value值"+old);
System.out.println(map);
}
}
Run results:
it can be concluded:
1.put method has additional features.
2.put way to increase the value of an existing key names when it will replace the original key
3.put way to increase an existing bond will be returned when the name of its original value
1.2.2 entrySet, keySet
Origin: Map interface does not inherit because Collection interface, meaning that it does not have the collection iterator approach
the entrySet (entire Map data is acquired)
operation code is as follows:
package com.wangcong.map;
import java.util.HashMap;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
public class MapTest {
public static void main(String[] args) {
Map<Object, Object> map=new HashMap();
map.put("zs", 12);
map.put("ww", 23);
map.put("ls", 33);
map.put("zl", 22);
map.put("zs", 42);
//entrySet得到的是一整个数据
Set<Entry<Object, Object>> entrySet = map.entrySet();
for (Entry<Object, Object> entry : entrySet) {
System.out.println("key:"+entry.getKey()+",value:"+entry.getValue());
}
}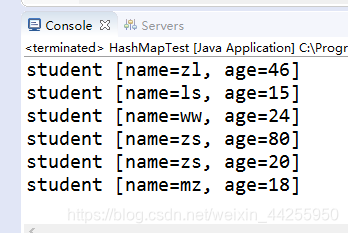
}
FIG output:
keySet (all acquired key)
running the following code:
package com.wangcong.map;
import java.util.HashMap;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
public class MapTest {
public static void main(String[] args) {
Map<Object, Object> map=new HashMap();
map.put("zs", 12);
map.put("ww", 23);
map.put("ls", 33);
map.put("zl", 22);
map.put("zs", 42);
//keySet
Set<Object> keySet = map.keySet();
for (Object object : keySet) {
System.out.println("key:"+object+",value"+map.get(object));
}
}
}
FIG results are as follows:
hha
2.Map applications
2.1 Before introducing me to explain about the following use to look at things (HaspMap, TreeMap)
HashMap: the underlying data structure is a hash table.
TreeMap: the underlying data structure is a binary tree.
2.2 Application of a
demand:
1, students as key addresses as values are stored, the name of the same age were identified as a man, and finally output
2, and finally sorted by age
3, requirements change, sorted by name
analysis: first point: the need to package a student class, sentenced to heavy print.
The second point: to enable students to have a class of its own comparative (implement Comparabel Interface)
The third point: changes in demand unrealistic to change the code again, so take additional comparator.
Run the following code:
package com.wangcong.map;
import java.util.Comparator;
import java.util.HashMap;
import java.util.Set;
import java.util.TreeMap;
/**
* 应用一:
* 1、将学生作为键,地址作为值进行存储,名字年龄相同则被认定为一个人,最后输出
* 思路:
* a、封装学生类
* b、判重(hashCode/equals)
* c、打印
* 2、最后按年龄进行排序
* a、需要让学生类自身具备比较性,实现Comparable接口
* 3、需求改变、按姓名进行排序
* 改变的代码是不可取的,我们需要新增个比较器来完成需求
* 实现java.util.comparator
*
*/
public class HashMapTest {
public static void main(String[] args) {
HashMap map=new HashMap<>();
map.put(new student("zs",20), "beijing");
map.put(new student("mz",18), "beijing");
map.put(new student("zl",46), "beijing");
map.put(new student("ww",24), "beijing");
map.put(new student("ls",15), "beijing");
map.put(new student("zs",20), "beijing");//和第一条数据一样用于验证判重是否成功
map.put(new student("zs",80), "beijing");
Set keySet = map.keySet();
for (Object object : keySet) {
System.out.println(object);
}
}
}
class student {//封装学生类
private String name;
private int age;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public student(String name, int age) {
super();
this.name = name;
this.age = age;
}
public student() {
super();
}
@Override
public String toString() {
return "student [name=" + name + ", age=" + age + "]";
}
@Override
public int hashCode() {
return this.name.hashCode()+this.age;
}
@Override
public boolean equals(Object obj) {
student stu=(student) obj;
return this.name.equals(stu.name) && this.age ==stu.age;
}
}
Output:
It can be seen: to repeat the successful completion of the first point
to continue editing :( because of the need to sort by age, all using TreeMap)
package com.wangcong.map;
import java.util.Comparator;
import java.util.HashMap;
import java.util.Set;
import java.util.TreeMap;
/**
* 应用一:
* 1、将学生作为键,地址作为值进行存储,名字年龄相同则被认定为一个人,最后输出
* 思路:
* a、封装学生类
* b、判重(hashCode/equals)
* c、打印
* 2、最后按年龄进行排序
* a、需要让学生类自身具备比较性,实现Comparable接口
* 3、需求改变、按姓名进行排序
* 改变的代码是不可取的,我们需要新增个比较器来完成需求
* 实现java.util.comparator
*
*/
public class HashMapTest {
public static void main(String[] args) {
TreeMap map=new TreeMap<>();
map.put(new student("zs",20), "beijing");
map.put(new student("mz",18), "beijing");
map.put(new student("zl",46), "beijing");
map.put(new student("ww",24), "beijing");
map.put(new student("ls",15), "beijing");
map.put(new student("zs",20), "beijing");//和第一条数据一样用于验证判重是否重复
map.put(new student("zs",80), "beijing");
Set keySet = map.keySet();
for (Object object : keySet) {
System.out.println(object);
}
}
}
class student implements Comparable<student>{
private String name;
private int age;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public student(String name, int age) {
super();
this.name = name;
this.age = age;
}
public student() {
super();
}
@Override
public String toString() {
return "student [name=" + name + ", age=" + age + "]";
}
@Override
public int hashCode() {
return this.name.hashCode()+this.age;
}
@Override
public boolean equals(Object obj) {
student stu=(student) obj;
return this.name.equals(stu.name) && this.age ==stu.age;
}
@Override
public int compareTo(student o) {
int num=this.age-o.age;
if(num == 0) {
this.name.compareTo(o.name);
}
return num;
}
}
Results are as follows:
you can see the age is from small to large sort. The second step is completed
import java.util.Comparator;
import java.util.HashMap;
import java.util.Set;
import java.util.TreeMap;
public class HashMapTest {
public static void main(String[] args) {
// HashMap map=new HashMap<>();
TreeMap map=new TreeMap<>(new StuNameComp());
map.put(new student("zs",20), "beijing");
map.put(new student("mz",18), "beijing");
map.put(new student("zl",46), "beijing");
map.put(new student("ww",24), "beijing");
map.put(new student("ls",15), "beijing");
map.put(new student("zs",20), "beijing");//和第一条数据一样用于验证判重是否重复
map.put(new student("zs",80), "beijing");
Set keySet = map.keySet();
for (Object object : keySet) {
System.out.println(object);
}
}
}
//比较器
class StuNameComp implements Comparator<student>{
public int compare(student o1, student o2) {
int num=o1.getName().compareTo(o2.getName());
if(num==0) {
return o1.getAge()-o2.getAge();
}
return num;
}
}
class student implements Comparable<student>{
private String name;
private int age;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public student(String name, int age) {
super();
this.name = name;
this.age = age;
}
public student() {
super();
}
@Override
public String toString() {
return "student [name=" + name + ", age=" + age + "]";
}
@Override
public int hashCode() {
return this.name.hashCode()+this.age;
}
@Override
public boolean equals(Object obj) {
student stu=(student) obj;
return this.name.equals(stu.name) && this.age ==stu.age;
}
@Override
public int compareTo(student o) {
int num=this.age-o.age;
if(num == 0) {
this.name.compareTo(o.name);
}
return num;
}
}
Results are as follows:
you can see is sorted by name; and when the same name is based on age from small to large.
Here in this case is considered completed
2.3 Application of two
requirements: a character string count the number of occurrences, times sorted (e.g.: sdlfjlsjflLjlsjlfsldjfslfljwlfejwlejfl) printed as
a (4) b (9) ... z (7)
Analysis:
1. We need to key characters az as a key, value as the value of the number of times, all you need to create a collection Map
2. string needs to be processed into a character array turn, in order to obtain a set of key map
whether or 3. the judgment is the first time, and then to give the keymap value value value of 1
4. press the print format. So to sort the key
code show as below:
package com.wangcong.map;
import java.util.HashMap;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import java.util.TreeMap;
public class TreeMapTest {
public static void main(String[] args) {
String str="sdlfjlsjflLjlsjlfsldjfslfljwlfejwlejfl";
//定义一个map
Map<Character, Integer> map=new HashMap<>();
//将字符串转为数组
char[] charArray = str.toCharArray();
for (char c : charArray) {
//得到vlaue值
Integer obj=map.get(c);
if(obj==null) {//代表没有出现过,这是第一次出现。
map.put(c, 1);
}else {
map.put(c, ++obj);
}
}
StringBuilder sb=new StringBuilder();
Set<Entry<Character, Integer>> entrySet = map.entrySet();
for (Entry<Character, Integer> entry : entrySet) {
sb.append(entry.getKey()+"("+entry.getValue()+")");
}
System.out.println(sb);
}
}
Results are as follows: As can be seen the results did not show the required
final step: change the HashMap TreeMap
code is as follows:
package com.wangcong.map;
import java.util.HashMap;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import java.util.TreeMap;
public class TreeMapTest {
public static void main(String[] args) {
String str="sdlfjlsjflLjlsjlfsldjfslfljwlfejwlejfl";
//定义一个map
Map<Character, Integer> map=new HashMap<>();
//Map<Character, Integer> map = new TreeMap<>();
//将字符串转为数组
char[] charArray = str.toCharArray();
for (char c : charArray) {
//得到vlaue值
Integer obj=map.get(c);
if(obj==null) {//代表没有出现过,这是第一次出现。
map.put(c, 1);
}else {
map.put(c, ++obj);
}
}
StringBuilder sb=new StringBuilder();
Set<Entry<Character, Integer>> entrySet = map.entrySet();
for (Entry<Character, Integer> entry : entrySet) {
sb.append(entry.getKey()+"("+entry.getValue()+")");
}
System.out.println(sb);
}
}
Results are as follows :( Capital letters before lowercase letters )
3. The frame collection tools (Collections, Arrays)
Simply introduce tools: As the name suggests (that is, a tool, which provides many convenient method)
package com.wangcong.map;
import java.util.Arrays;
public class UtilTest {
public static void main(String[] args) {
int arr[] = {32,453,654,757};
System.out.println(Arrays.toString(arr));
}
}
Output is as follows:
Here simply using a printing method
, if required. Can search Collections, Arrays in api online documentation inside. There are detailed description of the method