Foreword
This article is to read the notes after the "Unit Testing", which is also the company arranged my unit testing training materials, the original is a Powerpoint, it revised down, and do for Visual studio 2005 built-in unit testing of a sort , to devote myself for the purpose of learning and friends need to understand the reading unit testing. Wang pointed out that if wrong.
What is unit testing?
Unit testing is a piece of code developers to write for a small test code under test, it is clear function correctly. Typically, a test unit for judging a specific condition (or scene) acts at a particular function. For example, you might put a lot of value into an ordered list to go, then confirm the value appears in the end of the list. Or, you may remove a pattern matching characters from a string, and then confirm that the string does not contain these characters up.
Unit testing is to prove that the behavior of a piece of code does and developers expect the same.
Why unit test?
When the moment is to prepare, if we assume that the underlying code is correct, then the first high-level code to use the underlying code; then these higher-level code has been used by higher-level code, and so forth. When the basic underlying code no longer reliable, the necessary changes can not be confined to the ground floor. Although you can fix the underlying problem, but these effects modify the underlying code is bound to the higher-level code. Thus, a fix to the underlying code, could lead to a series of changes to almost all the code, so that more and more modifications, more and more complicated. So that the entire project has failed.
The core meaning of unit testing: This simple and effective technique is to make the code more perfect.
What is asserted
Assertion (assert), it is a simple method calls for determining whether a statement is true.
E.g:
public void IsTrue(bool condtion){
if(!condition) abort();
}
Application was:
int a=2;
IsTrue (a == 2);
You can also write more specific data type assertion.
Plan your unit tests
When we write a function that follows, it is used to find the maximum value in the list: static int Largest (int [] list);
I can think of testing as follows:
Entry |
expected results |
7,8,9 |
9 |
8,9,7 |
9 |
9,7,8 |
9 |
7,9,8,9 |
9 |
1 |
1 |
-9,-8,-7 |
-7 |
null |
Exception |
Creating Unit Tests
Right-click a test project in Solution Explorer, or in Visual Studio code editor, right-click to test the namespace, class or method and select "Create a unit test."
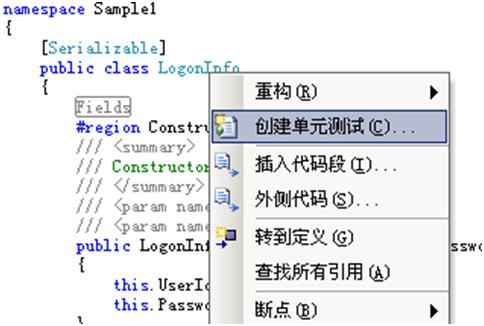
VsUnit various assertions
Assert
In the test method, any number can be called Assert class method as Assert.AreEqual (). Assert class has many methods to choose from, many of which have several overloaded.
CollectionAssert
Use CollectionAssert comparable class object collection, you may also validate a set of one or more states.
StringAssert
Use StringAssert class string comparison. This class contains a variety of useful methods, such as StringAssert.Contains, StringAssert.Matches and StringAssert.StartsWith.
AssertFailedException
As long as the test fails, it will lead to AssertFailedException exception. If the test timeout, causing unexpected exception, or contains the results generated Failed Assert statement, the test fails.
AssertInconclusiveException ( no results)
As long as the test results generated for Inconclusive, will lead AssertInconclusiveException. Typically, the statement added Assert.Inconclusive to the test is still processing may indicate that the test is not yet ready, not run.
UnitTestAssertException
The class when writing new Assert exception classes inherit from the base class UnitTestAssertException, can be more easily identified as an exception rather than the assertion failure caused by accidents or product code from the test abnormalities.
ExpectedExceptionAttribute
If you want to develop code in a method throws an exception, want to use test methods to verify whether it raises an exception, please be modified test method ExpectedExceptionAttribute property in the process.
Such as:
[Test Method]
[ExpectedException(typeof(ArgumentException),
"userID detect the abnormality of NULL.")]
public void NullUserIdInConstructor ()
{
LogonInfo logonInfo = new LogonInfo(null, "P@ss0word");
}
Properties Unit Test
In addition to [Test Method Unit TestMethod ()] [class attributes and their inclusion TestClass than ()] attribute, other attributes may be used to enable a particular unit testing. Among these properties, the most important attributes [ TestInitialize ()] and [ TestCleanup ()]. Using labeled with [ TestInitialize ()] of the method of preparation to be carried out in all aspects of the environment in which unit tests run; The purpose of this is to establish a known state to the running unit tests. For example, you can use [ TestInitialize ()] method to copy, change, or create some data files to be used in the test.
After running a test, you can have [by marking TestCleanup ()] method will return the environment to a known state; this may mean that you want to delete a file folder, or to return a database to a known state. For example, after testing a certain order-entry application method used, the inventory database can be reset to the initial state. In addition, we recommend that you [ TestCleanup ()] or ClassCleanup use cleanup code method, instead of the terminator methods ( ~ Constructor using this code) in. Will not be captured from an abnormal termination is caused by the method, and can lead to unpredictable results.
Calls for the establishment of property order
For assembly:
After the assembly is loaded and before unloading the assembly will be called AssemblyInitialize and AssemblyCleanup .
For the class:
After loading the class and before unloading the class will be called ClassInitialize and ClassCleanup .
For the test method:
Before each test method to load and unload, will call TestInitialize and TestCleanup
What is VsUnit class of TestContext
For information on the current test run attribute memory test context class. For example, TestContext.DataRow TestContext.DataConnection and attribute information contains the test for testing the data driving unit.
other
The test is an attribute of identification and sorting
The test configuration class
Properties for generating reports
Dedicated accessor class
What test: Right-BICEP
Right ---------- results are correct?
B --------------- whether all boundary conditions are correct?
The I ---------------- find out the reverse connection?
C --------------- can use other means to cross-check results?
E --------------- you can force an error condition occurs?
P --------------- meets the performance requirements?
RIGHT: results are correct
If the code to run correctly, how to know that it is correct?
1 , the use of more specific design documents
2 , real-world data
3、????
B: Boundary Conditions
As a variety of special or unexpected situations, testing whether the program can work at least, such as:
l completely forged or inconsistent input data, referred to as "(* @ Q! & # ? ± file.
l malformed data, such as e-mail address malformed
l null value or incomplete
l some of the expected value far from reasonable values, such as age, 10000
l if the requirement is a list repeat value allowed, but pass a list of values is repeated
l requirement is an ordered list, but passed an unordered list
l order of processing is wrong or inconsistent with the desired order. If you do not log into the system to try to print.
B: -correct Boundary Conditions
The Conformance (consistency) and the expected value is consistent
Ordering whether (sequential) value should be as ordered or disordered
The Range (section of) the value of a reasonable range is located
Reference (dependent) code is quoted some resources outside the control code itself
Existence (existence) value exists (if non-empty, non-zero, like in the collection)
The Cardinality (cardinality) if there is just enough value
Time occurs (relative or absolute timing) whether all the things orderly? Whether at the right time? Whether just in time?
I: Check the reverse association
Some results generally reverse logic may be used to verify that they are correct, such as: a * b function is calculated, the following test methods:
Public void UsingInverse () {
double x = MyMath.AB(4,4);
Assert.AreEqual<double>(x,4*4);
}
C: to use other means to achieve cross-check
A plurality of calculation results can be present algorithm, the same algorithm can be used to verify a new stable version improved version, such as:
Public void UsingStd(){
double number = 23214.01;
. double result1 = MyMath Old Method (number1);
. double result2 = MyMath new method (number1);
Assert.AreEqual<double>(result1,result2);
}
E: Forced to generate an error condition
Real operating environment of various unexpected things can happen, such as power outages, broken network, and so on. In the test to simulate these circumstances may be used to achieve the object Mock.
P: Performance Characteristics
Such as data acquisition function, normal collection work on 10 sites, then how to collect its speed on the 1000 website or on more sites? Whether writing unit tests?
How is a good unit test
Should have a good test of quality is: A-TRIP (collectively)
Automatic automation
Thorough thorough
Repeatable Repeatable
Independent independent
Professional Professional
Automatic automation
Call automation
Automated test results
Thorough thorough
All test cases may be a problem, and one extreme, for each line of code, branch code may arrive, each may throw exceptions and so on, can be used as a test subject. At the other extreme, you just test the most likely scenario --- boundary conditions, incomplete and malformed data, and so on. However, these decisions are based on the problem of project requirements.
These they said summed up as "code coverage."
Repeatable Repeatable
Testing should be independent of all other tests, and must be independent of the surrounding environment. Only one goal, that is, the test should be able to run again once in any order, and produce the same results. If the results are different, then there is BUG.
Independent independent
When writing tests to ensure that only tested once thing, but does not mean that only use one Assert a TestMethod, but a test function should focus on the function of a product code, or in combination and provide a common characteristic a set of functions.
Professional Professional
Test code must be written in the same style with the product code. This means that you need to extract and duplicate the common code, and place them in a class function, so that can be reused; stress as the unit test code ------ maintenance package, the DRY principle, reduce coupling.
Mock objects when you need it
l real object has a non-deterministic behavior (producing unpredictable results, such as stock quotes)
l real object is difficult to be created (such as a specific web container)
l Certain behavior is difficult to trigger real object (such as a network error)
l real situation so that running speed is very slow
l real object with a user interface
l test real objects need to ask how it is called (for example, testing may need to verify that a callback function is called)
l real object does not actually exist (and other times when you need the development team, or a new hardware system to deal with, which is a common problem)
Mock three steps objects
1. Prototype
ClassA call the ClassB's Method ()
2. Use an interface to describe the object
ClassA call ClassB through the interface Method
3. For test purposes, to achieve this object interface mock
The End
Reproduced in: https: //www.cnblogs.com/Yjianyong/archive/2011/01/27/1946369.html