This demonstration callback hell:
Analog movie site, to determine whether the user that the user vip URL (the highest authority for the vip)
If vpi to demonstrate vip movie, click the movie show a detailed description of the movie
------------------------------------------------------------------
First look at the finished map:
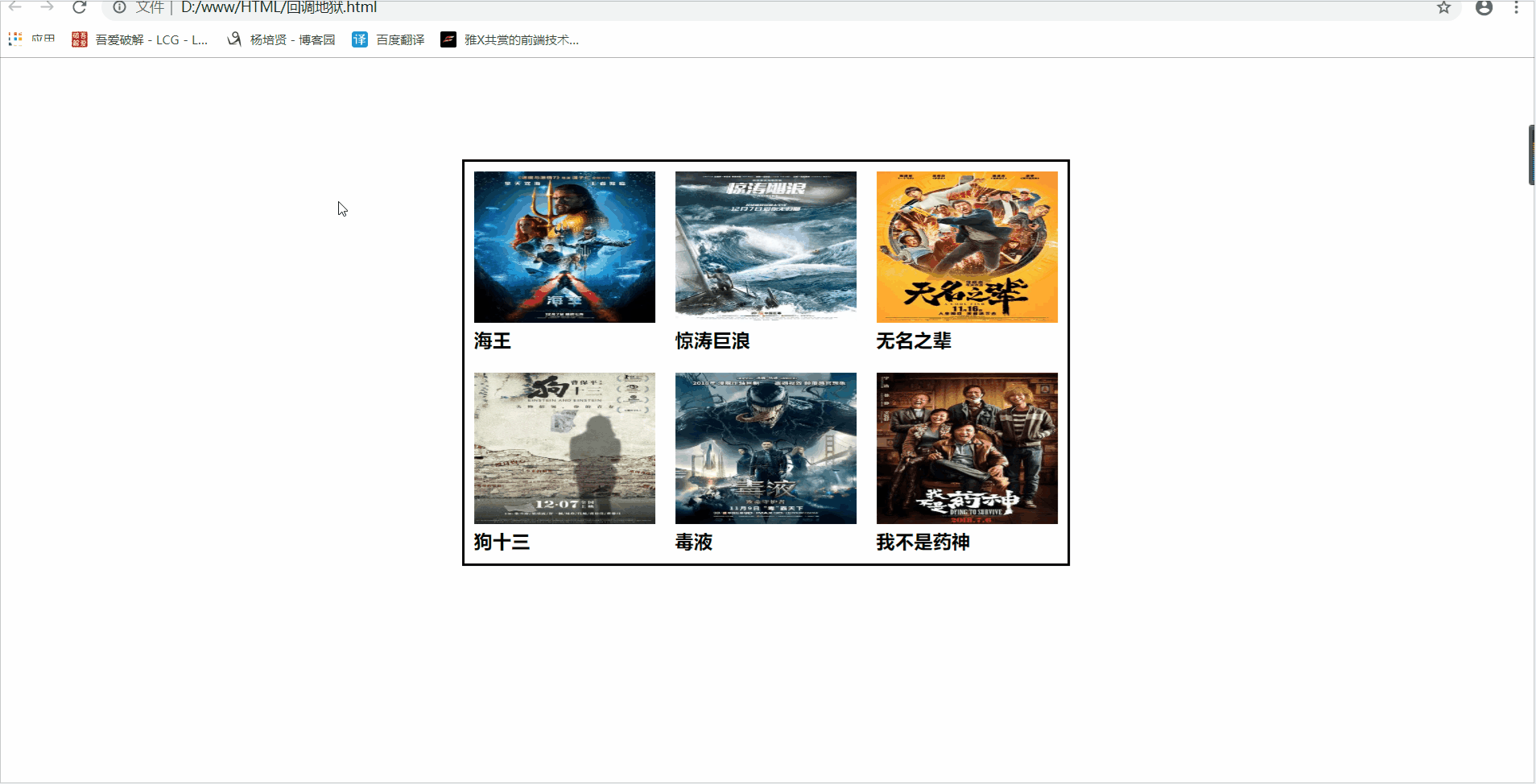
First build a good HTML + CSS structure, as follows:
1 <style> 2 *{ 3 padding: 0px; 4 margin:0px; 5 } 6 .tpl{ 7 display: none; 8 } 9 .wrapper{ 10 overflow: hidden; 11 border:2px solid black; 12 width:600px; 13 margin:100px auto 0px; 14 } 15 .movieSection{ 16 float:left; 17 width:180px; 18 height:180px; 19 padding:10px; 20 } 21 .movieSection img{ 22 width:100%; 23 height:150px; 24 cursor:pointer; 25 } 26 .movieSection h3{ 27 height:30px; 28 } 29 </style> 30 </head> 31 <body> 32 <div class="wrapper"> 33 <div class="tpl"> 34 <img src=""> 35 <h3 class="movieName"></h3> 36 </div> 37 </div>
第一次发送网络请求获取数据,验证是否为vip用户。
root是最高权限,表示为vip用户。
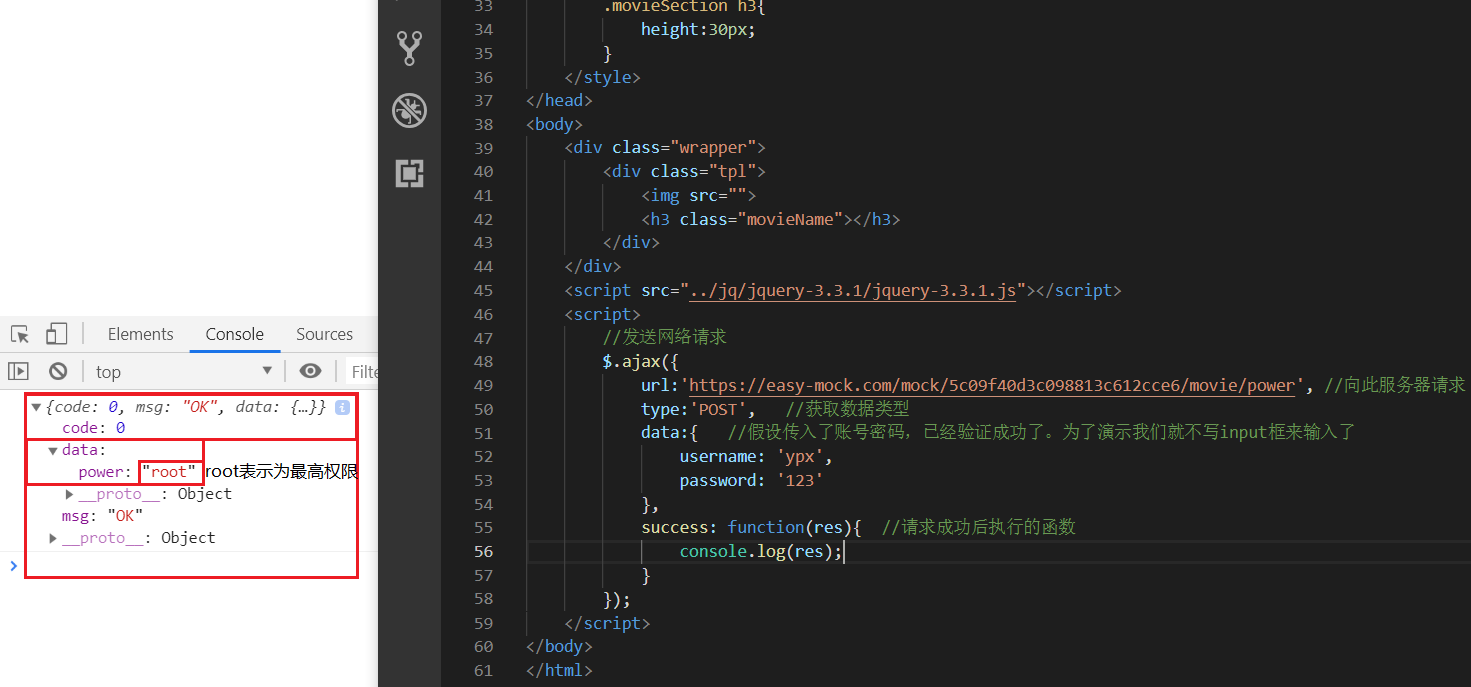
如果是vip用户,就返回出vip电影资源
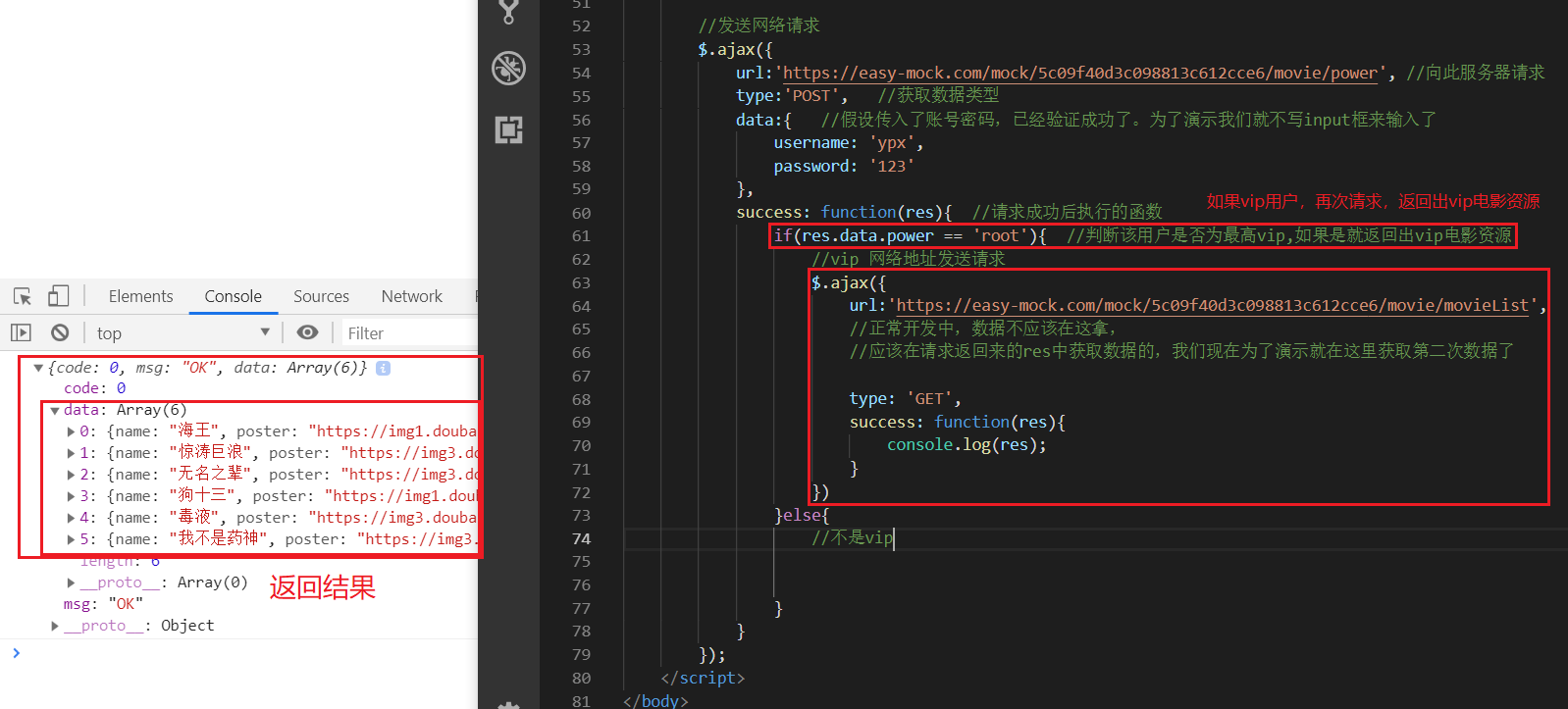
我们现在已经获取到数据了,接下来我们把获取到的数据给可视化出来
发送网络请求获取数据,请求成功然后渲染页面
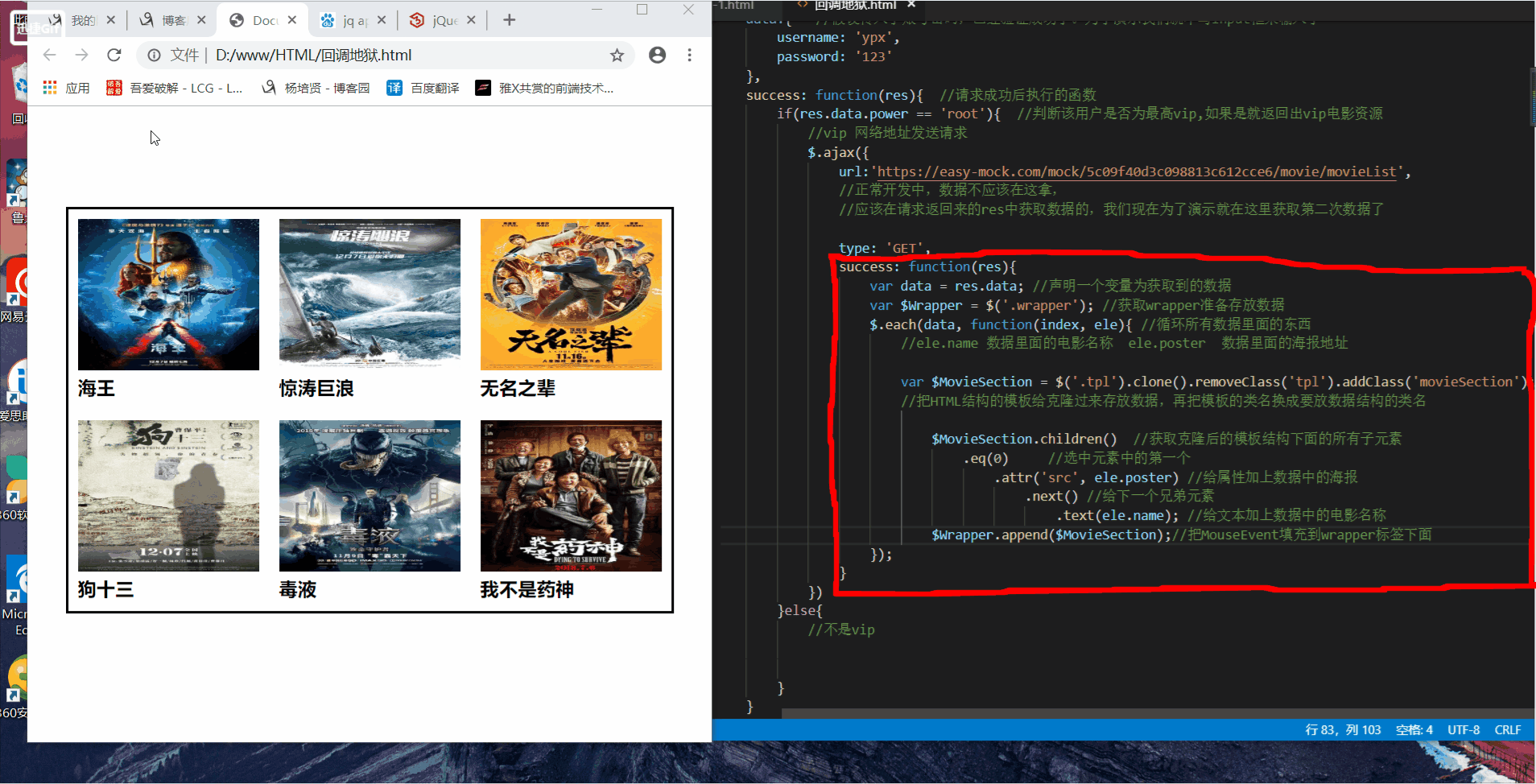
虽然现在我们已经成功获取了数据,但是我们还没有让服务器知道我们需要的是哪部电影,所以要给服务器发送被点击电影的ID,因为ID是只有一个的。
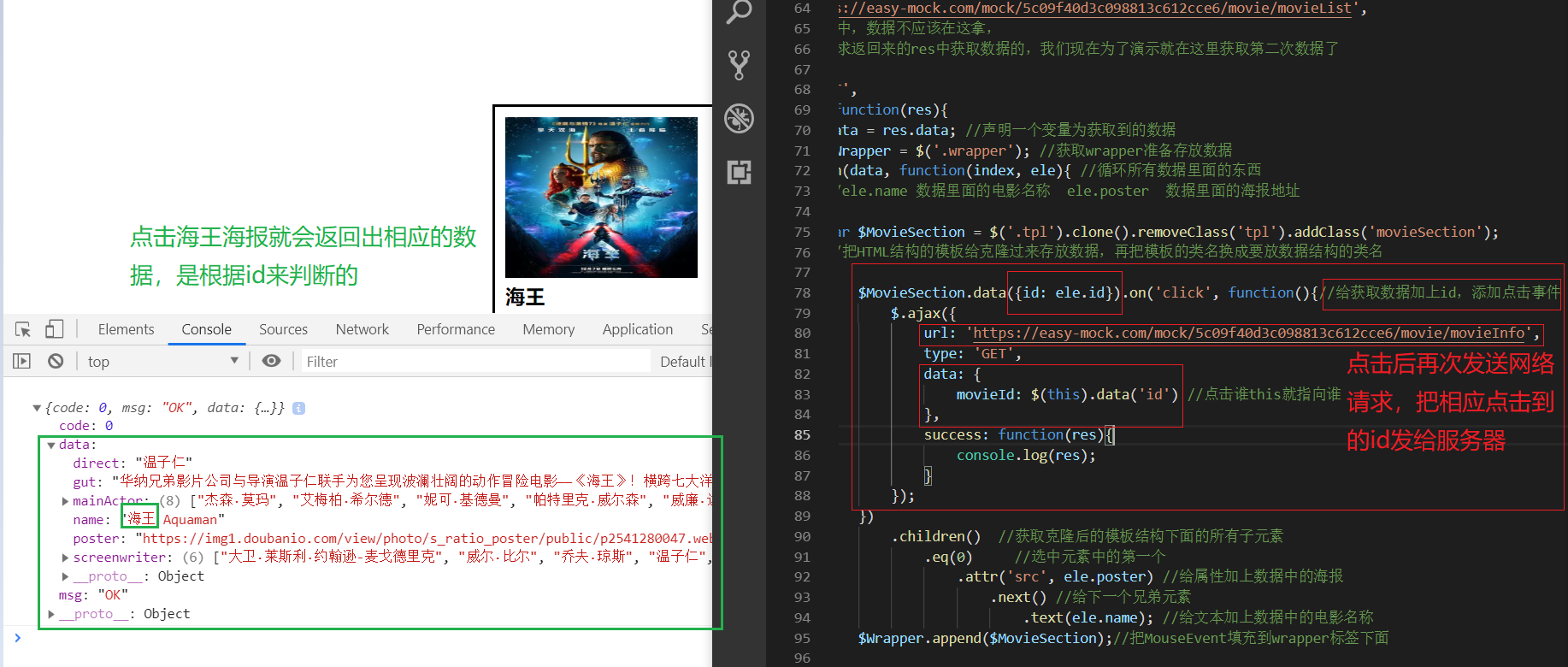
接下来我们再来做一个当点击后,展示出该电影的详情介绍
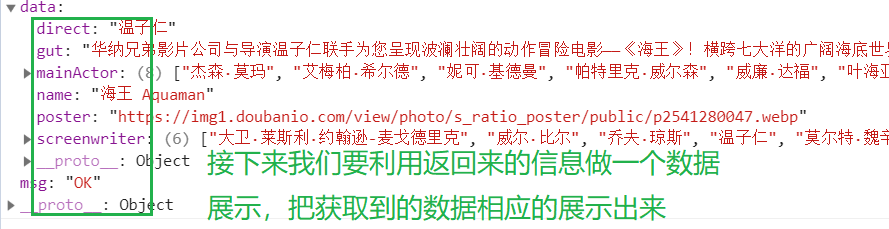
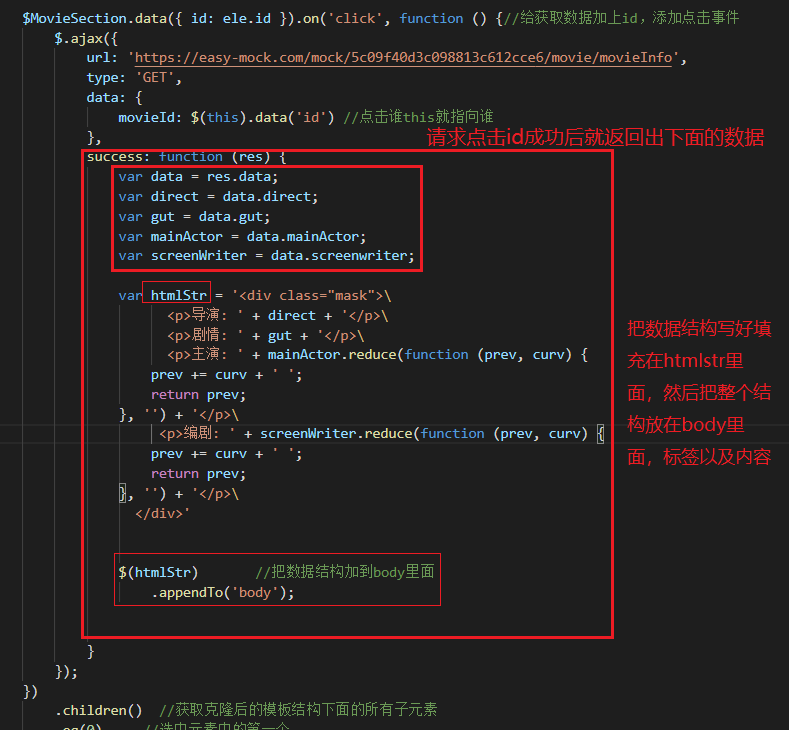
然后把获取到的数据可视化出来
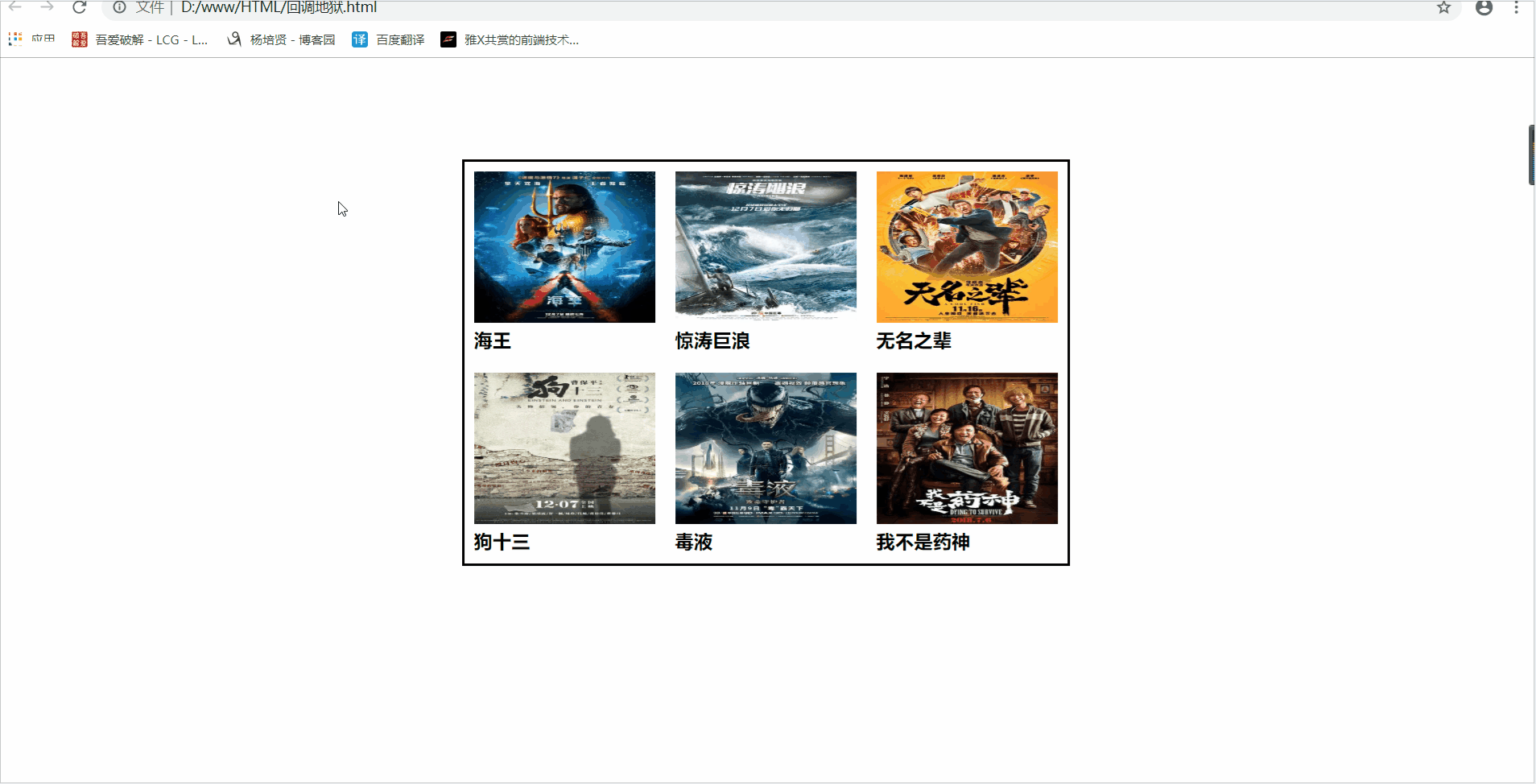
最后附上完整代码:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title>Document</title> <style> * { padding: 0px; margin: 0px; } .tpl { display: none; } .wrapper { overflow: hidden; border: 2px solid black; width: 600px; margin: 100px auto 0px; } .movieSection { float: left; width: 180px; height: 180px; padding: 10px; } .movieSection img { width: 100%; height: 150px; cursor: pointer; } .movieSection h3 { height: 30px; } </style> </head> <body> <div class="wrapper"> <div class="tpl"> <img></img> <h3 class="movieName"></h3> </div> </div> <script src="../jq/jquery-3.3.1/jquery-3.3.1.js"></script> <script> //模拟电影网站,判断用户是否为该网址的vip用户(最高权限为vip) //如果vpi就展示出vip电影,点击相应的电影显示出该电影的详细介绍 //发送网络请求 $.ajax({ url: 'https://easy-mock.com/mock/5c09f40d3c098813c612cce6/movie/power', //向此服务器请求 type: 'POST', //获取数据类型 data: { //假设传入了账号密码,已经验证成功了。为了演示我们就不写input框来输入了 username: 'ypx', password: '123' }, success: function (res) { //请求成功后执行的函数 if (res.data.power == 'root') { //判断该用户是否为最高vip,如果是就返回出vip电影资源 //vip 网络地址发送请求 $.ajax({ url: 'https://easy-mock.com/mock/5c09f40d3c098813c612cce6/movie/movieList', //正常开发中,数据不应该在这拿, //应该在请求返回来的res中获取数据的,我们现在为了演示就在这里获取第二次数据了 type: 'GET', success: function (res) { var data = res.data; //声明一个变量为获取到的数据 var $Wrapper = $('.wrapper'); //获取wrapper准备存放数据 $.each(data, function (index, ele) { //循环所有数据里面的东西 //ele.name 数据里面的电影名称 ele.poster 数据里面的海报地址 var $MovieSection = $('.tpl').clone().removeClass('tpl').addClass('movieSection'); //把HTML结构的模板给克隆过来存放数据,再把模板的类名换成要放数据结构的类名 $MovieSection.data({ id: ele.id }).on('click', function () {//给获取数据加上id,添加点击事件 $.ajax({ url: 'https://easy-mock.com/mock/5c09f40d3c098813c612cce6/movie/movieInfo', type: 'GET', data: { movieId: $(this).data('id') //点击谁this就指向谁 }, success: function (res) { var data = res.data; var direct = data.direct; var gut = data.gut; var mainActor = data.mainActor; var screenWriter = data.screenwriter; var htmlStr = '<div class="mask">\ <p>导演: ' + direct + '</p>\ <p>剧情: ' + gut + '</p>\ <p>主演: ' + mainActor.reduce(function (prev, curv) { prev += curv + ' '; return prev; }, '') + '</p>\ <p>编剧: ' + screenWriter.reduce(function (prev, curv) { prev += curv + ' '; return prev; }, '') + '</p>\ </div>' $(htmlStr) //把数据结构加到body里面 .appendTo('body'); } }); }) .children() //获取克隆后的模板结构下面的所有子元素 .eq(0) //选中元素中的第一个 .attr('src', ele.poster) //给属性加上数据中的海报 .next() //给下一个兄弟元素 .text(ele.name); //给文本加上数据中的电影名称 $Wrapper.append($MovieSection);//把MouseEvent填充到wrapper标签下面 }); } }) } else { //不是vip } } }); </script> </body> </html>
回调地狱不好的地方:可复用性不强。阅读性差也代表可维护性差。扩展性也差。解决方法请移步下一篇文章,jQuery笔记之工具方法—Ajax $.Deferrnd
回调地狱不好的地方:可复用性不强。阅读性差也代表可维护性差。扩展性也差。解决方法请移步下一篇文章,jQuery笔记之工具方法—Ajax $.Deferrnd
回调地狱不好的地方:可复用性不强。阅读性差也代表可维护性差。扩展性也差。解决方法请移步下一篇文章,jQuery笔记之工具方法—Ajax $.Deferrnd